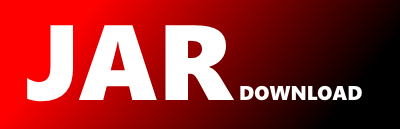
org.helenus.util.Inhibit Maven / Gradle / Ivy
Show all versions of helenus-commons Show documentation
/*
* Copyright (C) 2015-2015 The Helenus Driver Project Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.helenus.util;
import java.lang.reflect.InvocationTargetException;
import java.io.InputStream;
import java.io.Reader;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.concurrent.locks.Lock;
import org.helenus.commons.lang3.ExceptionUtils;
import org.helenus.util.function.E2Runnable;
import org.helenus.util.function.E2Supplier;
import org.helenus.util.function.EConsumer;
import org.helenus.util.function.EFunction;
import org.helenus.util.function.ERunnable;
import org.helenus.util.function.ESupplier;
/**
* The Inhibit
class defines methods that can be used for properly
* inhibiting errors and/or conditions in specific situations based on standard
* algorithms for doing so.
*
* @copyright 2015-2015 The Helenus Driver Project Authors
*
* @author The Helenus Driver Project Authors
* @version 1 - Jan 15, 2015 - paouelle - Creation
*
* @since 2.0
*/
public class Inhibit {
/**
* Invokes {@link Thread#sleep} while inhibiting interruptions while
* propagating them properly for the next level.
*
* Note: If interrupted, the control is returned right away after
* having propagated the interruption via the thread's interrupted flag.
*
* @author paouelle
*
* @param delay the number of milliseconds to sleep for
*/
public static void interruptionsWhileSleeping(long delay) {
try {
Thread.sleep(delay);
} catch (InterruptedException e) {
// propagate interruption
Thread.currentThread().interrupt();
}
}
/**
* Invokes {@link Thread#sleep} while inhibiting interruptions while
* propagating them properly for the next level. The specified exception is
* thrown in place of the interruption.
*
* Note: If interrupted, the control is returned right away via the
* specified exception after having propagated the interruption via the thread's
* interrupted flag.
*
* @author paouelle
*
* @param the type of error to throw in case of interruptions
*
* @param e the exception to throw if interrupted
* @param delay the number of milliseconds to sleep for
* @throws E as e
if an interruption is detected which will be attached
* as the root cause
*/
public static void interruptionsAndThrowOtherWhileSleeping(
long delay, E e
) throws E {
try {
Thread.sleep(delay);
} catch (InterruptedException ie) {
// propagate interruption
Thread.currentThread().interrupt();
// append the interruption to the root cause of the specified exception
throw ExceptionUtils.withRootCause(e, ie);
}
}
/**
* Invokes {@link Lock#tryLock} while inhibiting Java interruptions
* and propagating them after.
*
* Note: If interrupted, the control is returned right away after
* having propagated the interruption properly and false
is
* returned.
*
* @author paouelle
*
* @param lock the lock to try to acquire
* @param time the maximum time to wait for the lock
* @param unit the units of time
* @return true
if the lock was acquired and false
* if the waiting time elapsed before the lock was acquired or if the
* thread was interrupted
* @throws NullPointerException if lock
is null
*/
public static final boolean interruptionsWhileTryingLock(
Lock lock, long time, TimeUnit unit
) {
org.apache.commons.lang3.Validate.notNull(lock, "invalid null lock");
try {
return lock.tryLock(time, unit);
} catch (InterruptedException e) {
// propagate interruption
Thread.currentThread().interrupt();
return false;
}
}
/**
* Invokes {@link Object#wait} while inhibiting Java interruptions
* and propagating them after.
*
* Note: If interrupted, the control is returned right away after
* having propagated the interruption properly and null
is
* returned.
*
* @author paouelle
*
* @param obj the object to wait for a notification
* @throws NullPointerException if obj
is null
*/
public static final void interruptionsWhileWaitingForNotificationFrom(
Object obj
) {
org.apache.commons.lang3.Validate.notNull(obj, "invalid null object");
try {
synchronized (obj) {
obj.wait();
}
} catch (InterruptedException e) {
// propagate interruption
Thread.currentThread().interrupt();
}
}
/**
* Invokes {@link Future#get} while inhibiting Java interruptions
* and propagating them after.
*
* Note: If interrupted, the control is returned right away after
* having propagated the interruption properly and null
is
* returned.
*
* @author paouelle
*
* @param the type of result computed by the future
*
* @param future the future to try to get
* @return the computed result if the future returned and null
* if the waiting time elapsed before the future returned or if the
* thread was interrupted
* @throws ExecutionException if the computation threw an exception
* @throws NullPointerException if future
is null
*/
public static final T interruptionsWhileWaitingFor(
Future future
) throws ExecutionException {
org.apache.commons.lang3.Validate.notNull(future, "invalid null future");
try {
return future.get();
} catch (InterruptedException e) {
// propagate interruption
Thread.currentThread().interrupt();
return null;
}
}
/**
* Invokes {@link Future#get} while inhibiting Java interruptions
* and propagating them after.
*
* Note: If interrupted, the control is returned right away after
* having propagated the interruption properly and null
is
* returned.
*
* @author paouelle
*
* @param the type of result computed by the future
*
* @param future the future to try to get
* @param time the maximum time to wait for the future
* @param unit the units of time
* @return the computed result if the future returned and null
* if the waiting time elapsed before the future returned or if the
* thread was interrupted
* @throws ExecutionException if the computation threw an exception
* @throws TimeoutException if the wait timed out
* @throws NullPointerException if future
is null
*/
public static final T interruptionsWhileWaitingFor(
Future future, long time, TimeUnit unit
) throws ExecutionException, TimeoutException {
org.apache.commons.lang3.Validate.notNull(future, "invalid null future");
try {
return future.get(time, unit);
} catch (InterruptedException e) {
// propagate interruption
Thread.currentThread().interrupt();
return null;
}
}
/**
* Invokes the specified operation while inhibiting Java interruptions and
* propagating them after.
*
* Interruptions from the operation are handled by calling the handle function
* and then propagated properly.
*
* Note: Any runtime exceptions or errors thrown out of the
* handle function will be automatically thrown out.
*
* @author paouelle
*
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to inhibit interruptions for
* @param handle the non-null
function to handle any interruptions
* that occurs when calling the operation
* @return the result returned by c
or by handle
if
* an interruption occurs
* @throws E if an error occurs while executing the operation
* @throws RuntimeException if cmd
throws a runtime exception
* @throws Error if cmd
throws an error
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final T interruptionsAndReturn(
E2Supplier cmd,
EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle function");
try {
return cmd.get();
} catch (InterruptedException e) {
try {
return handle.apply(e);
} finally {
// propagate interruption
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes the specified command while inhibiting Java interruptions and
* propagating them after.
*
* Interruptions from the operation are handled by calling the handle consumer
* and then propagated properly.
*
* Note: Any runtime exceptions or errors thrown out of the
* handle consumer will be automatically thrown out.
*
* @author paouelle
*
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to inhibit interruptions for
* @param handle the non-null
consumer to handle any interruptions
* that occurs when calling the operation
* @throws E if an error occurs while executing the operation
* @throws RuntimeException if cmd
throws a runtime exception
* @throws Error if cmd
throws an error
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void interruptions(
E2Runnable cmd,
EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle consumer");
try {
cmd.run();
} catch (InterruptedException e) {
try {
handle.accept(e);
} finally {
// propagate interruption
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes the specified operation while inhibiting Java exceptions and
* interruptions and propagating the interruptions properly after; runtime
* exceptions are still propagated as expected.
*
* Non-runtime exceptions from the operation are handled by calling
* the handle function.
*
* Note: Any runtime exceptions or errors thrown out of the
* handle function will be automatically thrown out.
*
* @author paouelle
*
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to inhibit interruptions and
* exceptions for
* @param handle the non-null
function to handle any exceptions
* that occurs when calling the operation
* @return the result returned by cmd
or from the handle
* if a non runtime exception or an interruption occurs
* @throws E if thrown by the handle function
* @throws RuntimeException if cmd
or handle
throws
* a runtime exception
* @throws Error if cmd
or handle
throws an error
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final T interruptionsAndExceptionsAndReturn(
ESupplier cmd, EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle function");
try {
return cmd.get();
} catch (InterruptedException e) {
try {
return handle.apply(e);
} finally {
// propagate interruption
Thread.currentThread().interrupt();
}
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
return handle.apply(e);
}
}
/**
* Invokes the specified command while inhibiting Java exceptions and
* interruptions and propagating the interruptions properly after; runtime
* exceptions are still propagated as expected.
*
* Non-runtime exceptions from the operation are handled by calling
* the handle consumer.
*
* Note: Any runtime exceptions or errors thrown out of the
* handle consumer will be automatically thrown out.
*
* @author paouelle
*
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
command to inhibit interruptions and
* exceptions for
* @param handle the non-null
consumer to handle any exceptions
* that occurs when calling the operation
* @throws E if thrown by the handle function
* @throws RuntimeException if cmd
or handle
throws
* a runtime exception
* @throws Error if cmd
or handle
throws an error
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void interruptionsAndExceptions(
ERunnable cmd, EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle consumer");
try {
cmd.run();
} catch (InterruptedException e) {
try {
handle.accept(e);
} finally {
// propagate interruption
Thread.currentThread().interrupt();
}
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
handle.accept(e);
}
}
/**
* Invoked the specified operation object while inhibiting Java interruptions
* and propagating them after. This version of the method will retry calling
* the operation if an interruption occurs.
*
* @author paouelle
*
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to inhibit interruptions for
* @return the result returned by cmd
* @throws E if an error occurs while executing the operation
* @throws RuntimeException if cmd
throws a runtime exception
* @throws Error if cmd
throws an error
* @throws NullPointerException if cmd
is null
*/
public static final T interruptionsWhileRetryingAndReturn(
E2Supplier cmd
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
boolean interrupted = false;
try {
while (true) {
try {
return cmd.get();
} catch (InterruptedException e) {
// remember and continue;
interrupted = true;
}
}
} finally {
if (interrupted) {
// propagate interruptions
Thread.currentThread().interrupt();
}
}
}
/**
* Invoked the specified command object while inhibiting Java interruptions
* and propagating them after. This version of the method will retry calling
* the operation if an interruption occurs.
*
* @author paouelle
*
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
command to inhibit interruptions for
* @throws E if an error occurs while executing the command
* @throws RuntimeException if cmd
throws a runtime exception
* @throws Error if cmd
throws an error
* @throws NullPointerException if cmd
is null
*/
public static final void interruptionsWhileRetrying(
E2Runnable cmd
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
boolean interrupted = false;
try {
while (true) {
try {
cmd.run();
} catch (InterruptedException e) {
// remember and continue;
interrupted = true;
}
}
} finally {
if (interrupted) {
// propagate interruptions
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes {@link Lock#tryLock} while inhibiting Java interruptions
* and propagating them after. This version of the method will
* retry trying the lock if an interruption occurs.
*
* @author paouelle
*
* @param lock the lock to try to acquire
* @param time the maximum time to wait for the lock
* @param unit the units of time
* @return true
if the lock was acquired and false
* if the waiting time elapsed before the lock was acquired or if the
* thread was interrupted
* @throws NullPointerException if lock
is null
*/
public static final boolean interruptionsWhileRetryingTryingLock(
Lock lock, long time, TimeUnit unit
) {
long end = System.nanoTime() + unit.toNanos(time);
boolean interrupted = false;
try {
while (true) {
long nanos = end - System.nanoTime();
if (nanos < 0L) { // no more time left
return false;
}
try {
return lock.tryLock(nanos, TimeUnit.NANOSECONDS);
} catch (InterruptedException e) {
// remember and continue;
interrupted = true;
}
}
} finally {
if (interrupted) {
// propagate interruptions
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes {@link Future#get} while inhibiting Java interruptions
* and propagating them after. This version of the method will
* retry waiting for the future result if an interruption occurs.
*
* @author paouelle
*
* @param the type of result computed by the future
*
* @param future the future to try to get
* @return the computed result if the future returned and null
* if the waiting time elapsed before the future returned or if the
* thread was interrupted
* @throws ExecutionException if the computation threw an exception
* @throws NullPointerException if future
is null
*/
public static final T interruptionsWhileRetryingWaitingFor(
Future future
) throws ExecutionException {
org.apache.commons.lang3.Validate.notNull(future, "invalid null future");
boolean interrupted = false;
try {
while (true) {
try {
return future.get();
} catch (InterruptedException e) {
// remember and continue;
interrupted = true;
}
}
} finally {
if (interrupted) {
// propagate interruptions
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes {@link Future#get} while inhibiting Java interruptions
* and propagating them after. This version of the method will
* retry waiting for the future result if an interruption occurs.
*
* @author paouelle
*
* @param the type of result computed by the future
*
* @param future the future to try to get
* @param time the maximum time to wait for the future
* @param unit the units of time
* @return the computed result if the future returned and null
* if the waiting time elapsed before the future returned or if the
* thread was interrupted
* @throws ExecutionException if the computation threw an exception
* @throws TimeoutException if the wait timed out
* @throws NullPointerException if future
is null
*/
public static final T interruptionsWhileRetryingWaitingFor(
Future future, long time, TimeUnit unit
) throws ExecutionException, TimeoutException {
org.apache.commons.lang3.Validate.notNull(future, "invalid null future");
long end = System.nanoTime() + unit.toNanos(time);
boolean interrupted = false;
try {
while (true) {
long nanos = end - System.nanoTime();
if (nanos < 0L) { // no more time left
throw new TimeoutException();
}
try {
return future.get(nanos, TimeUnit.NANOSECONDS);
} catch (InterruptedException e) {
// remember and continue;
interrupted = true;
}
}
} finally {
if (interrupted) {
// propagate interruptions
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes {@link Object#wait} while inhibiting Java interruptions
* and propagating them after. This version of the method will
* retry waiting for notifications from the object if an interruption occurs.
*
* @author paouelle
*
* @param obj the object to wait for a notification
* @throws NullPointerException if obj
is null
*/
public static final void interruptionsWhileRetryingWaitingForNotificationFrom(
Object obj
) {
org.apache.commons.lang3.Validate.notNull(obj, "invalid null object");
boolean interrupted = false;
try {
synchronized (obj) {
while (true) {
try {
obj.wait();
return;
} catch (InterruptedException e) {
// remember and continue;
interrupted = true;
}
}
}
} finally {
if (interrupted) {
// propagate interruption
Thread.currentThread().interrupt();
}
}
}
/**
* Invokes the specified operation while inhibiting Java exceptions and
* interruptions and propagating the interruptions properly after. This
* version of the method will retry executing the operation if an interruption
* occurs.
*
* Non-runtime exceptions from the operation are handled by calling
* the handle function.
*
* Note: Any runtime exceptions or errors thrown out of the
* handle function will be automatically thrown out.
*
* @author paouelle
*
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to inhibit interruptions
* and exceptions for
* @param handle the non-null
function to handle any exceptions
* that occurs when calling the operation
* @return the result returned by cmd
or from the handle
* if a non runtime exception occurs
* @throws E if thrown by the handle function
* @throws RuntimeException if cmd
or handle
throws
* a runtime exception
* @throws Error if cmd
or handle
throws an error
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final T interruptionsWhileRetryingAndExceptionsAndReturn(
ESupplier cmd, EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle function");
try {
return Inhibit.interruptionsWhileRetryingAndReturn(
() -> cmd.get()
);
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
return handle.apply(e);
}
}
/**
* Invokes the specified command while inhibiting Java exceptions and
* interruptions and propagating the interruptions properly after. This
* version of the method will retry executing the operation if an interruption
* occurs.
*
* Non-runtime exceptions from the operation are handled by calling
* the handle consumer.
*
* Note: Any runtime exceptions or errors thrown out of the
* handle consumer will be automatically thrown out.
*
* @author paouelle
*
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
command to inhibit interruptions
* and exceptions for
* @param handle the non-null
consumer to handle any exceptions
* that occurs when calling the operation
* @throws E if thrown by the handle consumer
* @throws RuntimeException if cmd
or handle
throws
* a runtime exception
* @throws Error if cmd
or handle
throws an error
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void interruptionsWhileRetryingAndExceptions(
ERunnable cmd, EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle consumer");
try {
Inhibit.interruptionsWhileRetrying(
() -> cmd.run()
);
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
handle.accept(e);
}
}
/**
* Inhibits exceptions while closing the specified reader.
*
* @author paouelle
*
* @param r the reader to be closed
*/
public static final void exceptionsWhileClosing(Reader r) {
if (r != null) {
try {
r.close();
} catch (Exception e) { // ignore all exception
}
}
}
/**
* Inhibits exceptions while closing the specified input stream.
*
* @author paouelle
*
* @param is the input stream to be closed
*/
public static final void exceptionsWhileClosing(InputStream is) {
if (is != null) {
try {
is.close();
} catch (Exception e) { // ignore all exception
}
}
}
/**
* Invokes the specified operation while inhibiting Java errors and
* exceptions. This is done in a controlled way where:
*
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the operation are handled by calling
* the handle function.
*
* @author paouelle
*
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to execute and inhibit
* errors and exceptions for
* @param handle the non-null
function to handle any other exceptions
* or errors that occurs when calling the operation
* @return the result returned by cmd
or handle
* @throws E if thrown by the handle function
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final T throwablesAndReturn(
ESupplier cmd, EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle function");
try {
return cmd.get();
} catch (OutOfMemoryError e) {
throw e;
} catch (StackOverflowError e) {
throw e;
} catch (AssertionError e) {
throw e;
} catch (ThreadDeath t) {
throw t;
} catch (Throwable t) {
return handle.apply(t);
}
}
/**
* Invokes the specified command while inhibiting Java errors and
* exceptions. This is done in a controlled way where:
*
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the command are handled by calling
* the handle consumer.
*
* @author paouelle
*
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
command to execute and inhibit
* errors and exceptions for
* @param handle the non-null
consumer to handle any other exceptions
* or errors that occurs when calling the operation
* @throws E if thrown by the handle consumer
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void throwables(
ERunnable cmd, EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle consumer");
try {
cmd.run();
} catch (OutOfMemoryError e) {
throw e;
} catch (StackOverflowError e) {
throw e;
} catch (AssertionError e) {
throw e;
} catch (ThreadDeath t) {
throw t;
} catch (Throwable t) {
handle.accept(t);
}
}
/**
* Invokes the specified command while inhibiting Java errors and
* exceptions. This is done in a controlled way where:
*
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions are simply ignored.
*
* @author paouelle
*
* @param cmd the non-null
command to execute and inhibit
* errors and exceptions for
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void throwables(ERunnable cmd) {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
try {
cmd.run();
} catch (OutOfMemoryError e) {
throw e;
} catch (StackOverflowError e) {
throw e;
} catch (AssertionError e) {
throw e;
} catch (ThreadDeath t) {
throw t;
} catch (Throwable t) {
}
}
/**
* Invokes the specified function while inhibiting Java errors and
* exceptions. This is done in a controlled way where:
*
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the operation are handled by calling
* the handle function.
*
* @author paouelle
*
* @param the type of argument passed to cmd
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param arg the argument to pass to the function
* @param cmd the non-null
function to execute and inhibit
* errors and exceptions for
* @param handle the non-null
function to handle any other exceptions
* or errors that occurs when calling the operation
* @return the result returned by cmd
or handle
* @throws E if thrown by the handle function
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final R throwablesAndReturn(
T arg, EFunction cmd, EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle function");
try {
return cmd.apply(arg);
} catch (OutOfMemoryError e) {
throw e;
} catch (StackOverflowError e) {
throw e;
} catch (AssertionError e) {
throw e;
} catch (ThreadDeath t) {
throw t;
} catch (Throwable t) {
return handle.apply(t);
}
}
/**
* Invokes the specified consumer while inhibiting Java errors and
* exceptions. This is done in a controlled way where:
*
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the consumer are handled by calling
* the handle consumer.
*
* @author paouelle
*
* @param the type of argument passed to cmd
* @param the type of exceptions that can be thrown out
*
* @param arg the argument to pass to the consumer
* @param cmd the non-null
consumer to execute and inhibit
* errors and exceptions for
* @param handle the non-null
consumer to handle any other exceptions
* or errors that occurs when calling the consumer
* @throws E if thrown by the handle consumer
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void throwables(
T arg, EConsumer cmd, EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null consumer");
org.apache.commons.lang3.Validate.notNull(handle, "invalid null handle consumer");
try {
cmd.accept(arg);
} catch (OutOfMemoryError e) {
throw e;
} catch (StackOverflowError e) {
throw e;
} catch (AssertionError e) {
throw e;
} catch (ThreadDeath t) {
throw t;
} catch (Throwable t) {
handle.accept(t);
}
}
/**
* Invokes the specified operation while inhibiting Java errors, exceptions,
* and unwrapping target exceptions. This is done in a controlled way where:
*
* - {@link InvocationTargetException} are first unwrapped by calling
* {@link InvocationTargetException#getTargetException}.
* - {@link ExceptionInInitializerError} are first unwrapped by calling
* {@link ExceptionInInitializerError#getException()}.
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the operation are handled by calling
* the handle function.
*
* @author paouelle
*
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
operation to execute and inhibit
* errors and exceptions for
* @param handle the non-null
function to handle any other exceptions
* or errors that occurs when calling the operation
* @return the result returned by cmd
or handle
* @throws E if thrown by the handle function
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final T unwrappedThrowablesAndReturn(
ESupplier cmd, EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
return Inhibit.throwablesAndReturn(
() -> {
try {
return cmd.get();
} catch (InvocationTargetException e) {
final Throwable te = e.getTargetException();
throw (te != null) ? te : e;
} catch (ExceptionInInitializerError e) {
final Throwable te = e.getException();
throw (te != null) ? te : e;
}
},
handle
);
}
/**
* Invokes the specified command while inhibiting Java errors, exceptions,
* and unwrapping target exceptions. This is done in a controlled way where:
*
* - {@link InvocationTargetException} are first unwrapped by calling
* {@link InvocationTargetException#getTargetException}.
* - {@link ExceptionInInitializerError} are first unwrapped by calling
* {@link ExceptionInInitializerError#getException()}.
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the command are handled by calling
* the handle consumer.
*
* @author paouelle
*
* @param the type of exceptions that can be thrown out
*
* @param cmd the non-null
command to execute and inhibit
* errors and exceptions for
* @param handle the non-null
consumer to handle any other exceptions
* or errors that occurs when calling the operation
* @throws E if thrown by the handle consumer
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void unwrappedThrowables(
ERunnable cmd, EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null command");
Inhibit.unwrappedThrowables(
() -> {
try {
cmd.run();
} catch (InvocationTargetException e) {
final Throwable te = e.getTargetException();
throw (te != null) ? te : e;
} catch (ExceptionInInitializerError e) {
final Throwable te = e.getException();
throw (te != null) ? te : e;
}
},
handle
);
}
/**
* Invokes the specified function while inhibiting Java errors, exceptions,
* and unwrapping target exceptions. This is done in a controlled way where:
*
* - {@link InvocationTargetException} are first unwrapped by calling
* {@link InvocationTargetException#getTargetException}.
* - {@link ExceptionInInitializerError} are first unwrapped by calling
* {@link ExceptionInInitializerError#getException()}.
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the operation are handled by calling
* the handle function.
*
* @author paouelle
*
* @param the type of argument passed to cmd
* @param the type of result returned by cmd
* @param the type of exceptions that can be thrown out
*
* @param arg the argument to pass to the function
* @param cmd the non-null
function to execute and inhibit
* errors and exceptions for
* @param handle the non-null
function to handle any other exceptions
* or errors that occurs when calling the operation
* @return the result returned by cmd
or handle
* @throws E if thrown by the handle function
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final R unwrappedThrowablesAndReturn(
T arg, EFunction cmd, EFunction handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null operation");
return Inhibit.throwablesAndReturn(
arg,
a -> {
try {
return cmd.apply(a);
} catch (InvocationTargetException e) {
final Throwable te = e.getTargetException();
throw (te != null) ? te : e;
} catch (ExceptionInInitializerError e) {
final Throwable te = e.getException();
throw (te != null) ? te : e;
}
},
handle
);
}
/**
* Invokes the specified consumer while inhibiting Java errors, exceptions,
* and unwrapping target exceptions. This is done in a controlled way where:
*
* - {@link InvocationTargetException} are first unwrapped by calling
* {@link InvocationTargetException#getTargetException}.
* - {@link ExceptionInInitializerError} are first unwrapped by calling
* {@link ExceptionInInitializerError#getException()}.
* - {@link OutOfMemoryError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. Attempting to do anything
* else can cause additional memory issues and corruption.
* - {@link StackOverflowError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up.
* - {@link AssertionError} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up so JUnit test case can
* stopped when an assertion fails.
* - {@link ThreadDeath} are not intercepted; nor are they logged. These
* are thrown back as they should propagate up. These errors are part of Java's
* mechanism for stopping threads.
*
* All other errors and exceptions from the consumer are handled by calling
* the handle consumer.
*
* @author paouelle
*
* @param the type of argument passed to cmd
* @param the type of exceptions that can be thrown out
*
* @param arg the argument to pass to the consumer
* @param cmd the non-null
consumer to execute and inhibit
* errors and exceptions for
* @param handle the non-null
consumer to handle any other exceptions
* or errors that occurs when calling the consumer
* @throws E if thrown by the handle consumer
* @throws NullPointerException if cmd
or handle
is
* null
*/
public static final void unwrappedThrowables(
T arg, EConsumer cmd, EConsumer handle
) throws E {
org.apache.commons.lang3.Validate.notNull(cmd, "invalid null consumer");
Inhibit.throwables(
arg,
a -> {
try {
cmd.accept(a);
} catch (InvocationTargetException e) {
final Throwable te = e.getTargetException();
throw (te != null) ? te : e;
} catch (ExceptionInInitializerError e) {
final Throwable te = e.getException();
throw (te != null) ? te : e;
}
},
handle
);
}
/**
* Prevents instantiation.
*
* @author paouelle
*/
private Inhibit() {}
}