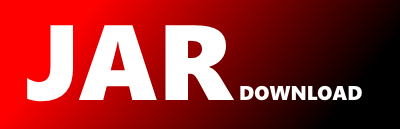
org.helenus.driver.junit.StatementCaptureListMatchers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helenus-junit Show documentation
Show all versions of helenus-junit Show documentation
JPA-like syntax for annotating POJO classes for persistence via Cassandra's Java driver - JUnit Framework
/*
* Copyright (C) 2015-2015 The Helenus Driver Project Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.helenus.driver.junit;
import org.hamcrest.BaseMatcher;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.StringDescription;
import org.helenus.driver.GenericStatement;
/**
* The StatementCaptureListMatchers
abstract class defines static
* factory method for a statement capture list.
*
* @copyright 2015-2015 The Helenus Driver Project Authors
*
* @author The Helenus Driver Project Authors
* @version 1 - Jun 30, 2015 - paouelle - Creation
*
* @since 1.0
*/
public abstract class StatementCaptureListMatchers {
/**
* Asserts that the given matcher matches the actual value.
*
* @author paouelle
*
* @param the type accepted by the matcher
*
* @param actual the value to match against
* @param matcher the matcher
* @throws AssertionError if the matcher doesn't match the actual value
*/
static void assertThat(T actual, Matcher matcher) {
StatementCaptureListMatchers.assertThat("", actual, matcher);
}
/**
* Asserts that the given matcher matches the actual value.
*
* @author paouelle
*
* @param the type accepted by the matcher
*
* @param reason the additional information about the error
* @param actual the value to match against
* @param matcher the matcher
* @throws AssertionError if the matcher doesn't match the actual value
*/
static void assertThat(String reason, T actual, Matcher matcher) {
if (!matcher.matches(actual)) {
final Description description = new StringDescription();
if (!reason.isEmpty()) {
description.appendText(reason).appendText("\n");
}
description
.appendText("Expected: ")
.appendDescriptionOf(matcher)
.appendText("\n but: ");
matcher.describeMismatch(actual, description);
throw new AssertionError(description.toString());
}
}
/**
* Accesses the size assertions with a Hamcrest match for asserting the
* the number of captured statements for a statement capture list.
*
* @author paouelle
*
* @param the type of statements captured
*
* @param matcher a matcher for the size expected for the statement capture
* list
* @return the corresponding statement matcher
*/
@SuppressWarnings("rawtypes")
public static StatementCaptureListMatcher size(
Matcher matcher
) {
return new StatementCaptureListMatcher() {
@Override
public void match(StatementCaptureList list) throws Exception {
StatementCaptureListMatchers.assertThat(
"Size", list.size(), matcher
);
}
};
}
/**
* Creates a matcher that matches if the captured list is empty.
*
* @param the type of statements captured
*
* @return a corresponding matcher
*/
@SuppressWarnings("rawtypes")
public static Matcher> isEmpty() {
return new BaseMatcher>() {
@SuppressWarnings("unchecked")
@Override
public boolean matches(java.lang.Object obj) {
return ((StatementCaptureList)obj).isEmpty();
}
@Override
public void describeMismatch(java.lang.Object obj, Description description) {
description.appendText("not empty");
}
@Override
public void describeTo(Description description) {
description.appendValue("is empty");
}
};
}
/**
* Creates a matcher that matches if the captured list is not empty.
*
* @param the type of statements captured
*
* @return a corresponding matcher
*/
@SuppressWarnings("rawtypes")
public static Matcher> isNotEmpty() {
return new BaseMatcher>() {
@SuppressWarnings("unchecked")
@Override
public boolean matches(java.lang.Object obj) {
return !((StatementCaptureList)obj).isEmpty();
}
@Override
public void describeMismatch(java.lang.Object obj, Description description) {
description.appendText("empty");
}
@Override
public void describeTo(Description description) {
description.appendValue("is not empty");
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy