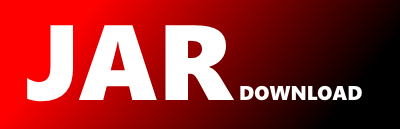
org.hepeng.commons.service.ServiceErrorLoader Maven / Gradle / Ivy
The newest version!
package org.hepeng.commons.service;
import org.apache.commons.lang3.StringUtils;
import org.hepeng.commons.exception.ApplicationRuntimeException;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
/**
* @author he peng
*/
public class ServiceErrorLoader {
private static final String SERVICE_ERROR_FILE_PATH_KEY = "hp.java.commons.service.error";
private static final String DEFAULT_SERVICE_ERROR_FILE_PATH = "META-INF/default-service-error.properties";
private static final Properties SERVICE_ERROR_PROPS = new Properties();
static {
String serviceErrorFilePath = System.getProperty(SERVICE_ERROR_FILE_PATH_KEY);
if (StringUtils.isBlank(serviceErrorFilePath)) {
serviceErrorFilePath = DEFAULT_SERVICE_ERROR_FILE_PATH;
}
InputStream inputStream = Thread.currentThread().getContextClassLoader().getResourceAsStream(serviceErrorFilePath);
try {
SERVICE_ERROR_PROPS.load(inputStream);
} catch (IOException e) {
throw new ApplicationRuntimeException("load " + serviceErrorFilePath + " file error" , e);
}
}
private ServiceErrorLoader() {}
/**
* 加载一个 ServiceError 实例
* @param name 配置文件中表示一个 {@link ServiceError} 配置的名称
* @return 返回一个 {@link ServiceError} 实例对象
*/
public static ServiceError loadServiceError(String name) {
return newServiceError(SERVICE_ERROR_PROPS.getProperty(name));
}
/**
* 创建一个新的 {@link ServiceError} 的实例对象.
* @param serviceError
* @return 返回一个 {@link ServiceError} 实例对象
*/
private static ServiceError newServiceError(String serviceError) {
if (StringUtils.isBlank(serviceError) || StringUtils.containsNone(serviceError , ",")) {
return null;
}
String[] serviceErrorArr = serviceError.split(",");
if (serviceErrorArr.length < 2) {
return null;
}
return new ServiceError() {
private Integer errorCode;
private String errorMsg;
{
this.errorCode = Integer.valueOf(serviceErrorArr[0]);
this.errorMsg = serviceErrorArr[1];
}
@Override
public Integer getErrorCode() {
return this.errorCode;
}
@Override
public String getErrorMsg() {
return this.errorMsg;
}
@Override
public String toString() {
return "ServiceError: Error Code [" + this.errorCode + "] , Error Msg [" + this.errorMsg + "]";
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy