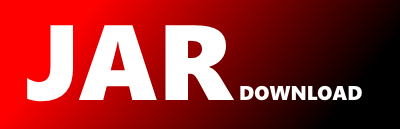
herddb.jdbc.HerdDBDatabaseMetadata Maven / Gradle / Ivy
/*
Licensed to Diennea S.r.l. under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. Diennea S.r.l. licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package herddb.jdbc;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import herddb.client.ScanResultSetMetadata;
import herddb.client.impl.EmptyScanResultSet;
import herddb.client.impl.MapListScanResultSet;
import herddb.model.TransactionContext;
import herddb.utils.SQLUtils;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.RowIdLifetime;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Database Metadata Implementation
*
* @author enrico.olivelli
*/
public class HerdDBDatabaseMetadata implements DatabaseMetaData {
private final HerdDBConnection con;
private final String tableSpace;
HerdDBDatabaseMetadata(HerdDBConnection con, String tableSpace) {
this.con = con;
this.tableSpace = tableSpace;
}
@Override
public boolean allProceduresAreCallable() throws SQLException {
return false;
}
@Override
public boolean allTablesAreSelectable() throws SQLException {
return true;
}
@Override
public String getURL() throws SQLException {
return "";
}
@Override
public String getUserName() throws SQLException {
return "";
}
@Override
public boolean isReadOnly() throws SQLException {
return false;
}
@Override
public boolean nullsAreSortedHigh() throws SQLException {
return false;
}
@Override
public boolean nullsAreSortedLow() throws SQLException {
return true;
}
@Override
public boolean nullsAreSortedAtStart() throws SQLException {
return false;
}
@Override
public boolean nullsAreSortedAtEnd() throws SQLException {
return true;
}
@Override
public String getDatabaseProductName() throws SQLException {
return "HerdDB";
}
@Override
public String getDatabaseProductVersion() throws SQLException {
return "";
}
@Override
public String getDriverName() throws SQLException {
return "HerdDB";
}
@Override
public String getDriverVersion() throws SQLException {
return "0.0";
}
@Override
public int getDriverMajorVersion() {
return 0;
}
@Override
public int getDriverMinorVersion() {
return 0;
}
@Override
public boolean usesLocalFiles() throws SQLException {
return false;
}
@Override
public boolean usesLocalFilePerTable() throws SQLException {
return false;
}
@Override
public boolean supportsMixedCaseIdentifiers() throws SQLException {
return true;
}
@Override
public boolean storesUpperCaseIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesLowerCaseIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesMixedCaseIdentifiers() throws SQLException {
return true;
}
@Override
public boolean supportsMixedCaseQuotedIdentifiers() throws SQLException {
return true;
}
@Override
public boolean storesUpperCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesLowerCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesMixedCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public String getIdentifierQuoteString() throws SQLException {
return "'";
}
@Override
public String getSQLKeywords() throws SQLException {
return "";
}
@Override
public String getNumericFunctions() throws SQLException {
return "";
}
@Override
public String getStringFunctions() throws SQLException {
return "";
}
@Override
public String getSystemFunctions() throws SQLException {
return "";
}
@Override
public String getTimeDateFunctions() throws SQLException {
return "";
}
@Override
public String getSearchStringEscape() throws SQLException {
return "\\";
}
@Override
public String getExtraNameCharacters() throws SQLException {
return "";
}
@Override
public boolean supportsAlterTableWithAddColumn() throws SQLException {
return true;
}
@Override
public boolean supportsAlterTableWithDropColumn() throws SQLException {
return true;
}
@Override
public boolean supportsColumnAliasing() throws SQLException {
return true;
}
@Override
public boolean nullPlusNonNullIsNull() throws SQLException {
return true;
}
@Override
public boolean supportsConvert() throws SQLException {
return false;
}
@Override
public boolean supportsConvert(int fromType, int toType) throws SQLException {
return false;
}
@Override
public boolean supportsTableCorrelationNames() throws SQLException {
return false;
}
@Override
public boolean supportsDifferentTableCorrelationNames() throws SQLException {
return false;
}
@Override
public boolean supportsExpressionsInOrderBy() throws SQLException {
return false;
}
@Override
public boolean supportsOrderByUnrelated() throws SQLException {
return false;
}
@Override
public boolean supportsGroupBy() throws SQLException {
return true;
}
@Override
public boolean supportsGroupByUnrelated() throws SQLException {
return false;
}
@Override
public boolean supportsGroupByBeyondSelect() throws SQLException {
return false;
}
@Override
public boolean supportsLikeEscapeClause() throws SQLException {
return false;
}
@Override
public boolean supportsMultipleResultSets() throws SQLException {
return false;
}
@Override
public boolean supportsMultipleTransactions() throws SQLException {
return false;
}
@Override
public boolean supportsNonNullableColumns() throws SQLException {
return false;
}
@Override
public boolean supportsMinimumSQLGrammar() throws SQLException {
return true;
}
@Override
public boolean supportsCoreSQLGrammar() throws SQLException {
return false;
}
@Override
public boolean supportsExtendedSQLGrammar() throws SQLException {
return false;
}
@Override
public boolean supportsANSI92EntryLevelSQL() throws SQLException {
return true;
}
@Override
public boolean supportsANSI92IntermediateSQL() throws SQLException {
return false;
}
@Override
public boolean supportsANSI92FullSQL() throws SQLException {
return false;
}
@Override
public boolean supportsIntegrityEnhancementFacility() throws SQLException {
return false;
}
@Override
public boolean supportsOuterJoins() throws SQLException {
return true;
}
@Override
public boolean supportsFullOuterJoins() throws SQLException {
return false;
}
@Override
public boolean supportsLimitedOuterJoins() throws SQLException {
return false;
}
@Override
public String getSchemaTerm() throws SQLException {
return "tablespace";
}
@Override
public String getProcedureTerm() throws SQLException {
return "procedure";
}
@Override
public String getCatalogTerm() throws SQLException {
return "catalog";
}
@Override
public boolean isCatalogAtStart() throws SQLException {
return true;
}
@Override
public String getCatalogSeparator() throws SQLException {
return ".";
}
@Override
public boolean supportsSchemasInDataManipulation() throws SQLException {
return true;
}
@Override
public boolean supportsSchemasInProcedureCalls() throws SQLException {
return false;
}
@Override
public boolean supportsSchemasInTableDefinitions() throws SQLException {
return true;
}
@Override
public boolean supportsSchemasInIndexDefinitions() throws SQLException {
return true;
}
@Override
public boolean supportsSchemasInPrivilegeDefinitions() throws SQLException {
return false;
}
@Override
public boolean supportsCatalogsInDataManipulation() throws SQLException {
return true;
}
@Override
public boolean supportsCatalogsInProcedureCalls() throws SQLException {
return false;
}
@Override
public boolean supportsCatalogsInTableDefinitions() throws SQLException {
return false;
}
@Override
public boolean supportsCatalogsInIndexDefinitions() throws SQLException {
return false;
}
@Override
public boolean supportsCatalogsInPrivilegeDefinitions() throws SQLException {
return false;
}
@Override
public boolean supportsPositionedDelete() throws SQLException {
return false;
}
@Override
public boolean supportsPositionedUpdate() throws SQLException {
return false;
}
@Override
public boolean supportsSelectForUpdate() throws SQLException {
return false;
}
@Override
public boolean supportsStoredProcedures() throws SQLException {
return false;
}
@Override
public boolean supportsSubqueriesInComparisons() throws SQLException {
return false;
}
@Override
public boolean supportsSubqueriesInExists() throws SQLException {
return true;
}
@Override
public boolean supportsSubqueriesInIns() throws SQLException {
return true;
}
@Override
public boolean supportsSubqueriesInQuantifieds() throws SQLException {
return false;
}
@Override
public boolean supportsCorrelatedSubqueries() throws SQLException {
return true;
}
@Override
public boolean supportsUnion() throws SQLException {
return true;
}
@Override
public boolean supportsUnionAll() throws SQLException {
return true;
}
@Override
public boolean supportsOpenCursorsAcrossCommit() throws SQLException {
return false;
}
@Override
public boolean supportsOpenCursorsAcrossRollback() throws SQLException {
return false;
}
@Override
public boolean supportsOpenStatementsAcrossCommit() throws SQLException {
return true;
}
@Override
public boolean supportsOpenStatementsAcrossRollback() throws SQLException {
return true;
}
@Override
public int getMaxBinaryLiteralLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxCharLiteralLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxColumnNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxColumnsInGroupBy() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxColumnsInIndex() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxColumnsInOrderBy() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxColumnsInSelect() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxColumnsInTable() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxConnections() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxCursorNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxIndexLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxSchemaNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxProcedureNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxCatalogNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxRowSize() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public boolean doesMaxRowSizeIncludeBlobs() throws SQLException {
return true;
}
@Override
public int getMaxStatementLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxStatements() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxTableNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxTablesInSelect() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getMaxUserNameLength() throws SQLException {
return Integer.MAX_VALUE;
}
@Override
public int getDefaultTransactionIsolation() throws SQLException {
return Connection.TRANSACTION_READ_COMMITTED;
}
@Override
public boolean supportsTransactions() throws SQLException {
return true;
}
@Override
public boolean supportsTransactionIsolationLevel(int level) throws SQLException {
switch (level) {
case Connection.TRANSACTION_READ_COMMITTED:
return true;
default:
return false;
}
}
@Override
public boolean supportsDataDefinitionAndDataManipulationTransactions() throws SQLException {
return false;
}
@Override
public boolean supportsDataManipulationTransactionsOnly() throws SQLException {
return false;
}
@Override
public boolean dataDefinitionCausesTransactionCommit() throws SQLException {
return false;
}
@Override
public boolean dataDefinitionIgnoredInTransactions() throws SQLException {
return false;
}
@Override
public ResultSet getProcedures(String catalog, String schemaPattern, String procedureNamePattern) throws SQLException {
return new HerdDBResultSet(new EmptyScanResultSet(TransactionContext.NOTRANSACTION_ID));
}
@Override
public ResultSet getProcedureColumns(String catalog, String schemaPattern, String procedureNamePattern, String columnNamePattern) throws SQLException {
return new HerdDBResultSet(new EmptyScanResultSet(TransactionContext.NOTRANSACTION_ID));
}
private static final String[] GET_TABLES_SCHEMA = new String[]{
"TABLE_CAT",
"TABLE_SCHEM",
"TABLE_NAME",
"TABLE_TYPE",
"REMARKS",
"TYPE_CAT",
"TYPE_SCHEM",
"TYPE_NAME",
"SELF_REFERENCING_COL_NAME",
"REF_GENERATION"
};
private static final String[] GET_INDEXES_SCHEMA = new String[]{
"TABLE_CAT",
"TABLE_SCHEM",
"TABLE_NAME",
"NON_UNIQUE",
"INDEX_QUALIFIER",
"INDEX_NAME",
"TYPE",
"ORDINAL_POSITION",
"COLUMN_NAME",
"ASC_OR_DESC",
"CARDINALITY",
"FILTER_CONDITION"
};
@Override
/**
* Retrieves a description of the tables available in the given catalog.
* Only table descriptions matching the catalog, schema, table name and type
* criteria are returned. They are ordered by TABLE_TYPE
,
* TABLE_CAT
, TABLE_SCHEM
and
* TABLE_NAME
.
*
* Each table description has the following columns:
*
* - TABLE_CAT String {@code =>} table catalog (may be
*
null
)
* - TABLE_SCHEM String {@code =>} table schema (may be
*
null
)
* - TABLE_NAME String {@code =>} table name
*
- TABLE_TYPE String {@code =>} table type. Typical types are
* "TABLE", "VIEW", "SYSTEM TABLE", "GLOBAL TEMPORARY", "LOCAL TEMPORARY",
* "ALIAS", "SYNONYM".
*
- REMARKS String {@code =>} explanatory comment on the table
*
- TYPE_CAT String {@code =>} the types catalog (may be
*
null
)
* - TYPE_SCHEM String {@code =>} the types schema (may be
*
null
)
* - TYPE_NAME String {@code =>} type name (may be
*
null
)
* - SELF_REFERENCING_COL_NAME String {@code =>} name of the
* designated "identifier" column of a typed table (may be
*
null
)
* - REF_GENERATION String {@code =>} specifies how values in
* SELF_REFERENCING_COL_NAME are created. Values are "SYSTEM", "USER",
* "DERIVED". (may be
null
)
*
*
*
* Note: Some databases may not return information for all tables.
*
* @param catalog a catalog name; must match the catalog name as it is
* stored in the database; "" retrieves those without a catalog;
* null
means that the catalog name should not be used to
* narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as
* it is stored in the database; "" retrieves those without a schema;
* null
means that the schema name should not be used to narrow
* the search
* @param tableNamePattern a table name pattern; must match the table name
* as it is stored in the database
* @param types a list of table types, which must be from the list of table
* types returned from {@link #getTableTypes},to include; null
* returns all types
* @return ResultSet
- each row is a table description
* @exception SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
@SuppressFBWarnings("SQL_NONCONSTANT_STRING_PASSED_TO_EXECUTE")
public ResultSet getTables(String catalog, String schemaPattern, String tableNamePattern, String[] types) throws SQLException {
String query = "SELECT table_name FROM SYSTABLES";
if (tableNamePattern != null && !tableNamePattern.isEmpty()) {
query = query + " WHERE table_name LIKE '" + SQLUtils.escape(tableNamePattern) + "'";
}
try (Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(query)) {
List