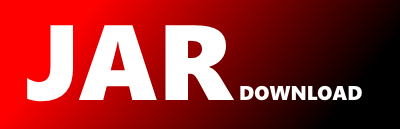
org.hertsstack.codegen.CacheGenCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of herts-codegen Show documentation
Show all versions of herts-codegen Show documentation
Herts API Client generator for Codegen
package org.hertsstack.codegen;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
class CacheGenCode {
private final Map customStructure = new HashMap<>();
private List generatedPackageNames = new ArrayList<>();
private static CacheGenCode thisClass;
private CacheGenCode() {}
public static CacheGenCode getInstance() {
if (thisClass != null) {
return thisClass;
}
thisClass = new CacheGenCode();
return thisClass;
}
public void addGeneratedPackageNames(String packageName) {
this.generatedPackageNames.add(packageName);
this.generatedPackageNames = this.generatedPackageNames.stream()
.distinct()
.collect(Collectors.toList());
}
public List getGeneratedPackageNames() {
return this.generatedPackageNames;
}
public void removeCustomStructure(String packageName) {
this.customStructure.remove(packageName);
}
public void setCustomStructure(String packageName, CustomStructure customStructure) {
this.customStructure.put(packageName, customStructure);
}
public CustomStructure getCustomStructure(String packageName) {
return this.customStructure.get(packageName);
}
public static class CustomStructure {
private List parameterTypeNames;
private String returnTypeName;
public CustomStructure(List parameterTypeNames, String returnTypeName) {
this.parameterTypeNames = parameterTypeNames;
this.returnTypeName = returnTypeName;
}
public void setParameterTypeNames(List parameterTypeNames) {
this.parameterTypeNames = parameterTypeNames;
}
public void setReturnTypeName(String returnTypeName) {
this.returnTypeName = returnTypeName;
}
public List getParameterTypeNames() {
return parameterTypeNames;
}
public String getReturnTypeName() {
return returnTypeName;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy