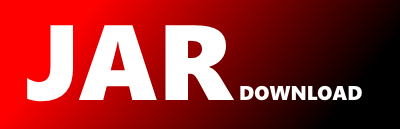
org.hibernate.search.engine.environment.bean.BeanReference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Hibernate Search engine, always required
/*
* SPDX-License-Identifier: Apache-2.0
* Copyright Red Hat Inc. and Hibernate Authors
*/
package org.hibernate.search.engine.environment.bean;
import org.hibernate.search.util.common.impl.StringHelper;
/**
* A reference to a bean, allowing the retrieval of that bean
* when {@link #resolve(BeanResolver) passed} a {@link BeanResolver}.
*
* @param The type of the referenced bean.
*/
public interface BeanReference {
/**
* Resolve this reference into a bean using the given resolver.
*
* @param beanResolver A resolver to resolve this reference with.
* @return The bean instance.
*/
BeanHolder resolve(BeanResolver beanResolver);
/**
* Cast this reference into a reference whose {@link #resolve(BeanResolver)} method is is guaranteed to
* either fail or return an instance of the given type.
*
* @param expectedType The expected bean type.
* @param The expected bean type.
* @return A bean reference.
* @throws ClassCastException If this reference is certain to never return an instance of the given type.
*/
default BeanReference extends U> asSubTypeOf(Class expectedType) {
return new CastingBeanReference<>( this, expectedType );
}
/**
* Create a {@link BeanReference} referencing a bean by its type only.
*
* @param type The bean type. Must not be null.
* @param The bean type.
* @return The corresponding {@link BeanReference}.
*/
static BeanReference of(Class type) {
return of( type, BeanRetrieval.ANY );
}
/**
* Create a {@link BeanReference} referencing a bean by its type only.
*
* @param type The bean type. Must not be null.
* @param retrieval How to retrieve the bean. See {@link BeanRetrieval}.
* @param The bean type.
* @return The corresponding {@link BeanReference}.
*/
static BeanReference of(Class type, BeanRetrieval retrieval) {
return new TypeBeanReference<>( type, retrieval );
}
/**
* Create a {@link BeanReference} referencing a bean by type and name.
*
* @param type The bean type. Must not be null.
* @param name The bean name. May be null or empty.
* @param The bean type.
* @return The corresponding {@link BeanReference}.
*/
static BeanReference of(Class type, String name) {
return of( type, name, BeanRetrieval.ANY );
}
/**
* Create a {@link BeanReference} referencing a bean by type and name.
*
* @param type The bean type. Must not be null.
* @param name The bean name. May be null or empty.
* @param retrieval How to retrieve the bean. See {@link BeanRetrieval}.
* @param The bean type.
* @return The corresponding {@link BeanReference}.
*/
static BeanReference of(Class type, String name, BeanRetrieval retrieval) {
if ( StringHelper.isNotEmpty( name ) ) {
return new TypeAndNameBeanReference<>( type, name, retrieval );
}
else {
return new TypeBeanReference<>( type, retrieval );
}
}
/**
* Create a {@link BeanReference} referencing a bean instance directly.
*
* @param instance The bean instance. Must not be null.
* @param The bean type.
* @return The corresponding {@link BeanReference}.
*/
static BeanReference ofInstance(T instance) {
return new InstanceBeanReference<>( instance );
}
// This method conforms to the MicroProfile Config specification. Do not change its signature.
static BeanReference> parse(String value) {
return BeanReference.parse( Object.class, value );
}
static BeanReference parse(Class expectedType, String value) {
return BeanReferences.parse( expectedType, value );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy