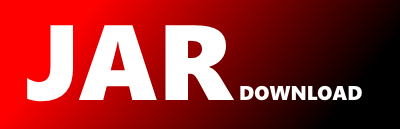
org.hibernate.search.mapper.javabean.mapping.SearchMappingBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-mapper-javabean Show documentation
Show all versions of hibernate-search-mapper-javabean Show documentation
Hibernate Search Mapper for POJOs following the JavaBean conventions
The newest version!
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.mapper.javabean.mapping;
import java.lang.invoke.MethodHandles;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.hibernate.search.engine.cfg.spi.ConfigurationPropertyChecker;
import org.hibernate.search.engine.cfg.spi.ConfigurationPropertySource;
import org.hibernate.search.engine.common.spi.SearchIntegration;
import org.hibernate.search.engine.common.spi.SearchIntegrationBuilder;
import org.hibernate.search.engine.common.spi.SearchIntegrationFinalizer;
import org.hibernate.search.engine.common.spi.SearchIntegrationPartialBuildState;
import org.hibernate.search.engine.environment.bean.BeanReference;
import org.hibernate.search.mapper.javabean.impl.JavaBeanMappingInitiator;
import org.hibernate.search.mapper.javabean.mapping.impl.JavaBeanMapping;
import org.hibernate.search.mapper.javabean.mapping.impl.JavaBeanMappingKey;
import org.hibernate.search.mapper.javabean.model.impl.JavaBeanBootstrapIntrospector;
import org.hibernate.search.mapper.javabean.session.SearchSession;
import org.hibernate.search.mapper.pojo.bridge.IdentifierBridge;
import org.hibernate.search.mapper.pojo.extractor.ContainerExtractorConfigurationContext;
import org.hibernate.search.mapper.pojo.mapping.definition.annotation.AnnotationMappingConfigurationContext;
import org.hibernate.search.mapper.pojo.mapping.definition.programmatic.ProgrammaticMappingConfigurationContext;
import org.hibernate.search.util.common.impl.SuppressingCloser;
public final class SearchMappingBuilder {
private static ConfigurationPropertySource getPropertySource(Map properties,
ConfigurationPropertyChecker propertyChecker) {
return propertyChecker.wrap( ConfigurationPropertySource.fromMap( properties ) );
}
private final ConfigurationPropertyChecker propertyChecker;
private final Map properties = new HashMap<>();
private final ConfigurationPropertySource propertySource;
private final SearchIntegrationBuilder integrationBuilder;
private final JavaBeanMappingKey mappingKey;
private final JavaBeanMappingInitiator mappingInitiator;
SearchMappingBuilder(MethodHandles.Lookup lookup) {
propertyChecker = ConfigurationPropertyChecker.create();
propertySource = getPropertySource( properties, propertyChecker );
integrationBuilder = SearchIntegration.builder( propertySource, propertyChecker );
JavaBeanBootstrapIntrospector introspector = JavaBeanBootstrapIntrospector.create( lookup );
mappingKey = new JavaBeanMappingKey();
mappingInitiator = new JavaBeanMappingInitiator( introspector );
integrationBuilder.addMappingInitiator( mappingKey, mappingInitiator );
// Enable annotated type discovery by default
mappingInitiator.setAnnotatedTypeDiscoveryEnabled( true );
}
public ProgrammaticMappingConfigurationContext programmaticMapping() {
return mappingInitiator.programmaticMapping();
}
public AnnotationMappingConfigurationContext annotationMapping() {
return mappingInitiator.annotationMapping();
}
public ContainerExtractorConfigurationContext containerExtractors() {
return mappingInitiator.containerExtractors();
}
/**
* Register a type as an entity type with the default name, its simple class name.
* @param type The type to be considered as an entity type, i.e. a type that may be indexed
* and whose instances be added/updated/deleted through the {@link SearchSession#indexingPlan() indexing plan}.
* @return {@code this}, for call chaining.
*/
public SearchMappingBuilder addEntityType(Class> type) {
return addEntityType( type, type.getSimpleName() );
}
/**
* Register a type as an entity type with the given name.
* @param type The type to be considered as an entity type, i.e. a type that may be indexed
* and whose instances be added/updated/deleted through the {@link SearchSession#indexingPlan() indexing plan}.
* @param entityName The name of the entity.
* @return {@code this}, for call chaining.
*/
public SearchMappingBuilder addEntityType(Class> type, String entityName) {
mappingInitiator.addEntityType( type, entityName );
return this;
}
/**
* @param types The types to be considered as entity types, i.e. types that may be indexed
* and whose instances be added/updated/deleted through the {@link SearchSession#indexingPlan() indexing plan}.
* @return {@code this}, for call chaining.
*/
public SearchMappingBuilder addEntityTypes(Set> types) {
for ( Class> type : types ) {
addEntityType( type );
}
return this;
}
public SearchMappingBuilder setMultiTenancyEnabled(boolean multiTenancyEnabled) {
mappingInitiator.setMultiTenancyEnabled( multiTenancyEnabled );
return this;
}
public SearchMappingBuilder setProvidedIdentifierBridge(BeanReference extends IdentifierBridge
© 2015 - 2024 Weber Informatics LLC | Privacy Policy