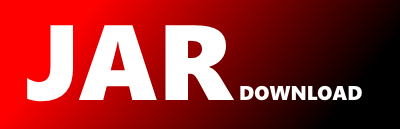
org.hibernate.search.util.AnalyzerUtils Maven / Gradle / Ivy
Show all versions of hibernate-search-v5migrationhelper-engine Show documentation
/*
* SPDX-License-Identifier: Apache-2.0
* Copyright Red Hat Inc. and Hibernate Authors
*/
package org.hibernate.search.util;
import java.io.IOException;
import java.io.StringReader;
import java.lang.invoke.MethodHandles;
import java.util.ArrayList;
import java.util.List;
import org.hibernate.search.util.logging.impl.LoggerFactory;
import org.hibernate.search.util.logging.impl.MigrationHelperLog;
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.TokenStream;
import org.apache.lucene.analysis.tokenattributes.CharTermAttribute;
/**
* Helper class to hide boilerplate code when using Lucene Analyzers.
*
* Taken and modified from Lucene in Action.
*
* @author Hardy Ferentschik
* @deprecated Will be removed without replacement.
*/
@Deprecated
public final class AnalyzerUtils {
private AnalyzerUtils() {
//not allowed
}
public static final MigrationHelperLog log = LoggerFactory.make( MethodHandles.lookup() );
public static List tokenizedTermValues(Analyzer analyzer, String field, String text) throws IOException {
final List tokenList = new ArrayList();
final TokenStream stream = analyzer.tokenStream( field, new StringReader( text ) );
try {
CharTermAttribute term = stream.addAttribute( CharTermAttribute.class );
stream.reset();
while ( stream.incrementToken() ) {
String s = new String( term.buffer(), 0, term.length() );
tokenList.add( s );
}
stream.end();
}
finally {
stream.close();
}
return tokenList;
}
}