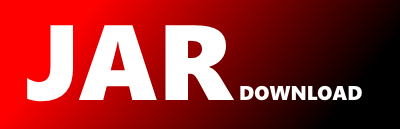
org.hibernate.cache.infinispan.util.VersionedEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-infinispan
Show all versions of hibernate-infinispan
A module of the Hibernate Core project
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.cache.infinispan.util;
import org.infinispan.commons.marshall.AdvancedExternalizer;
import org.infinispan.filter.Converter;
import org.infinispan.filter.KeyValueFilter;
import org.infinispan.metadata.Metadata;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Collections;
import java.util.Set;
/**
* @author Radim Vansa <[email protected]>
*/
public class VersionedEntry {
public static final ExcludeEmptyFilter EXCLUDE_EMPTY_EXTRACT_VALUE = new ExcludeEmptyFilter();
private final Object value;
private final Object version;
private final long timestamp;
public VersionedEntry(Object value, Object version, long timestamp) {
this.value = value;
this.version = version;
this.timestamp = timestamp;
}
public Object getValue() {
return value;
}
public Object getVersion() {
return version;
}
public long getTimestamp() {
return timestamp;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("VersionedEntry{");
sb.append("value=").append(value);
sb.append(", version=").append(version);
sb.append(", timestamp=").append(timestamp);
sb.append('}');
return sb.toString();
}
private static class ExcludeEmptyFilter implements KeyValueFilter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy