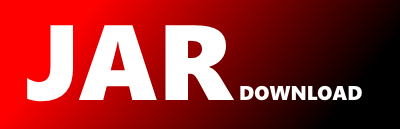
org.hibernate.search.bridge.builtin.impl.NullEncodingTwoWayFieldBridge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Core of the Object/Lucene mapper, query engine and index management
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.bridge.builtin.impl;
import org.apache.lucene.document.Document;
import org.apache.lucene.index.IndexableField;
import org.apache.lucene.search.Query;
import org.hibernate.search.bridge.LuceneOptions;
import org.hibernate.search.bridge.TwoWayFieldBridge;
import org.hibernate.search.bridge.spi.NullMarker;
import org.hibernate.search.bridge.util.impl.BridgeAdaptor;
import org.hibernate.search.bridge.util.impl.BridgeAdaptorUtils;
import org.hibernate.search.engine.nulls.codec.impl.NullMarkerCodec;
import org.hibernate.search.util.logging.impl.Log;
import org.hibernate.search.util.logging.impl.LoggerFactory;
import java.lang.invoke.MethodHandles;
/**
* @author Hardy Ferentschik
*/
public class NullEncodingTwoWayFieldBridge implements TwoWayFieldBridge, BridgeAdaptor {
private static final Log LOG = LoggerFactory.make( MethodHandles.lookup() );
private final TwoWayFieldBridge fieldBridge;
private final NullMarkerCodec nullTokenCodec;
public NullEncodingTwoWayFieldBridge(TwoWayFieldBridge fieldBridge, NullMarkerCodec nullTokenCodec) {
this.fieldBridge = fieldBridge;
this.nullTokenCodec = nullTokenCodec;
}
@Override
public Object get(String name, Document document) {
final IndexableField field = document.getField( name );
if ( field == null ) {
//Avoid an NPE if the field isn't defined
LOG.loadingNonExistentField( name );
return null;
}
if ( nullTokenCodec.representsNullValue( field ) ) {
return null;
}
else {
return fieldBridge.get( name, document );
}
}
@Override
public String objectToString(Object object) {
if ( object == null ) {
NullMarker marker = nullTokenCodec.getNullMarker();
return marker == null ? null : marker.nullRepresentedAsString();
}
else {
return fieldBridge.objectToString( object );
}
}
@Override
public T unwrap(Class bridgeClass) {
return BridgeAdaptorUtils.unwrapAdaptorOnly( fieldBridge, bridgeClass );
}
@Override
public void set(String name, Object value, Document document, LuceneOptions luceneOptions) {
if ( value == null ) {
nullTokenCodec.encodeNullValue( name, document, luceneOptions );
}
else {
fieldBridge.set( name, value, document, luceneOptions );
}
}
public Query buildNullQuery(String fieldName) {
return nullTokenCodec.createNullMatchingQuery( fieldName );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy