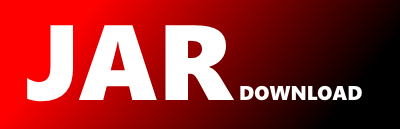
org.hibernate.search.cfg.PropertyMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Core of the Object/Lucene mapper, query engine and index management
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.cfg;
import java.lang.annotation.ElementType;
import java.util.Map;
import java.util.HashMap;
import org.apache.lucene.analysis.util.TokenizerFactory;
import org.hibernate.search.analyzer.Discriminator;
import org.hibernate.search.annotations.Resolution;
import org.hibernate.search.bridge.FieldBridge;
import org.hibernate.search.engine.BoostStrategy;
/**
* @author Emmanuel Bernard
*/
public class PropertyMapping {
protected final SearchMapping mapping;
protected final EntityDescriptor entity;
protected final PropertyDescriptor property;
public PropertyMapping(String name, ElementType type, EntityDescriptor entity, SearchMapping mapping) {
this.mapping = mapping;
this.entity = entity;
this.property = entity.getProperty( name, type );
}
protected PropertyMapping(PropertyDescriptor property, EntityDescriptor entity, SearchMapping mapping) {
this.mapping = mapping;
this.entity = entity;
this.property = property;
}
public DocumentIdMapping documentId() {
return new DocumentIdMapping( property, entity, mapping );
}
public FieldMapping field() {
return new FieldMapping( property, entity, mapping );
}
public PropertySpatialMapping spatial() {
return new PropertySpatialMapping( property, entity, mapping );
}
public PropertyLatitudeMapping latitude() {
return new PropertyLatitudeMapping( property, entity, mapping );
}
public PropertyLongitudeMapping longitude() {
return new PropertyLongitudeMapping( property, entity, mapping );
}
public DateBridgeMapping dateBridge(Resolution resolution) {
return new DateBridgeMapping( mapping, entity, property, resolution );
}
public CalendarBridgeMapping calendarBridge(Resolution resolution) {
return new CalendarBridgeMapping( mapping, entity, property, resolution );
}
public PropertyMapping analyzerDiscriminator(Class extends Discriminator> discriminator) {
Map analyzerDiscriminatorAnn = new HashMap();
analyzerDiscriminatorAnn.put( "impl", discriminator );
property.setAnalyzerDiscriminator( analyzerDiscriminatorAnn );
return this;
}
/**
* @param impl The class for an implementation of {@link BoostStrategy}.
* @return this mapping, for chained calls.
*
* @deprecated Index-time boosting will not be possible anymore starting from Lucene 7.
* You should use query-time boosting instead, for instance by calling
* {@link org.hibernate.search.query.dsl.FieldCustomization#boostedTo(float) boostedTo(float)}
* when building queries with the Hibernate Search query DSL.
*/
@Deprecated
public PropertyMapping dynamicBoost(Class extends BoostStrategy> impl) {
final Map dynamicBoostAnn = new HashMap();
dynamicBoostAnn.put( "impl", impl );
property.setDynamicBoost( dynamicBoostAnn );
return this;
}
public PropertyMapping property(String name, ElementType type) {
return new PropertyMapping( name, type, entity, mapping );
}
/**
* @deprecated See {@link org.hibernate.search.annotations.AnalyzerDef}
*/
@Deprecated
public AnalyzerDefMapping analyzerDef(String name, Class extends TokenizerFactory> tokenizerFactory) {
return analyzerDef( name, "", tokenizerFactory );
}
/**
* @deprecated See {@link org.hibernate.search.annotations.AnalyzerDef}
*/
@Deprecated
public AnalyzerDefMapping analyzerDef(String name, String tokenizerName, Class extends TokenizerFactory> tokenizerFactory) {
return new AnalyzerDefMapping( name, tokenizerName, tokenizerFactory, mapping );
}
/**
* @deprecated See {@link org.hibernate.search.annotations.AnalyzerDef}
*/
@Deprecated
public NormalizerDefMapping normalizerDef(String name) {
return new NormalizerDefMapping( name, mapping );
}
public EntityMapping entity(Class> entityType) {
return new EntityMapping( entityType, mapping );
}
public IndexEmbeddedMapping indexEmbedded() {
return new IndexEmbeddedMapping( mapping, property, entity );
}
public ContainedInMapping containedIn() {
return new ContainedInMapping( mapping, property, entity );
}
public PropertyMapping bridge(Class extends FieldBridge> fieldBridge) {
return new FieldBridgeDirectMapping( property, entity, mapping, fieldBridge );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy