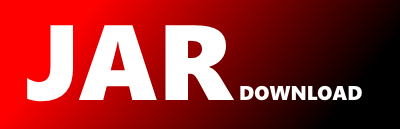
org.hibernate.search.store.ShardIdentifierProviderTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Core of the Object/Lucene mapper, query engine and index management
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.store;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashSet;
import java.util.Properties;
import java.util.Set;
import org.hibernate.search.filter.FullTextFilterImplementor;
import org.hibernate.search.spi.BuildContext;
/**
* Recommended parent class to create custom {@link ShardIdentifierProvider} implementations. Sub-classes must provide a
* no-arg constructor.
*
* @hsearch.experimental The exact method signatures are likely to change in future.
*
* @author Sanne Grinovero
*/
public abstract class ShardIdentifierProviderTemplate implements ShardIdentifierProvider {
private volatile Set knownShards = Collections.emptySet();
@Override
public final void initialize(Properties properties, BuildContext buildContext) {
Set initialShardNames = loadInitialShardNames( properties, buildContext );
knownShards = Collections.unmodifiableSet( new HashSet( initialShardNames ) );
}
protected abstract Set loadInitialShardNames(Properties properties, BuildContext buildContext);
/**
* @param shardName The name of a shard to add.
* @deprecated Support for
* dynamic sharding will be removed in Hibernate Search 6.
* Future-proof implementations must not call this method and
* must make sure the list of shards is fully defined on startup
* ({@link #loadInitialShardNames(Properties, BuildContext)}).
*/
@Deprecated
protected final void addShard(final String shardName) {
if ( ! knownShards.contains( shardName ) ) {
addShardSynchronized( shardName );
}
}
private synchronized void addShardSynchronized(final String shardName) {
HashSet newCopy = new HashSet( knownShards );
newCopy.add( shardName );
knownShards = Collections.unmodifiableSet( newCopy );
}
@Override
public final Set getAllShardIdentifiers() {
return knownShards;
}
/**
* Potentially suited to be overridden if you are able to narrow down the shard
* selection based on the active FullTextFilters.
*
* @deprecated Support for
* dynamic sharding will be removed in Hibernate Search 6.
* Future-proof implementations must not override this method.
*/
@Override
@Deprecated
public Set getShardIdentifiersForQuery(FullTextFilterImplementor[] fullTextFilters) {
return getAllShardIdentifiers();
}
/**
* Override this method if the mapping to a specific shard can be inferred just from the pair (id, type).
* The default implementation will perform delete and purge operations on all known indexes.
*
* @deprecated Support for
* dynamic sharding will be removed in Hibernate Search 6.
* Future-proof implementations must not override this method.
*/
@Override
@Deprecated
public Set getShardIdentifiersForDeletion(Class> entity, Serializable id, String idInString) {
return getAllShardIdentifiers();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy