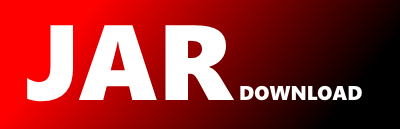
org.hibernate.search.indexes.impl.DefaultIndexReaderAccessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Core of the Object/Lucene mapper, query engine and index management
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.indexes.impl;
import java.util.HashMap;
import java.util.TreeSet;
import org.apache.lucene.index.IndexReader;
import org.hibernate.search.engine.impl.ImmutableSearchFactory;
import org.hibernate.search.engine.spi.EntityIndexBinding;
import org.hibernate.search.indexes.IndexReaderAccessor;
import org.hibernate.search.indexes.spi.IndexManager;
import org.hibernate.search.reader.impl.MultiReaderFactory;
import org.hibernate.search.spi.IndexedTypeIdentifier;
import org.hibernate.search.spi.IndexedTypeSet;
import org.hibernate.search.util.logging.impl.Log;
import org.hibernate.search.util.logging.impl.LoggerFactory;
import java.lang.invoke.MethodHandles;
/**
* Provides access to IndexReaders.
* IndexReaders opened through this service need to be closed using this service.
*
* @author Sanne Grinovero (C) 2011 Red Hat Inc.
*/
public class DefaultIndexReaderAccessor implements IndexReaderAccessor {
private static final Log log = LoggerFactory.make( MethodHandles.lookup() );
private final ImmutableSearchFactory searchFactory;
public DefaultIndexReaderAccessor(ImmutableSearchFactory immutableSearchFactory) {
this.searchFactory = immutableSearchFactory;
}
@Override
public void close(IndexReader indexReader) {
MultiReaderFactory.closeReader( indexReader );
}
@Override
public IndexReader open(Class>... entities) {
if ( entities.length == 0 ) {
throw log.needAtLeastOneIndexedEntityType();
}
HashMap indexManagers = new HashMap<>();
for ( Class> type : entities ) {
collectAllIndexManagersInto( searchFactory.getSafeIndexBindingForEntity( type ), indexManagers );
}
return MultiReaderFactory.openReader( indexManagers );
}
@Override
public IndexReader open(IndexedTypeSet types) {
if ( types.isEmpty() ) {
throw log.needAtLeastOneIndexedEntityType();
}
HashMap indexManagers = new HashMap<>();
for ( IndexedTypeIdentifier type : types ) {
collectAllIndexManagersInto( searchFactory.getSafeIndexBindingForEntity( type ), indexManagers );
}
return MultiReaderFactory.openReader( indexManagers );
}
private static void collectAllIndexManagersInto(EntityIndexBinding bindings, HashMap indexManagers) {
for ( IndexManager im : bindings.getIndexManagerSelector().all() ) {
indexManagers.put( im.getIndexName(), im );
}
}
@Override
public IndexReader open(String... indexNames) {
TreeSet names = new TreeSet();
for ( String name : indexNames ) {
if ( name != null ) {
names.add( name );
}
}
final int size = names.size();
if ( size == 0 ) {
throw log.needAtLeastOneIndexName();
}
String[] indexManagerNames = names.toArray( new String[size] );
IndexManagerHolder managerSource = searchFactory.getIndexManagerHolder();
IndexManager[] managers = new IndexManager[size];
for ( int i = 0; i < size; i++ ) {
String indexName = indexManagerNames[i];
managers[i] = managerSource.getIndexManager( indexName );
if ( managers[i] == null ) {
throw log.requestedIndexNotDefined( indexName );
}
}
return MultiReaderFactory.openReader( managers );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy