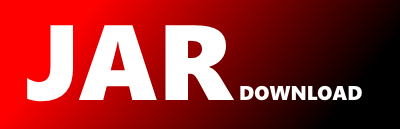
org.hibernate.search.cfg.SearchMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Core of the Object/Lucene mapper, query engine and index management
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.cfg;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.lucene.analysis.util.TokenizerFactory;
/**
* Allows to configure indexing and search related aspects of a domain model using a fluent Java API. This API can be
* used instead of or in conjunction with the annotation based configuration via
* {@link org.hibernate.search.annotations.Indexed} etc. In case of conflicts the programmatic configuration for an
* element takes precedence over the annotation-based configuration.
*
* @author Emmanuel Bernard
*/
public class SearchMapping {
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy