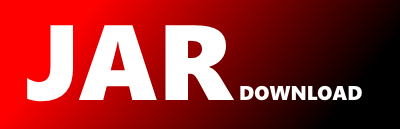
org.hibernate.search.batchindexing.MassIndexerProgressMonitor Maven / Gradle / Ivy
Show all versions of hibernate-search-engine Show documentation
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.batchindexing;
import org.hibernate.search.backend.IndexingMonitor;
/**
* As a MassIndexer can take some time to finish it's job,
* a MassIndexerProgressMonitor can be defined in the configuration
* property hibernate.search.worker.indexing.monitor
* implementing this interface to track indexing performance.
*
* Implementations must:
*
* - be threadsafe
* - have a no-arg constructor
*
*
* @author Sanne Grinovero
* @author Hardy Ferentschik
*/
public interface MassIndexerProgressMonitor extends IndexingMonitor {
/**
* Notify the monitor that {@code increment} more documents have been built.
*
* Summing the numbers passed to this method gives the total
* number of documents that have been built so far.
*
* This method is invoked several times during indexing,
* and calls are incremental:
* calling {@code documentsBuilt(3)} and then {@code documentsBuilt(1)}
* should be understood as "3+1 documents, i.e. 4 documents have been built".
*
* This method can be invoked from several threads thus implementors are required to be thread-safe.
*
* @param increment additional number of documents built
*/
void documentsBuilt(int increment);
/**
* Notify the monitor that {@code increment} more entities have been loaded from the database.
*
* Summing the numbers passed to this method gives the total
* number of entities that have been loaded so far.
*
* This method is invoked several times during indexing,
* and calls are incremental:
* calling {@code entitiesLoaded(3)} and then {@code entitiesLoaded(1)}
* should be understood as "3+1 documents, i.e. 4 documents have been loaded".
*
* This method can be invoked from several threads thus implementors are required to be thread-safe.
*
* @param increment additional number of entities loaded from database
*/
void entitiesLoaded(int increment);
/**
* Notify the monitor that {@code increment} more entities have been
* detected in the database and will be indexed.
*
* Summing the numbers passed to this method gives the total
* number of entities that Hibernate Search plans to index.
* This number can be incremented during indexing
* as Hibernate Search moves from one entity type to the next.
*
* This method is invoked several times during indexing,
* and calls are incremental:
* calling {@code addToTotalCount(3)} and then {@code addToTotalCount(1)}
* should be understood as "3+1 documents, i.e. 4 documents will be indexed".
*
* This method can be invoked from several threads thus implementors are required to be thread-safe.
*
* @param increment additional number of entities that will be indexed
*/
void addToTotalCount(long increment);
/**
* Notify the monitor that indexing is complete.
*/
void indexingCompleted();
}