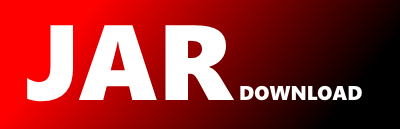
org.hibernate.search.exception.impl.ErrorContextBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-engine Show documentation
Show all versions of hibernate-search-engine Show documentation
Core of the Object/Lucene mapper, query engine and index management
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.exception.impl;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import org.hibernate.search.backend.LuceneWork;
import org.hibernate.search.exception.ErrorContext;
import org.hibernate.search.indexes.spi.IndexManager;
/**
* @author Amin Mohammed-Coleman
* @since 3.2
*/
public class ErrorContextBuilder {
private Throwable th;
private LuceneWork operationAtFault;
private Iterable workToBeDone;
private List failingOperations;
private List operationsThatWorked;
private IndexManager indexManager;
public ErrorContextBuilder errorThatOccurred(Throwable th) {
this.th = th;
return this;
}
public ErrorContextBuilder operationAtFault(LuceneWork operationAtFault) {
this.operationAtFault = operationAtFault;
return this;
}
public ErrorContextBuilder addWorkThatFailed(LuceneWork failedWork) {
this.getFailingOperations().add( failedWork );
return this;
}
public ErrorContextBuilder addAllWorkThatFailed(List worksThatFailed) {
this.getFailingOperations().addAll( worksThatFailed );
return this;
}
public ErrorContextBuilder workCompleted(LuceneWork luceneWork) {
this.getOperationsThatWorked().add( luceneWork );
return this;
}
public ErrorContextBuilder indexManager(IndexManager indexName) {
this.indexManager = indexName;
return this;
}
public ErrorContextBuilder allWorkToBeDone(Iterable workOnWriter) {
this.workToBeDone = workOnWriter;
return this;
}
public ErrorContext createErrorContext() {
ErrorContextImpl context = new ErrorContextImpl();
context.setThrowable( th );
// for situation when there is a primary failure
if ( operationAtFault != null ) {
context.setOperationAtFault( operationAtFault );
}
else if ( workToBeDone != null ) {
List workLeft = new ArrayList();
for ( LuceneWork work : workToBeDone ) {
workLeft.add( work );
}
if ( operationsThatWorked != null ) {
workLeft.removeAll( operationsThatWorked );
}
if ( !workLeft.isEmpty() ) {
context.setOperationAtFault( workLeft.remove( 0 ) );
getFailingOperations().addAll( workLeft );
}
}
context.setFailingOperations( getFailingOperations() );
context.setIndexManager( indexManager );
return context;
}
private List getFailingOperations() {
if ( failingOperations == null ) {
failingOperations = new ArrayList();
}
return failingOperations;
}
private List getOperationsThatWorked() {
if ( operationsThatWorked == null ) {
operationsThatWorked = new LinkedList();
}
return operationsThatWorked;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy