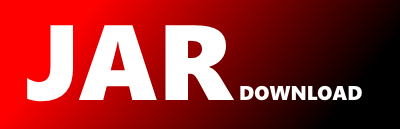
org.hibernate.search.jsr352.jberet.impl.HibernateSearchJsr352Extension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-search-jsr352-jberet
Show all versions of hibernate-search-jsr352-jberet
Hibernate Search JSR-352 integration - for JBeret
/*
* Hibernate Search, full-text search for your domain model
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.search.jsr352.jberet.impl;
import java.lang.annotation.Annotation;
import javax.enterprise.context.ContextNotActiveException;
import javax.enterprise.context.spi.Context;
import javax.enterprise.context.spi.Contextual;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.event.Observes;
import javax.enterprise.inject.spi.AfterBeanDiscovery;
import javax.enterprise.inject.spi.AfterTypeDiscovery;
import javax.enterprise.inject.spi.AnnotatedType;
import javax.enterprise.inject.spi.BeanManager;
import javax.enterprise.inject.spi.Extension;
import org.hibernate.search.jsr352.context.jpa.spi.EntityManagerFactoryRegistry;
import org.hibernate.search.jsr352.inject.scope.spi.HibernateSearchJobScoped;
import org.hibernate.search.jsr352.inject.scope.spi.HibernateSearchPartitionScoped;
import org.hibernate.search.jsr352.massindexing.impl.JobContextSetupListener;
import org.hibernate.search.jsr352.massindexing.impl.steps.lucene.EntityReader;
import org.jberet.cdi.JobScoped;
import org.jberet.cdi.PartitionScoped;
/**
* A CDI extension to explicitly add hibernate-search-jsr352-core types to the CDI context.
*
* These types must be registered into the CDI context because they expect one of their
* attributes to be injected, thus they need to be instantiated by CDI.
*
* Ideally we should use component scanning to that effect, but:
*
* - In CDI, component scanning is configured per-module in META-INF/beans.xml,
* with no way to add a package from another module.
*
- In Wildfly, component scanning in dependencies is always done with the "all" discovery type,
* meaning that even components without CDI annotations will be discovered.
* Besides exposing private types unnecessarily,
* this is annoying because it puts default {@link EntityManagerFactoryRegistry} implementations into the CDI context,
* leading to conflicts when injecting such types
* (since this module, hibernate-search-jsr352-jberet, also provides an implementation).
*
See WFLY-8656.
* - Even if we could solve the above, JBeret requires specific scope annotations to be set on the
* beans so that they are correctly scoped. Since the core isn't dependent on JBeret, we must specify these
* scopes manually when registering the beans.
*
* Thus we use explicit type and scope registration as a workaround.
*
* @author Yoann Rodiere
*/
public class HibernateSearchJsr352Extension implements Extension {
public void afterTypeDiscovery(@Observes AfterTypeDiscovery event, BeanManager beanManager) {
registerType( event, beanManager, JobContextSetupListener.class );
registerType( event, beanManager, EntityReader.class );
}
public void afterBeanDiscovery(@Observes AfterBeanDiscovery event, BeanManager beanManager) {
addScopeAlias( event, beanManager, HibernateSearchJobScoped.class, JobScoped.class );
addScopeAlias( event, beanManager, HibernateSearchPartitionScoped.class, PartitionScoped.class );
}
private void registerType(AfterTypeDiscovery event, BeanManager beanManager, Class> clazz) {
AnnotatedType> annotatedType = beanManager.createAnnotatedType( clazz );
event.addAnnotatedType( annotatedType, clazz.getName() );
}
private void addScopeAlias(AfterBeanDiscovery event, BeanManager beanManager,
Class extends Annotation> alias, Class extends Annotation> target) {
event.addContext( new AliasedContext( alias, beanManager, target ) );
}
private static class AliasedContext implements Context {
private final Class extends Annotation> scopeType;
private final BeanManager targetBeanManager;
private final Class extends Annotation> targetScopeType;
public AliasedContext(Class extends Annotation> scopeType,
BeanManager targetBeanManager, Class extends Annotation> targetScopeType) {
super();
this.scopeType = scopeType;
this.targetBeanManager = targetBeanManager;
this.targetScopeType = targetScopeType;
}
private Context delegate() {
return targetBeanManager.getContext( targetScopeType );
}
@Override
public Class extends Annotation> getScope() {
return scopeType;
}
@Override
public T get(Contextual contextual, CreationalContext creationalContext) {
return delegate().get( contextual, creationalContext );
}
@Override
public T get(Contextual contextual) {
return delegate().get( contextual );
}
@Override
public boolean isActive() {
try {
delegate();
return true;
}
catch (ContextNotActiveException e) {
return false;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy