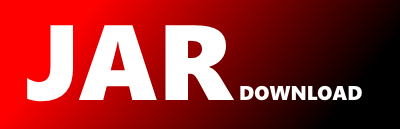
org.hibernate.mapping.PersistentClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate Show documentation
Show all versions of hibernate Show documentation
Relational Persistence for Java
//$Id: PersistentClass.java 10319 2006-08-23 21:57:10Z epbernard $
package org.hibernate.mapping;
import java.io.Serializable;
import java.util.*;
import java.util.Set;
import org.hibernate.MappingException;
import org.hibernate.EntityMode;
import org.hibernate.dialect.Dialect;
import org.hibernate.engine.Mapping;
import org.hibernate.engine.ExecuteUpdateResultCheckStyle;
import org.hibernate.sql.Alias;
import org.hibernate.util.EmptyIterator;
import org.hibernate.util.JoinedIterator;
import org.hibernate.util.ReflectHelper;
import org.hibernate.util.SingletonIterator;
import org.hibernate.util.StringHelper;
/**
* Mapping for an entity.
*
* @author Gavin King
*/
public abstract class PersistentClass implements Serializable, Filterable, MetaAttributable {
private static final Alias PK_ALIAS = new Alias(15, "PK");
public static final String NULL_DISCRIMINATOR_MAPPING = "null";
public static final String NOT_NULL_DISCRIMINATOR_MAPPING = "not null";
private String entityName;
private String className;
private String proxyInterfaceName;
private String nodeName;
private String discriminatorValue;
private boolean lazy;
private ArrayList properties = new ArrayList();
private final ArrayList subclasses = new ArrayList();
private final ArrayList subclassProperties = new ArrayList();
private final ArrayList subclassTables = new ArrayList();
private boolean dynamicInsert;
private boolean dynamicUpdate;
private int batchSize=-1;
private boolean selectBeforeUpdate;
private java.util.Map metaAttributes;
private ArrayList joins = new ArrayList();
private final ArrayList subclassJoins = new ArrayList();
private final java.util.Map filters = new HashMap();
protected final java.util.Set synchronizedTables = new HashSet();
private String loaderName;
private Boolean isAbstract;
private boolean hasSubselectLoadableCollections;
private Component identifierMapper;
// Custom SQL
private String customSQLInsert;
private boolean customInsertCallable;
private ExecuteUpdateResultCheckStyle insertCheckStyle;
private String customSQLUpdate;
private boolean customUpdateCallable;
private ExecuteUpdateResultCheckStyle updateCheckStyle;
private String customSQLDelete;
private boolean customDeleteCallable;
private ExecuteUpdateResultCheckStyle deleteCheckStyle;
private String temporaryIdTableName;
private String temporaryIdTableDDL;
private java.util.Map tuplizerImpls;
protected int optimisticLockMode;
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className==null ? null : className.intern();
}
public String getProxyInterfaceName() {
return proxyInterfaceName;
}
public void setProxyInterfaceName(String proxyInterfaceName) {
this.proxyInterfaceName = proxyInterfaceName;
}
public Class getMappedClass() throws MappingException {
if (className==null) return null;
try {
return ReflectHelper.classForName(className);
}
catch (ClassNotFoundException cnfe) {
throw new MappingException("entity class not found: " + className, cnfe);
}
}
public Class getProxyInterface() {
if (proxyInterfaceName==null) return null;
try {
return ReflectHelper.classForName(proxyInterfaceName);
}
catch (ClassNotFoundException cnfe) {
throw new MappingException("proxy class not found: " + proxyInterfaceName, cnfe);
}
}
public boolean useDynamicInsert() {
return dynamicInsert;
}
abstract int nextSubclassId();
public abstract int getSubclassId();
public boolean useDynamicUpdate() {
return dynamicUpdate;
}
public void setDynamicInsert(boolean dynamicInsert) {
this.dynamicInsert = dynamicInsert;
}
public void setDynamicUpdate(boolean dynamicUpdate) {
this.dynamicUpdate = dynamicUpdate;
}
public String getDiscriminatorValue() {
return discriminatorValue;
}
public void addSubclass(Subclass subclass) throws MappingException {
// inheritance cycle detection (paranoid check)
PersistentClass superclass = getSuperclass();
while (superclass!=null) {
if( subclass.getEntityName().equals( superclass.getEntityName() ) ) {
throw new MappingException(
"Circular inheritance mapping detected: " +
subclass.getEntityName() +
" will have it self as superclass when extending " +
getEntityName()
);
}
superclass = superclass.getSuperclass();
}
subclasses.add(subclass);
}
public boolean hasSubclasses() {
return subclasses.size() > 0;
}
public int getSubclassSpan() {
int n = subclasses.size();
Iterator iter = subclasses.iterator();
while ( iter.hasNext() ) {
n += ( (Subclass) iter.next() ).getSubclassSpan();
}
return n;
}
/**
* Iterate over subclasses in a special 'order', most derived subclasses
* first.
*/
public Iterator getSubclassIterator() {
Iterator[] iters = new Iterator[ subclasses.size() + 1 ];
Iterator iter = subclasses.iterator();
int i=0;
while ( iter.hasNext() ) {
iters[i++] = ( (Subclass) iter.next() ).getSubclassIterator();
}
iters[i] = subclasses.iterator();
return new JoinedIterator(iters);
}
public Iterator getSubclassClosureIterator() {
ArrayList iters = new ArrayList();
iters.add( new SingletonIterator(this) );
Iterator iter = getSubclassIterator();
while ( iter.hasNext() ) {
PersistentClass clazz = (PersistentClass) iter.next();
iters.add( clazz.getSubclassClosureIterator() );
}
return new JoinedIterator(iters);
}
public Table getIdentityTable() {
return getRootTable();
}
public Iterator getDirectSubclasses() {
return subclasses.iterator();
}
public void addProperty(Property p) {
properties.add(p);
p.setPersistentClass(this);
}
public abstract Table getTable();
public String getEntityName() {
return entityName;
}
public abstract boolean isMutable();
public abstract boolean hasIdentifierProperty();
public abstract Property getIdentifierProperty();
public abstract KeyValue getIdentifier();
public abstract Property getVersion();
public abstract Value getDiscriminator();
public abstract boolean isInherited();
public abstract boolean isPolymorphic();
public abstract boolean isVersioned();
public abstract String getCacheConcurrencyStrategy();
public abstract PersistentClass getSuperclass();
public abstract boolean isExplicitPolymorphism();
public abstract boolean isDiscriminatorInsertable();
public abstract Iterator getPropertyClosureIterator();
public abstract Iterator getTableClosureIterator();
public abstract Iterator getKeyClosureIterator();
protected void addSubclassProperty(Property prop) {
subclassProperties.add(prop);
}
protected void addSubclassJoin(Join join) {
subclassJoins.add(join);
}
protected void addSubclassTable(Table subclassTable) {
subclassTables.add(subclassTable);
}
public Iterator getSubclassPropertyClosureIterator() {
ArrayList iters = new ArrayList();
iters.add( getPropertyClosureIterator() );
iters.add( subclassProperties.iterator() );
for ( int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy