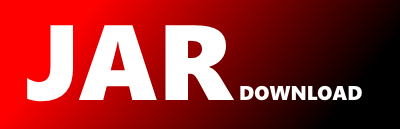
org.hibernate.dialect.IngresDialect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate Show documentation
Show all versions of hibernate Show documentation
Relational Persistence for Java
//$Id: IngresDialect.java 12861 2007-07-31 15:23:40Z [email protected] $
package org.hibernate.dialect;
import java.sql.Types;
import org.hibernate.Hibernate;
import org.hibernate.dialect.function.SQLFunctionTemplate;
import org.hibernate.dialect.function.NoArgSQLFunction;
import org.hibernate.dialect.function.StandardSQLFunction;
import org.hibernate.dialect.function.VarArgsSQLFunction;
/**
* An Ingres SQL dialect.
*
* Known limitations:
* - only supports simple constants or columns on the left side of an IN, making (1,2,3) in (...) or (FOR UPDATE OF, allowing
* particular rows to be locked?
*
* @return True (Ingres does support "for update of" syntax...)
*/
public boolean supportsForUpdateOf() {
return true;
}
/**
* The syntax used to add a column to a table (optional).
*/
public String getAddColumnString() {
return "add column";
}
/**
* The keyword used to specify a nullable column.
*
* @return String
*/
public String getNullColumnString() {
return " with null";
}
/**
* Does this dialect support sequences?
*
* @return boolean
*/
public boolean supportsSequences() {
return true;
}
/**
* The syntax that fetches the next value of a sequence, if sequences are supported.
*
* @param sequenceName the name of the sequence
*
* @return String
*/
public String getSequenceNextValString(String sequenceName) {
return "select nextval for " + sequenceName;
}
public String getSelectSequenceNextValString(String sequenceName) {
return sequenceName + ".nextval";
}
/**
* The syntax used to create a sequence, if sequences are supported.
*
* @param sequenceName the name of the sequence
*
* @return String
*/
public String getCreateSequenceString(String sequenceName) {
return "create sequence " + sequenceName;
}
/**
* The syntax used to drop a sequence, if sequences are supported.
*
* @param sequenceName the name of the sequence
*
* @return String
*/
public String getDropSequenceString(String sequenceName) {
return "drop sequence " + sequenceName + " restrict";
}
/**
* A query used to find all sequences
*/
public String getQuerySequencesString() {
return "select seq_name from iisequence";
}
/**
* The name of the SQL function that transforms a string to
* lowercase
*
* @return String
*/
public String getLowercaseFunction() {
return "lowercase";
}
/**
* Does this Dialect have some kind of LIMIT syntax?
*/
public boolean supportsLimit() {
return true;
}
/**
* Does this dialect support an offset?
*/
public boolean supportsLimitOffset() {
return false;
}
/**
* Add a LIMIT clause to the given SQL SELECT
*
* @return the modified SQL
*/
public String getLimitString(String querySelect, int offset, int limit) {
if ( offset > 0 ) {
throw new UnsupportedOperationException( "offset not supported" );
}
return new StringBuffer( querySelect.length() + 16 )
.append( querySelect )
.insert( 6, " first " + limit )
.toString();
}
public boolean supportsVariableLimit() {
return false;
}
/**
* Does the LIMIT clause take a "maximum" row number instead
* of a total number of returned rows?
*/
public boolean useMaxForLimit() {
return true;
}
/**
* Ingres explicitly needs "unique not null", because "with null" is default
*/
public boolean supportsNotNullUnique() {
return false;
}
/**
* Does this dialect support temporary tables?
*/
public boolean supportsTemporaryTables() {
return true;
}
public String getCreateTemporaryTableString() {
return "declare global temporary table";
}
public String getCreateTemporaryTablePostfix() {
return "on commit preserve rows with norecovery";
}
public String generateTemporaryTableName(String baseTableName) {
return "session." + super.generateTemporaryTableName( baseTableName );
}
/**
* Expression for current_timestamp
*/
public String getCurrentTimestampSQLFunctionName() {
return "date(now)";
}
// Overridden informational metadata ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
public boolean supportsSubselectAsInPredicateLHS() {
return false;
}
public boolean supportsEmptyInList() {
return false;
}
public boolean supportsExpectedLobUsagePattern () {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy