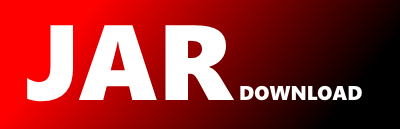
org.hisp.dhis.parser.expression.antlr.ExpressionParser Maven / Gradle / Ivy
// Generated from org/hisp/dhis/parser/expression/antlr/Expression.g4 by ANTLR 4.7.2
package org.hisp.dhis.parser.expression.antlr;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class ExpressionParser extends Parser {
static { RuntimeMetaData.checkVersion("4.7.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
T__0=1, T__1=2, T__2=3, T__3=4, T__4=5, T__5=6, T__6=7, PAREN=8, PERIOD_OFFSET=9,
STAGE_OFFSET=10, PLUS=11, MINUS=12, POWER=13, MUL=14, DIV=15, MOD=16,
EQ=17, NE=18, GT=19, LT=20, GEQ=21, LEQ=22, NOT=23, AND=24, OR=25, EXCLAMATION_POINT=26,
AMPERSAND_2=27, VERTICAL_BAR_2=28, FIRST_NON_NULL=29, GREATEST=30, IF=31,
IS_NOT_NULL=32, IS_NULL=33, LEAST=34, LOG=35, LOG10=36, ORGUNIT_ANCESTOR=37,
ORGUNIT_GROUP=38, AVG=39, COUNT=40, MAX=41, MEDIAN=42, MIN=43, PERCENTILE_CONT=44,
STDDEV=45, STDDEV_POP=46, STDDEV_SAMP=47, SUM=48, VARIANCE=49, V_ANALYTICS_PERIOD_END=50,
V_ANALYTICS_PERIOD_START=51, V_COMPLETED_DATE=52, V_CREATION_DATE=53,
V_CURRENT_DATE=54, V_DUE_DATE=55, V_ENROLLMENT_COUNT=56, V_ENROLLMENT_DATE=57,
V_ENROLLMENT_ID=58, V_ENROLLMENT_STATUS=59, V_ENVIRONMENT=60, V_EVENT_COUNT=61,
V_EVENT_DATE=62, V_EVENT_ID=63, V_EVENT_STATUS=64, V_EXECUTION_DATE=65,
V_INCIDENT_DATE=66, V_ORG_UNIT_COUNT=67, V_OU=68, V_OU_CODE=69, V_PROGRAM_NAME=70,
V_PROGRAM_STAGE_ID=71, V_PROGRAM_STAGE_NAME=72, V_SYNC_DATE=73, V_TEI_COUNT=74,
V_VALUE_COUNT=75, V_ZERO_POS_VALUE_COUNT=76, D2_ADD_DAYS=77, D2_CEIL=78,
D2_CONCATENATE=79, D2_CONDITION=80, D2_COUNT=81, D2_COUNT_IF_CONDITION=82,
D2_COUNT_IF_VALUE=83, D2_COUNT_IF_ZERO_POS=84, D2_DAYS_BETWEEN=85, D2_FLOOR=86,
D2_HAS_USER_ROLE=87, D2_HAS_VALUE=88, D2_IN_ORG_UNIT_GROUP=89, D2_LAST_EVENT_DATE=90,
D2_LEFT=91, D2_LENGTH=92, D2_MAX_VALUE=93, D2_MINUTES_BETWEEN=94, D2_MIN_VALUE=95,
D2_MODULUS=96, D2_MONTHS_BETWEEN=97, D2_OIZP=98, D2_RELATIONSHIP_COUNT=99,
D2_RIGHT=100, D2_ROUND=101, D2_SPLIT=102, D2_SUBSTRING=103, D2_VALIDATE_PATTERN=104,
D2_WEEKS_BETWEEN=105, D2_YEARS_BETWEEN=106, D2_ZING=107, D2_ZPVC=108,
D2_ZSCOREHFA=109, D2_ZSCOREWFA=110, D2_ZSCOREWFH=111, HASH_BRACE=112,
A_BRACE=113, C_BRACE=114, D_BRACE=115, I_BRACE=116, N_BRACE=117, OUG_BRACE=118,
PS_EVENTDATE=119, R_BRACE=120, V_BRACE=121, X_BRACE=122, DAYS=123, REPORTING_RATE_TYPE=124,
INTEGER_LITERAL=125, NUMERIC_LITERAL=126, BOOLEAN_LITERAL=127, QUOTED_UID=128,
STRING_LITERAL=129, Q1=130, Q2=131, UID=132, TAGGED_UID0=133, TAGGED_UID1=134,
CO_TAG=135, CO_GROUP_TAG=136, DE_GROUP_TAG=137, IDENTIFIER=138, EMPTY=139,
WS=140;
public static final int
RULE_expression = 0, RULE_expr = 1, RULE_programVariable = 2, RULE_numericLiteral = 3,
RULE_integerLiteral = 4, RULE_programRuleStringVariableName = 5, RULE_stringLiteral = 6,
RULE_booleanLiteral = 7, RULE_programRuleVariableName = 8, RULE_programRuleVariablePart = 9;
private static String[] makeRuleNames() {
return new String[] {
"expression", "expr", "programVariable", "numericLiteral", "integerLiteral",
"programRuleStringVariableName", "stringLiteral", "booleanLiteral", "programRuleVariableName",
"programRuleVariablePart"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, "')'", "','", "'distinct'", "'.'", "'}'", "'.*'", "'.*.'", "'('",
"'.periodOffset('", "'.stageOffset('", "'+'", "'-'", "'^'", "'*'", "'/'",
"'%'", "'=='", "'!='", "'>'", "'<'", "'>='", "'<='", "'not'", "'and'",
"'or'", "'!'", "'&&'", "'||'", "'firstNonNull('", "'greatest('", "'if('",
"'isNotNull('", "'isNull('", "'least('", "'log('", "'log10('", "'orgUnit.ancestor('",
"'orgUnit.group('", "'avg('", "'count('", "'max('", "'median('", "'min('",
"'percentileCont('", "'stddev('", "'stddevPop('", "'stddevSamp('", "'sum('",
"'variance('", "'analytics_period_end'", "'analytics_period_start'",
"'completed_date'", "'creation_date'", "'current_date'", "'due_date'",
"'enrollment_count'", "'enrollment_date'", "'enrollment_id'", "'enrollment_status'",
"'environment'", "'event_count'", "'event_date'", "'event_id'", "'event_status'",
"'execution_date'", "'incident_date'", "'org_unit_count'", "'org_unit'",
"'orgunit_code'", "'program_name'", "'program_stage_id'", "'program_stage_name'",
"'sync_date'", "'tei_count'", "'value_count'", "'zero_pos_value_count'",
"'d2:addDays('", "'d2:ceil('", "'d2:concatenate('", "'d2:condition('",
"'d2:count('", "'d2:countIfCondition('", "'d2:countIfValue('", "'d2:countIfZeroPos('",
"'d2:daysBetween('", "'d2:floor('", "'d2:hasUserRole('", "'d2:hasValue('",
"'d2:inOrgUnitGroup('", "'d2:lastEventDate('", "'d2:left('", "'d2:length('",
"'d2:maxValue('", "'d2:minutesBetween('", "'d2:minValue('", "'d2:modulus('",
"'d2:monthsBetween('", "'d2:oizp('", "'d2:relationshipCount('", "'d2:right('",
"'d2:round('", "'d2:split('", "'d2:substring('", "'d2:validatePattern('",
"'d2:weeksBetween('", "'d2:yearsBetween('", "'d2:zing('", "'d2:zpvc('",
"'d2:zScoreHFA('", "'d2:zScoreWFA('", "'d2:zScoreWFH('", "'#{'", "'A{'",
"'C{'", "'D{'", "'I{'", "'N{'", "'OUG{'", "'PS_EVENTDATE:'", "'R{'",
"'V{'", "'X{'", "'[days]'", null, null, null, null, null, null, "'''",
"'\"'", null, null, null, "'co:'", "'coGroup:'", "'deGroup:'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, null, null, null, null, null, null, null, "PAREN", "PERIOD_OFFSET",
"STAGE_OFFSET", "PLUS", "MINUS", "POWER", "MUL", "DIV", "MOD", "EQ",
"NE", "GT", "LT", "GEQ", "LEQ", "NOT", "AND", "OR", "EXCLAMATION_POINT",
"AMPERSAND_2", "VERTICAL_BAR_2", "FIRST_NON_NULL", "GREATEST", "IF",
"IS_NOT_NULL", "IS_NULL", "LEAST", "LOG", "LOG10", "ORGUNIT_ANCESTOR",
"ORGUNIT_GROUP", "AVG", "COUNT", "MAX", "MEDIAN", "MIN", "PERCENTILE_CONT",
"STDDEV", "STDDEV_POP", "STDDEV_SAMP", "SUM", "VARIANCE", "V_ANALYTICS_PERIOD_END",
"V_ANALYTICS_PERIOD_START", "V_COMPLETED_DATE", "V_CREATION_DATE", "V_CURRENT_DATE",
"V_DUE_DATE", "V_ENROLLMENT_COUNT", "V_ENROLLMENT_DATE", "V_ENROLLMENT_ID",
"V_ENROLLMENT_STATUS", "V_ENVIRONMENT", "V_EVENT_COUNT", "V_EVENT_DATE",
"V_EVENT_ID", "V_EVENT_STATUS", "V_EXECUTION_DATE", "V_INCIDENT_DATE",
"V_ORG_UNIT_COUNT", "V_OU", "V_OU_CODE", "V_PROGRAM_NAME", "V_PROGRAM_STAGE_ID",
"V_PROGRAM_STAGE_NAME", "V_SYNC_DATE", "V_TEI_COUNT", "V_VALUE_COUNT",
"V_ZERO_POS_VALUE_COUNT", "D2_ADD_DAYS", "D2_CEIL", "D2_CONCATENATE",
"D2_CONDITION", "D2_COUNT", "D2_COUNT_IF_CONDITION", "D2_COUNT_IF_VALUE",
"D2_COUNT_IF_ZERO_POS", "D2_DAYS_BETWEEN", "D2_FLOOR", "D2_HAS_USER_ROLE",
"D2_HAS_VALUE", "D2_IN_ORG_UNIT_GROUP", "D2_LAST_EVENT_DATE", "D2_LEFT",
"D2_LENGTH", "D2_MAX_VALUE", "D2_MINUTES_BETWEEN", "D2_MIN_VALUE", "D2_MODULUS",
"D2_MONTHS_BETWEEN", "D2_OIZP", "D2_RELATIONSHIP_COUNT", "D2_RIGHT",
"D2_ROUND", "D2_SPLIT", "D2_SUBSTRING", "D2_VALIDATE_PATTERN", "D2_WEEKS_BETWEEN",
"D2_YEARS_BETWEEN", "D2_ZING", "D2_ZPVC", "D2_ZSCOREHFA", "D2_ZSCOREWFA",
"D2_ZSCOREWFH", "HASH_BRACE", "A_BRACE", "C_BRACE", "D_BRACE", "I_BRACE",
"N_BRACE", "OUG_BRACE", "PS_EVENTDATE", "R_BRACE", "V_BRACE", "X_BRACE",
"DAYS", "REPORTING_RATE_TYPE", "INTEGER_LITERAL", "NUMERIC_LITERAL",
"BOOLEAN_LITERAL", "QUOTED_UID", "STRING_LITERAL", "Q1", "Q2", "UID",
"TAGGED_UID0", "TAGGED_UID1", "CO_TAG", "CO_GROUP_TAG", "DE_GROUP_TAG",
"IDENTIFIER", "EMPTY", "WS"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "Expression.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public ExpressionParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class ExpressionContext extends ParserRuleContext {
public ExprContext expr() {
return getRuleContext(ExprContext.class,0);
}
public TerminalNode EOF() { return getToken(ExpressionParser.EOF, 0); }
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitExpression(this);
else return visitor.visitChildren(this);
}
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 0, RULE_expression);
try {
enterOuterAlt(_localctx, 1);
{
setState(20);
expr(0);
setState(21);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExprContext extends ParserRuleContext {
public Token it;
public Token distinct;
public Token uid0;
public Token uid1;
public Token psEventDate;
public Token wild1;
public Token wild2;
public Token uid2;
public IntegerLiteralContext period;
public IntegerLiteralContext stage;
public List expr() {
return getRuleContexts(ExprContext.class);
}
public ExprContext expr(int i) {
return getRuleContext(ExprContext.class,i);
}
public List WS() { return getTokens(ExpressionParser.WS); }
public TerminalNode WS(int i) {
return getToken(ExpressionParser.WS, i);
}
public TerminalNode PAREN() { return getToken(ExpressionParser.PAREN, 0); }
public TerminalNode PLUS() { return getToken(ExpressionParser.PLUS, 0); }
public TerminalNode MINUS() { return getToken(ExpressionParser.MINUS, 0); }
public TerminalNode EXCLAMATION_POINT() { return getToken(ExpressionParser.EXCLAMATION_POINT, 0); }
public TerminalNode NOT() { return getToken(ExpressionParser.NOT, 0); }
public TerminalNode FIRST_NON_NULL() { return getToken(ExpressionParser.FIRST_NON_NULL, 0); }
public TerminalNode GREATEST() { return getToken(ExpressionParser.GREATEST, 0); }
public TerminalNode IF() { return getToken(ExpressionParser.IF, 0); }
public TerminalNode IS_NOT_NULL() { return getToken(ExpressionParser.IS_NOT_NULL, 0); }
public TerminalNode IS_NULL() { return getToken(ExpressionParser.IS_NULL, 0); }
public TerminalNode LEAST() { return getToken(ExpressionParser.LEAST, 0); }
public TerminalNode LOG() { return getToken(ExpressionParser.LOG, 0); }
public TerminalNode LOG10() { return getToken(ExpressionParser.LOG10, 0); }
public List UID() { return getTokens(ExpressionParser.UID); }
public TerminalNode UID(int i) {
return getToken(ExpressionParser.UID, i);
}
public TerminalNode ORGUNIT_ANCESTOR() { return getToken(ExpressionParser.ORGUNIT_ANCESTOR, 0); }
public TerminalNode ORGUNIT_GROUP() { return getToken(ExpressionParser.ORGUNIT_GROUP, 0); }
public TerminalNode AVG() { return getToken(ExpressionParser.AVG, 0); }
public TerminalNode COUNT() { return getToken(ExpressionParser.COUNT, 0); }
public TerminalNode MAX() { return getToken(ExpressionParser.MAX, 0); }
public TerminalNode MEDIAN() { return getToken(ExpressionParser.MEDIAN, 0); }
public TerminalNode MIN() { return getToken(ExpressionParser.MIN, 0); }
public TerminalNode PERCENTILE_CONT() { return getToken(ExpressionParser.PERCENTILE_CONT, 0); }
public TerminalNode STDDEV() { return getToken(ExpressionParser.STDDEV, 0); }
public TerminalNode STDDEV_POP() { return getToken(ExpressionParser.STDDEV_POP, 0); }
public TerminalNode STDDEV_SAMP() { return getToken(ExpressionParser.STDDEV_SAMP, 0); }
public TerminalNode SUM() { return getToken(ExpressionParser.SUM, 0); }
public TerminalNode VARIANCE() { return getToken(ExpressionParser.VARIANCE, 0); }
public TerminalNode D2_ADD_DAYS() { return getToken(ExpressionParser.D2_ADD_DAYS, 0); }
public TerminalNode D2_CEIL() { return getToken(ExpressionParser.D2_CEIL, 0); }
public TerminalNode D2_CONCATENATE() { return getToken(ExpressionParser.D2_CONCATENATE, 0); }
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public TerminalNode D2_CONDITION() { return getToken(ExpressionParser.D2_CONDITION, 0); }
public TerminalNode HASH_BRACE() { return getToken(ExpressionParser.HASH_BRACE, 0); }
public TerminalNode D2_COUNT() { return getToken(ExpressionParser.D2_COUNT, 0); }
public ProgramRuleVariableNameContext programRuleVariableName() {
return getRuleContext(ProgramRuleVariableNameContext.class,0);
}
public TerminalNode A_BRACE() { return getToken(ExpressionParser.A_BRACE, 0); }
public ProgramRuleStringVariableNameContext programRuleStringVariableName() {
return getRuleContext(ProgramRuleStringVariableNameContext.class,0);
}
public TerminalNode D2_COUNT_IF_CONDITION() { return getToken(ExpressionParser.D2_COUNT_IF_CONDITION, 0); }
public TerminalNode D2_COUNT_IF_VALUE() { return getToken(ExpressionParser.D2_COUNT_IF_VALUE, 0); }
public TerminalNode D2_COUNT_IF_ZERO_POS() { return getToken(ExpressionParser.D2_COUNT_IF_ZERO_POS, 0); }
public TerminalNode D2_DAYS_BETWEEN() { return getToken(ExpressionParser.D2_DAYS_BETWEEN, 0); }
public TerminalNode D2_FLOOR() { return getToken(ExpressionParser.D2_FLOOR, 0); }
public TerminalNode D2_HAS_USER_ROLE() { return getToken(ExpressionParser.D2_HAS_USER_ROLE, 0); }
public TerminalNode D2_HAS_VALUE() { return getToken(ExpressionParser.D2_HAS_VALUE, 0); }
public TerminalNode V_BRACE() { return getToken(ExpressionParser.V_BRACE, 0); }
public ProgramVariableContext programVariable() {
return getRuleContext(ProgramVariableContext.class,0);
}
public TerminalNode D2_IN_ORG_UNIT_GROUP() { return getToken(ExpressionParser.D2_IN_ORG_UNIT_GROUP, 0); }
public TerminalNode D2_LAST_EVENT_DATE() { return getToken(ExpressionParser.D2_LAST_EVENT_DATE, 0); }
public TerminalNode D2_LEFT() { return getToken(ExpressionParser.D2_LEFT, 0); }
public TerminalNode D2_LENGTH() { return getToken(ExpressionParser.D2_LENGTH, 0); }
public TerminalNode D2_MAX_VALUE() { return getToken(ExpressionParser.D2_MAX_VALUE, 0); }
public TerminalNode PS_EVENTDATE() { return getToken(ExpressionParser.PS_EVENTDATE, 0); }
public TerminalNode D2_MINUTES_BETWEEN() { return getToken(ExpressionParser.D2_MINUTES_BETWEEN, 0); }
public TerminalNode D2_MIN_VALUE() { return getToken(ExpressionParser.D2_MIN_VALUE, 0); }
public TerminalNode D2_MODULUS() { return getToken(ExpressionParser.D2_MODULUS, 0); }
public TerminalNode D2_MONTHS_BETWEEN() { return getToken(ExpressionParser.D2_MONTHS_BETWEEN, 0); }
public TerminalNode D2_OIZP() { return getToken(ExpressionParser.D2_OIZP, 0); }
public TerminalNode D2_RELATIONSHIP_COUNT() { return getToken(ExpressionParser.D2_RELATIONSHIP_COUNT, 0); }
public TerminalNode QUOTED_UID() { return getToken(ExpressionParser.QUOTED_UID, 0); }
public TerminalNode D2_RIGHT() { return getToken(ExpressionParser.D2_RIGHT, 0); }
public TerminalNode D2_ROUND() { return getToken(ExpressionParser.D2_ROUND, 0); }
public TerminalNode D2_SPLIT() { return getToken(ExpressionParser.D2_SPLIT, 0); }
public TerminalNode D2_SUBSTRING() { return getToken(ExpressionParser.D2_SUBSTRING, 0); }
public TerminalNode D2_VALIDATE_PATTERN() { return getToken(ExpressionParser.D2_VALIDATE_PATTERN, 0); }
public TerminalNode D2_WEEKS_BETWEEN() { return getToken(ExpressionParser.D2_WEEKS_BETWEEN, 0); }
public TerminalNode D2_YEARS_BETWEEN() { return getToken(ExpressionParser.D2_YEARS_BETWEEN, 0); }
public TerminalNode D2_ZING() { return getToken(ExpressionParser.D2_ZING, 0); }
public TerminalNode D2_ZPVC() { return getToken(ExpressionParser.D2_ZPVC, 0); }
public TerminalNode D2_ZSCOREHFA() { return getToken(ExpressionParser.D2_ZSCOREHFA, 0); }
public TerminalNode D2_ZSCOREWFA() { return getToken(ExpressionParser.D2_ZSCOREWFA, 0); }
public TerminalNode D2_ZSCOREWFH() { return getToken(ExpressionParser.D2_ZSCOREWFH, 0); }
public TerminalNode TAGGED_UID0() { return getToken(ExpressionParser.TAGGED_UID0, 0); }
public TerminalNode TAGGED_UID1() { return getToken(ExpressionParser.TAGGED_UID1, 0); }
public TerminalNode C_BRACE() { return getToken(ExpressionParser.C_BRACE, 0); }
public TerminalNode D_BRACE() { return getToken(ExpressionParser.D_BRACE, 0); }
public TerminalNode I_BRACE() { return getToken(ExpressionParser.I_BRACE, 0); }
public TerminalNode N_BRACE() { return getToken(ExpressionParser.N_BRACE, 0); }
public TerminalNode OUG_BRACE() { return getToken(ExpressionParser.OUG_BRACE, 0); }
public TerminalNode REPORTING_RATE_TYPE() { return getToken(ExpressionParser.REPORTING_RATE_TYPE, 0); }
public TerminalNode R_BRACE() { return getToken(ExpressionParser.R_BRACE, 0); }
public TerminalNode DAYS() { return getToken(ExpressionParser.DAYS, 0); }
public NumericLiteralContext numericLiteral() {
return getRuleContext(NumericLiteralContext.class,0);
}
public BooleanLiteralContext booleanLiteral() {
return getRuleContext(BooleanLiteralContext.class,0);
}
public TerminalNode POWER() { return getToken(ExpressionParser.POWER, 0); }
public TerminalNode MUL() { return getToken(ExpressionParser.MUL, 0); }
public TerminalNode DIV() { return getToken(ExpressionParser.DIV, 0); }
public TerminalNode MOD() { return getToken(ExpressionParser.MOD, 0); }
public TerminalNode LT() { return getToken(ExpressionParser.LT, 0); }
public TerminalNode GT() { return getToken(ExpressionParser.GT, 0); }
public TerminalNode LEQ() { return getToken(ExpressionParser.LEQ, 0); }
public TerminalNode GEQ() { return getToken(ExpressionParser.GEQ, 0); }
public TerminalNode EQ() { return getToken(ExpressionParser.EQ, 0); }
public TerminalNode NE() { return getToken(ExpressionParser.NE, 0); }
public TerminalNode AMPERSAND_2() { return getToken(ExpressionParser.AMPERSAND_2, 0); }
public TerminalNode AND() { return getToken(ExpressionParser.AND, 0); }
public TerminalNode VERTICAL_BAR_2() { return getToken(ExpressionParser.VERTICAL_BAR_2, 0); }
public TerminalNode OR() { return getToken(ExpressionParser.OR, 0); }
public TerminalNode PERIOD_OFFSET() { return getToken(ExpressionParser.PERIOD_OFFSET, 0); }
public IntegerLiteralContext integerLiteral() {
return getRuleContext(IntegerLiteralContext.class,0);
}
public TerminalNode STAGE_OFFSET() { return getToken(ExpressionParser.STAGE_OFFSET, 0); }
public ExprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expr; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterExpr(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitExpr(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitExpr(this);
else return visitor.visitChildren(this);
}
}
public final ExprContext expr() throws RecognitionException {
return expr(0);
}
private ExprContext expr(int _p) throws RecognitionException {
ParserRuleContext _parentctx = _ctx;
int _parentState = getState();
ExprContext _localctx = new ExprContext(_ctx, _parentState);
ExprContext _prevctx = _localctx;
int _startState = 2;
enterRecursionRule(_localctx, 2, RULE_expr, _p);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1140);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,100,_ctx) ) {
case 1:
{
setState(25);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(24);
match(WS);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(27);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,0,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
setState(29);
expr(115);
}
break;
case 2:
{
setState(30);
((ExprContext)_localctx).it = match(PAREN);
setState(31);
expr(0);
setState(32);
match(T__0);
}
break;
case 3:
{
setState(34);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << PLUS) | (1L << MINUS) | (1L << NOT) | (1L << EXCLAMATION_POINT))) != 0)) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(35);
expr(109);
}
break;
case 4:
{
setState(36);
((ExprContext)_localctx).it = match(FIRST_NON_NULL);
setState(37);
expr(0);
setState(42);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(38);
match(T__1);
setState(39);
expr(0);
}
}
setState(44);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(45);
match(T__0);
}
break;
case 5:
{
setState(47);
((ExprContext)_localctx).it = match(GREATEST);
setState(48);
expr(0);
setState(53);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(49);
match(T__1);
setState(50);
expr(0);
}
}
setState(55);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(56);
match(T__0);
}
break;
case 6:
{
setState(58);
((ExprContext)_localctx).it = match(IF);
setState(59);
expr(0);
setState(60);
match(T__1);
setState(61);
expr(0);
setState(62);
match(T__1);
setState(63);
expr(0);
setState(64);
match(T__0);
}
break;
case 7:
{
setState(66);
((ExprContext)_localctx).it = match(IS_NOT_NULL);
setState(67);
expr(0);
setState(68);
match(T__0);
}
break;
case 8:
{
setState(70);
((ExprContext)_localctx).it = match(IS_NULL);
setState(71);
expr(0);
setState(72);
match(T__0);
}
break;
case 9:
{
setState(74);
((ExprContext)_localctx).it = match(LEAST);
setState(75);
expr(0);
setState(80);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(76);
match(T__1);
setState(77);
expr(0);
}
}
setState(82);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(83);
match(T__0);
}
break;
case 10:
{
setState(85);
((ExprContext)_localctx).it = match(LOG);
setState(86);
expr(0);
setState(89);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__1) {
{
setState(87);
match(T__1);
setState(88);
expr(0);
}
}
setState(91);
match(T__0);
}
break;
case 11:
{
setState(93);
((ExprContext)_localctx).it = match(LOG10);
setState(94);
expr(0);
setState(95);
match(T__0);
}
break;
case 12:
{
setState(97);
((ExprContext)_localctx).it = match(ORGUNIT_ANCESTOR);
setState(101);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(98);
match(WS);
}
}
setState(103);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(104);
match(UID);
setState(108);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(105);
match(WS);
}
}
setState(110);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(127);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(111);
match(T__1);
setState(115);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(112);
match(WS);
}
}
setState(117);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(118);
match(UID);
setState(122);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(119);
match(WS);
}
}
setState(124);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(129);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(130);
match(T__0);
}
break;
case 13:
{
setState(131);
((ExprContext)_localctx).it = match(ORGUNIT_GROUP);
setState(135);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(132);
match(WS);
}
}
setState(137);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(138);
match(UID);
setState(142);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(139);
match(WS);
}
}
setState(144);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(161);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(145);
match(T__1);
setState(149);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(146);
match(WS);
}
}
setState(151);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(152);
match(UID);
setState(156);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(153);
match(WS);
}
}
setState(158);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(163);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(164);
match(T__0);
}
break;
case 14:
{
setState(165);
((ExprContext)_localctx).it = match(AVG);
setState(166);
expr(0);
setState(167);
match(T__0);
}
break;
case 15:
{
setState(169);
((ExprContext)_localctx).it = match(COUNT);
setState(177);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,16,_ctx) ) {
case 1:
{
setState(173);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(170);
match(WS);
}
}
setState(175);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(176);
((ExprContext)_localctx).distinct = match(T__2);
}
break;
}
setState(179);
expr(0);
setState(180);
match(T__0);
}
break;
case 16:
{
setState(182);
((ExprContext)_localctx).it = match(MAX);
setState(183);
expr(0);
setState(184);
match(T__0);
}
break;
case 17:
{
setState(186);
((ExprContext)_localctx).it = match(MEDIAN);
setState(187);
expr(0);
setState(188);
match(T__0);
}
break;
case 18:
{
setState(190);
((ExprContext)_localctx).it = match(MIN);
setState(191);
expr(0);
setState(192);
match(T__0);
}
break;
case 19:
{
setState(194);
((ExprContext)_localctx).it = match(PERCENTILE_CONT);
setState(195);
expr(0);
setState(196);
match(T__1);
setState(197);
expr(0);
setState(198);
match(T__0);
}
break;
case 20:
{
setState(200);
((ExprContext)_localctx).it = match(STDDEV);
setState(201);
expr(0);
setState(202);
match(T__0);
}
break;
case 21:
{
setState(204);
((ExprContext)_localctx).it = match(STDDEV_POP);
setState(205);
expr(0);
setState(206);
match(T__0);
}
break;
case 22:
{
setState(208);
((ExprContext)_localctx).it = match(STDDEV_SAMP);
setState(209);
expr(0);
setState(210);
match(T__0);
}
break;
case 23:
{
setState(212);
((ExprContext)_localctx).it = match(SUM);
setState(213);
expr(0);
setState(214);
match(T__0);
}
break;
case 24:
{
setState(216);
((ExprContext)_localctx).it = match(VARIANCE);
setState(217);
expr(0);
setState(218);
match(T__0);
}
break;
case 25:
{
setState(220);
((ExprContext)_localctx).it = match(D2_ADD_DAYS);
setState(221);
expr(0);
setState(222);
match(T__1);
setState(223);
expr(0);
setState(224);
match(T__0);
}
break;
case 26:
{
setState(226);
((ExprContext)_localctx).it = match(D2_CEIL);
setState(227);
expr(0);
setState(228);
match(T__0);
}
break;
case 27:
{
setState(230);
((ExprContext)_localctx).it = match(D2_CONCATENATE);
setState(231);
expr(0);
setState(236);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(232);
match(T__1);
setState(233);
expr(0);
}
}
setState(238);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(239);
match(T__0);
}
break;
case 28:
{
setState(241);
((ExprContext)_localctx).it = match(D2_CONDITION);
setState(245);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(242);
match(WS);
}
}
setState(247);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(248);
stringLiteral();
setState(252);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(249);
match(WS);
}
}
setState(254);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(255);
match(T__1);
setState(256);
expr(0);
setState(257);
match(T__1);
setState(258);
expr(0);
setState(259);
match(T__0);
}
break;
case 29:
{
setState(261);
((ExprContext)_localctx).it = match(D2_COUNT);
setState(265);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(262);
match(WS);
}
}
setState(267);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(268);
match(HASH_BRACE);
setState(269);
((ExprContext)_localctx).uid0 = match(UID);
setState(270);
match(T__3);
setState(271);
((ExprContext)_localctx).uid1 = match(UID);
setState(272);
match(T__4);
setState(276);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(273);
match(WS);
}
}
setState(278);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(279);
match(T__0);
}
break;
case 30:
{
setState(280);
((ExprContext)_localctx).it = match(D2_COUNT);
setState(284);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(281);
match(WS);
}
}
setState(286);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(287);
match(HASH_BRACE);
setState(288);
programRuleVariableName();
setState(289);
match(T__4);
setState(293);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(290);
match(WS);
}
}
setState(295);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(296);
match(T__0);
}
break;
case 31:
{
setState(298);
((ExprContext)_localctx).it = match(D2_COUNT);
setState(302);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(299);
match(WS);
}
}
setState(304);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(305);
match(A_BRACE);
setState(306);
programRuleVariableName();
setState(307);
match(T__4);
setState(311);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(308);
match(WS);
}
}
setState(313);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(314);
match(T__0);
}
break;
case 32:
{
setState(316);
((ExprContext)_localctx).it = match(D2_COUNT);
setState(320);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(317);
match(WS);
}
}
setState(322);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(323);
programRuleStringVariableName();
setState(327);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(324);
match(WS);
}
}
setState(329);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(330);
match(T__0);
}
break;
case 33:
{
setState(332);
((ExprContext)_localctx).it = match(D2_COUNT_IF_CONDITION);
setState(336);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(333);
match(WS);
}
}
setState(338);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(339);
match(HASH_BRACE);
setState(340);
((ExprContext)_localctx).uid0 = match(UID);
setState(341);
match(T__3);
setState(342);
((ExprContext)_localctx).uid1 = match(UID);
setState(343);
match(T__4);
setState(347);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(344);
match(WS);
}
}
setState(349);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(350);
match(T__1);
setState(354);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(351);
match(WS);
}
}
setState(356);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(357);
stringLiteral();
setState(361);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(358);
match(WS);
}
}
setState(363);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(364);
match(T__0);
}
break;
case 34:
{
setState(366);
((ExprContext)_localctx).it = match(D2_COUNT_IF_CONDITION);
setState(370);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(367);
match(WS);
}
}
setState(372);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(373);
match(HASH_BRACE);
setState(374);
programRuleVariableName();
setState(375);
match(T__4);
setState(379);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(376);
match(WS);
}
}
setState(381);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(382);
match(T__1);
setState(386);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(383);
match(WS);
}
}
setState(388);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(389);
stringLiteral();
setState(393);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(390);
match(WS);
}
}
setState(395);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(396);
match(T__0);
}
break;
case 35:
{
setState(398);
((ExprContext)_localctx).it = match(D2_COUNT_IF_CONDITION);
setState(402);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(399);
match(WS);
}
}
setState(404);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(405);
match(A_BRACE);
setState(406);
programRuleVariableName();
setState(407);
match(T__4);
setState(411);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(408);
match(WS);
}
}
setState(413);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(414);
match(T__1);
setState(418);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(415);
match(WS);
}
}
setState(420);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(421);
stringLiteral();
setState(425);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(422);
match(WS);
}
}
setState(427);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(428);
match(T__0);
}
break;
case 36:
{
setState(430);
((ExprContext)_localctx).it = match(D2_COUNT_IF_CONDITION);
setState(434);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(431);
match(WS);
}
}
setState(436);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(437);
programRuleStringVariableName();
setState(441);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(438);
match(WS);
}
}
setState(443);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(444);
match(T__1);
setState(448);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(445);
match(WS);
}
}
setState(450);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(451);
stringLiteral();
setState(455);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(452);
match(WS);
}
}
setState(457);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(458);
match(T__0);
}
break;
case 37:
{
setState(460);
((ExprContext)_localctx).it = match(D2_COUNT_IF_VALUE);
setState(464);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(461);
match(WS);
}
}
setState(466);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(467);
match(HASH_BRACE);
setState(468);
((ExprContext)_localctx).uid0 = match(UID);
setState(469);
match(T__3);
setState(470);
((ExprContext)_localctx).uid1 = match(UID);
setState(471);
match(T__4);
setState(475);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(472);
match(WS);
}
}
setState(477);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(478);
match(T__1);
setState(479);
expr(0);
setState(480);
match(T__0);
}
break;
case 38:
{
setState(482);
((ExprContext)_localctx).it = match(D2_COUNT_IF_VALUE);
setState(486);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(483);
match(WS);
}
}
setState(488);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(489);
match(HASH_BRACE);
setState(490);
programRuleVariableName();
setState(491);
match(T__4);
setState(495);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(492);
match(WS);
}
}
setState(497);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(498);
match(T__1);
setState(499);
expr(0);
setState(500);
match(T__0);
}
break;
case 39:
{
setState(502);
((ExprContext)_localctx).it = match(D2_COUNT_IF_VALUE);
setState(506);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(503);
match(WS);
}
}
setState(508);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(509);
match(A_BRACE);
setState(510);
programRuleVariableName();
setState(511);
match(T__4);
setState(515);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(512);
match(WS);
}
}
setState(517);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(518);
match(T__1);
setState(519);
expr(0);
setState(520);
match(T__0);
}
break;
case 40:
{
setState(522);
((ExprContext)_localctx).it = match(D2_COUNT_IF_VALUE);
setState(526);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(523);
match(WS);
}
}
setState(528);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(529);
programRuleStringVariableName();
setState(533);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(530);
match(WS);
}
}
setState(535);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(536);
match(T__1);
setState(537);
expr(0);
setState(538);
match(T__0);
}
break;
case 41:
{
setState(540);
((ExprContext)_localctx).it = match(D2_COUNT_IF_ZERO_POS);
setState(544);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(541);
match(WS);
}
}
setState(546);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(547);
match(HASH_BRACE);
setState(548);
((ExprContext)_localctx).uid0 = match(UID);
setState(549);
match(T__3);
setState(550);
((ExprContext)_localctx).uid1 = match(UID);
setState(551);
match(T__4);
setState(555);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(552);
match(WS);
}
}
setState(557);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(558);
match(T__0);
}
break;
case 42:
{
setState(559);
((ExprContext)_localctx).it = match(D2_COUNT_IF_ZERO_POS);
setState(563);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(560);
match(WS);
}
}
setState(565);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(566);
match(HASH_BRACE);
setState(567);
programRuleVariableName();
setState(568);
match(T__4);
setState(572);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(569);
match(WS);
}
}
setState(574);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(575);
match(T__0);
}
break;
case 43:
{
setState(577);
((ExprContext)_localctx).it = match(D2_COUNT_IF_ZERO_POS);
setState(581);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(578);
match(WS);
}
}
setState(583);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(584);
match(A_BRACE);
setState(585);
programRuleVariableName();
setState(586);
match(T__4);
setState(590);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(587);
match(WS);
}
}
setState(592);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(593);
match(T__0);
}
break;
case 44:
{
setState(595);
((ExprContext)_localctx).it = match(D2_COUNT_IF_ZERO_POS);
setState(599);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(596);
match(WS);
}
}
setState(601);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(602);
programRuleStringVariableName();
setState(606);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(603);
match(WS);
}
}
setState(608);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(609);
match(T__0);
}
break;
case 45:
{
setState(611);
((ExprContext)_localctx).it = match(D2_DAYS_BETWEEN);
setState(612);
expr(0);
setState(613);
match(T__1);
setState(614);
expr(0);
setState(615);
match(T__0);
}
break;
case 46:
{
setState(617);
((ExprContext)_localctx).it = match(D2_FLOOR);
setState(618);
expr(0);
setState(619);
match(T__0);
}
break;
case 47:
{
setState(621);
((ExprContext)_localctx).it = match(D2_HAS_USER_ROLE);
setState(622);
expr(0);
setState(623);
match(T__0);
}
break;
case 48:
{
setState(625);
((ExprContext)_localctx).it = match(D2_HAS_VALUE);
setState(629);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(626);
match(WS);
}
}
setState(631);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(632);
match(HASH_BRACE);
setState(633);
((ExprContext)_localctx).uid0 = match(UID);
setState(634);
match(T__3);
setState(635);
((ExprContext)_localctx).uid1 = match(UID);
setState(636);
match(T__4);
setState(640);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(637);
match(WS);
}
}
setState(642);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(643);
match(T__0);
}
break;
case 49:
{
setState(644);
((ExprContext)_localctx).it = match(D2_HAS_VALUE);
setState(648);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(645);
match(WS);
}
}
setState(650);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(651);
match(HASH_BRACE);
setState(652);
programRuleVariableName();
setState(653);
match(T__4);
setState(657);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(654);
match(WS);
}
}
setState(659);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(660);
match(T__0);
}
break;
case 50:
{
setState(662);
((ExprContext)_localctx).it = match(D2_HAS_VALUE);
setState(666);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(663);
match(WS);
}
}
setState(668);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(669);
programRuleStringVariableName();
setState(673);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(670);
match(WS);
}
}
setState(675);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(676);
match(T__0);
}
break;
case 51:
{
setState(678);
((ExprContext)_localctx).it = match(D2_HAS_VALUE);
setState(682);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(679);
match(WS);
}
}
setState(684);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(685);
match(A_BRACE);
setState(686);
((ExprContext)_localctx).uid0 = match(UID);
setState(687);
match(T__4);
setState(691);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(688);
match(WS);
}
}
setState(693);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(694);
match(T__0);
}
break;
case 52:
{
setState(695);
((ExprContext)_localctx).it = match(D2_HAS_VALUE);
setState(699);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(696);
match(WS);
}
}
setState(701);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(702);
match(A_BRACE);
setState(703);
programRuleVariableName();
setState(704);
match(T__4);
setState(708);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(705);
match(WS);
}
}
setState(710);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(711);
match(T__0);
}
break;
case 53:
{
setState(713);
((ExprContext)_localctx).it = match(D2_HAS_VALUE);
setState(717);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(714);
match(WS);
}
}
setState(719);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(720);
match(V_BRACE);
setState(721);
programVariable();
setState(722);
match(T__4);
setState(726);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(723);
match(WS);
}
}
setState(728);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(729);
match(T__0);
}
break;
case 54:
{
setState(731);
((ExprContext)_localctx).it = match(D2_IN_ORG_UNIT_GROUP);
setState(732);
expr(0);
setState(733);
match(T__0);
}
break;
case 55:
{
setState(735);
((ExprContext)_localctx).it = match(D2_LAST_EVENT_DATE);
setState(736);
expr(0);
setState(737);
match(T__0);
}
break;
case 56:
{
setState(739);
((ExprContext)_localctx).it = match(D2_LEFT);
setState(740);
expr(0);
setState(741);
match(T__1);
setState(742);
expr(0);
setState(743);
match(T__0);
}
break;
case 57:
{
setState(745);
((ExprContext)_localctx).it = match(D2_LENGTH);
setState(746);
expr(0);
setState(747);
match(T__0);
}
break;
case 58:
{
setState(749);
((ExprContext)_localctx).it = match(D2_MAX_VALUE);
setState(753);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(750);
match(WS);
}
}
setState(755);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(756);
match(HASH_BRACE);
setState(757);
((ExprContext)_localctx).uid0 = match(UID);
setState(758);
match(T__3);
setState(759);
((ExprContext)_localctx).uid1 = match(UID);
setState(760);
match(T__4);
setState(764);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(761);
match(WS);
}
}
setState(766);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(767);
match(T__0);
}
break;
case 59:
{
setState(768);
((ExprContext)_localctx).it = match(D2_MAX_VALUE);
setState(772);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(769);
match(WS);
}
}
setState(774);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(775);
match(HASH_BRACE);
setState(776);
programRuleVariableName();
setState(777);
match(T__4);
setState(781);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(778);
match(WS);
}
}
setState(783);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(784);
match(T__0);
}
break;
case 60:
{
setState(786);
((ExprContext)_localctx).it = match(D2_MAX_VALUE);
setState(790);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(787);
match(WS);
}
}
setState(792);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(793);
match(A_BRACE);
setState(794);
programRuleVariableName();
setState(795);
match(T__4);
setState(799);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(796);
match(WS);
}
}
setState(801);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(802);
match(T__0);
}
break;
case 61:
{
setState(804);
((ExprContext)_localctx).it = match(D2_MAX_VALUE);
setState(808);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(805);
match(WS);
}
}
setState(810);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(811);
programRuleStringVariableName();
setState(815);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(812);
match(WS);
}
}
setState(817);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(818);
match(T__0);
}
break;
case 62:
{
setState(820);
((ExprContext)_localctx).it = match(D2_MAX_VALUE);
setState(824);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(821);
match(WS);
}
}
setState(826);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(827);
((ExprContext)_localctx).psEventDate = match(PS_EVENTDATE);
setState(831);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(828);
match(WS);
}
}
setState(833);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(834);
((ExprContext)_localctx).uid0 = match(UID);
setState(838);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(835);
match(WS);
}
}
setState(840);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(841);
match(T__0);
}
break;
case 63:
{
setState(842);
((ExprContext)_localctx).it = match(D2_MINUTES_BETWEEN);
setState(843);
expr(0);
setState(844);
match(T__1);
setState(845);
expr(0);
setState(846);
match(T__0);
}
break;
case 64:
{
setState(848);
((ExprContext)_localctx).it = match(D2_MIN_VALUE);
setState(852);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(849);
match(WS);
}
}
setState(854);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(855);
match(HASH_BRACE);
setState(856);
((ExprContext)_localctx).uid0 = match(UID);
setState(857);
match(T__3);
setState(858);
((ExprContext)_localctx).uid1 = match(UID);
setState(859);
match(T__4);
setState(863);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(860);
match(WS);
}
}
setState(865);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(866);
match(T__0);
}
break;
case 65:
{
setState(867);
((ExprContext)_localctx).it = match(D2_MIN_VALUE);
setState(871);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(868);
match(WS);
}
}
setState(873);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(874);
match(HASH_BRACE);
setState(875);
programRuleVariableName();
setState(876);
match(T__4);
setState(880);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(877);
match(WS);
}
}
setState(882);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(883);
match(T__0);
}
break;
case 66:
{
setState(885);
((ExprContext)_localctx).it = match(D2_MIN_VALUE);
setState(889);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(886);
match(WS);
}
}
setState(891);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(892);
match(A_BRACE);
setState(893);
programRuleVariableName();
setState(894);
match(T__4);
setState(898);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(895);
match(WS);
}
}
setState(900);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(901);
match(T__0);
}
break;
case 67:
{
setState(903);
((ExprContext)_localctx).it = match(D2_MIN_VALUE);
setState(907);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(904);
match(WS);
}
}
setState(909);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(910);
programRuleStringVariableName();
setState(914);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(911);
match(WS);
}
}
setState(916);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(917);
match(T__0);
}
break;
case 68:
{
setState(919);
((ExprContext)_localctx).it = match(D2_MIN_VALUE);
setState(923);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(920);
match(WS);
}
}
setState(925);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(926);
((ExprContext)_localctx).psEventDate = match(PS_EVENTDATE);
setState(930);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(927);
match(WS);
}
}
setState(932);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(933);
((ExprContext)_localctx).uid0 = match(UID);
setState(937);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(934);
match(WS);
}
}
setState(939);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(940);
match(T__0);
}
break;
case 69:
{
setState(941);
((ExprContext)_localctx).it = match(D2_MODULUS);
setState(942);
expr(0);
setState(943);
match(T__1);
setState(944);
expr(0);
setState(945);
match(T__0);
}
break;
case 70:
{
setState(947);
((ExprContext)_localctx).it = match(D2_MONTHS_BETWEEN);
setState(948);
expr(0);
setState(949);
match(T__1);
setState(950);
expr(0);
setState(951);
match(T__0);
}
break;
case 71:
{
setState(953);
((ExprContext)_localctx).it = match(D2_OIZP);
setState(954);
expr(0);
setState(955);
match(T__0);
}
break;
case 72:
{
setState(957);
((ExprContext)_localctx).it = match(D2_RELATIONSHIP_COUNT);
setState(961);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,94,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(958);
match(WS);
}
}
}
setState(963);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,94,_ctx);
}
setState(965);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==QUOTED_UID) {
{
setState(964);
match(QUOTED_UID);
}
}
setState(970);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(967);
match(WS);
}
}
setState(972);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(973);
match(T__0);
}
break;
case 73:
{
setState(974);
((ExprContext)_localctx).it = match(D2_RIGHT);
setState(975);
expr(0);
setState(976);
match(T__1);
setState(977);
expr(0);
setState(978);
match(T__0);
}
break;
case 74:
{
setState(980);
((ExprContext)_localctx).it = match(D2_ROUND);
setState(981);
expr(0);
setState(982);
match(T__0);
}
break;
case 75:
{
setState(984);
((ExprContext)_localctx).it = match(D2_SPLIT);
setState(985);
expr(0);
setState(986);
match(T__1);
setState(987);
expr(0);
setState(988);
match(T__1);
setState(989);
expr(0);
setState(990);
match(T__0);
}
break;
case 76:
{
setState(992);
((ExprContext)_localctx).it = match(D2_SUBSTRING);
setState(993);
expr(0);
setState(994);
match(T__1);
setState(995);
expr(0);
setState(996);
match(T__1);
setState(997);
expr(0);
setState(998);
match(T__0);
}
break;
case 77:
{
setState(1000);
((ExprContext)_localctx).it = match(D2_VALIDATE_PATTERN);
setState(1001);
expr(0);
setState(1002);
match(T__1);
setState(1003);
expr(0);
setState(1004);
match(T__0);
}
break;
case 78:
{
setState(1006);
((ExprContext)_localctx).it = match(D2_WEEKS_BETWEEN);
setState(1007);
expr(0);
setState(1008);
match(T__1);
setState(1009);
expr(0);
setState(1010);
match(T__0);
}
break;
case 79:
{
setState(1012);
((ExprContext)_localctx).it = match(D2_YEARS_BETWEEN);
setState(1013);
expr(0);
setState(1014);
match(T__1);
setState(1015);
expr(0);
setState(1016);
match(T__0);
}
break;
case 80:
{
setState(1018);
((ExprContext)_localctx).it = match(D2_ZING);
setState(1019);
expr(0);
setState(1020);
match(T__0);
}
break;
case 81:
{
setState(1022);
((ExprContext)_localctx).it = match(D2_ZPVC);
setState(1023);
expr(0);
setState(1028);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__1) {
{
{
setState(1024);
match(T__1);
setState(1025);
expr(0);
}
}
setState(1030);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1031);
match(T__0);
}
break;
case 82:
{
setState(1033);
((ExprContext)_localctx).it = match(D2_ZSCOREHFA);
setState(1034);
expr(0);
setState(1035);
match(T__1);
setState(1036);
expr(0);
setState(1037);
match(T__1);
setState(1038);
expr(0);
setState(1039);
match(T__0);
}
break;
case 83:
{
setState(1041);
((ExprContext)_localctx).it = match(D2_ZSCOREWFA);
setState(1042);
expr(0);
setState(1043);
match(T__1);
setState(1044);
expr(0);
setState(1045);
match(T__1);
setState(1046);
expr(0);
setState(1047);
match(T__0);
}
break;
case 84:
{
setState(1049);
((ExprContext)_localctx).it = match(D2_ZSCOREWFH);
setState(1050);
expr(0);
setState(1051);
match(T__1);
setState(1052);
expr(0);
setState(1053);
match(T__1);
setState(1054);
expr(0);
setState(1055);
match(T__0);
}
break;
case 85:
{
setState(1057);
((ExprContext)_localctx).it = match(HASH_BRACE);
setState(1058);
((ExprContext)_localctx).uid0 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID0) ) {
((ExprContext)_localctx).uid0 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1060);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__5) {
{
setState(1059);
((ExprContext)_localctx).wild1 = match(T__5);
}
}
setState(1062);
match(T__4);
}
break;
case 86:
{
setState(1063);
((ExprContext)_localctx).it = match(HASH_BRACE);
setState(1064);
((ExprContext)_localctx).uid0 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID0) ) {
((ExprContext)_localctx).uid0 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1065);
match(T__3);
setState(1066);
((ExprContext)_localctx).uid1 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID1) ) {
((ExprContext)_localctx).uid1 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1067);
match(T__4);
}
break;
case 87:
{
setState(1068);
((ExprContext)_localctx).it = match(HASH_BRACE);
setState(1069);
((ExprContext)_localctx).uid0 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID0) ) {
((ExprContext)_localctx).uid0 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1070);
match(T__3);
setState(1071);
((ExprContext)_localctx).uid1 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID1) ) {
((ExprContext)_localctx).uid1 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1072);
((ExprContext)_localctx).wild2 = match(T__5);
setState(1073);
match(T__4);
}
break;
case 88:
{
setState(1074);
((ExprContext)_localctx).it = match(HASH_BRACE);
setState(1075);
((ExprContext)_localctx).uid0 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID0) ) {
((ExprContext)_localctx).uid0 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1076);
match(T__6);
setState(1077);
((ExprContext)_localctx).uid2 = match(UID);
setState(1078);
match(T__4);
}
break;
case 89:
{
setState(1079);
((ExprContext)_localctx).it = match(HASH_BRACE);
setState(1080);
((ExprContext)_localctx).uid0 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID0) ) {
((ExprContext)_localctx).uid0 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1081);
match(T__3);
setState(1082);
((ExprContext)_localctx).uid1 = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==UID || _la==TAGGED_UID1) ) {
((ExprContext)_localctx).uid1 = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1083);
match(T__3);
setState(1084);
((ExprContext)_localctx).uid2 = match(UID);
setState(1085);
match(T__4);
}
break;
case 90:
{
setState(1086);
((ExprContext)_localctx).it = match(HASH_BRACE);
setState(1087);
programRuleVariableName();
setState(1088);
match(T__4);
}
break;
case 91:
{
setState(1090);
((ExprContext)_localctx).it = match(A_BRACE);
setState(1091);
((ExprContext)_localctx).uid0 = match(UID);
setState(1092);
match(T__3);
setState(1093);
((ExprContext)_localctx).uid1 = match(UID);
setState(1094);
match(T__4);
}
break;
case 92:
{
setState(1095);
((ExprContext)_localctx).it = match(A_BRACE);
setState(1096);
((ExprContext)_localctx).uid0 = match(UID);
setState(1097);
match(T__4);
}
break;
case 93:
{
setState(1098);
((ExprContext)_localctx).it = match(A_BRACE);
setState(1099);
programRuleVariableName();
setState(1100);
match(T__4);
}
break;
case 94:
{
setState(1102);
((ExprContext)_localctx).it = match(C_BRACE);
setState(1103);
((ExprContext)_localctx).uid0 = match(UID);
setState(1104);
match(T__4);
}
break;
case 95:
{
setState(1105);
((ExprContext)_localctx).it = match(D_BRACE);
setState(1106);
((ExprContext)_localctx).uid0 = match(UID);
setState(1107);
match(T__3);
setState(1108);
((ExprContext)_localctx).uid1 = match(UID);
setState(1109);
match(T__4);
}
break;
case 96:
{
setState(1110);
((ExprContext)_localctx).it = match(I_BRACE);
setState(1111);
((ExprContext)_localctx).uid0 = match(UID);
setState(1112);
match(T__4);
}
break;
case 97:
{
setState(1113);
((ExprContext)_localctx).it = match(N_BRACE);
setState(1114);
((ExprContext)_localctx).uid0 = match(UID);
setState(1115);
match(T__4);
}
break;
case 98:
{
setState(1116);
((ExprContext)_localctx).it = match(OUG_BRACE);
setState(1117);
((ExprContext)_localctx).uid0 = match(UID);
setState(1118);
match(T__4);
}
break;
case 99:
{
setState(1119);
((ExprContext)_localctx).it = match(PS_EVENTDATE);
setState(1123);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(1120);
match(WS);
}
}
setState(1125);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1126);
((ExprContext)_localctx).uid0 = match(UID);
}
break;
case 100:
{
setState(1127);
((ExprContext)_localctx).it = match(R_BRACE);
setState(1128);
((ExprContext)_localctx).uid0 = match(UID);
setState(1129);
match(T__3);
setState(1130);
match(REPORTING_RATE_TYPE);
setState(1131);
match(T__4);
}
break;
case 101:
{
setState(1132);
((ExprContext)_localctx).it = match(DAYS);
}
break;
case 102:
{
setState(1133);
((ExprContext)_localctx).it = match(V_BRACE);
setState(1134);
programVariable();
setState(1135);
match(T__4);
}
break;
case 103:
{
setState(1137);
numericLiteral();
}
break;
case 104:
{
setState(1138);
stringLiteral();
}
break;
case 105:
{
setState(1139);
booleanLiteral();
}
break;
}
_ctx.stop = _input.LT(-1);
setState(1205);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,107,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
if ( _parseListeners!=null ) triggerExitRuleEvent();
_prevctx = _localctx;
{
setState(1203);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,106,_ctx) ) {
case 1:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1142);
if (!(precpred(_ctx, 110))) throw new FailedPredicateException(this, "precpred(_ctx, 110)");
setState(1143);
((ExprContext)_localctx).it = match(POWER);
setState(1144);
expr(110);
}
break;
case 2:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1145);
if (!(precpred(_ctx, 108))) throw new FailedPredicateException(this, "precpred(_ctx, 108)");
setState(1146);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << MUL) | (1L << DIV) | (1L << MOD))) != 0)) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1147);
expr(109);
}
break;
case 3:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1148);
if (!(precpred(_ctx, 107))) throw new FailedPredicateException(this, "precpred(_ctx, 107)");
setState(1149);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==PLUS || _la==MINUS) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1150);
expr(108);
}
break;
case 4:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1151);
if (!(precpred(_ctx, 106))) throw new FailedPredicateException(this, "precpred(_ctx, 106)");
setState(1152);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << GT) | (1L << LT) | (1L << GEQ) | (1L << LEQ))) != 0)) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1153);
expr(107);
}
break;
case 5:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1154);
if (!(precpred(_ctx, 105))) throw new FailedPredicateException(this, "precpred(_ctx, 105)");
setState(1155);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==EQ || _la==NE) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1156);
expr(106);
}
break;
case 6:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1157);
if (!(precpred(_ctx, 104))) throw new FailedPredicateException(this, "precpred(_ctx, 104)");
setState(1158);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==AND || _la==AMPERSAND_2) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1159);
expr(105);
}
break;
case 7:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1160);
if (!(precpred(_ctx, 103))) throw new FailedPredicateException(this, "precpred(_ctx, 103)");
setState(1161);
((ExprContext)_localctx).it = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==OR || _la==VERTICAL_BAR_2) ) {
((ExprContext)_localctx).it = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1162);
expr(104);
}
break;
case 8:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1163);
if (!(precpred(_ctx, 114))) throw new FailedPredicateException(this, "precpred(_ctx, 114)");
setState(1165);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(1164);
match(WS);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(1167);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,101,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
break;
case 9:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1169);
if (!(precpred(_ctx, 112))) throw new FailedPredicateException(this, "precpred(_ctx, 112)");
setState(1170);
((ExprContext)_localctx).it = match(PERIOD_OFFSET);
setState(1174);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(1171);
match(WS);
}
}
setState(1176);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1177);
((ExprContext)_localctx).period = integerLiteral();
setState(1181);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(1178);
match(WS);
}
}
setState(1183);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1184);
match(T__0);
}
break;
case 10:
{
_localctx = new ExprContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_expr);
setState(1186);
if (!(precpred(_ctx, 111))) throw new FailedPredicateException(this, "precpred(_ctx, 111)");
setState(1187);
((ExprContext)_localctx).it = match(STAGE_OFFSET);
setState(1191);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(1188);
match(WS);
}
}
setState(1193);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1194);
((ExprContext)_localctx).stage = integerLiteral();
setState(1198);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(1195);
match(WS);
}
}
setState(1200);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1201);
match(T__0);
}
break;
}
}
}
setState(1207);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,107,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
unrollRecursionContexts(_parentctx);
}
return _localctx;
}
public static class ProgramVariableContext extends ParserRuleContext {
public Token var;
public TerminalNode V_ANALYTICS_PERIOD_END() { return getToken(ExpressionParser.V_ANALYTICS_PERIOD_END, 0); }
public TerminalNode V_ANALYTICS_PERIOD_START() { return getToken(ExpressionParser.V_ANALYTICS_PERIOD_START, 0); }
public TerminalNode V_COMPLETED_DATE() { return getToken(ExpressionParser.V_COMPLETED_DATE, 0); }
public TerminalNode V_CREATION_DATE() { return getToken(ExpressionParser.V_CREATION_DATE, 0); }
public TerminalNode V_CURRENT_DATE() { return getToken(ExpressionParser.V_CURRENT_DATE, 0); }
public TerminalNode V_DUE_DATE() { return getToken(ExpressionParser.V_DUE_DATE, 0); }
public TerminalNode V_ENROLLMENT_COUNT() { return getToken(ExpressionParser.V_ENROLLMENT_COUNT, 0); }
public TerminalNode V_ENROLLMENT_DATE() { return getToken(ExpressionParser.V_ENROLLMENT_DATE, 0); }
public TerminalNode V_ENROLLMENT_ID() { return getToken(ExpressionParser.V_ENROLLMENT_ID, 0); }
public TerminalNode V_ENROLLMENT_STATUS() { return getToken(ExpressionParser.V_ENROLLMENT_STATUS, 0); }
public TerminalNode V_ENVIRONMENT() { return getToken(ExpressionParser.V_ENVIRONMENT, 0); }
public TerminalNode V_EVENT_COUNT() { return getToken(ExpressionParser.V_EVENT_COUNT, 0); }
public TerminalNode V_EVENT_DATE() { return getToken(ExpressionParser.V_EVENT_DATE, 0); }
public TerminalNode V_EVENT_ID() { return getToken(ExpressionParser.V_EVENT_ID, 0); }
public TerminalNode V_EVENT_STATUS() { return getToken(ExpressionParser.V_EVENT_STATUS, 0); }
public TerminalNode V_EXECUTION_DATE() { return getToken(ExpressionParser.V_EXECUTION_DATE, 0); }
public TerminalNode V_INCIDENT_DATE() { return getToken(ExpressionParser.V_INCIDENT_DATE, 0); }
public TerminalNode V_ORG_UNIT_COUNT() { return getToken(ExpressionParser.V_ORG_UNIT_COUNT, 0); }
public TerminalNode V_OU() { return getToken(ExpressionParser.V_OU, 0); }
public TerminalNode V_OU_CODE() { return getToken(ExpressionParser.V_OU_CODE, 0); }
public TerminalNode V_PROGRAM_NAME() { return getToken(ExpressionParser.V_PROGRAM_NAME, 0); }
public TerminalNode V_PROGRAM_STAGE_ID() { return getToken(ExpressionParser.V_PROGRAM_STAGE_ID, 0); }
public TerminalNode V_PROGRAM_STAGE_NAME() { return getToken(ExpressionParser.V_PROGRAM_STAGE_NAME, 0); }
public TerminalNode V_SYNC_DATE() { return getToken(ExpressionParser.V_SYNC_DATE, 0); }
public TerminalNode V_TEI_COUNT() { return getToken(ExpressionParser.V_TEI_COUNT, 0); }
public TerminalNode V_VALUE_COUNT() { return getToken(ExpressionParser.V_VALUE_COUNT, 0); }
public TerminalNode V_ZERO_POS_VALUE_COUNT() { return getToken(ExpressionParser.V_ZERO_POS_VALUE_COUNT, 0); }
public ProgramVariableContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_programVariable; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterProgramVariable(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitProgramVariable(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitProgramVariable(this);
else return visitor.visitChildren(this);
}
}
public final ProgramVariableContext programVariable() throws RecognitionException {
ProgramVariableContext _localctx = new ProgramVariableContext(_ctx, getState());
enterRule(_localctx, 4, RULE_programVariable);
try {
setState(1235);
_errHandler.sync(this);
switch (_input.LA(1)) {
case V_ANALYTICS_PERIOD_END:
enterOuterAlt(_localctx, 1);
{
setState(1208);
((ProgramVariableContext)_localctx).var = match(V_ANALYTICS_PERIOD_END);
}
break;
case V_ANALYTICS_PERIOD_START:
enterOuterAlt(_localctx, 2);
{
setState(1209);
((ProgramVariableContext)_localctx).var = match(V_ANALYTICS_PERIOD_START);
}
break;
case V_COMPLETED_DATE:
enterOuterAlt(_localctx, 3);
{
setState(1210);
((ProgramVariableContext)_localctx).var = match(V_COMPLETED_DATE);
}
break;
case V_CREATION_DATE:
enterOuterAlt(_localctx, 4);
{
setState(1211);
((ProgramVariableContext)_localctx).var = match(V_CREATION_DATE);
}
break;
case V_CURRENT_DATE:
enterOuterAlt(_localctx, 5);
{
setState(1212);
((ProgramVariableContext)_localctx).var = match(V_CURRENT_DATE);
}
break;
case V_DUE_DATE:
enterOuterAlt(_localctx, 6);
{
setState(1213);
((ProgramVariableContext)_localctx).var = match(V_DUE_DATE);
}
break;
case V_ENROLLMENT_COUNT:
enterOuterAlt(_localctx, 7);
{
setState(1214);
((ProgramVariableContext)_localctx).var = match(V_ENROLLMENT_COUNT);
}
break;
case V_ENROLLMENT_DATE:
enterOuterAlt(_localctx, 8);
{
setState(1215);
((ProgramVariableContext)_localctx).var = match(V_ENROLLMENT_DATE);
}
break;
case V_ENROLLMENT_ID:
enterOuterAlt(_localctx, 9);
{
setState(1216);
((ProgramVariableContext)_localctx).var = match(V_ENROLLMENT_ID);
}
break;
case V_ENROLLMENT_STATUS:
enterOuterAlt(_localctx, 10);
{
setState(1217);
((ProgramVariableContext)_localctx).var = match(V_ENROLLMENT_STATUS);
}
break;
case V_ENVIRONMENT:
enterOuterAlt(_localctx, 11);
{
setState(1218);
((ProgramVariableContext)_localctx).var = match(V_ENVIRONMENT);
}
break;
case V_EVENT_COUNT:
enterOuterAlt(_localctx, 12);
{
setState(1219);
((ProgramVariableContext)_localctx).var = match(V_EVENT_COUNT);
}
break;
case V_EVENT_DATE:
enterOuterAlt(_localctx, 13);
{
setState(1220);
((ProgramVariableContext)_localctx).var = match(V_EVENT_DATE);
}
break;
case V_EVENT_ID:
enterOuterAlt(_localctx, 14);
{
setState(1221);
((ProgramVariableContext)_localctx).var = match(V_EVENT_ID);
}
break;
case V_EVENT_STATUS:
enterOuterAlt(_localctx, 15);
{
setState(1222);
((ProgramVariableContext)_localctx).var = match(V_EVENT_STATUS);
}
break;
case V_EXECUTION_DATE:
enterOuterAlt(_localctx, 16);
{
setState(1223);
((ProgramVariableContext)_localctx).var = match(V_EXECUTION_DATE);
}
break;
case V_INCIDENT_DATE:
enterOuterAlt(_localctx, 17);
{
setState(1224);
((ProgramVariableContext)_localctx).var = match(V_INCIDENT_DATE);
}
break;
case V_ORG_UNIT_COUNT:
enterOuterAlt(_localctx, 18);
{
setState(1225);
((ProgramVariableContext)_localctx).var = match(V_ORG_UNIT_COUNT);
}
break;
case V_OU:
enterOuterAlt(_localctx, 19);
{
setState(1226);
((ProgramVariableContext)_localctx).var = match(V_OU);
}
break;
case V_OU_CODE:
enterOuterAlt(_localctx, 20);
{
setState(1227);
((ProgramVariableContext)_localctx).var = match(V_OU_CODE);
}
break;
case V_PROGRAM_NAME:
enterOuterAlt(_localctx, 21);
{
setState(1228);
((ProgramVariableContext)_localctx).var = match(V_PROGRAM_NAME);
}
break;
case V_PROGRAM_STAGE_ID:
enterOuterAlt(_localctx, 22);
{
setState(1229);
((ProgramVariableContext)_localctx).var = match(V_PROGRAM_STAGE_ID);
}
break;
case V_PROGRAM_STAGE_NAME:
enterOuterAlt(_localctx, 23);
{
setState(1230);
((ProgramVariableContext)_localctx).var = match(V_PROGRAM_STAGE_NAME);
}
break;
case V_SYNC_DATE:
enterOuterAlt(_localctx, 24);
{
setState(1231);
((ProgramVariableContext)_localctx).var = match(V_SYNC_DATE);
}
break;
case V_TEI_COUNT:
enterOuterAlt(_localctx, 25);
{
setState(1232);
((ProgramVariableContext)_localctx).var = match(V_TEI_COUNT);
}
break;
case V_VALUE_COUNT:
enterOuterAlt(_localctx, 26);
{
setState(1233);
((ProgramVariableContext)_localctx).var = match(V_VALUE_COUNT);
}
break;
case V_ZERO_POS_VALUE_COUNT:
enterOuterAlt(_localctx, 27);
{
setState(1234);
((ProgramVariableContext)_localctx).var = match(V_ZERO_POS_VALUE_COUNT);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NumericLiteralContext extends ParserRuleContext {
public TerminalNode NUMERIC_LITERAL() { return getToken(ExpressionParser.NUMERIC_LITERAL, 0); }
public TerminalNode INTEGER_LITERAL() { return getToken(ExpressionParser.INTEGER_LITERAL, 0); }
public NumericLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numericLiteral; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterNumericLiteral(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitNumericLiteral(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitNumericLiteral(this);
else return visitor.visitChildren(this);
}
}
public final NumericLiteralContext numericLiteral() throws RecognitionException {
NumericLiteralContext _localctx = new NumericLiteralContext(_ctx, getState());
enterRule(_localctx, 6, RULE_numericLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1237);
_la = _input.LA(1);
if ( !(_la==INTEGER_LITERAL || _la==NUMERIC_LITERAL) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntegerLiteralContext extends ParserRuleContext {
public TerminalNode INTEGER_LITERAL() { return getToken(ExpressionParser.INTEGER_LITERAL, 0); }
public TerminalNode MINUS() { return getToken(ExpressionParser.MINUS, 0); }
public TerminalNode PLUS() { return getToken(ExpressionParser.PLUS, 0); }
public IntegerLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_integerLiteral; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterIntegerLiteral(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitIntegerLiteral(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitIntegerLiteral(this);
else return visitor.visitChildren(this);
}
}
public final IntegerLiteralContext integerLiteral() throws RecognitionException {
IntegerLiteralContext _localctx = new IntegerLiteralContext(_ctx, getState());
enterRule(_localctx, 8, RULE_integerLiteral);
try {
setState(1244);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INTEGER_LITERAL:
enterOuterAlt(_localctx, 1);
{
setState(1239);
match(INTEGER_LITERAL);
}
break;
case MINUS:
enterOuterAlt(_localctx, 2);
{
setState(1240);
match(MINUS);
setState(1241);
match(INTEGER_LITERAL);
}
break;
case PLUS:
enterOuterAlt(_localctx, 3);
{
setState(1242);
match(PLUS);
setState(1243);
match(INTEGER_LITERAL);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ProgramRuleStringVariableNameContext extends ParserRuleContext {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public ProgramRuleStringVariableNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_programRuleStringVariableName; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterProgramRuleStringVariableName(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitProgramRuleStringVariableName(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitProgramRuleStringVariableName(this);
else return visitor.visitChildren(this);
}
}
public final ProgramRuleStringVariableNameContext programRuleStringVariableName() throws RecognitionException {
ProgramRuleStringVariableNameContext _localctx = new ProgramRuleStringVariableNameContext(_ctx, getState());
enterRule(_localctx, 10, RULE_programRuleStringVariableName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1246);
stringLiteral();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StringLiteralContext extends ParserRuleContext {
public TerminalNode STRING_LITERAL() { return getToken(ExpressionParser.STRING_LITERAL, 0); }
public TerminalNode QUOTED_UID() { return getToken(ExpressionParser.QUOTED_UID, 0); }
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringLiteral; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterStringLiteral(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitStringLiteral(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitStringLiteral(this);
else return visitor.visitChildren(this);
}
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 12, RULE_stringLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1248);
_la = _input.LA(1);
if ( !(_la==QUOTED_UID || _la==STRING_LITERAL) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BooleanLiteralContext extends ParserRuleContext {
public TerminalNode BOOLEAN_LITERAL() { return getToken(ExpressionParser.BOOLEAN_LITERAL, 0); }
public BooleanLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_booleanLiteral; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterBooleanLiteral(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitBooleanLiteral(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitBooleanLiteral(this);
else return visitor.visitChildren(this);
}
}
public final BooleanLiteralContext booleanLiteral() throws RecognitionException {
BooleanLiteralContext _localctx = new BooleanLiteralContext(_ctx, getState());
enterRule(_localctx, 14, RULE_booleanLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(1250);
match(BOOLEAN_LITERAL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ProgramRuleVariableNameContext extends ParserRuleContext {
public List programRuleVariablePart() {
return getRuleContexts(ProgramRuleVariablePartContext.class);
}
public ProgramRuleVariablePartContext programRuleVariablePart(int i) {
return getRuleContext(ProgramRuleVariablePartContext.class,i);
}
public ProgramRuleVariableNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_programRuleVariableName; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterProgramRuleVariableName(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitProgramRuleVariableName(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitProgramRuleVariableName(this);
else return visitor.visitChildren(this);
}
}
public final ProgramRuleVariableNameContext programRuleVariableName() throws RecognitionException {
ProgramRuleVariableNameContext _localctx = new ProgramRuleVariableNameContext(_ctx, getState());
enterRule(_localctx, 16, RULE_programRuleVariableName);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1253);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1252);
programRuleVariablePart();
}
}
setState(1255);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==T__3 || _la==MINUS || ((((_la - 125)) & ~0x3f) == 0 && ((1L << (_la - 125)) & ((1L << (INTEGER_LITERAL - 125)) | (1L << (NUMERIC_LITERAL - 125)) | (1L << (UID - 125)) | (1L << (IDENTIFIER - 125)) | (1L << (WS - 125)))) != 0) );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ProgramRuleVariablePartContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ExpressionParser.IDENTIFIER, 0); }
public TerminalNode INTEGER_LITERAL() { return getToken(ExpressionParser.INTEGER_LITERAL, 0); }
public TerminalNode NUMERIC_LITERAL() { return getToken(ExpressionParser.NUMERIC_LITERAL, 0); }
public TerminalNode UID() { return getToken(ExpressionParser.UID, 0); }
public TerminalNode WS() { return getToken(ExpressionParser.WS, 0); }
public TerminalNode MINUS() { return getToken(ExpressionParser.MINUS, 0); }
public ProgramRuleVariablePartContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_programRuleVariablePart; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).enterProgramRuleVariablePart(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ExpressionListener ) ((ExpressionListener)listener).exitProgramRuleVariablePart(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ExpressionVisitor ) return ((ExpressionVisitor extends T>)visitor).visitProgramRuleVariablePart(this);
else return visitor.visitChildren(this);
}
}
public final ProgramRuleVariablePartContext programRuleVariablePart() throws RecognitionException {
ProgramRuleVariablePartContext _localctx = new ProgramRuleVariablePartContext(_ctx, getState());
enterRule(_localctx, 18, RULE_programRuleVariablePart);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1257);
_la = _input.LA(1);
if ( !(_la==T__3 || _la==MINUS || ((((_la - 125)) & ~0x3f) == 0 && ((1L << (_la - 125)) & ((1L << (INTEGER_LITERAL - 125)) | (1L << (NUMERIC_LITERAL - 125)) | (1L << (UID - 125)) | (1L << (IDENTIFIER - 125)) | (1L << (WS - 125)))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 1:
return expr_sempred((ExprContext)_localctx, predIndex);
}
return true;
}
private boolean expr_sempred(ExprContext _localctx, int predIndex) {
switch (predIndex) {
case 0:
return precpred(_ctx, 110);
case 1:
return precpred(_ctx, 108);
case 2:
return precpred(_ctx, 107);
case 3:
return precpred(_ctx, 106);
case 4:
return precpred(_ctx, 105);
case 5:
return precpred(_ctx, 104);
case 6:
return precpred(_ctx, 103);
case 7:
return precpred(_ctx, 114);
case 8:
return precpred(_ctx, 112);
case 9:
return precpred(_ctx, 111);
}
return true;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3\u008e\u04ee\4\2\t"+
"\2\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13"+
"\t\13\3\2\3\2\3\2\3\3\3\3\6\3\34\n\3\r\3\16\3\35\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\7\3+\n\3\f\3\16\3.\13\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\7\3\66\n\3\f\3\16\39\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3Q\n\3\f\3\16\3T\13\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\5\3\\\n\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3f"+
"\n\3\f\3\16\3i\13\3\3\3\3\3\7\3m\n\3\f\3\16\3p\13\3\3\3\3\3\7\3t\n\3\f"+
"\3\16\3w\13\3\3\3\3\3\7\3{\n\3\f\3\16\3~\13\3\7\3\u0080\n\3\f\3\16\3\u0083"+
"\13\3\3\3\3\3\3\3\7\3\u0088\n\3\f\3\16\3\u008b\13\3\3\3\3\3\7\3\u008f"+
"\n\3\f\3\16\3\u0092\13\3\3\3\3\3\7\3\u0096\n\3\f\3\16\3\u0099\13\3\3\3"+
"\3\3\7\3\u009d\n\3\f\3\16\3\u00a0\13\3\7\3\u00a2\n\3\f\3\16\3\u00a5\13"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u00ae\n\3\f\3\16\3\u00b1\13\3\3\3\5"+
"\3\u00b4\n\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\7\3\u00ed\n\3\f\3\16\3\u00f0\13\3\3\3\3\3\3\3\3"+
"\3\7\3\u00f6\n\3\f\3\16\3\u00f9\13\3\3\3\3\3\7\3\u00fd\n\3\f\3\16\3\u0100"+
"\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u010a\n\3\f\3\16\3\u010d\13"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u0115\n\3\f\3\16\3\u0118\13\3\3\3\3\3\3"+
"\3\7\3\u011d\n\3\f\3\16\3\u0120\13\3\3\3\3\3\3\3\3\3\7\3\u0126\n\3\f\3"+
"\16\3\u0129\13\3\3\3\3\3\3\3\3\3\7\3\u012f\n\3\f\3\16\3\u0132\13\3\3\3"+
"\3\3\3\3\3\3\7\3\u0138\n\3\f\3\16\3\u013b\13\3\3\3\3\3\3\3\3\3\7\3\u0141"+
"\n\3\f\3\16\3\u0144\13\3\3\3\3\3\7\3\u0148\n\3\f\3\16\3\u014b\13\3\3\3"+
"\3\3\3\3\3\3\7\3\u0151\n\3\f\3\16\3\u0154\13\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\7\3\u015c\n\3\f\3\16\3\u015f\13\3\3\3\3\3\7\3\u0163\n\3\f\3\16\3\u0166"+
"\13\3\3\3\3\3\7\3\u016a\n\3\f\3\16\3\u016d\13\3\3\3\3\3\3\3\3\3\7\3\u0173"+
"\n\3\f\3\16\3\u0176\13\3\3\3\3\3\3\3\3\3\7\3\u017c\n\3\f\3\16\3\u017f"+
"\13\3\3\3\3\3\7\3\u0183\n\3\f\3\16\3\u0186\13\3\3\3\3\3\7\3\u018a\n\3"+
"\f\3\16\3\u018d\13\3\3\3\3\3\3\3\3\3\7\3\u0193\n\3\f\3\16\3\u0196\13\3"+
"\3\3\3\3\3\3\3\3\7\3\u019c\n\3\f\3\16\3\u019f\13\3\3\3\3\3\7\3\u01a3\n"+
"\3\f\3\16\3\u01a6\13\3\3\3\3\3\7\3\u01aa\n\3\f\3\16\3\u01ad\13\3\3\3\3"+
"\3\3\3\3\3\7\3\u01b3\n\3\f\3\16\3\u01b6\13\3\3\3\3\3\7\3\u01ba\n\3\f\3"+
"\16\3\u01bd\13\3\3\3\3\3\7\3\u01c1\n\3\f\3\16\3\u01c4\13\3\3\3\3\3\7\3"+
"\u01c8\n\3\f\3\16\3\u01cb\13\3\3\3\3\3\3\3\3\3\7\3\u01d1\n\3\f\3\16\3"+
"\u01d4\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u01dc\n\3\f\3\16\3\u01df\13\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u01e7\n\3\f\3\16\3\u01ea\13\3\3\3\3\3\3\3"+
"\3\3\7\3\u01f0\n\3\f\3\16\3\u01f3\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u01fb"+
"\n\3\f\3\16\3\u01fe\13\3\3\3\3\3\3\3\3\3\7\3\u0204\n\3\f\3\16\3\u0207"+
"\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u020f\n\3\f\3\16\3\u0212\13\3\3\3\3"+
"\3\7\3\u0216\n\3\f\3\16\3\u0219\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u0221"+
"\n\3\f\3\16\3\u0224\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u022c\n\3\f\3\16"+
"\3\u022f\13\3\3\3\3\3\3\3\7\3\u0234\n\3\f\3\16\3\u0237\13\3\3\3\3\3\3"+
"\3\3\3\7\3\u023d\n\3\f\3\16\3\u0240\13\3\3\3\3\3\3\3\3\3\7\3\u0246\n\3"+
"\f\3\16\3\u0249\13\3\3\3\3\3\3\3\3\3\7\3\u024f\n\3\f\3\16\3\u0252\13\3"+
"\3\3\3\3\3\3\3\3\7\3\u0258\n\3\f\3\16\3\u025b\13\3\3\3\3\3\7\3\u025f\n"+
"\3\f\3\16\3\u0262\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u0276\n\3\f\3\16\3\u0279\13\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\7\3\u0281\n\3\f\3\16\3\u0284\13\3\3\3\3\3\3\3\7\3\u0289\n"+
"\3\f\3\16\3\u028c\13\3\3\3\3\3\3\3\3\3\7\3\u0292\n\3\f\3\16\3\u0295\13"+
"\3\3\3\3\3\3\3\3\3\7\3\u029b\n\3\f\3\16\3\u029e\13\3\3\3\3\3\7\3\u02a2"+
"\n\3\f\3\16\3\u02a5\13\3\3\3\3\3\3\3\3\3\7\3\u02ab\n\3\f\3\16\3\u02ae"+
"\13\3\3\3\3\3\3\3\3\3\7\3\u02b4\n\3\f\3\16\3\u02b7\13\3\3\3\3\3\3\3\7"+
"\3\u02bc\n\3\f\3\16\3\u02bf\13\3\3\3\3\3\3\3\3\3\7\3\u02c5\n\3\f\3\16"+
"\3\u02c8\13\3\3\3\3\3\3\3\3\3\7\3\u02ce\n\3\f\3\16\3\u02d1\13\3\3\3\3"+
"\3\3\3\3\3\7\3\u02d7\n\3\f\3\16\3\u02da\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u02f2"+
"\n\3\f\3\16\3\u02f5\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u02fd\n\3\f\3\16"+
"\3\u0300\13\3\3\3\3\3\3\3\7\3\u0305\n\3\f\3\16\3\u0308\13\3\3\3\3\3\3"+
"\3\3\3\7\3\u030e\n\3\f\3\16\3\u0311\13\3\3\3\3\3\3\3\3\3\7\3\u0317\n\3"+
"\f\3\16\3\u031a\13\3\3\3\3\3\3\3\3\3\7\3\u0320\n\3\f\3\16\3\u0323\13\3"+
"\3\3\3\3\3\3\3\3\7\3\u0329\n\3\f\3\16\3\u032c\13\3\3\3\3\3\7\3\u0330\n"+
"\3\f\3\16\3\u0333\13\3\3\3\3\3\3\3\3\3\7\3\u0339\n\3\f\3\16\3\u033c\13"+
"\3\3\3\3\3\7\3\u0340\n\3\f\3\16\3\u0343\13\3\3\3\3\3\7\3\u0347\n\3\f\3"+
"\16\3\u034a\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u0355\n\3\f\3"+
"\16\3\u0358\13\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u0360\n\3\f\3\16\3\u0363"+
"\13\3\3\3\3\3\3\3\7\3\u0368\n\3\f\3\16\3\u036b\13\3\3\3\3\3\3\3\3\3\7"+
"\3\u0371\n\3\f\3\16\3\u0374\13\3\3\3\3\3\3\3\3\3\7\3\u037a\n\3\f\3\16"+
"\3\u037d\13\3\3\3\3\3\3\3\3\3\7\3\u0383\n\3\f\3\16\3\u0386\13\3\3\3\3"+
"\3\3\3\3\3\7\3\u038c\n\3\f\3\16\3\u038f\13\3\3\3\3\3\7\3\u0393\n\3\f\3"+
"\16\3\u0396\13\3\3\3\3\3\3\3\3\3\7\3\u039c\n\3\f\3\16\3\u039f\13\3\3\3"+
"\3\3\7\3\u03a3\n\3\f\3\16\3\u03a6\13\3\3\3\3\3\7\3\u03aa\n\3\f\3\16\3"+
"\u03ad\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\7\3\u03c2\n\3\f\3\16\3\u03c5\13\3\3\3\5\3\u03c8\n\3"+
"\3\3\7\3\u03cb\n\3\f\3\16\3\u03ce\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\7\3\u0405\n\3\f\3\16\3\u0408"+
"\13\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\5\3\u0427\n\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\7\3\u0464\n\3\f\3\16\3\u0467\13\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\5\3\u0477\n\3\3\3\3\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3\3"+
"\3\3\3\3\6\3\u0490\n\3\r\3\16\3\u0491\3\3\3\3\3\3\7\3\u0497\n\3\f\3\16"+
"\3\u049a\13\3\3\3\3\3\7\3\u049e\n\3\f\3\16\3\u04a1\13\3\3\3\3\3\3\3\3"+
"\3\3\3\7\3\u04a8\n\3\f\3\16\3\u04ab\13\3\3\3\3\3\7\3\u04af\n\3\f\3\16"+
"\3\u04b2\13\3\3\3\3\3\7\3\u04b6\n\3\f\3\16\3\u04b9\13\3\3\4\3\4\3\4\3"+
"\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4\3\4"+
"\3\4\3\4\3\4\3\4\3\4\3\4\5\4\u04d6\n\4\3\5\3\5\3\6\3\6\3\6\3\6\3\6\5\6"+
"\u04df\n\6\3\7\3\7\3\b\3\b\3\t\3\t\3\n\6\n\u04e8\n\n\r\n\16\n\u04e9\3"+
"\13\3\13\3\13\2\3\4\f\2\4\6\b\n\f\16\20\22\24\2\16\5\2\r\16\31\31\34\34"+
"\3\2\u0086\u0087\4\2\u0086\u0086\u0088\u0088\3\2\20\22\3\2\r\16\3\2\25"+
"\30\3\2\23\24\4\2\32\32\35\35\4\2\33\33\36\36\3\2\177\u0080\3\2\u0082"+
"\u0083\b\2\6\6\16\16\177\u0080\u0086\u0086\u008c\u008c\u008e\u008e\2\u05db"+
"\2\26\3\2\2\2\4\u0476\3\2\2\2\6\u04d5\3\2\2\2\b\u04d7\3\2\2\2\n\u04de"+
"\3\2\2\2\f\u04e0\3\2\2\2\16\u04e2\3\2\2\2\20\u04e4\3\2\2\2\22\u04e7\3"+
"\2\2\2\24\u04eb\3\2\2\2\26\27\5\4\3\2\27\30\7\2\2\3\30\3\3\2\2\2\31\33"+
"\b\3\1\2\32\34\7\u008e\2\2\33\32\3\2\2\2\34\35\3\2\2\2\35\33\3\2\2\2\35"+
"\36\3\2\2\2\36\37\3\2\2\2\37\u0477\5\4\3u !\7\n\2\2!\"\5\4\3\2\"#\7\3"+
"\2\2#\u0477\3\2\2\2$%\t\2\2\2%\u0477\5\4\3o&\'\7\37\2\2\',\5\4\3\2()\7"+
"\4\2\2)+\5\4\3\2*(\3\2\2\2+.\3\2\2\2,*\3\2\2\2,-\3\2\2\2-/\3\2\2\2.,\3"+
"\2\2\2/\60\7\3\2\2\60\u0477\3\2\2\2\61\62\7 \2\2\62\67\5\4\3\2\63\64\7"+
"\4\2\2\64\66\5\4\3\2\65\63\3\2\2\2\669\3\2\2\2\67\65\3\2\2\2\678\3\2\2"+
"\28:\3\2\2\29\67\3\2\2\2:;\7\3\2\2;\u0477\3\2\2\2<=\7!\2\2=>\5\4\3\2>"+
"?\7\4\2\2?@\5\4\3\2@A\7\4\2\2AB\5\4\3\2BC\7\3\2\2C\u0477\3\2\2\2DE\7\""+
"\2\2EF\5\4\3\2FG\7\3\2\2G\u0477\3\2\2\2HI\7#\2\2IJ\5\4\3\2JK\7\3\2\2K"+
"\u0477\3\2\2\2LM\7$\2\2MR\5\4\3\2NO\7\4\2\2OQ\5\4\3\2PN\3\2\2\2QT\3\2"+
"\2\2RP\3\2\2\2RS\3\2\2\2SU\3\2\2\2TR\3\2\2\2UV\7\3\2\2V\u0477\3\2\2\2"+
"WX\7%\2\2X[\5\4\3\2YZ\7\4\2\2Z\\\5\4\3\2[Y\3\2\2\2[\\\3\2\2\2\\]\3\2\2"+
"\2]^\7\3\2\2^\u0477\3\2\2\2_`\7&\2\2`a\5\4\3\2ab\7\3\2\2b\u0477\3\2\2"+
"\2cg\7\'\2\2df\7\u008e\2\2ed\3\2\2\2fi\3\2\2\2ge\3\2\2\2gh\3\2\2\2hj\3"+
"\2\2\2ig\3\2\2\2jn\7\u0086\2\2km\7\u008e\2\2lk\3\2\2\2mp\3\2\2\2nl\3\2"+
"\2\2no\3\2\2\2o\u0081\3\2\2\2pn\3\2\2\2qu\7\4\2\2rt\7\u008e\2\2sr\3\2"+
"\2\2tw\3\2\2\2us\3\2\2\2uv\3\2\2\2vx\3\2\2\2wu\3\2\2\2x|\7\u0086\2\2y"+
"{\7\u008e\2\2zy\3\2\2\2{~\3\2\2\2|z\3\2\2\2|}\3\2\2\2}\u0080\3\2\2\2~"+
"|\3\2\2\2\177q\3\2\2\2\u0080\u0083\3\2\2\2\u0081\177\3\2\2\2\u0081\u0082"+
"\3\2\2\2\u0082\u0084\3\2\2\2\u0083\u0081\3\2\2\2\u0084\u0477\7\3\2\2\u0085"+
"\u0089\7(\2\2\u0086\u0088\7\u008e\2\2\u0087\u0086\3\2\2\2\u0088\u008b"+
"\3\2\2\2\u0089\u0087\3\2\2\2\u0089\u008a\3\2\2\2\u008a\u008c\3\2\2\2\u008b"+
"\u0089\3\2\2\2\u008c\u0090\7\u0086\2\2\u008d\u008f\7\u008e\2\2\u008e\u008d"+
"\3\2\2\2\u008f\u0092\3\2\2\2\u0090\u008e\3\2\2\2\u0090\u0091\3\2\2\2\u0091"+
"\u00a3\3\2\2\2\u0092\u0090\3\2\2\2\u0093\u0097\7\4\2\2\u0094\u0096\7\u008e"+
"\2\2\u0095\u0094\3\2\2\2\u0096\u0099\3\2\2\2\u0097\u0095\3\2\2\2\u0097"+
"\u0098\3\2\2\2\u0098\u009a\3\2\2\2\u0099\u0097\3\2\2\2\u009a\u009e\7\u0086"+
"\2\2\u009b\u009d\7\u008e\2\2\u009c\u009b\3\2\2\2\u009d\u00a0\3\2\2\2\u009e"+
"\u009c\3\2\2\2\u009e\u009f\3\2\2\2\u009f\u00a2\3\2\2\2\u00a0\u009e\3\2"+
"\2\2\u00a1\u0093\3\2\2\2\u00a2\u00a5\3\2\2\2\u00a3\u00a1\3\2\2\2\u00a3"+
"\u00a4\3\2\2\2\u00a4\u00a6\3\2\2\2\u00a5\u00a3\3\2\2\2\u00a6\u0477\7\3"+
"\2\2\u00a7\u00a8\7)\2\2\u00a8\u00a9\5\4\3\2\u00a9\u00aa\7\3\2\2\u00aa"+
"\u0477\3\2\2\2\u00ab\u00b3\7*\2\2\u00ac\u00ae\7\u008e\2\2\u00ad\u00ac"+
"\3\2\2\2\u00ae\u00b1\3\2\2\2\u00af\u00ad\3\2\2\2\u00af\u00b0\3\2\2\2\u00b0"+
"\u00b2\3\2\2\2\u00b1\u00af\3\2\2\2\u00b2\u00b4\7\5\2\2\u00b3\u00af\3\2"+
"\2\2\u00b3\u00b4\3\2\2\2\u00b4\u00b5\3\2\2\2\u00b5\u00b6\5\4\3\2\u00b6"+
"\u00b7\7\3\2\2\u00b7\u0477\3\2\2\2\u00b8\u00b9\7+\2\2\u00b9\u00ba\5\4"+
"\3\2\u00ba\u00bb\7\3\2\2\u00bb\u0477\3\2\2\2\u00bc\u00bd\7,\2\2\u00bd"+
"\u00be\5\4\3\2\u00be\u00bf\7\3\2\2\u00bf\u0477\3\2\2\2\u00c0\u00c1\7-"+
"\2\2\u00c1\u00c2\5\4\3\2\u00c2\u00c3\7\3\2\2\u00c3\u0477\3\2\2\2\u00c4"+
"\u00c5\7.\2\2\u00c5\u00c6\5\4\3\2\u00c6\u00c7\7\4\2\2\u00c7\u00c8\5\4"+
"\3\2\u00c8\u00c9\7\3\2\2\u00c9\u0477\3\2\2\2\u00ca\u00cb\7/\2\2\u00cb"+
"\u00cc\5\4\3\2\u00cc\u00cd\7\3\2\2\u00cd\u0477\3\2\2\2\u00ce\u00cf\7\60"+
"\2\2\u00cf\u00d0\5\4\3\2\u00d0\u00d1\7\3\2\2\u00d1\u0477\3\2\2\2\u00d2"+
"\u00d3\7\61\2\2\u00d3\u00d4\5\4\3\2\u00d4\u00d5\7\3\2\2\u00d5\u0477\3"+
"\2\2\2\u00d6\u00d7\7\62\2\2\u00d7\u00d8\5\4\3\2\u00d8\u00d9\7\3\2\2\u00d9"+
"\u0477\3\2\2\2\u00da\u00db\7\63\2\2\u00db\u00dc\5\4\3\2\u00dc\u00dd\7"+
"\3\2\2\u00dd\u0477\3\2\2\2\u00de\u00df\7O\2\2\u00df\u00e0\5\4\3\2\u00e0"+
"\u00e1\7\4\2\2\u00e1\u00e2\5\4\3\2\u00e2\u00e3\7\3\2\2\u00e3\u0477\3\2"+
"\2\2\u00e4\u00e5\7P\2\2\u00e5\u00e6\5\4\3\2\u00e6\u00e7\7\3\2\2\u00e7"+
"\u0477\3\2\2\2\u00e8\u00e9\7Q\2\2\u00e9\u00ee\5\4\3\2\u00ea\u00eb\7\4"+
"\2\2\u00eb\u00ed\5\4\3\2\u00ec\u00ea\3\2\2\2\u00ed\u00f0\3\2\2\2\u00ee"+
"\u00ec\3\2\2\2\u00ee\u00ef\3\2\2\2\u00ef\u00f1\3\2\2\2\u00f0\u00ee\3\2"+
"\2\2\u00f1\u00f2\7\3\2\2\u00f2\u0477\3\2\2\2\u00f3\u00f7\7R\2\2\u00f4"+
"\u00f6\7\u008e\2\2\u00f5\u00f4\3\2\2\2\u00f6\u00f9\3\2\2\2\u00f7\u00f5"+
"\3\2\2\2\u00f7\u00f8\3\2\2\2\u00f8\u00fa\3\2\2\2\u00f9\u00f7\3\2\2\2\u00fa"+
"\u00fe\5\16\b\2\u00fb\u00fd\7\u008e\2\2\u00fc\u00fb\3\2\2\2\u00fd\u0100"+
"\3\2\2\2\u00fe\u00fc\3\2\2\2\u00fe\u00ff\3\2\2\2\u00ff\u0101\3\2\2\2\u0100"+
"\u00fe\3\2\2\2\u0101\u0102\7\4\2\2\u0102\u0103\5\4\3\2\u0103\u0104\7\4"+
"\2\2\u0104\u0105\5\4\3\2\u0105\u0106\7\3\2\2\u0106\u0477\3\2\2\2\u0107"+
"\u010b\7S\2\2\u0108\u010a\7\u008e\2\2\u0109\u0108\3\2\2\2\u010a\u010d"+
"\3\2\2\2\u010b\u0109\3\2\2\2\u010b\u010c\3\2\2\2\u010c\u010e\3\2\2\2\u010d"+
"\u010b\3\2\2\2\u010e\u010f\7r\2\2\u010f\u0110\7\u0086\2\2\u0110\u0111"+
"\7\6\2\2\u0111\u0112\7\u0086\2\2\u0112\u0116\7\7\2\2\u0113\u0115\7\u008e"+
"\2\2\u0114\u0113\3\2\2\2\u0115\u0118\3\2\2\2\u0116\u0114\3\2\2\2\u0116"+
"\u0117\3\2\2\2\u0117\u0119\3\2\2\2\u0118\u0116\3\2\2\2\u0119\u0477\7\3"+
"\2\2\u011a\u011e\7S\2\2\u011b\u011d\7\u008e\2\2\u011c\u011b\3\2\2\2\u011d"+
"\u0120\3\2\2\2\u011e\u011c\3\2\2\2\u011e\u011f\3\2\2\2\u011f\u0121\3\2"+
"\2\2\u0120\u011e\3\2\2\2\u0121\u0122\7r\2\2\u0122\u0123\5\22\n\2\u0123"+
"\u0127\7\7\2\2\u0124\u0126\7\u008e\2\2\u0125\u0124\3\2\2\2\u0126\u0129"+
"\3\2\2\2\u0127\u0125\3\2\2\2\u0127\u0128\3\2\2\2\u0128\u012a\3\2\2\2\u0129"+
"\u0127\3\2\2\2\u012a\u012b\7\3\2\2\u012b\u0477\3\2\2\2\u012c\u0130\7S"+
"\2\2\u012d\u012f\7\u008e\2\2\u012e\u012d\3\2\2\2\u012f\u0132\3\2\2\2\u0130"+
"\u012e\3\2\2\2\u0130\u0131\3\2\2\2\u0131\u0133\3\2\2\2\u0132\u0130\3\2"+
"\2\2\u0133\u0134\7s\2\2\u0134\u0135\5\22\n\2\u0135\u0139\7\7\2\2\u0136"+
"\u0138\7\u008e\2\2\u0137\u0136\3\2\2\2\u0138\u013b\3\2\2\2\u0139\u0137"+
"\3\2\2\2\u0139\u013a\3\2\2\2\u013a\u013c\3\2\2\2\u013b\u0139\3\2\2\2\u013c"+
"\u013d\7\3\2\2\u013d\u0477\3\2\2\2\u013e\u0142\7S\2\2\u013f\u0141\7\u008e"+
"\2\2\u0140\u013f\3\2\2\2\u0141\u0144\3\2\2\2\u0142\u0140\3\2\2\2\u0142"+
"\u0143\3\2\2\2\u0143\u0145\3\2\2\2\u0144\u0142\3\2\2\2\u0145\u0149\5\f"+
"\7\2\u0146\u0148\7\u008e\2\2\u0147\u0146\3\2\2\2\u0148\u014b\3\2\2\2\u0149"+
"\u0147\3\2\2\2\u0149\u014a\3\2\2\2\u014a\u014c\3\2\2\2\u014b\u0149\3\2"+
"\2\2\u014c\u014d\7\3\2\2\u014d\u0477\3\2\2\2\u014e\u0152\7T\2\2\u014f"+
"\u0151\7\u008e\2\2\u0150\u014f\3\2\2\2\u0151\u0154\3\2\2\2\u0152\u0150"+
"\3\2\2\2\u0152\u0153\3\2\2\2\u0153\u0155\3\2\2\2\u0154\u0152\3\2\2\2\u0155"+
"\u0156\7r\2\2\u0156\u0157\7\u0086\2\2\u0157\u0158\7\6\2\2\u0158\u0159"+
"\7\u0086\2\2\u0159\u015d\7\7\2\2\u015a\u015c\7\u008e\2\2\u015b\u015a\3"+
"\2\2\2\u015c\u015f\3\2\2\2\u015d\u015b\3\2\2\2\u015d\u015e\3\2\2\2\u015e"+
"\u0160\3\2\2\2\u015f\u015d\3\2\2\2\u0160\u0164\7\4\2\2\u0161\u0163\7\u008e"+
"\2\2\u0162\u0161\3\2\2\2\u0163\u0166\3\2\2\2\u0164\u0162\3\2\2\2\u0164"+
"\u0165\3\2\2\2\u0165\u0167\3\2\2\2\u0166\u0164\3\2\2\2\u0167\u016b\5\16"+
"\b\2\u0168\u016a\7\u008e\2\2\u0169\u0168\3\2\2\2\u016a\u016d\3\2\2\2\u016b"+
"\u0169\3\2\2\2\u016b\u016c\3\2\2\2\u016c\u016e\3\2\2\2\u016d\u016b\3\2"+
"\2\2\u016e\u016f\7\3\2\2\u016f\u0477\3\2\2\2\u0170\u0174\7T\2\2\u0171"+
"\u0173\7\u008e\2\2\u0172\u0171\3\2\2\2\u0173\u0176\3\2\2\2\u0174\u0172"+
"\3\2\2\2\u0174\u0175\3\2\2\2\u0175\u0177\3\2\2\2\u0176\u0174\3\2\2\2\u0177"+
"\u0178\7r\2\2\u0178\u0179\5\22\n\2\u0179\u017d\7\7\2\2\u017a\u017c\7\u008e"+
"\2\2\u017b\u017a\3\2\2\2\u017c\u017f\3\2\2\2\u017d\u017b\3\2\2\2\u017d"+
"\u017e\3\2\2\2\u017e\u0180\3\2\2\2\u017f\u017d\3\2\2\2\u0180\u0184\7\4"+
"\2\2\u0181\u0183\7\u008e\2\2\u0182\u0181\3\2\2\2\u0183\u0186\3\2\2\2\u0184"+
"\u0182\3\2\2\2\u0184\u0185\3\2\2\2\u0185\u0187\3\2\2\2\u0186\u0184\3\2"+
"\2\2\u0187\u018b\5\16\b\2\u0188\u018a\7\u008e\2\2\u0189\u0188\3\2\2\2"+
"\u018a\u018d\3\2\2\2\u018b\u0189\3\2\2\2\u018b\u018c\3\2\2\2\u018c\u018e"+
"\3\2\2\2\u018d\u018b\3\2\2\2\u018e\u018f\7\3\2\2\u018f\u0477\3\2\2\2\u0190"+
"\u0194\7T\2\2\u0191\u0193\7\u008e\2\2\u0192\u0191\3\2\2\2\u0193\u0196"+
"\3\2\2\2\u0194\u0192\3\2\2\2\u0194\u0195\3\2\2\2\u0195\u0197\3\2\2\2\u0196"+
"\u0194\3\2\2\2\u0197\u0198\7s\2\2\u0198\u0199\5\22\n\2\u0199\u019d\7\7"+
"\2\2\u019a\u019c\7\u008e\2\2\u019b\u019a\3\2\2\2\u019c\u019f\3\2\2\2\u019d"+
"\u019b\3\2\2\2\u019d\u019e\3\2\2\2\u019e\u01a0\3\2\2\2\u019f\u019d\3\2"+
"\2\2\u01a0\u01a4\7\4\2\2\u01a1\u01a3\7\u008e\2\2\u01a2\u01a1\3\2\2\2\u01a3"+
"\u01a6\3\2\2\2\u01a4\u01a2\3\2\2\2\u01a4\u01a5\3\2\2\2\u01a5\u01a7\3\2"+
"\2\2\u01a6\u01a4\3\2\2\2\u01a7\u01ab\5\16\b\2\u01a8\u01aa\7\u008e\2\2"+
"\u01a9\u01a8\3\2\2\2\u01aa\u01ad\3\2\2\2\u01ab\u01a9\3\2\2\2\u01ab\u01ac"+
"\3\2\2\2\u01ac\u01ae\3\2\2\2\u01ad\u01ab\3\2\2\2\u01ae\u01af\7\3\2\2\u01af"+
"\u0477\3\2\2\2\u01b0\u01b4\7T\2\2\u01b1\u01b3\7\u008e\2\2\u01b2\u01b1"+
"\3\2\2\2\u01b3\u01b6\3\2\2\2\u01b4\u01b2\3\2\2\2\u01b4\u01b5\3\2\2\2\u01b5"+
"\u01b7\3\2\2\2\u01b6\u01b4\3\2\2\2\u01b7\u01bb\5\f\7\2\u01b8\u01ba\7\u008e"+
"\2\2\u01b9\u01b8\3\2\2\2\u01ba\u01bd\3\2\2\2\u01bb\u01b9\3\2\2\2\u01bb"+
"\u01bc\3\2\2\2\u01bc\u01be\3\2\2\2\u01bd\u01bb\3\2\2\2\u01be\u01c2\7\4"+
"\2\2\u01bf\u01c1\7\u008e\2\2\u01c0\u01bf\3\2\2\2\u01c1\u01c4\3\2\2\2\u01c2"+
"\u01c0\3\2\2\2\u01c2\u01c3\3\2\2\2\u01c3\u01c5\3\2\2\2\u01c4\u01c2\3\2"+
"\2\2\u01c5\u01c9\5\16\b\2\u01c6\u01c8\7\u008e\2\2\u01c7\u01c6\3\2\2\2"+
"\u01c8\u01cb\3\2\2\2\u01c9\u01c7\3\2\2\2\u01c9\u01ca\3\2\2\2\u01ca\u01cc"+
"\3\2\2\2\u01cb\u01c9\3\2\2\2\u01cc\u01cd\7\3\2\2\u01cd\u0477\3\2\2\2\u01ce"+
"\u01d2\7U\2\2\u01cf\u01d1\7\u008e\2\2\u01d0\u01cf\3\2\2\2\u01d1\u01d4"+
"\3\2\2\2\u01d2\u01d0\3\2\2\2\u01d2\u01d3\3\2\2\2\u01d3\u01d5\3\2\2\2\u01d4"+
"\u01d2\3\2\2\2\u01d5\u01d6\7r\2\2\u01d6\u01d7\7\u0086\2\2\u01d7\u01d8"+
"\7\6\2\2\u01d8\u01d9\7\u0086\2\2\u01d9\u01dd\7\7\2\2\u01da\u01dc\7\u008e"+
"\2\2\u01db\u01da\3\2\2\2\u01dc\u01df\3\2\2\2\u01dd\u01db\3\2\2\2\u01dd"+
"\u01de\3\2\2\2\u01de\u01e0\3\2\2\2\u01df\u01dd\3\2\2\2\u01e0\u01e1\7\4"+
"\2\2\u01e1\u01e2\5\4\3\2\u01e2\u01e3\7\3\2\2\u01e3\u0477\3\2\2\2\u01e4"+
"\u01e8\7U\2\2\u01e5\u01e7\7\u008e\2\2\u01e6\u01e5\3\2\2\2\u01e7\u01ea"+
"\3\2\2\2\u01e8\u01e6\3\2\2\2\u01e8\u01e9\3\2\2\2\u01e9\u01eb\3\2\2\2\u01ea"+
"\u01e8\3\2\2\2\u01eb\u01ec\7r\2\2\u01ec\u01ed\5\22\n\2\u01ed\u01f1\7\7"+
"\2\2\u01ee\u01f0\7\u008e\2\2\u01ef\u01ee\3\2\2\2\u01f0\u01f3\3\2\2\2\u01f1"+
"\u01ef\3\2\2\2\u01f1\u01f2\3\2\2\2\u01f2\u01f4\3\2\2\2\u01f3\u01f1\3\2"+
"\2\2\u01f4\u01f5\7\4\2\2\u01f5\u01f6\5\4\3\2\u01f6\u01f7\7\3\2\2\u01f7"+
"\u0477\3\2\2\2\u01f8\u01fc\7U\2\2\u01f9\u01fb\7\u008e\2\2\u01fa\u01f9"+
"\3\2\2\2\u01fb\u01fe\3\2\2\2\u01fc\u01fa\3\2\2\2\u01fc\u01fd\3\2\2\2\u01fd"+
"\u01ff\3\2\2\2\u01fe\u01fc\3\2\2\2\u01ff\u0200\7s\2\2\u0200\u0201\5\22"+
"\n\2\u0201\u0205\7\7\2\2\u0202\u0204\7\u008e\2\2\u0203\u0202\3\2\2\2\u0204"+
"\u0207\3\2\2\2\u0205\u0203\3\2\2\2\u0205\u0206\3\2\2\2\u0206\u0208\3\2"+
"\2\2\u0207\u0205\3\2\2\2\u0208\u0209\7\4\2\2\u0209\u020a\5\4\3\2\u020a"+
"\u020b\7\3\2\2\u020b\u0477\3\2\2\2\u020c\u0210\7U\2\2\u020d\u020f\7\u008e"+
"\2\2\u020e\u020d\3\2\2\2\u020f\u0212\3\2\2\2\u0210\u020e\3\2\2\2\u0210"+
"\u0211\3\2\2\2\u0211\u0213\3\2\2\2\u0212\u0210\3\2\2\2\u0213\u0217\5\f"+
"\7\2\u0214\u0216\7\u008e\2\2\u0215\u0214\3\2\2\2\u0216\u0219\3\2\2\2\u0217"+
"\u0215\3\2\2\2\u0217\u0218\3\2\2\2\u0218\u021a\3\2\2\2\u0219\u0217\3\2"+
"\2\2\u021a\u021b\7\4\2\2\u021b\u021c\5\4\3\2\u021c\u021d\7\3\2\2\u021d"+
"\u0477\3\2\2\2\u021e\u0222\7V\2\2\u021f\u0221\7\u008e\2\2\u0220\u021f"+
"\3\2\2\2\u0221\u0224\3\2\2\2\u0222\u0220\3\2\2\2\u0222\u0223\3\2\2\2\u0223"+
"\u0225\3\2\2\2\u0224\u0222\3\2\2\2\u0225\u0226\7r\2\2\u0226\u0227\7\u0086"+
"\2\2\u0227\u0228\7\6\2\2\u0228\u0229\7\u0086\2\2\u0229\u022d\7\7\2\2\u022a"+
"\u022c\7\u008e\2\2\u022b\u022a\3\2\2\2\u022c\u022f\3\2\2\2\u022d\u022b"+
"\3\2\2\2\u022d\u022e\3\2\2\2\u022e\u0230\3\2\2\2\u022f\u022d\3\2\2\2\u0230"+
"\u0477\7\3\2\2\u0231\u0235\7V\2\2\u0232\u0234\7\u008e\2\2\u0233\u0232"+
"\3\2\2\2\u0234\u0237\3\2\2\2\u0235\u0233\3\2\2\2\u0235\u0236\3\2\2\2\u0236"+
"\u0238\3\2\2\2\u0237\u0235\3\2\2\2\u0238\u0239\7r\2\2\u0239\u023a\5\22"+
"\n\2\u023a\u023e\7\7\2\2\u023b\u023d\7\u008e\2\2\u023c\u023b\3\2\2\2\u023d"+
"\u0240\3\2\2\2\u023e\u023c\3\2\2\2\u023e\u023f\3\2\2\2\u023f\u0241\3\2"+
"\2\2\u0240\u023e\3\2\2\2\u0241\u0242\7\3\2\2\u0242\u0477\3\2\2\2\u0243"+
"\u0247\7V\2\2\u0244\u0246\7\u008e\2\2\u0245\u0244\3\2\2\2\u0246\u0249"+
"\3\2\2\2\u0247\u0245\3\2\2\2\u0247\u0248\3\2\2\2\u0248\u024a\3\2\2\2\u0249"+
"\u0247\3\2\2\2\u024a\u024b\7s\2\2\u024b\u024c\5\22\n\2\u024c\u0250\7\7"+
"\2\2\u024d\u024f\7\u008e\2\2\u024e\u024d\3\2\2\2\u024f\u0252\3\2\2\2\u0250"+
"\u024e\3\2\2\2\u0250\u0251\3\2\2\2\u0251\u0253\3\2\2\2\u0252\u0250\3\2"+
"\2\2\u0253\u0254\7\3\2\2\u0254\u0477\3\2\2\2\u0255\u0259\7V\2\2\u0256"+
"\u0258\7\u008e\2\2\u0257\u0256\3\2\2\2\u0258\u025b\3\2\2\2\u0259\u0257"+
"\3\2\2\2\u0259\u025a\3\2\2\2\u025a\u025c\3\2\2\2\u025b\u0259\3\2\2\2\u025c"+
"\u0260\5\f\7\2\u025d\u025f\7\u008e\2\2\u025e\u025d\3\2\2\2\u025f\u0262"+
"\3\2\2\2\u0260\u025e\3\2\2\2\u0260\u0261\3\2\2\2\u0261\u0263\3\2\2\2\u0262"+
"\u0260\3\2\2\2\u0263\u0264\7\3\2\2\u0264\u0477\3\2\2\2\u0265\u0266\7W"+
"\2\2\u0266\u0267\5\4\3\2\u0267\u0268\7\4\2\2\u0268\u0269\5\4\3\2\u0269"+
"\u026a\7\3\2\2\u026a\u0477\3\2\2\2\u026b\u026c\7X\2\2\u026c\u026d\5\4"+
"\3\2\u026d\u026e\7\3\2\2\u026e\u0477\3\2\2\2\u026f\u0270\7Y\2\2\u0270"+
"\u0271\5\4\3\2\u0271\u0272\7\3\2\2\u0272\u0477\3\2\2\2\u0273\u0277\7Z"+
"\2\2\u0274\u0276\7\u008e\2\2\u0275\u0274\3\2\2\2\u0276\u0279\3\2\2\2\u0277"+
"\u0275\3\2\2\2\u0277\u0278\3\2\2\2\u0278\u027a\3\2\2\2\u0279\u0277\3\2"+
"\2\2\u027a\u027b\7r\2\2\u027b\u027c\7\u0086\2\2\u027c\u027d\7\6\2\2\u027d"+
"\u027e\7\u0086\2\2\u027e\u0282\7\7\2\2\u027f\u0281\7\u008e\2\2\u0280\u027f"+
"\3\2\2\2\u0281\u0284\3\2\2\2\u0282\u0280\3\2\2\2\u0282\u0283\3\2\2\2\u0283"+
"\u0285\3\2\2\2\u0284\u0282\3\2\2\2\u0285\u0477\7\3\2\2\u0286\u028a\7Z"+
"\2\2\u0287\u0289\7\u008e\2\2\u0288\u0287\3\2\2\2\u0289\u028c\3\2\2\2\u028a"+
"\u0288\3\2\2\2\u028a\u028b\3\2\2\2\u028b\u028d\3\2\2\2\u028c\u028a\3\2"+
"\2\2\u028d\u028e\7r\2\2\u028e\u028f\5\22\n\2\u028f\u0293\7\7\2\2\u0290"+
"\u0292\7\u008e\2\2\u0291\u0290\3\2\2\2\u0292\u0295\3\2\2\2\u0293\u0291"+
"\3\2\2\2\u0293\u0294\3\2\2\2\u0294\u0296\3\2\2\2\u0295\u0293\3\2\2\2\u0296"+
"\u0297\7\3\2\2\u0297\u0477\3\2\2\2\u0298\u029c\7Z\2\2\u0299\u029b\7\u008e"+
"\2\2\u029a\u0299\3\2\2\2\u029b\u029e\3\2\2\2\u029c\u029a\3\2\2\2\u029c"+
"\u029d\3\2\2\2\u029d\u029f\3\2\2\2\u029e\u029c\3\2\2\2\u029f\u02a3\5\f"+
"\7\2\u02a0\u02a2\7\u008e\2\2\u02a1\u02a0\3\2\2\2\u02a2\u02a5\3\2\2\2\u02a3"+
"\u02a1\3\2\2\2\u02a3\u02a4\3\2\2\2\u02a4\u02a6\3\2\2\2\u02a5\u02a3\3\2"+
"\2\2\u02a6\u02a7\7\3\2\2\u02a7\u0477\3\2\2\2\u02a8\u02ac\7Z\2\2\u02a9"+
"\u02ab\7\u008e\2\2\u02aa\u02a9\3\2\2\2\u02ab\u02ae\3\2\2\2\u02ac\u02aa"+
"\3\2\2\2\u02ac\u02ad\3\2\2\2\u02ad\u02af\3\2\2\2\u02ae\u02ac\3\2\2\2\u02af"+
"\u02b0\7s\2\2\u02b0\u02b1\7\u0086\2\2\u02b1\u02b5\7\7\2\2\u02b2\u02b4"+
"\7\u008e\2\2\u02b3\u02b2\3\2\2\2\u02b4\u02b7\3\2\2\2\u02b5\u02b3\3\2\2"+
"\2\u02b5\u02b6\3\2\2\2\u02b6\u02b8\3\2\2\2\u02b7\u02b5\3\2\2\2\u02b8\u0477"+
"\7\3\2\2\u02b9\u02bd\7Z\2\2\u02ba\u02bc\7\u008e\2\2\u02bb\u02ba\3\2\2"+
"\2\u02bc\u02bf\3\2\2\2\u02bd\u02bb\3\2\2\2\u02bd\u02be\3\2\2\2\u02be\u02c0"+
"\3\2\2\2\u02bf\u02bd\3\2\2\2\u02c0\u02c1\7s\2\2\u02c1\u02c2\5\22\n\2\u02c2"+
"\u02c6\7\7\2\2\u02c3\u02c5\7\u008e\2\2\u02c4\u02c3\3\2\2\2\u02c5\u02c8"+
"\3\2\2\2\u02c6\u02c4\3\2\2\2\u02c6\u02c7\3\2\2\2\u02c7\u02c9\3\2\2\2\u02c8"+
"\u02c6\3\2\2\2\u02c9\u02ca\7\3\2\2\u02ca\u0477\3\2\2\2\u02cb\u02cf\7Z"+
"\2\2\u02cc\u02ce\7\u008e\2\2\u02cd\u02cc\3\2\2\2\u02ce\u02d1\3\2\2\2\u02cf"+
"\u02cd\3\2\2\2\u02cf\u02d0\3\2\2\2\u02d0\u02d2\3\2\2\2\u02d1\u02cf\3\2"+
"\2\2\u02d2\u02d3\7{\2\2\u02d3\u02d4\5\6\4\2\u02d4\u02d8\7\7\2\2\u02d5"+
"\u02d7\7\u008e\2\2\u02d6\u02d5\3\2\2\2\u02d7\u02da\3\2\2\2\u02d8\u02d6"+
"\3\2\2\2\u02d8\u02d9\3\2\2\2\u02d9\u02db\3\2\2\2\u02da\u02d8\3\2\2\2\u02db"+
"\u02dc\7\3\2\2\u02dc\u0477\3\2\2\2\u02dd\u02de\7[\2\2\u02de\u02df\5\4"+
"\3\2\u02df\u02e0\7\3\2\2\u02e0\u0477\3\2\2\2\u02e1\u02e2\7\\\2\2\u02e2"+
"\u02e3\5\4\3\2\u02e3\u02e4\7\3\2\2\u02e4\u0477\3\2\2\2\u02e5\u02e6\7]"+
"\2\2\u02e6\u02e7\5\4\3\2\u02e7\u02e8\7\4\2\2\u02e8\u02e9\5\4\3\2\u02e9"+
"\u02ea\7\3\2\2\u02ea\u0477\3\2\2\2\u02eb\u02ec\7^\2\2\u02ec\u02ed\5\4"+
"\3\2\u02ed\u02ee\7\3\2\2\u02ee\u0477\3\2\2\2\u02ef\u02f3\7_\2\2\u02f0"+
"\u02f2\7\u008e\2\2\u02f1\u02f0\3\2\2\2\u02f2\u02f5\3\2\2\2\u02f3\u02f1"+
"\3\2\2\2\u02f3\u02f4\3\2\2\2\u02f4\u02f6\3\2\2\2\u02f5\u02f3\3\2\2\2\u02f6"+
"\u02f7\7r\2\2\u02f7\u02f8\7\u0086\2\2\u02f8\u02f9\7\6\2\2\u02f9\u02fa"+
"\7\u0086\2\2\u02fa\u02fe\7\7\2\2\u02fb\u02fd\7\u008e\2\2\u02fc\u02fb\3"+
"\2\2\2\u02fd\u0300\3\2\2\2\u02fe\u02fc\3\2\2\2\u02fe\u02ff\3\2\2\2\u02ff"+
"\u0301\3\2\2\2\u0300\u02fe\3\2\2\2\u0301\u0477\7\3\2\2\u0302\u0306\7_"+
"\2\2\u0303\u0305\7\u008e\2\2\u0304\u0303\3\2\2\2\u0305\u0308\3\2\2\2\u0306"+
"\u0304\3\2\2\2\u0306\u0307\3\2\2\2\u0307\u0309\3\2\2\2\u0308\u0306\3\2"+
"\2\2\u0309\u030a\7r\2\2\u030a\u030b\5\22\n\2\u030b\u030f\7\7\2\2\u030c"+
"\u030e\7\u008e\2\2\u030d\u030c\3\2\2\2\u030e\u0311\3\2\2\2\u030f\u030d"+
"\3\2\2\2\u030f\u0310\3\2\2\2\u0310\u0312\3\2\2\2\u0311\u030f\3\2\2\2\u0312"+
"\u0313\7\3\2\2\u0313\u0477\3\2\2\2\u0314\u0318\7_\2\2\u0315\u0317\7\u008e"+
"\2\2\u0316\u0315\3\2\2\2\u0317\u031a\3\2\2\2\u0318\u0316\3\2\2\2\u0318"+
"\u0319\3\2\2\2\u0319\u031b\3\2\2\2\u031a\u0318\3\2\2\2\u031b\u031c\7s"+
"\2\2\u031c\u031d\5\22\n\2\u031d\u0321\7\7\2\2\u031e\u0320\7\u008e\2\2"+
"\u031f\u031e\3\2\2\2\u0320\u0323\3\2\2\2\u0321\u031f\3\2\2\2\u0321\u0322"+
"\3\2\2\2\u0322\u0324\3\2\2\2\u0323\u0321\3\2\2\2\u0324\u0325\7\3\2\2\u0325"+
"\u0477\3\2\2\2\u0326\u032a\7_\2\2\u0327\u0329\7\u008e\2\2\u0328\u0327"+
"\3\2\2\2\u0329\u032c\3\2\2\2\u032a\u0328\3\2\2\2\u032a\u032b\3\2\2\2\u032b"+
"\u032d\3\2\2\2\u032c\u032a\3\2\2\2\u032d\u0331\5\f\7\2\u032e\u0330\7\u008e"+
"\2\2\u032f\u032e\3\2\2\2\u0330\u0333\3\2\2\2\u0331\u032f\3\2\2\2\u0331"+
"\u0332\3\2\2\2\u0332\u0334\3\2\2\2\u0333\u0331\3\2\2\2\u0334\u0335\7\3"+
"\2\2\u0335\u0477\3\2\2\2\u0336\u033a\7_\2\2\u0337\u0339\7\u008e\2\2\u0338"+
"\u0337\3\2\2\2\u0339\u033c\3\2\2\2\u033a\u0338\3\2\2\2\u033a\u033b\3\2"+
"\2\2\u033b\u033d\3\2\2\2\u033c\u033a\3\2\2\2\u033d\u0341\7y\2\2\u033e"+
"\u0340\7\u008e\2\2\u033f\u033e\3\2\2\2\u0340\u0343\3\2\2\2\u0341\u033f"+
"\3\2\2\2\u0341\u0342\3\2\2\2\u0342\u0344\3\2\2\2\u0343\u0341\3\2\2\2\u0344"+
"\u0348\7\u0086\2\2\u0345\u0347\7\u008e\2\2\u0346\u0345\3\2\2\2\u0347\u034a"+
"\3\2\2\2\u0348\u0346\3\2\2\2\u0348\u0349\3\2\2\2\u0349\u034b\3\2\2\2\u034a"+
"\u0348\3\2\2\2\u034b\u0477\7\3\2\2\u034c\u034d\7`\2\2\u034d\u034e\5\4"+
"\3\2\u034e\u034f\7\4\2\2\u034f\u0350\5\4\3\2\u0350\u0351\7\3\2\2\u0351"+
"\u0477\3\2\2\2\u0352\u0356\7a\2\2\u0353\u0355\7\u008e\2\2\u0354\u0353"+
"\3\2\2\2\u0355\u0358\3\2\2\2\u0356\u0354\3\2\2\2\u0356\u0357\3\2\2\2\u0357"+
"\u0359\3\2\2\2\u0358\u0356\3\2\2\2\u0359\u035a\7r\2\2\u035a\u035b\7\u0086"+
"\2\2\u035b\u035c\7\6\2\2\u035c\u035d\7\u0086\2\2\u035d\u0361\7\7\2\2\u035e"+
"\u0360\7\u008e\2\2\u035f\u035e\3\2\2\2\u0360\u0363\3\2\2\2\u0361\u035f"+
"\3\2\2\2\u0361\u0362\3\2\2\2\u0362\u0364\3\2\2\2\u0363\u0361\3\2\2\2\u0364"+
"\u0477\7\3\2\2\u0365\u0369\7a\2\2\u0366\u0368\7\u008e\2\2\u0367\u0366"+
"\3\2\2\2\u0368\u036b\3\2\2\2\u0369\u0367\3\2\2\2\u0369\u036a\3\2\2\2\u036a"+
"\u036c\3\2\2\2\u036b\u0369\3\2\2\2\u036c\u036d\7r\2\2\u036d\u036e\5\22"+
"\n\2\u036e\u0372\7\7\2\2\u036f\u0371\7\u008e\2\2\u0370\u036f\3\2\2\2\u0371"+
"\u0374\3\2\2\2\u0372\u0370\3\2\2\2\u0372\u0373\3\2\2\2\u0373\u0375\3\2"+
"\2\2\u0374\u0372\3\2\2\2\u0375\u0376\7\3\2\2\u0376\u0477\3\2\2\2\u0377"+
"\u037b\7a\2\2\u0378\u037a\7\u008e\2\2\u0379\u0378\3\2\2\2\u037a\u037d"+
"\3\2\2\2\u037b\u0379\3\2\2\2\u037b\u037c\3\2\2\2\u037c\u037e\3\2\2\2\u037d"+
"\u037b\3\2\2\2\u037e\u037f\7s\2\2\u037f\u0380\5\22\n\2\u0380\u0384\7\7"+
"\2\2\u0381\u0383\7\u008e\2\2\u0382\u0381\3\2\2\2\u0383\u0386\3\2\2\2\u0384"+
"\u0382\3\2\2\2\u0384\u0385\3\2\2\2\u0385\u0387\3\2\2\2\u0386\u0384\3\2"+
"\2\2\u0387\u0388\7\3\2\2\u0388\u0477\3\2\2\2\u0389\u038d\7a\2\2\u038a"+
"\u038c\7\u008e\2\2\u038b\u038a\3\2\2\2\u038c\u038f\3\2\2\2\u038d\u038b"+
"\3\2\2\2\u038d\u038e\3\2\2\2\u038e\u0390\3\2\2\2\u038f\u038d\3\2\2\2\u0390"+
"\u0394\5\f\7\2\u0391\u0393\7\u008e\2\2\u0392\u0391\3\2\2\2\u0393\u0396"+
"\3\2\2\2\u0394\u0392\3\2\2\2\u0394\u0395\3\2\2\2\u0395\u0397\3\2\2\2\u0396"+
"\u0394\3\2\2\2\u0397\u0398\7\3\2\2\u0398\u0477\3\2\2\2\u0399\u039d\7a"+
"\2\2\u039a\u039c\7\u008e\2\2\u039b\u039a\3\2\2\2\u039c\u039f\3\2\2\2\u039d"+
"\u039b\3\2\2\2\u039d\u039e\3\2\2\2\u039e\u03a0\3\2\2\2\u039f\u039d\3\2"+
"\2\2\u03a0\u03a4\7y\2\2\u03a1\u03a3\7\u008e\2\2\u03a2\u03a1\3\2\2\2\u03a3"+
"\u03a6\3\2\2\2\u03a4\u03a2\3\2\2\2\u03a4\u03a5\3\2\2\2\u03a5\u03a7\3\2"+
"\2\2\u03a6\u03a4\3\2\2\2\u03a7\u03ab\7\u0086\2\2\u03a8\u03aa\7\u008e\2"+
"\2\u03a9\u03a8\3\2\2\2\u03aa\u03ad\3\2\2\2\u03ab\u03a9\3\2\2\2\u03ab\u03ac"+
"\3\2\2\2\u03ac\u03ae\3\2\2\2\u03ad\u03ab\3\2\2\2\u03ae\u0477\7\3\2\2\u03af"+
"\u03b0\7b\2\2\u03b0\u03b1\5\4\3\2\u03b1\u03b2\7\4\2\2\u03b2\u03b3\5\4"+
"\3\2\u03b3\u03b4\7\3\2\2\u03b4\u0477\3\2\2\2\u03b5\u03b6\7c\2\2\u03b6"+
"\u03b7\5\4\3\2\u03b7\u03b8\7\4\2\2\u03b8\u03b9\5\4\3\2\u03b9\u03ba\7\3"+
"\2\2\u03ba\u0477\3\2\2\2\u03bb\u03bc\7d\2\2\u03bc\u03bd\5\4\3\2\u03bd"+
"\u03be\7\3\2\2\u03be\u0477\3\2\2\2\u03bf\u03c3\7e\2\2\u03c0\u03c2\7\u008e"+
"\2\2\u03c1\u03c0\3\2\2\2\u03c2\u03c5\3\2\2\2\u03c3\u03c1\3\2\2\2\u03c3"+
"\u03c4\3\2\2\2\u03c4\u03c7\3\2\2\2\u03c5\u03c3\3\2\2\2\u03c6\u03c8\7\u0082"+
"\2\2\u03c7\u03c6\3\2\2\2\u03c7\u03c8\3\2\2\2\u03c8\u03cc\3\2\2\2\u03c9"+
"\u03cb\7\u008e\2\2\u03ca\u03c9\3\2\2\2\u03cb\u03ce\3\2\2\2\u03cc\u03ca"+
"\3\2\2\2\u03cc\u03cd\3\2\2\2\u03cd\u03cf\3\2\2\2\u03ce\u03cc\3\2\2\2\u03cf"+
"\u0477\7\3\2\2\u03d0\u03d1\7f\2\2\u03d1\u03d2\5\4\3\2\u03d2\u03d3\7\4"+
"\2\2\u03d3\u03d4\5\4\3\2\u03d4\u03d5\7\3\2\2\u03d5\u0477\3\2\2\2\u03d6"+
"\u03d7\7g\2\2\u03d7\u03d8\5\4\3\2\u03d8\u03d9\7\3\2\2\u03d9\u0477\3\2"+
"\2\2\u03da\u03db\7h\2\2\u03db\u03dc\5\4\3\2\u03dc\u03dd\7\4\2\2\u03dd"+
"\u03de\5\4\3\2\u03de\u03df\7\4\2\2\u03df\u03e0\5\4\3\2\u03e0\u03e1\7\3"+
"\2\2\u03e1\u0477\3\2\2\2\u03e2\u03e3\7i\2\2\u03e3\u03e4\5\4\3\2\u03e4"+
"\u03e5\7\4\2\2\u03e5\u03e6\5\4\3\2\u03e6\u03e7\7\4\2\2\u03e7\u03e8\5\4"+
"\3\2\u03e8\u03e9\7\3\2\2\u03e9\u0477\3\2\2\2\u03ea\u03eb\7j\2\2\u03eb"+
"\u03ec\5\4\3\2\u03ec\u03ed\7\4\2\2\u03ed\u03ee\5\4\3\2\u03ee\u03ef\7\3"+
"\2\2\u03ef\u0477\3\2\2\2\u03f0\u03f1\7k\2\2\u03f1\u03f2\5\4\3\2\u03f2"+
"\u03f3\7\4\2\2\u03f3\u03f4\5\4\3\2\u03f4\u03f5\7\3\2\2\u03f5\u0477\3\2"+
"\2\2\u03f6\u03f7\7l\2\2\u03f7\u03f8\5\4\3\2\u03f8\u03f9\7\4\2\2\u03f9"+
"\u03fa\5\4\3\2\u03fa\u03fb\7\3\2\2\u03fb\u0477\3\2\2\2\u03fc\u03fd\7m"+
"\2\2\u03fd\u03fe\5\4\3\2\u03fe\u03ff\7\3\2\2\u03ff\u0477\3\2\2\2\u0400"+
"\u0401\7n\2\2\u0401\u0406\5\4\3\2\u0402\u0403\7\4\2\2\u0403\u0405\5\4"+
"\3\2\u0404\u0402\3\2\2\2\u0405\u0408\3\2\2\2\u0406\u0404\3\2\2\2\u0406"+
"\u0407\3\2\2\2\u0407\u0409\3\2\2\2\u0408\u0406\3\2\2\2\u0409\u040a\7\3"+
"\2\2\u040a\u0477\3\2\2\2\u040b\u040c\7o\2\2\u040c\u040d\5\4\3\2\u040d"+
"\u040e\7\4\2\2\u040e\u040f\5\4\3\2\u040f\u0410\7\4\2\2\u0410\u0411\5\4"+
"\3\2\u0411\u0412\7\3\2\2\u0412\u0477\3\2\2\2\u0413\u0414\7p\2\2\u0414"+
"\u0415\5\4\3\2\u0415\u0416\7\4\2\2\u0416\u0417\5\4\3\2\u0417\u0418\7\4"+
"\2\2\u0418\u0419\5\4\3\2\u0419\u041a\7\3\2\2\u041a\u0477\3\2\2\2\u041b"+
"\u041c\7q\2\2\u041c\u041d\5\4\3\2\u041d\u041e\7\4\2\2\u041e\u041f\5\4"+
"\3\2\u041f\u0420\7\4\2\2\u0420\u0421\5\4\3\2\u0421\u0422\7\3\2\2\u0422"+
"\u0477\3\2\2\2\u0423\u0424\7r\2\2\u0424\u0426\t\3\2\2\u0425\u0427\7\b"+
"\2\2\u0426\u0425\3\2\2\2\u0426\u0427\3\2\2\2\u0427\u0428\3\2\2\2\u0428"+
"\u0477\7\7\2\2\u0429\u042a\7r\2\2\u042a\u042b\t\3\2\2\u042b\u042c\7\6"+
"\2\2\u042c\u042d\t\4\2\2\u042d\u0477\7\7\2\2\u042e\u042f\7r\2\2\u042f"+
"\u0430\t\3\2\2\u0430\u0431\7\6\2\2\u0431\u0432\t\4\2\2\u0432\u0433\7\b"+
"\2\2\u0433\u0477\7\7\2\2\u0434\u0435\7r\2\2\u0435\u0436\t\3\2\2\u0436"+
"\u0437\7\t\2\2\u0437\u0438\7\u0086\2\2\u0438\u0477\7\7\2\2\u0439\u043a"+
"\7r\2\2\u043a\u043b\t\3\2\2\u043b\u043c\7\6\2\2\u043c\u043d\t\4\2\2\u043d"+
"\u043e\7\6\2\2\u043e\u043f\7\u0086\2\2\u043f\u0477\7\7\2\2\u0440\u0441"+
"\7r\2\2\u0441\u0442\5\22\n\2\u0442\u0443\7\7\2\2\u0443\u0477\3\2\2\2\u0444"+
"\u0445\7s\2\2\u0445\u0446\7\u0086\2\2\u0446\u0447\7\6\2\2\u0447\u0448"+
"\7\u0086\2\2\u0448\u0477\7\7\2\2\u0449\u044a\7s\2\2\u044a\u044b\7\u0086"+
"\2\2\u044b\u0477\7\7\2\2\u044c\u044d\7s\2\2\u044d\u044e\5\22\n\2\u044e"+
"\u044f\7\7\2\2\u044f\u0477\3\2\2\2\u0450\u0451\7t\2\2\u0451\u0452\7\u0086"+
"\2\2\u0452\u0477\7\7\2\2\u0453\u0454\7u\2\2\u0454\u0455\7\u0086\2\2\u0455"+
"\u0456\7\6\2\2\u0456\u0457\7\u0086\2\2\u0457\u0477\7\7\2\2\u0458\u0459"+
"\7v\2\2\u0459\u045a\7\u0086\2\2\u045a\u0477\7\7\2\2\u045b\u045c\7w\2\2"+
"\u045c\u045d\7\u0086\2\2\u045d\u0477\7\7\2\2\u045e\u045f\7x\2\2\u045f"+
"\u0460\7\u0086\2\2\u0460\u0477\7\7\2\2\u0461\u0465\7y\2\2\u0462\u0464"+
"\7\u008e\2\2\u0463\u0462\3\2\2\2\u0464\u0467\3\2\2\2\u0465\u0463\3\2\2"+
"\2\u0465\u0466\3\2\2\2\u0466\u0468\3\2\2\2\u0467\u0465\3\2\2\2\u0468\u0477"+
"\7\u0086\2\2\u0469\u046a\7z\2\2\u046a\u046b\7\u0086\2\2\u046b\u046c\7"+
"\6\2\2\u046c\u046d\7~\2\2\u046d\u0477\7\7\2\2\u046e\u0477\7}\2\2\u046f"+
"\u0470\7{\2\2\u0470\u0471\5\6\4\2\u0471\u0472\7\7\2\2\u0472\u0477\3\2"+
"\2\2\u0473\u0477\5\b\5\2\u0474\u0477\5\16\b\2\u0475\u0477\5\20\t\2\u0476"+
"\31\3\2\2\2\u0476 \3\2\2\2\u0476$\3\2\2\2\u0476&\3\2\2\2\u0476\61\3\2"+
"\2\2\u0476<\3\2\2\2\u0476D\3\2\2\2\u0476H\3\2\2\2\u0476L\3\2\2\2\u0476"+
"W\3\2\2\2\u0476_\3\2\2\2\u0476c\3\2\2\2\u0476\u0085\3\2\2\2\u0476\u00a7"+
"\3\2\2\2\u0476\u00ab\3\2\2\2\u0476\u00b8\3\2\2\2\u0476\u00bc\3\2\2\2\u0476"+
"\u00c0\3\2\2\2\u0476\u00c4\3\2\2\2\u0476\u00ca\3\2\2\2\u0476\u00ce\3\2"+
"\2\2\u0476\u00d2\3\2\2\2\u0476\u00d6\3\2\2\2\u0476\u00da\3\2\2\2\u0476"+
"\u00de\3\2\2\2\u0476\u00e4\3\2\2\2\u0476\u00e8\3\2\2\2\u0476\u00f3\3\2"+
"\2\2\u0476\u0107\3\2\2\2\u0476\u011a\3\2\2\2\u0476\u012c\3\2\2\2\u0476"+
"\u013e\3\2\2\2\u0476\u014e\3\2\2\2\u0476\u0170\3\2\2\2\u0476\u0190\3\2"+
"\2\2\u0476\u01b0\3\2\2\2\u0476\u01ce\3\2\2\2\u0476\u01e4\3\2\2\2\u0476"+
"\u01f8\3\2\2\2\u0476\u020c\3\2\2\2\u0476\u021e\3\2\2\2\u0476\u0231\3\2"+
"\2\2\u0476\u0243\3\2\2\2\u0476\u0255\3\2\2\2\u0476\u0265\3\2\2\2\u0476"+
"\u026b\3\2\2\2\u0476\u026f\3\2\2\2\u0476\u0273\3\2\2\2\u0476\u0286\3\2"+
"\2\2\u0476\u0298\3\2\2\2\u0476\u02a8\3\2\2\2\u0476\u02b9\3\2\2\2\u0476"+
"\u02cb\3\2\2\2\u0476\u02dd\3\2\2\2\u0476\u02e1\3\2\2\2\u0476\u02e5\3\2"+
"\2\2\u0476\u02eb\3\2\2\2\u0476\u02ef\3\2\2\2\u0476\u0302\3\2\2\2\u0476"+
"\u0314\3\2\2\2\u0476\u0326\3\2\2\2\u0476\u0336\3\2\2\2\u0476\u034c\3\2"+
"\2\2\u0476\u0352\3\2\2\2\u0476\u0365\3\2\2\2\u0476\u0377\3\2\2\2\u0476"+
"\u0389\3\2\2\2\u0476\u0399\3\2\2\2\u0476\u03af\3\2\2\2\u0476\u03b5\3\2"+
"\2\2\u0476\u03bb\3\2\2\2\u0476\u03bf\3\2\2\2\u0476\u03d0\3\2\2\2\u0476"+
"\u03d6\3\2\2\2\u0476\u03da\3\2\2\2\u0476\u03e2\3\2\2\2\u0476\u03ea\3\2"+
"\2\2\u0476\u03f0\3\2\2\2\u0476\u03f6\3\2\2\2\u0476\u03fc\3\2\2\2\u0476"+
"\u0400\3\2\2\2\u0476\u040b\3\2\2\2\u0476\u0413\3\2\2\2\u0476\u041b\3\2"+
"\2\2\u0476\u0423\3\2\2\2\u0476\u0429\3\2\2\2\u0476\u042e\3\2\2\2\u0476"+
"\u0434\3\2\2\2\u0476\u0439\3\2\2\2\u0476\u0440\3\2\2\2\u0476\u0444\3\2"+
"\2\2\u0476\u0449\3\2\2\2\u0476\u044c\3\2\2\2\u0476\u0450\3\2\2\2\u0476"+
"\u0453\3\2\2\2\u0476\u0458\3\2\2\2\u0476\u045b\3\2\2\2\u0476\u045e\3\2"+
"\2\2\u0476\u0461\3\2\2\2\u0476\u0469\3\2\2\2\u0476\u046e\3\2\2\2\u0476"+
"\u046f\3\2\2\2\u0476\u0473\3\2\2\2\u0476\u0474\3\2\2\2\u0476\u0475\3\2"+
"\2\2\u0477\u04b7\3\2\2\2\u0478\u0479\fp\2\2\u0479\u047a\7\17\2\2\u047a"+
"\u04b6\5\4\3p\u047b\u047c\fn\2\2\u047c\u047d\t\5\2\2\u047d\u04b6\5\4\3"+
"o\u047e\u047f\fm\2\2\u047f\u0480\t\6\2\2\u0480\u04b6\5\4\3n\u0481\u0482"+
"\fl\2\2\u0482\u0483\t\7\2\2\u0483\u04b6\5\4\3m\u0484\u0485\fk\2\2\u0485"+
"\u0486\t\b\2\2\u0486\u04b6\5\4\3l\u0487\u0488\fj\2\2\u0488\u0489\t\t\2"+
"\2\u0489\u04b6\5\4\3k\u048a\u048b\fi\2\2\u048b\u048c\t\n\2\2\u048c\u04b6"+
"\5\4\3j\u048d\u048f\ft\2\2\u048e\u0490\7\u008e\2\2\u048f\u048e\3\2\2\2"+
"\u0490\u0491\3\2\2\2\u0491\u048f\3\2\2\2\u0491\u0492\3\2\2\2\u0492\u04b6"+
"\3\2\2\2\u0493\u0494\fr\2\2\u0494\u0498\7\13\2\2\u0495\u0497\7\u008e\2"+
"\2\u0496\u0495\3\2\2\2\u0497\u049a\3\2\2\2\u0498\u0496\3\2\2\2\u0498\u0499"+
"\3\2\2\2\u0499\u049b\3\2\2\2\u049a\u0498\3\2\2\2\u049b\u049f\5\n\6\2\u049c"+
"\u049e\7\u008e\2\2\u049d\u049c\3\2\2\2\u049e\u04a1\3\2\2\2\u049f\u049d"+
"\3\2\2\2\u049f\u04a0\3\2\2\2\u04a0\u04a2\3\2\2\2\u04a1\u049f\3\2\2\2\u04a2"+
"\u04a3\7\3\2\2\u04a3\u04b6\3\2\2\2\u04a4\u04a5\fq\2\2\u04a5\u04a9\7\f"+
"\2\2\u04a6\u04a8\7\u008e\2\2\u04a7\u04a6\3\2\2\2\u04a8\u04ab\3\2\2\2\u04a9"+
"\u04a7\3\2\2\2\u04a9\u04aa\3\2\2\2\u04aa\u04ac\3\2\2\2\u04ab\u04a9\3\2"+
"\2\2\u04ac\u04b0\5\n\6\2\u04ad\u04af\7\u008e\2\2\u04ae\u04ad\3\2\2\2\u04af"+
"\u04b2\3\2\2\2\u04b0\u04ae\3\2\2\2\u04b0\u04b1\3\2\2\2\u04b1\u04b3\3\2"+
"\2\2\u04b2\u04b0\3\2\2\2\u04b3\u04b4\7\3\2\2\u04b4\u04b6\3\2\2\2\u04b5"+
"\u0478\3\2\2\2\u04b5\u047b\3\2\2\2\u04b5\u047e\3\2\2\2\u04b5\u0481\3\2"+
"\2\2\u04b5\u0484\3\2\2\2\u04b5\u0487\3\2\2\2\u04b5\u048a\3\2\2\2\u04b5"+
"\u048d\3\2\2\2\u04b5\u0493\3\2\2\2\u04b5\u04a4\3\2\2\2\u04b6\u04b9\3\2"+
"\2\2\u04b7\u04b5\3\2\2\2\u04b7\u04b8\3\2\2\2\u04b8\5\3\2\2\2\u04b9\u04b7"+
"\3\2\2\2\u04ba\u04d6\7\64\2\2\u04bb\u04d6\7\65\2\2\u04bc\u04d6\7\66\2"+
"\2\u04bd\u04d6\7\67\2\2\u04be\u04d6\78\2\2\u04bf\u04d6\79\2\2\u04c0\u04d6"+
"\7:\2\2\u04c1\u04d6\7;\2\2\u04c2\u04d6\7<\2\2\u04c3\u04d6\7=\2\2\u04c4"+
"\u04d6\7>\2\2\u04c5\u04d6\7?\2\2\u04c6\u04d6\7@\2\2\u04c7\u04d6\7A\2\2"+
"\u04c8\u04d6\7B\2\2\u04c9\u04d6\7C\2\2\u04ca\u04d6\7D\2\2\u04cb\u04d6"+
"\7E\2\2\u04cc\u04d6\7F\2\2\u04cd\u04d6\7G\2\2\u04ce\u04d6\7H\2\2\u04cf"+
"\u04d6\7I\2\2\u04d0\u04d6\7J\2\2\u04d1\u04d6\7K\2\2\u04d2\u04d6\7L\2\2"+
"\u04d3\u04d6\7M\2\2\u04d4\u04d6\7N\2\2\u04d5\u04ba\3\2\2\2\u04d5\u04bb"+
"\3\2\2\2\u04d5\u04bc\3\2\2\2\u04d5\u04bd\3\2\2\2\u04d5\u04be\3\2\2\2\u04d5"+
"\u04bf\3\2\2\2\u04d5\u04c0\3\2\2\2\u04d5\u04c1\3\2\2\2\u04d5\u04c2\3\2"+
"\2\2\u04d5\u04c3\3\2\2\2\u04d5\u04c4\3\2\2\2\u04d5\u04c5\3\2\2\2\u04d5"+
"\u04c6\3\2\2\2\u04d5\u04c7\3\2\2\2\u04d5\u04c8\3\2\2\2\u04d5\u04c9\3\2"+
"\2\2\u04d5\u04ca\3\2\2\2\u04d5\u04cb\3\2\2\2\u04d5\u04cc\3\2\2\2\u04d5"+
"\u04cd\3\2\2\2\u04d5\u04ce\3\2\2\2\u04d5\u04cf\3\2\2\2\u04d5\u04d0\3\2"+
"\2\2\u04d5\u04d1\3\2\2\2\u04d5\u04d2\3\2\2\2\u04d5\u04d3\3\2\2\2\u04d5"+
"\u04d4\3\2\2\2\u04d6\7\3\2\2\2\u04d7\u04d8\t\13\2\2\u04d8\t\3\2\2\2\u04d9"+
"\u04df\7\177\2\2\u04da\u04db\7\16\2\2\u04db\u04df\7\177\2\2\u04dc\u04dd"+
"\7\r\2\2\u04dd\u04df\7\177\2\2\u04de\u04d9\3\2\2\2\u04de\u04da\3\2\2\2"+
"\u04de\u04dc\3\2\2\2\u04df\13\3\2\2\2\u04e0\u04e1\5\16\b\2\u04e1\r\3\2"+
"\2\2\u04e2\u04e3\t\f\2\2\u04e3\17\3\2\2\2\u04e4\u04e5\7\u0081\2\2\u04e5"+
"\21\3\2\2\2\u04e6\u04e8\5\24\13\2\u04e7\u04e6\3\2\2\2\u04e8\u04e9\3\2"+
"\2\2\u04e9\u04e7\3\2\2\2\u04e9\u04ea\3\2\2\2\u04ea\23\3\2\2\2\u04eb\u04ec"+
"\t\r\2\2\u04ec\25\3\2\2\2q\35,\67R[gnu|\u0081\u0089\u0090\u0097\u009e"+
"\u00a3\u00af\u00b3\u00ee\u00f7\u00fe\u010b\u0116\u011e\u0127\u0130\u0139"+
"\u0142\u0149\u0152\u015d\u0164\u016b\u0174\u017d\u0184\u018b\u0194\u019d"+
"\u01a4\u01ab\u01b4\u01bb\u01c2\u01c9\u01d2\u01dd\u01e8\u01f1\u01fc\u0205"+
"\u0210\u0217\u0222\u022d\u0235\u023e\u0247\u0250\u0259\u0260\u0277\u0282"+
"\u028a\u0293\u029c\u02a3\u02ac\u02b5\u02bd\u02c6\u02cf\u02d8\u02f3\u02fe"+
"\u0306\u030f\u0318\u0321\u032a\u0331\u033a\u0341\u0348\u0356\u0361\u0369"+
"\u0372\u037b\u0384\u038d\u0394\u039d\u03a4\u03ab\u03c3\u03c7\u03cc\u0406"+
"\u0426\u0465\u0476\u0491\u0498\u049f\u04a9\u04b0\u04b5\u04b7\u04d5\u04de"+
"\u04e9";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy