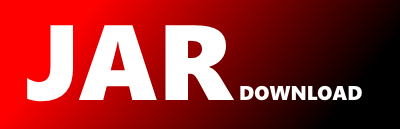
org.hisp.dhis.Dhis2Config Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dhis2-java-client Show documentation
Show all versions of dhis2-java-client Show documentation
DHIS 2 API client for Java.
package org.hisp.dhis;
import java.net.URI;
import java.net.URISyntaxException;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.Validate;
import org.apache.http.client.utils.URIBuilder;
import lombok.Getter;
/**
* Configuration information about a DHIS 2 instance.
*
* @author Lars Helge Overland
*/
@Getter
public class Dhis2Config
{
private final String url;
private final String username;
private final String password;
/**
* Main constructor.
*
* @param url the URL to the DHIS 2 instance, do not include the {@code /api} part or a trailing {@code /}.
* @param username the DHIS 2 account username.
* @param password the DHIS 2 account password.
*/
public Dhis2Config( String url, String username, String password )
{
Validate.notNull( url );
Validate.notNull( username );
Validate.notNull( password );
this.url = normalizeUrl( url );
this.username = username;
this.password = password;
}
/**
* Normalizes the given URL.
*
* @param url the URL string.
* @return a URL string.
*/
private String normalizeUrl( String url )
{
return StringUtils.removeEnd( url, "/" );
}
/**
* Provides a fully qualified {@link URI} to the DHIS 2 instance API.
*
* @param path the URL path (the URL part after {@code /api/}.
* @return a fully qualified {@link URI} to the DHIS 2 instance API.
*/
public URI getResolvedUrl( String path )
{
Validate.notNull( path );
try
{
return new UriBuilder( url )
.pathSegment( "api" )
.pathSegment( path )
.build();
}
catch ( URISyntaxException ex )
{
throw new RuntimeException( String.format( "Invalid URI syntax: '%s'", url ), ex );
}
}
/**
* Provides a {@link URIBuilder} which is resolved to the DHIS 2
* instance API.
*
* @return a {@link URIBuilder}.
*/
public UriBuilder getResolvedUriBuilder()
{
try
{
return new UriBuilder( url )
.pathSegment( "api" );
}
catch ( URISyntaxException ex )
{
throw new RuntimeException( String.format( "Invalid URI syntax: '%s'", url ), ex );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy