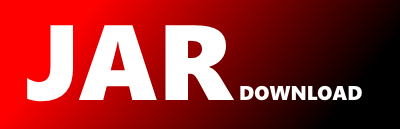
org.hisp.dhis.util.CollectionUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dhis2-java-client Show documentation
Show all versions of dhis2-java-client Show documentation
DHIS 2 API client for Java.
package org.hisp.dhis.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import lombok.AccessLevel;
import lombok.NoArgsConstructor;
@NoArgsConstructor( access = AccessLevel.PRIVATE )
public class CollectionUtils
{
/**
* Returns an immutable set containing the given items. Accepts null items.
*
* @param type.
* @param items the items.
* @return an immutable set.
*/
@SafeVarargs
public static Set set( T... items )
{
return Collections.unmodifiableSet( mutableSet( items ) );
}
/**
* Returns a mutable set containing the given items. Accepts null items.
*
* @param type.
* @param items the items.
* @return an immutable set.
*/
@SafeVarargs
public static Set mutableSet( T... items )
{
Set set = new HashSet<>();
for ( T item : items )
{
set.add( item );
}
return set;
}
/**
* Returns an immutable list containing the given items. Accepts null items.
*
* @param type.
* @param items the items.
* @return an immutable list.
*/
@SafeVarargs
public static List list( T... items )
{
return Collections.unmodifiableList( mutableList( items ) );
}
/**
* Returns a mutable list containing the given items. Accepts null items.
*
* @param type.
* @param items the items.
* @return an immutable list.
*/
@SafeVarargs
public static List mutableList( T... items )
{
List list = new ArrayList<>();
for ( T item : items )
{
list.add( item );
}
return list;
}
/**
* Converts the given array to an {@link ArrayList}.
*
* @param array the array.
* @param class.
* @return a list.
*/
public static List asList( T[] array )
{
return new ArrayList<>( Arrays.asList( array ) );
}
/**
* Returns the first matching item in the given collection based on the
* given predicate. Returns null if no match is found.
*
* @param type.
* @param collection the collection.
* @param predicate the predicate.
* @return the first matching item, or null if no match is found.
*/
public static T firstMatch( Collection collection, Predicate predicate )
{
return collection.stream()
.filter( predicate )
.findFirst()
.orElse( null );
}
/**
* Returns a new mutable list of the items in the given collection which
* match the given predicate.
*
* @param type.
* @param collection the collection.
* @param predicate the predicate.
* @return a new mutable list.
*/
public static List list( Collection collection, Predicate predicate )
{
return collection.stream()
.filter( predicate )
.collect( Collectors.toList() );
}
/**
* Indicates if the given collection is not null and not empty.
*
* @param
* @param collection the collection.
* @return true if the given collection is not null and not empty.
*/
public static boolean notEmpty( Collection collection )
{
return collection != null && !collection.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy