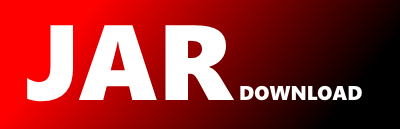
org.hisrc.jscm.codemodel.statement.impl.TryStatementImpl Maven / Gradle / Ivy
The newest version!
package org.hisrc.jscm.codemodel.statement.impl;
import org.hisrc.jscm.codemodel.JSCodeModel;
import org.hisrc.jscm.codemodel.expression.JSVariable;
import org.hisrc.jscm.codemodel.expression.impl.VariableImpl;
import org.hisrc.jscm.codemodel.lang.Validate;
import org.hisrc.jscm.codemodel.statement.JSBlock;
import org.hisrc.jscm.codemodel.statement.JSStatementVisitor;
import org.hisrc.jscm.codemodel.statement.JSTryStatement;
public class TryStatementImpl extends StatementImpl implements JSTryStatement {
private final JSBlock _try;
private final JSVariable exception;
private final JSBlock _catch;
private final JSBlock _finally;
public TryStatementImpl(JSCodeModel codeModel, String exception,
boolean _finally) {
super(codeModel);
if (!_finally) {
Validate.notNull(exception);
}
this._try = new BlockImpl(codeModel);
if (exception == null) {
this.exception = null;
this._catch = null;
} else {
this.exception = new VariableImpl(codeModel, exception);
this._catch = new BlockImpl(codeModel);
}
if (_finally) {
this._finally = new BlockImpl(codeModel);
} else {
this._finally = null;
}
}
@Override
public JSBlock getBody() {
return _try;
}
@Override
public JSBlock getCatch() {
return _catch;
}
@Override
public JSBlock getFinally() {
return _finally;
}
@Override
public JSVariable getException() {
return exception;
}
@Override
public V acceptStatementVisitor(
JSStatementVisitor visitor) throws E {
return visitor.visitTry(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy