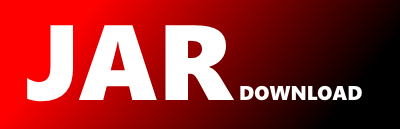
org.hisrc.jsonix.configuration.MappingConfiguration Maven / Gradle / Ivy
package org.hisrc.jsonix.configuration;
import java.text.MessageFormat;
import java.util.Map;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.namespace.QName;
import org.apache.commons.lang3.Validate;
import org.hisrc.jsonix.analysis.ModelInfoGraphAnalyzer;
import org.hisrc.jsonix.context.JsonixContext;
import org.hisrc.jsonix.definition.Mapping;
import org.jvnet.jaxb2_commons.xml.bind.model.MElementInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.MModelInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.MPackageInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.MPropertyInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.MTypeInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.util.PackageInfoQNameAnalyzer;
import org.slf4j.Logger;
@XmlRootElement(name = MappingConfiguration.LOCAL_ELEMENT_NAME)
@XmlType(propOrder = {})
public class MappingConfiguration {
public static final String LOCAL_ELEMENT_NAME = "mapping";
private String id;
private String name;
private String _package;
private String defaultElementNamespaceURI;
private String defaultAttributeNamespaceURI;
private IncludesConfiguration includesConfiguration;
private ExcludesConfiguration excludesConfiguration;
public static final QName MAPPING_NAME = new QName(
ModulesConfiguration.NAMESPACE_URI,
MappingConfiguration.LOCAL_ELEMENT_NAME,
ModulesConfiguration.DEFAULT_PREFIX);
@XmlAttribute(name = "id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
@XmlAttribute(name = "name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlAttribute(name = "package")
public String getPackage() {
return _package;
}
public void setPackage(String _package) {
this._package = _package;
}
@XmlAttribute(name = "defaultElementNamespaceURI")
public String getDefaultElementNamespaceURI() {
return defaultElementNamespaceURI;
}
public void setDefaultElementNamespaceURI(String defaultElementNamespaceURI) {
this.defaultElementNamespaceURI = defaultElementNamespaceURI;
}
@XmlAttribute(name = "defaultAttributeNamespaceURI")
public String getDefaultAttributeNamespaceURI() {
return defaultAttributeNamespaceURI;
}
public void setDefaultAttributeNamespaceURI(
String defaultAttributeNamespaceURI) {
this.defaultAttributeNamespaceURI = defaultAttributeNamespaceURI;
}
@XmlElement(name = IncludesConfiguration.LOCAL_ELEMENT_NAME)
public IncludesConfiguration getIncludesConfiguration() {
return includesConfiguration;
}
public void setIncludesConfiguration(
IncludesConfiguration includesConfiguration) {
this.includesConfiguration = includesConfiguration;
}
@XmlElement(name = ExcludesConfiguration.LOCAL_ELEMENT_NAME)
public ExcludesConfiguration getExcludesConfiguration() {
return excludesConfiguration;
}
public void setExcludesConfiguration(
ExcludesConfiguration excludesConfiguration) {
this.excludesConfiguration = excludesConfiguration;
}
public Mapping build(JsonixContext context,
ModelInfoGraphAnalyzer analyzer, MModelInfo modelInfo,
MPackageInfo packageInfo, Map> mappings) {
Validate.notNull(modelInfo);
Validate.notNull(packageInfo);
Validate.notNull(mappings);
final String packageName = getPackage();
final String mappingName = getName();
final Logger logger = context.getLoggerFactory().getLogger(
MappingConfiguration.class.getName());
logger.debug(MessageFormat.format(
"Package [{0}] will be mapped by the mapping [{1}].",
packageName, mappingName));
final PackageInfoQNameAnalyzer qnameAnalyzer = new PackageInfoQNameAnalyzer(
modelInfo);
final String draftMostUsedElementNamespaceURI = qnameAnalyzer
.getMostUsedElementNamespaceURI(packageInfo);
final String mostUsedElementNamespaceURI = draftMostUsedElementNamespaceURI == null ? ""
: draftMostUsedElementNamespaceURI;
final String defaultElementNamespaceURI;
if (this.defaultElementNamespaceURI != null) {
defaultElementNamespaceURI = this.defaultElementNamespaceURI;
} else {
logger.debug(MessageFormat
.format("Mapping [{0}] will use \"{1}\" as it is the most used element namespace URI in the package [{2}].",
mappingName, mostUsedElementNamespaceURI,
packageName));
defaultElementNamespaceURI = mostUsedElementNamespaceURI;
}
final String draftMostUsedAttributeNamespaceURI = qnameAnalyzer
.getMostUsedAttributeNamespaceURI(packageInfo);
final String mostUsedAttributeNamespaceURI = draftMostUsedAttributeNamespaceURI == null ? ""
: draftMostUsedAttributeNamespaceURI;
final String defaultAttributeNamespaceURI;
if (this.defaultAttributeNamespaceURI != null) {
defaultAttributeNamespaceURI = this.defaultAttributeNamespaceURI;
} else {
logger.debug(MessageFormat
.format("Mapping [{0}] will use \"{1}\" as it is the most used attribute namespace URI in the package [{2}].",
mappingName, mostUsedAttributeNamespaceURI,
packageName));
defaultAttributeNamespaceURI = mostUsedAttributeNamespaceURI;
}
final Mapping mapping = new Mapping(context, analyzer,
packageInfo, mappingName, defaultElementNamespaceURI,
defaultAttributeNamespaceURI);
if (getExcludesConfiguration() != null) {
final ExcludesConfiguration excludesConfiguration = getExcludesConfiguration();
for (TypeInfoConfiguration typeInfoConfiguration : excludesConfiguration
.getTypeInfoConfigurations()) {
final MTypeInfo typeInfo = typeInfoConfiguration
.findTypeInfo(context, analyzer, packageInfo);
if (typeInfo != null) {
mapping.excludeTypeInfo(typeInfo);
}
}
for (ElementInfoConfiguration elementInfoConfiguration : excludesConfiguration
.getElementInfoConfigurations()) {
final MElementInfo elementInfo = elementInfoConfiguration
.findElementInfo(context, analyzer, packageInfo);
if (elementInfo != null) {
mapping.excludeElementInfo(elementInfo);
}
}
for (PropertyInfoConfiguration propertyInfoConfiguration : excludesConfiguration
.getPropertyInfoConfigurations()) {
final MPropertyInfo propertyInfo = propertyInfoConfiguration
.findPropertyInfo(context, analyzer, packageInfo);
if (propertyInfo != null) {
mapping.excludePropertyInfo(propertyInfo);
}
}
}
if (getIncludesConfiguration() == null) {
logger.trace(MessageFormat
.format("Includes configuration for the mapping [{0}] is not provided, including the whole package.",
mappingName));
mapping.includePackage(packageInfo);
} else {
final IncludesConfiguration includesConfiguration = getIncludesConfiguration();
for (TypeInfoConfiguration typeInfoConfiguration : includesConfiguration
.getTypeInfoConfigurations()) {
final MTypeInfo typeInfo = typeInfoConfiguration
.findTypeInfo(context, analyzer, packageInfo);
if (typeInfo != null) {
mapping.includeTypeInfo(typeInfo);
}
}
for (ElementInfoConfiguration elementInfoConfiguration : includesConfiguration
.getElementInfoConfigurations()) {
final MElementInfo elementInfo = elementInfoConfiguration
.findElementInfo(context, analyzer, packageInfo);
if (elementInfo != null) {
mapping.includeElementInfo(elementInfo);
}
}
for (PropertyInfoConfiguration propertyInfoConfiguration : includesConfiguration
.getPropertyInfoConfigurations()) {
final MPropertyInfo propertyInfo = propertyInfoConfiguration
.findPropertyInfo(context, analyzer, packageInfo);
if (propertyInfo != null) {
mapping.includePropertyInfo(propertyInfo);
}
}
for (DependenciesOfMappingConfiguration dependenciesOfMappingConfiguration : includesConfiguration
.getDependenciesOfMappingConfiguration()) {
final String id = dependenciesOfMappingConfiguration.getId();
final Mapping dependingMapping = mappings.get(id);
if (dependingMapping == null) {
throw new MissingMappingWithIdException(id);
}
mapping.includeDependenciesOfMapping(dependingMapping);
}
}
return mapping;
}
@Override
public String toString() {
return MessageFormat.format("[{0}:{1}]", getId(), getName());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy