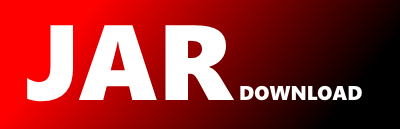
org.hisrc.jsonix.analysis.PropertyInfoVertex Maven / Gradle / Ivy
package org.hisrc.jsonix.analysis;
import java.text.MessageFormat;
import org.apache.commons.lang3.Validate;
import org.jvnet.jaxb2_commons.xml.bind.model.MClassInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.MPackageInfo;
import org.jvnet.jaxb2_commons.xml.bind.model.MPropertyInfo;
public class PropertyInfoVertex extends InfoVertex {
private final MPackageInfo packageInfo;
private final MClassInfo classInfo;
private final MPropertyInfo propertyInfo;
public PropertyInfoVertex(MPropertyInfo propertyInfo) {
Validate.notNull(propertyInfo);
this.propertyInfo = propertyInfo;
this.packageInfo = propertyInfo.getClassInfo().getPackageInfo();
this.classInfo = propertyInfo.getClassInfo();
}
@Override
public MPackageInfo getPackageInfo() {
return packageInfo;
}
public MClassInfo getClassInfo() {
return classInfo;
}
public MPropertyInfo getPropertyInfo() {
return propertyInfo;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result
+ ((propertyInfo == null) ? 0 : propertyInfo.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
@SuppressWarnings("rawtypes")
PropertyInfoVertex other = (PropertyInfoVertex) obj;
if (propertyInfo == null) {
if (other.propertyInfo != null)
return false;
} else if (!propertyInfo.equals(other.propertyInfo))
return false;
return true;
}
@Override
public String toString() {
final String propertyName = propertyInfo.getClassInfo().getName()
+ "." + propertyInfo.getPrivateName();
return MessageFormat.format("Property [{0}]", propertyName);
}
@Override
public V accept(InfoVertexVisitor visitor) {
return visitor.visitPropertyInfoVertex(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy