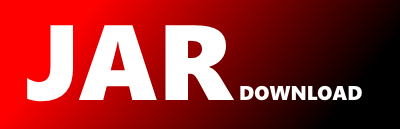
org.hisrc.jsonix.tests.zero.ObjectFactory Maven / Gradle / Ivy
//
// Diese Datei wurde mit der JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6 generiert
// Siehe http://java.sun.com/xml/jaxb
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
// Generiert: 2014.02.24 um 02:10:12 AM CET
//
package org.hisrc.jsonix.tests.zero;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.hisrc.jsonix.tests.zero package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AnyElementStrict_QNAME = new QName("", "anyElementStrict");
private final static QName _Element_QNAME = new QName("", "element");
private final static QName _AnyElementSkip_QNAME = new QName("", "anyElementSkip");
private final static QName _Extended_QNAME = new QName("", "extended");
private final static QName _Attribute_QNAME = new QName("", "attribute");
private final static QName _Enum_QNAME = new QName("", "enum");
private final static QName _AnyAttribute_QNAME = new QName("", "anyAttribute");
private final static QName _AbstractElement_QNAME = new QName("", "abstractElement");
private final static QName _ElementRef_QNAME = new QName("", "elementRef");
private final static QName _AnyElementLax_QNAME = new QName("", "anyElementLax");
private final static QName _ElementRefMixed_QNAME = new QName("", "elementRefMixed");
private final static QName _ElementRefs_QNAME = new QName("", "elementRefs");
private final static QName _String_QNAME = new QName("", "string");
private final static QName _Value_QNAME = new QName("", "value");
private final static QName _SimpleTypes_QNAME = new QName("", "simpleTypes");
private final static QName _Base_QNAME = new QName("", "base");
private final static QName _Elements_QNAME = new QName("", "elements");
private final static QName _ElementTypeItems_QNAME = new QName("", "items");
private final static QName _ElementRefsTypeAlpha_QNAME = new QName("", "alpha");
private final static QName _ElementRefsTypeBeta_QNAME = new QName("", "beta");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.hisrc.jsonix.tests.zero
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AnyElementStrictType }
*
*/
public AnyElementStrictType createAnyElementStrictType() {
return new AnyElementStrictType();
}
/**
* Create an instance of {@link ElementType }
*
*/
public ElementType createElementType() {
return new ElementType();
}
/**
* Create an instance of {@link AnyElementSkipType }
*
*/
public AnyElementSkipType createAnyElementSkipType() {
return new AnyElementSkipType();
}
/**
* Create an instance of {@link ExtendedType }
*
*/
public ExtendedType createExtendedType() {
return new ExtendedType();
}
/**
* Create an instance of {@link BaseType }
*
*/
public BaseType createBaseType() {
return new BaseType();
}
/**
* Create an instance of {@link AttributeType }
*
*/
public AttributeType createAttributeType() {
return new AttributeType();
}
/**
* Create an instance of {@link AnyAttributeType }
*
*/
public AnyAttributeType createAnyAttributeType() {
return new AnyAttributeType();
}
/**
* Create an instance of {@link ElementRefType }
*
*/
public ElementRefType createElementRefType() {
return new ElementRefType();
}
/**
* Create an instance of {@link AnyElementLaxType }
*
*/
public AnyElementLaxType createAnyElementLaxType() {
return new AnyElementLaxType();
}
/**
* Create an instance of {@link ElementRefMixedType }
*
*/
public ElementRefMixedType createElementRefMixedType() {
return new ElementRefMixedType();
}
/**
* Create an instance of {@link ElementRefsType }
*
*/
public ElementRefsType createElementRefsType() {
return new ElementRefsType();
}
/**
* Create an instance of {@link SimpleTypesType }
*
*/
public SimpleTypesType createSimpleTypesType() {
return new SimpleTypesType();
}
/**
* Create an instance of {@link ValueType }
*
*/
public ValueType createValueType() {
return new ValueType();
}
/**
* Create an instance of {@link ElementsType }
*
*/
public ElementsType createElementsType() {
return new ElementsType();
}
/**
* Create an instance of {@link ExtendedMixedType }
*
*/
public ExtendedMixedType createExtendedMixedType() {
return new ExtendedMixedType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AnyElementStrictType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "anyElementStrict")
public JAXBElement createAnyElementStrict(AnyElementStrictType value) {
return new JAXBElement(_AnyElementStrict_QNAME, AnyElementStrictType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ElementType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "element")
public JAXBElement createElement(ElementType value) {
return new JAXBElement(_Element_QNAME, ElementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AnyElementSkipType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "anyElementSkip")
public JAXBElement createAnyElementSkip(AnyElementSkipType value) {
return new JAXBElement(_AnyElementSkip_QNAME, AnyElementSkipType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtendedType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "extended", substitutionHeadNamespace = "", substitutionHeadName = "base")
public JAXBElement createExtended(ExtendedType value) {
return new JAXBElement(_Extended_QNAME, ExtendedType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AttributeType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "attribute")
public JAXBElement createAttribute(AttributeType value) {
return new JAXBElement(_Attribute_QNAME, AttributeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EnumType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "enum")
public JAXBElement createEnum(EnumType value) {
return new JAXBElement(_Enum_QNAME, EnumType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AnyAttributeType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "anyAttribute")
public JAXBElement createAnyAttribute(AnyAttributeType value) {
return new JAXBElement(_AnyAttribute_QNAME, AnyAttributeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "abstractElement")
public JAXBElement