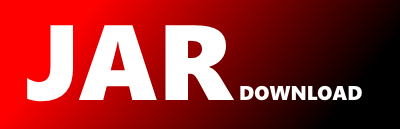
org.hobsoft.symmetry.ui.traversal.VisibleComponentVisitor Maven / Gradle / Ivy
Show all versions of symmetry-ui Show documentation
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.hobsoft.symmetry.ui.traversal;
import org.hobsoft.symmetry.ui.Component;
import org.hobsoft.symmetry.ui.Deck;
import org.hobsoft.symmetry.ui.Table;
import org.hobsoft.symmetry.ui.Tree;
import com.google.common.reflect.TypeToken;
import static org.hobsoft.symmetry.ui.traversal.ComponentVisitors.asContainerVisitor;
import static org.hobsoft.symmetry.ui.traversal.ComponentVisitors.asTableVisitor;
import static org.hobsoft.symmetry.ui.traversal.ComponentVisitors.asTreeVisitor;
import static org.hobsoft.symmetry.ui.traversal.ComponentVisitors.visibleNodes;
import static org.hobsoft.symmetry.ui.traversal.ComponentVisitors.visibleRows;
/**
*
*
* @author Mark Hobson
* @param
* the parameter type this visitor takes
* @param
* the exception type this visitor can throw
*/
class VisibleComponentVisitor extends DelegatingComponentVisitor
{
// TODO: should we consider component visibility?
// TODO: should this visitor handle all these cases or be broken into smaller parts?
// constructors -----------------------------------------------------------
public VisibleComponentVisitor(ComponentVisitor
delegate)
{
super(delegate);
}
// ComponentVisitor methods -----------------------------------------------
/**
* {@inheritDoc}
*/
@Override
public HierarchicalComponentVisitor visit(TypeToken componentType, T component,
P parameter) throws E
{
HierarchicalComponentVisitor subvisitor = super.visit(componentType, component, parameter);
// TODO: this isn't pretty
if (TypeToken.of(Deck.class).equals(componentType))
{
HierarchicalComponentVisitor deckVisitor =
(HierarchicalComponentVisitor) subvisitor;
deckVisitor = ComponentVisitors.selectedComponent(asContainerVisitor(deckVisitor));
subvisitor = (HierarchicalComponentVisitor) deckVisitor;
}
else if (TypeToken.of(Table.class).equals(componentType))
{
HierarchicalComponentVisitor tableVisitor =
(HierarchicalComponentVisitor) subvisitor;
tableVisitor = visibleRows(asTableVisitor(tableVisitor));
subvisitor = (HierarchicalComponentVisitor) tableVisitor;
}
else if (TypeToken.of(Tree.class).equals(componentType))
{
HierarchicalComponentVisitor treeVisitor =
(HierarchicalComponentVisitor) subvisitor;
treeVisitor = visibleNodes(asTreeVisitor(treeVisitor));
subvisitor = (HierarchicalComponentVisitor) treeVisitor;
}
return subvisitor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy