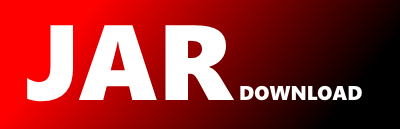
org.holmes.HolmesEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of holmes-validation Show documentation
Show all versions of holmes-validation Show documentation
Holmes is a library that provides a simple and fluent API for writing business rules validations on Java projects.
The newest version!
package org.holmes;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import org.holmes.evaluator.BooleanEvaluator;
import org.holmes.evaluator.CollectionEvaluator;
import org.holmes.evaluator.DateEvaluator;
import org.holmes.evaluator.NumberEvaluator;
import org.holmes.evaluator.ObjectEvaluator;
import org.holmes.evaluator.StringEvaluator;
import org.holmes.exception.RuleViolationException;
import org.holmes.exception.ValidationException;
/**
* The main class of the framework.
*
* @author diegossilveira
*/
public class HolmesEngine {
private final List rules;
private final ResultCollector collector;
private String defaultViolationDescriptor;
private HolmesEngine(ResultCollector collector) {
rules = new ArrayList();
this.collector = collector;
}
/**
* Initializes the engine with GREEDY {@link Op2erationMode}.
*
* @return initialized engine instance.
*/
public static HolmesEngine init() {
return new HolmesEngine(OperationMode.GREEDY.getResultCollector());
}
/**
* Initializes the engine with the given {@link OperationMode}.
*
* @param mode
* the {@link OperationMode}
* @return initialized engine instance.
*/
public static HolmesEngine init(OperationMode mode) {
return new HolmesEngine(mode.getResultCollector());
}
/**
* Initializes the engine with the given {@link ResultCollector}.
*
* @param collector
* @return initialized engine instance.
*/
public static HolmesEngine init(ResultCollector collector) {
return new HolmesEngine(collector);
}
/**
* Creates a new {@link Rule} for a {@link Boolean} target type.
*
* @param bool
* the target
* @return an appropriated {@link Evaluator} for the given target type.
*/
public BooleanEvaluator ensureThat(final Boolean bool) {
return configure(new BooleanEvaluator(bool));
}
/**
* Creates a new {@link Rule} for a {@link String} target type.
*
* @param string
* the target
* @return an appropriated {@link Evaluator} for the given target type.
*/
public StringEvaluator ensureThat(final String string) {
return configure(new StringEvaluator(string));
}
/**
* Creates a new {@link Rule} for a {@link Collection} target type.
*
* @param collection
* the target
* @return an appropriated {@link Evaluator} for the given target type.
*/
public CollectionEvaluator ensureThat(final Collection collection) {
return configure(new CollectionEvaluator(collection));
}
/**
* Creates a new {@link Rule} for a {@link Number} target type.
*
* @param number
* the target
* @return an appropriated {@link Evaluator} for the given target type.
*/
public NumberEvaluator ensureThat(final Number number) {
return configure(new NumberEvaluator(number));
}
/**
* Creates a new {@link Rule} for a {@link Date} target type.
*
* @param date
* the target
* @return an appropriated {@link Evaluator} for the given target type.
*/
public DateEvaluator ensureThat(final Date date) {
return configure(new DateEvaluator(date));
}
/**
* Creates a new {@link Rule} for a generic {@link Object} target type.
*
* @param object
* the target
* @return an appropriated {@link Evaluator} for the given target type.
*/
public ObjectEvaluator ensureThat(final T object) {
return configure(new ObjectEvaluator(object));
}
/**
* Runs all evaluation rules on the context.
*
* @return the result of the validation process.
* @throws ValidationException
*/
public ValidationResult run() throws ValidationException {
ValidationResult result = ValidationResult.init();
for (Rule rule : rules) {
evaluateRule(rule, result);
}
collector.finish(result);
return result;
}
/**
* Specifies a default violation descriptor used to represent violations to
* rules which do not specify its own descriptor.
*
* @param defaultViolationDescriptor
* the violation descriptor.
*/
public HolmesEngine withDefaultDescriptor(String defaultViolationDescriptor) {
this.defaultViolationDescriptor = defaultViolationDescriptor;
return this;
}
private > E configure(E evaluator) {
Rule rule = Rule.simpleFor(evaluator);
evaluator.setJoint(new Joint(rule));
rules.add(rule);
return evaluator;
}
private void evaluateRule(Rule rule, ValidationResult result) {
try {
if(!rule.hasViolationDescriptor()) {
rule.setViolationDescriptor(defaultViolationDescriptor);
}
rule.evaluate();
} catch (RuleViolationException e) {
collector.onRuleViolation(e, result);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy