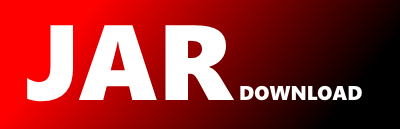
org.holmes.evaluator.CollectionEvaluator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of holmes-validation Show documentation
Show all versions of holmes-validation Show documentation
Holmes is a library that provides a simple and fluent API for writing business rules validations on Java projects.
The newest version!
package org.holmes.evaluator;
import java.util.Collection;
import org.holmes.Evaluator;
import org.holmes.Joint;
import org.holmes.Validator;
import org.holmes.evaluator.support.FutureNumber;
/**
* An {@link Evaluator} for the {@link Collection} type.
*
* @author diegossilveira
*/
public class CollectionEvaluator extends ObjectEvaluator> {
public CollectionEvaluator(Collection target) {
super(target);
}
/**
* Evaluates the number of occurrences of
* element in the target collection
*
* @param element
* an element.
* @return an instance of {@link NumberEvaluator}
*/
public NumberEvaluator cardinalityOf(final E element) {
final FutureNumber futureNumber = new FutureNumber();
final NumberEvaluator evaluator = new NumberEvaluator(futureNumber);
setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
if (target == null) {
return false;
}
futureNumber.wrap(getCardinality(element, target));
return evaluator.evaluate();
}
});
evaluator.setJoint(getJoint());
return evaluator;
}
/**
* Evaluates the size of the target collection. A null collection has no
* size!
*
* @return an instance of {@link NumberEvaluator}
*/
public NumberEvaluator size() {
final FutureNumber futureNumber = new FutureNumber();
final NumberEvaluator evaluator = new NumberEvaluator(futureNumber);
setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
if (target == null) {
return false;
}
futureNumber.wrap(target.size());
return evaluator.evaluate();
}
});
evaluator.setJoint(getJoint());
return evaluator;
}
/**
* Ensures that the target does not contain elements.
*
* @return an instance of {@link Joint} class
*/
public Joint isEmpty() {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
return target == null || target.isEmpty();
}
}).getJoint();
}
/**
* Ensures that the target contains any element.
*
* @return an instance of {@link Joint} class
*/
public Joint isNotEmpty() {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
return target != null && !target.isEmpty();
}
}).getJoint();
}
/**
* Ensures that the target collection contains an element
.
*
* @return an instance of {@link Joint} class
*/
public Joint contains(final E element) {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
return target != null && target.contains(element);
}
}).getJoint();
}
/**
* Ensures that the target collection does not contain an
* element
.
*
* @return an instance of {@link Joint} class
*/
public Joint doesNotContain(final E element) {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
return target != null && !target.contains(element);
}
}).getJoint();
}
/**
* Ensures that the target collection contains all elements of
* collection
.
*
* @return an instance of {@link Joint} class
*/
public Joint containsAll(final Collection collection) {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
if (collection == null || target == null) {
return false;
}
for (E e : collection) {
if (!target.contains(e)) {
return false;
}
}
return true;
}
}).getJoint();
}
/**
* Ensures that the target collection contains any elements of
* collection
.
*
* @return an instance of {@link Joint} class
*/
public Joint containsAny(final Collection collection) {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
if (collection == null || target == null) {
return false;
}
for (E e : collection) {
if (target.contains(e)) {
return true;
}
}
return false;
}
}).getJoint();
}
/**
* Ensures that all the elements of the target collection are valid by the
* validator
.
*
* @return an instance of {@link Joint} class
*/
public Joint hasAllValidBy(final Validator validator) {
return setEvaluation(new Evaluation>() {
public boolean evaluate(Collection target) {
if (target == null || validator == null) {
return false;
}
for (E e : target) {
if (!validator.isValid(e)) {
return false;
}
}
return true;
}
}).getJoint();
}
private int getCardinality(E element, Collection collection) {
int cardinality = 0;
for (E e : collection) {
if ((element == null && e == null)
|| (element != null && element.equals(e))) {
cardinality++;
}
}
return cardinality;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy