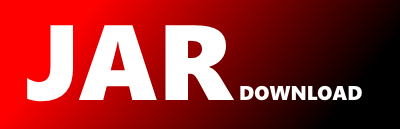
org.holmes.evaluator.support.Interval Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of holmes-validation Show documentation
Show all versions of holmes-validation Show documentation
Holmes is a library that provides a simple and fluent API for writing business rules validations on Java projects.
The newest version!
package org.holmes.evaluator.support;
/**
* Represents a interval, between left boundary and right boundary.
*
* @author diegossilveira
*/
public final class Interval> {
private final T leftBoundary;
private final T rightBoundary;
private final boolean leftOpen;
private final boolean rightOpen;
private Interval(T leftBoundary, T rightBoundary, boolean leftOpen, boolean rightOpen) {
this.leftBoundary = leftBoundary;
this.rightBoundary = rightBoundary;
this.leftOpen = leftOpen;
this.rightOpen = rightOpen;
}
/**
* Creates a closed interval.
*
* @param leftBoundary
* @param rightBoundary
* @return the configured {@link Interval} instance.
*/
public static > Interval closedInterval(T leftBoundary, T rightBoundary) {
return new Interval(leftBoundary, rightBoundary, false, false);
}
/**
* Creates a left-open interval.
*
* @param leftBoundary
* @param rightBoundary
* @return the configured {@link Interval} instance.
*/
public static > Interval leftOpenInterval(T leftBoundary, T rightBoundary) {
return new Interval(leftBoundary, rightBoundary, true, false);
}
/**
* Creates a right-open interval.
*
* @param leftBoundary
* @param rightBoundary
* @return the configured {@link Interval} instance.
*/
public static > Interval rightOpenInterval(T leftBoundary, T rightBoundary) {
return new Interval(leftBoundary, rightBoundary, false, true);
}
/**
* Creates an open interval.
*
* @param leftBoundary
* @param rightBoundary
* @return the configured {@link Interval} instance.
*/
public static > Interval openInterval(T leftBoundary, T rightBoundary) {
return new Interval(leftBoundary, rightBoundary, true, true);
}
/**
* Checks if this interval contains the element.
*
* @param element
* @return true
if the interval contains the element,
* otherwise
.
*/
public boolean contains(T element) {
selfCheck();
return analyzeLeftBoundary(element) && analyzeRightBoundary(element);
}
private void selfCheck() {
if (leftBoundary == null && rightBoundary == null) {
throw new IllegalArgumentException("both leftBoundary and rightBoundary must been set.");
}
}
private boolean analyzeLeftBoundary(T element) {
if (leftOpen) {
return element.compareTo(leftBoundary) > 0;
}
return element.compareTo(leftBoundary) >= 0;
}
private boolean analyzeRightBoundary(T element) {
if (rightOpen) {
return element.compareTo(rightBoundary) < 0;
}
return element.compareTo(rightBoundary) <= 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy