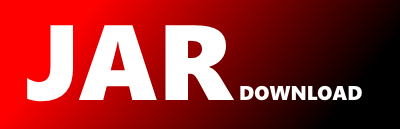
org.honton.chas.compose.maven.plugin.ExecHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compose-maven-plugin
Show all versions of compose-maven-plugin
Use Compose to run docker/podman images from Maven. Store compose configuration in Maven
repository for use by downstream image consumers.
package org.honton.chas.compose.maven.plugin;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.UncheckedIOException;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorCompletionService;
import java.util.concurrent.Future;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import java.util.function.Consumer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.maven.plugin.logging.Log;
public class ExecHelper {
private static final Pattern WARNING =
Pattern.compile("\\[?(warning)]?:? ?(.+)", Pattern.CASE_INSENSITIVE);
private static final Pattern ERROR =
Pattern.compile("\\[?(error)]?:? ?(.+)", Pattern.CASE_INSENSITIVE);
private final ExecutorCompletionService
© 2015 - 2025 Weber Informatics LLC | Privacy Policy