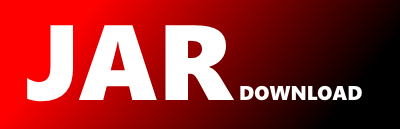
oms3.dsl.Tests Maven / Gradle / Ivy
//package oms3.dsl;
//
//import groovy.lang.Closure;
//import java.lang.reflect.Method;
//import java.util.ArrayList;
//import java.util.Collection;
//import java.util.HashMap;
//import java.util.List;
//import java.util.Map;
//import java.util.concurrent.Callable;
//import java.util.concurrent.ExecutorService;
//import java.util.concurrent.Executors;
//import java.util.concurrent.Future;
//import java.util.concurrent.TimeUnit;
//import java.util.concurrent.TimeoutException;
//import java.util.logging.Level;
//import java.util.logging.Logger;
//import oms3.Access;
//import oms3.ComponentAccess;
//import oms3.ComponentException;
//import oms3.annotations.*;
//
//public class Tests extends AbstractSimulation {
//
// static final Logger log = Logger.getLogger("oms3.sim");
// List testCases = new ArrayList();
//
// class TestCase implements Buildable {
//
// String name;
// int count = 1;
// Closure pre; // Closure
// Closure post; // Closure
// String ignore = null;
// Throwable expected;
// long timeout = 0;
// boolean rangecheck = false;
// List data;
//
// public void setData(List data) {
// this.data = data;
// }
//
// public void setTimeout(long timeout) {
// if (timeout < 1) {
// throw new ComponentException("Illegal timeout value: " + timeout);
// }
// this.timeout = timeout;
// }
//
// public void setRangecheck(boolean rangecheck) {
// this.rangecheck = rangecheck;
// }
//
// public void setIgnore(String ignore) {
// this.ignore = ignore;
// }
//
// public void setExpected(Throwable expected) {
// this.expected = expected;
// }
//
// public void setName(String name) {
// this.name = name;
// }
//
// public void setCount(int count) {
// if (count < 1) {
// throw new ComponentException("Illegal number of test cases: " + count);
// }
// this.count = count;
// }
//
// public void setPre(Closure pre) {
// this.pre = pre;
// }
//
// public void setPost(Closure post) {
// this.post = post;
// }
//
// @Override
// public Buildable create(Object name, Object value) {
// return LEAF;
// }
//
// private String getTestName(int no) {
// return name != null ? name : ("test-" + no);
// }
//
// /**
// *
// * @param testNo
// * @throws Exception
// */
// private void run(int testNo) throws Exception {
// if (ignore!=null) {
// System.out.println(getTestName(testNo) + " [ignored: " + ignore + "]");
// return;
// }
//
//
// System.out.print(getTestName(testNo) + " ");
//
// // Path
// String libPath = model.getLibpath();
// if (libPath != null) {
// System.setProperty("jna.library.path", libPath);
// if (log.isLoggable(Level.CONFIG)) {
// log.config("Setting jna.library.path to " + libPath);
// }
// }
//
// final Object comp = model.getComponent();
// if (log.isLoggable(Level.CONFIG)) {
// log.config("TL component " + comp);
// }
//
// Map parameter = model.getParameter();
// ComponentAccess.setInputData(parameter, comp, log);
//
// List data_ins = null;
// Map[] ps = null;
//
// int numruns = count;
//
// if (data != null) {
// data_ins = getfromData(data, comp);
// numruns = data.size() / data_ins.size() - 1;
// ps = getParamsets(data_ins, data);
// }
//
// for (int i = 0; i < numruns; i++) {
// if (log.isLoggable(Level.INFO)) {
// log.info("Test ... " + i);
// }
//
// try {
// if (data != null) {
// ComponentAccess.setInputData(ps[i], comp, log);
// }
//
// if (pre != null) {
// if (log.isLoggable(Level.INFO)) {
// log.info(" Pre ...");
// }
// pre.call(comp);
// }
// /////////////// Init
// if (log.isLoggable(Level.INFO)) {
// log.info(" Init ...");
// }
// ComponentAccess.callAnnotated(comp, Initialize.class, true);
//
// if (rangecheck) {
// ComponentAccess.rangeCheck(comp, true, false);
// }
//
// /////////////// Execute
// if (log.isLoggable(Level.INFO)) {
// log.info(" Execute ...");
// }
// if (timeout > 0) {
// // with execution timeout
// ExecutorService service = Executors.newSingleThreadExecutor();
// Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy