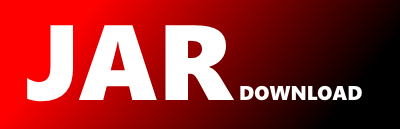
org.hortonmachine.gears.utils.StreamUtils Maven / Gradle / Ivy
/*
* This file is part of HortonMachine (http://www.hortonmachine.org)
* (C) HydroloGIS - www.hydrologis.com
*
* The HortonMachine is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.hortonmachine.gears.utils;
import java.util.List;
import java.util.LongSummaryStatistics;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.TreeSet;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.function.ToLongFunction;
import java.util.stream.Collectors;
import java.util.stream.DoubleStream;
import java.util.stream.IntStream;
import java.util.stream.LongStream;
import java.util.stream.Stream;
/**
* Utilities for Streams. This more a streams j8 documentation then something to really use.
*
* Use .peek(e->sysout) to debug without interference on the stream's items
* Use primitive streams were possible: {@link IntStream}, {@link LongStream}, {@link DoubleStream}.
*
* Intermediate Methods, which produce again streams:
*
* - map
* - filter
* - distinct
* - sorted
* - peek
* - limit
* - substream
* - parallel
* - sequential
* - unordered
*
*
* Terminate Methods, after which the stream is consumed and no more operations can be performed on it:
*
- forEach
* - forEachOrdered
* - toArray
* - reduce
* - collect
* - min
* - max
* - count
* - anyMatch
* - allMatch
* - noneMatch
* - findFirst
* - findAny
* - iterator
*
* Short-circuit Methods, that stop stream processing once conditions are satisfied.
*
- anyMatch
* - allMatch
* - noneMatch
* - findFirst
* - findAny
* - limit
* - substream
*
*
* @author Antonello Andrea (www.hydrologis.com)
*/
public class StreamUtils {
public static Stream fromArray( T[] array ) {
return Stream.of(array);
}
public static Stream fromList( List list ) {
return list.stream();
}
public static Stream fromListParallel( List list ) {
return list.parallelStream();
}
public static Stream fromSupplier( Supplier supplier ) {
return Stream.generate(supplier);
}
public static Stream distinct( Stream stream ) {
return stream.distinct();
}
public static long countStrings( Stream stream, boolean distinct, boolean ignoreCase ) {
if (ignoreCase && distinct) {
return stream.map(String::toLowerCase).distinct().count();
} else if (ignoreCase) {
return stream.map(String::toLowerCase).count();
} else if (distinct) {
return stream.distinct().count();
} else {
return stream.count();
}
}
public static String getString( Stream
© 2015 - 2025 Weber Informatics LLC | Privacy Policy