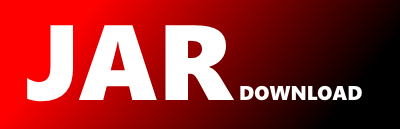
org.hothub.module.common.entity.BinaryDigit Maven / Gradle / Ivy
The newest version!
package org.hothub.module.common.entity;
import org.hothub.module.common.base.BinaryUnit;
import java.io.Serializable;
import java.math.BigDecimal;
import java.math.RoundingMode;
/**
* 数字转存储单位
*/
public class BinaryDigit implements Serializable {
private BigDecimal origin;
private BigDecimal result;
private BinaryUnit fromUnit = BinaryUnit.B;
private BinaryUnit toUnit;
private int scale = 0;
private RoundingMode roundingMode = RoundingMode.HALF_DOWN;
public static BinaryDigit with(BigDecimal origin) {
return new BinaryDigit(origin);
}
private BinaryDigit(BigDecimal origin) {
this.origin = origin;
}
public BinaryDigit from(BinaryUnit fromUnit) {
if (fromUnit != null) {
this.fromUnit = fromUnit;
}
return this;
}
public BinaryDigit scale(int newScale, RoundingMode roundingMode) {
if (newScale > 0) {
this.scale = newScale;
}
if (roundingMode != null) {
this.roundingMode = roundingMode;
}
return this;
}
public BinaryDigit compute() {
if (origin == null || origin.compareTo(BigDecimal.ZERO) <= 0) {
result = BigDecimal.ZERO.setScale(scale, roundingMode);
} else {
double i = Math.floor(Math.log(origin.doubleValue()) / Math.log(1024));
this.result = new BigDecimal(origin.doubleValue() / Math.pow(1024, i)).setScale(scale, roundingMode);
this.toUnit = BinaryUnit.getUnit((int) (fromUnit.ordinal() + i));
}
return this;
}
public BinaryDigit compute(BinaryUnit toUnit) {
if (origin == null || origin.compareTo(BigDecimal.ZERO) <= 0) {
result = BigDecimal.ZERO.setScale(scale, roundingMode);
} else {
double i = Math.floor(Math.log(origin.doubleValue()) / Math.log(1024));
double value = origin.doubleValue() / Math.pow(1024, i);
int from = fromUnit.ordinal();
int to = toUnit.ordinal();
this.result = new BigDecimal(from > to ? value * (1024 * (from - to)) : (from < to ? value / (1024 * (to - from)) : value)).setScale(scale, roundingMode);
this.toUnit = toUnit;
}
return this;
}
public BigDecimal getResult() {
return this.result;
}
public BinaryUnit getUnit() {
return this.toUnit;
}
@Override
public String toString() {
return result + " " + (this.toUnit != null ? this.toUnit.name() : "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy