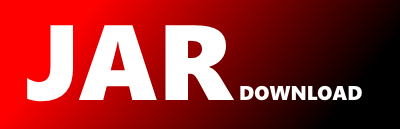
org.hotrod.runtime.livesql.dialects.PostgreSQLDialect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hotrod-livesql Show documentation
Show all versions of hotrod-livesql Show documentation
HotRod is an ORM for Java, Spring and SpringBoot.
package org.hotrod.runtime.livesql.dialects;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import org.hotrod.runtime.livesql.exceptions.UnsupportedLiveSQLFeatureException;
import org.hotrod.runtime.livesql.expressions.datetime.DateTimeExpression;
import org.hotrod.runtime.livesql.expressions.numbers.NumberExpression;
import org.hotrod.runtime.livesql.expressions.strings.StringExpression;
import org.hotrod.runtime.livesql.ordering.OrderingTerm;
import org.hotrod.runtime.livesql.queries.select.CrossJoin;
import org.hotrod.runtime.livesql.queries.select.FullOuterJoin;
import org.hotrod.runtime.livesql.queries.select.InnerJoin;
import org.hotrod.runtime.livesql.queries.select.Join;
import org.hotrod.runtime.livesql.queries.select.JoinLateral;
import org.hotrod.runtime.livesql.queries.select.LeftJoinLateral;
import org.hotrod.runtime.livesql.queries.select.LeftOuterJoin;
import org.hotrod.runtime.livesql.queries.select.NaturalFullOuterJoin;
import org.hotrod.runtime.livesql.queries.select.NaturalInnerJoin;
import org.hotrod.runtime.livesql.queries.select.NaturalLeftOuterJoin;
import org.hotrod.runtime.livesql.queries.select.NaturalRightOuterJoin;
import org.hotrod.runtime.livesql.queries.select.QueryWriter;
import org.hotrod.runtime.livesql.queries.select.RightOuterJoin;
import org.hotrod.runtime.livesql.queries.select.UnionJoin;
import org.hotrodorm.hotrod.utils.Separator;
public class PostgreSQLDialect extends LiveSQLDialect {
public PostgreSQLDialect(final boolean discovered, final String productName, final String productVersion,
final int majorVersion, final int minorVersion) {
super(discovered, productName, productVersion, majorVersion, minorVersion);
}
// WITH rendering
@Override
public WithRenderer getWithRenderer() {
return (c) -> "WITH" + (c ? " RECURSIVE" : "");
}
// From rendering
@Override
public FromRenderer getFromRenderer() {
return () -> "";
}
// Table Expression rendering
@Override
public TableExpressionRenderer getTableExpressionRenderer() {
return (columns) -> " ("
+ Arrays.stream(columns).map(c -> this.canonicalToNatural(c)).collect(Collectors.joining(", ")) + ")";
}
// Join rendering
@Override
public JoinRenderer getJoinRenderer() {
return new JoinRenderer() {
@Override
public String renderJoinKeywords(final Join join) throws UnsupportedLiveSQLFeatureException {
if (join instanceof InnerJoin) {
return "JOIN";
} else if (join instanceof LeftOuterJoin) {
return "LEFT JOIN";
} else if (join instanceof RightOuterJoin) {
return "RIGHT JOIN";
} else if (join instanceof FullOuterJoin) {
return "FULL JOIN";
} else if (join instanceof CrossJoin) {
return "CROSS JOIN";
} else if (join instanceof NaturalInnerJoin) {
return "NATURAL JOIN";
} else if (join instanceof NaturalLeftOuterJoin) {
return "NATURAL LEFT JOIN";
} else if (join instanceof NaturalRightOuterJoin) {
return "NATURAL RIGHT JOIN";
} else if (join instanceof NaturalFullOuterJoin) {
return "NATURAL FULL JOIN";
} else if (join instanceof JoinLateral) {
if (versionIsAtLeast(9, 3)) {
return "JOIN LATERAL";
}
throw new UnsupportedLiveSQLFeatureException(
"LiveSQL supports lateral joins in the PostgreSQL database starting in version 9.3, "
+ "but the current version is " + renderVersion());
} else if (join instanceof LeftJoinLateral) {
if (versionIsAtLeast(9, 3)) {
return "LEFT JOIN LATERAL";
}
throw new UnsupportedLiveSQLFeatureException(
"LiveSQL supports lateral joins in the PostgreSQL database starting in version 9.3, "
+ "but the current version is " + renderVersion());
} else if (join instanceof UnionJoin) {
throw new UnsupportedLiveSQLFeatureException("Union joins are not supported in PostgreSQL database");
} else {
throw new UnsupportedLiveSQLFeatureException(
"Invalid join type (" + join.getClass().getSimpleName() + ") in PostgreSQL database");
}
}
@Override
public String renderOptionalOnPredicate(final Join join) throws UnsupportedLiveSQLFeatureException {
if (join instanceof InnerJoin) {
return "";
} else if (join instanceof LeftOuterJoin) {
return "";
} else if (join instanceof RightOuterJoin) {
return "";
} else if (join instanceof FullOuterJoin) {
return "";
} else if (join instanceof CrossJoin) {
return "";
} else if (join instanceof NaturalInnerJoin) {
return "";
} else if (join instanceof NaturalLeftOuterJoin) {
return "";
} else if (join instanceof NaturalRightOuterJoin) {
return "";
} else if (join instanceof NaturalFullOuterJoin) {
return "";
} else if (join instanceof JoinLateral) {
return " ON true";
} else if (join instanceof LeftJoinLateral) {
return " ON true";
} else if (join instanceof UnionJoin) {
throw new UnsupportedLiveSQLFeatureException("Union joins are not supported in Oracle database");
} else {
throw new UnsupportedLiveSQLFeatureException(
"Invalid join type (" + join.getClass().getSimpleName() + ") in Oracle database");
}
}
};
}
// Pagination rendering
public PaginationRenderer getPaginationRenderer() {
return new PaginationRenderer() {
@Override
public PaginationType getPaginationType(final Integer offset, final Integer limit) {
return offset != null || limit != null ? PaginationType.BOTTOM : null;
}
@Override
public void renderTopPagination(final Integer offset, final Integer limit, final QueryWriter w) {
throw new UnsupportedLiveSQLFeatureException("Pagination can only be rendered at the bottom in PostgreSQL");
}
@Override
public void renderBottomPagination(final Integer offset, final Integer limit, final QueryWriter w) {
if (limit != null) {
if (offset != null) {
w.write("\nLIMIT " + limit + " OFFSET " + offset);
} else {
w.write("\nLIMIT " + limit);
}
} else {
w.write("\nOFFSET " + offset);
}
}
@Override
public void renderBeginEnclosingPagination(final Integer offset, final Integer limit, final QueryWriter w) {
throw new UnsupportedLiveSQLFeatureException("Pagination can only be rendered at the bottom in PostgreSQL");
}
@Override
public void renderEndEnclosingPagination(final Integer offset, final Integer limit, final QueryWriter w) {
throw new UnsupportedLiveSQLFeatureException("Pagination can only be rendered at the bottom in PostgreSQL");
}
};
}
// Set operation rendering
@Override
public SetOperatorRenderer getSetOperationRenderer() {
return new SetOperatorRenderer() {
@Override
public void renderUnion(final QueryWriter w) {
w.write("UNION");
}
@Override
public void renderUnionAll(final QueryWriter w) {
w.write("UNION ALL");
}
@Override
public void renderExcept(final QueryWriter w) {
w.write("EXCEPT");
}
@Override
public void renderExceptAll(final QueryWriter w) {
w.write("EXCEPT ALL");
}
@Override
public void renderIntersect(final QueryWriter w) {
w.write("INTERSECT");
}
@Override
public void renderIntersectAll(final QueryWriter w) {
w.write("INTERSECT ALL");
}
};
}
// Function rendering
@Override
public FunctionRenderer getFunctionRenderer() {
return new FunctionRenderer() {
// General purpose functions
@Override
public void groupConcat(final QueryWriter w, final boolean distinct, final StringExpression value,
final List ordering, final StringExpression separator) {
if (!versionIsAtLeast(9)) {
throw new UnsupportedLiveSQLFeatureException("This PostgreSQL version (" + renderVersion()
+ ") does not support the GROUP_CONCAT() function (string_agg()). Only available on PostgreSQL 9.0 or newer");
}
if (distinct) {
throw new UnsupportedLiveSQLFeatureException(
"PostgreSQL does not support DISTINCT on the GROUP_CONCAT() function (string_agg())");
}
if (separator == null) {
throw new UnsupportedLiveSQLFeatureException(
"PostgreSQL requires the separator to be specified on the GROUP_CONCAT() function (string_agg())");
}
w.write("string_agg(");
value.renderTo(w);
w.write(", ");
separator.renderTo(w);
if (ordering != null && !ordering.isEmpty()) {
w.write(" ORDER BY ");
Separator sep = new Separator();
for (OrderingTerm t : ordering) {
w.write(sep.render());
t.renderTo(w);
}
}
w.write(")");
}
// Arithmetic functions
@Override
public void logarithm(final QueryWriter w, final NumberExpression x, final NumberExpression base) {
if (base == null) {
this.write(w, "ln", x);
} else {
this.write(w, "log", base, x);
}
}
// String functions
@Override
public void locate(final QueryWriter w, final StringExpression substring, final StringExpression string,
final NumberExpression from) {
if (from == null) {
this.write(w, "strpos", string, substring);
} else {
throw new UnsupportedLiveSQLFeatureException(
"PostgreSQL does not support the parameter 'from' in the LOCATE function ('strpos' in PostgreSQL lingo)");
}
}
// Date/Time functions
@Override
public void currentDate(final QueryWriter w) {
w.write("current_date");
}
@Override
public void currentTime(final QueryWriter w) {
w.write("current_time");
}
@Override
public void currentDateTime(final QueryWriter w) {
w.write("current_timestamp");
}
@Override
public void date(final QueryWriter w, final DateTimeExpression datetime) {
datetime.renderTo(w);
w.write("::date");
}
@Override
public void time(final QueryWriter w, final DateTimeExpression datetime) {
datetime.renderTo(w);
w.write("::time");
}
@Override
public void dateTime(final QueryWriter w, final DateTimeExpression date, final DateTimeExpression time) {
w.write("(");
date.renderTo(w);
w.write(" + ");
time.renderTo(w);
w.write(")");
}
};
}
// New SQL Identifier rendering
private final String UNQUOTED_NATURAL = "[A-Za-z][A-Za-z0-9_]*";
private final String UNQUOTED_CANONICAL = "[a-z][a-z0-9_]*";
@Override
public String naturalToCanonical(final String natural) {
if (natural == null)
return null;
if (natural.matches(UNQUOTED_NATURAL)) {
return natural.toLowerCase();
}
return natural;
}
@Override
public String canonicalToNatural(final String canonical) {
if (canonical == null)
return null;
if (canonical.matches(UNQUOTED_CANONICAL)) {
return canonical.toLowerCase();
} else {
return "\"" + canonical.replace("\"", "\"\"") + "\"";
}
}
@Override
public DateTimeLiteralRenderer getDateTimeLiteralRenderer() {
return new DateTimeLiteralRenderer() {
@Override
public String renderDate(final String isoDate) {
return "DATE '" + isoDate + "'";
}
@Override
public String renderTime(final String isoTime, final int precision) {
return "TIME '" + isoTime + "'";
}
@Override
public String renderTimestamp(final String isoTimestamp, final int precision) {
return "TIMESTAMP '" + isoTimestamp + "'";
}
@Override
public String renderOffsetTime(final String isoTime, final String isoOffset, final int precision) {
return "TIME WITH TIME ZONE '" + isoTime + isoOffset + "'";
}
@Override
public String renderOffsetTimestamp(final String isoTimestamp, final String isoOffset, final int precision) {
return "TIMESTAMP WITH TIME ZONE '" + isoTimestamp + isoOffset + "'";
}
};
}
@Override
public BooleanLiteralRenderer getBooleanLiteralRenderer() {
return new BooleanLiteralRenderer() {
@Override
public void renderTrue(final QueryWriter w) {
w.write("true");
}
@Override
public void renderFalse(final QueryWriter w) {
w.write("false");
}
};
}
@Override
public boolean mandatoryColumnNamesInRecursiveCTEs() {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy