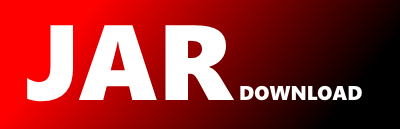
org.hpccsystems.ws.client.extended.HPCCWsSQLClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wsclient Show documentation
Show all versions of wsclient Show documentation
This project allows a user to interact with ESP services in a controlled manner. The API calls available under org.hpccsystems.ws.client.platform allow for a user to target ESP's across multiple environments running a range of hpccsystems-platform versions. There is no guarantee that if a user utilizes org.hpccsystems.ws.client.gen generated stub code from wsdl, that the calls will be backwards compatible with older hpccsystems-platform versions.
package org.hpccsystems.ws.client.extended;
import java.net.MalformedURLException;
import java.net.URL;
import java.rmi.RemoteException;
import java.util.List;
import org.apache.axis.client.Stub;
import org.apache.log4j.Logger;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ArrayOfEspException;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ECLException;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ECLWorkunit;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.EchoRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.EchoResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.EspException;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ExecutePreparedSQLRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ExecutePreparedSQLResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ExecuteSQLRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.ExecuteSQLResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.GetDBMetaDataRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.GetDBMetaDataResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.GetDBSystemInfoRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.GetDBSystemInfoResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.GetResultsRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.GetResultsResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.HPCCColumn;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.HPCCQuerySet;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.HPCCTable;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.NamedValue;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.OutputDataset;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.PrepareSQLRequest;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.PrepareSQLResponse;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.PublishedQuery;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.QuerySetAliasMap;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.QuerySignature;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.WssqlServiceSoap;
import org.hpccsystems.ws.client.gen.extended.wssql.v3_05.WssqlServiceSoapProxy;
import org.hpccsystems.ws.client.platform.Version;
import org.hpccsystems.ws.client.utils.Connection;
import org.hpccsystems.ws.client.utils.DataSingleton;
import org.hpccsystems.ws.client.utils.EqualsUtil;
import org.hpccsystems.ws.client.utils.HashCodeUtil;
import org.hpccsystems.ws.client.utils.Utils;
/**
* Use as soap client for HPCC WsSQL web service.
*
*/
public class HPCCWsSQLClient extends DataSingleton
{
private static final Logger log = Logger.getLogger(HPCCWsSQLClient.class.getName());
public static final String WSSQLURI = "/WsSQL";
private WssqlServiceSoapProxy wsSqlServiceSoapProxy = null;
private boolean verbose = false;
private Version version = null;
private static final int DEFAULT_RESULT_LIMIT = 100;
private static final String PINGSTATEMENT = "HPCCWsSQLClient Greets you.";
private static URL originalURL;
public static URL getOriginalURL() throws MalformedURLException
{
if (originalURL == null)
originalURL = new URL(getOriginalWSDLURL());
return originalURL;
}
public static int getOriginalPort() throws MalformedURLException
{
return getOriginalURL().getPort();
}
/**
* @param verbose - sets verbose mode
*/
public void setVerbose(boolean verbose)
{
this.verbose = verbose;
}
/**
* Provides soapproxy object for HPCCWsSQLClient which can be used to access the web service methods directly
*
* @return soapproxy for HPCCWsSQLClient
* @throws Exception
*/
public WssqlServiceSoapProxy getSoapProxy() throws Exception
{
if (wsSqlServiceSoapProxy != null)
{
return wsSqlServiceSoapProxy;
}
else
{
throw new Exception("wsSqlServiceSoapProxy not available.");
}
}
/**
* Provides the WSDL URL originally used to create the underlying stub code
*
* @return original WSLD URL
*/
public static String getOriginalWSDLURL()
{
return (new org.hpccsystems.ws.client.gen.extended.wssql.v3_05.WssqlLocator()).getwssqlServiceSoapAddress();
}
/**
* @param wsDfuServiceSoapProxy
*/
protected HPCCWsSQLClient(WssqlServiceSoapProxy wsSqlServiceSoapProxy)
{
this.wsSqlServiceSoapProxy = wsSqlServiceSoapProxy;
}
/**
* @param baseConnection
*/
public HPCCWsSQLClient(Connection baseConnection)
{
this(baseConnection.getProtocol(), baseConnection.getHost(), baseConnection.getPort(), baseConnection.getUserName(), baseConnection.getPassword());
}
public static HPCCWsSQLClient get(Connection connection)
{
return new HPCCWsSQLClient(connection);
}
/**
* @param protocol - http or https
* @param targetHost - server IP/name of the HPCC Cluster
* @param targetPort - port of the HPCC Cluster
* @param user - username to use when connecting to the HPCC Cluster
* @param pass - Password to use when connecting to the HPCC Cluster
*/
protected HPCCWsSQLClient(String protocol, String targetHost, String targetPort, String user, String pass)
{
String address = Connection.buildUrl(protocol, targetHost, targetPort, WSSQLURI);
initHPCCWsSQLSoapProxy(address, user, pass);
}
/**
* Initializes the service's underlying soap proxy. Should only be used by constructors
*
* @param baseURL
* Target service base URL
* @param user
* User credentials
* @param pass
* User credentials
*/
private void initHPCCWsSQLSoapProxy(String baseURL, String user, String pass)
{
wsSqlServiceSoapProxy = new WssqlServiceSoapProxy(baseURL);
if (wsSqlServiceSoapProxy != null)
{
WssqlServiceSoap wsSqlServiceSoap = wsSqlServiceSoapProxy.getWssqlServiceSoap();
if (wsSqlServiceSoap != null)
{
if (user != null && pass != null) Connection.initStub((Stub) wsSqlServiceSoap, user, pass);
}
}
}
public boolean isWsSQLReachable()
{
boolean reachable = false;
try
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
EchoResponse echoResponse = soapProxy.echo(new EchoRequest(PINGSTATEMENT));
if (echoResponse != null && echoResponse.getResponse().equals(PINGSTATEMENT))
reachable = true;
}
catch (Exception e) {}
return reachable;
}
public String [] getTargetClusters(String filter) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
String[] clusterNames = null;
try
{
GetDBMetaDataRequest getDBMetaDataRequest = new GetDBMetaDataRequest();
getDBMetaDataRequest.setIncludeTargetClusters(true);
if (filter != null)
getDBMetaDataRequest.setClusterType(filter);
getDBMetaDataRequest.setIncludeStoredProcedures(false);
getDBMetaDataRequest.setIncludeTables(false);
GetDBMetaDataResponse clusters = soapProxy.getDBMetaData(getDBMetaDataRequest);
clusterNames = clusters.getClusterNames();
}
catch (Exception e) {}
return clusterNames;
}
public HPCCTable[] getTables(String filter) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
HPCCTable[] tablesList = null;
try
{
GetDBMetaDataRequest getDBMetaDataRequest = new GetDBMetaDataRequest();
getDBMetaDataRequest.setIncludeTables(true);
if (filter != null)
getDBMetaDataRequest.setClusterType(filter);
getDBMetaDataRequest.setIncludeStoredProcedures(false);
getDBMetaDataRequest.setIncludeTargetClusters(false);
GetDBMetaDataResponse tables = soapProxy.getDBMetaData(getDBMetaDataRequest);
tablesList = tables.getTables();
}
catch (Exception e)
{
log.error("Could not fetch file(s) " + e.getLocalizedMessage());
throw e;
}
return tablesList;
}
public HPCCQuerySet[] getStoredProcedures(String querysetname) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
HPCCQuerySet[] querysets = null;
try
{
GetDBMetaDataRequest getDBMetaDataRequest = new GetDBMetaDataRequest();
getDBMetaDataRequest.setIncludeStoredProcedures(true);
if (querysetname != null)
getDBMetaDataRequest.setQuerySet(querysetname);
getDBMetaDataRequest.setIncludeTables(false);
getDBMetaDataRequest.setIncludeTargetClusters(false);
GetDBMetaDataResponse storedProcs = soapProxy.getDBMetaData(getDBMetaDataRequest);
querysets = storedProcs.getQuerySets();
if (querysets != null && querysets.length > 0)
{
for (int i = 0; i < querysets.length; i++)
{
HPCCQuerySet queryset = querysets[i];
String qsname = queryset.getName();
QuerySetAliasMap[] querySetAliases = queryset.getQuerySetAliases();
for (int aliases = 0; aliases < querySetAliases.length; aliases++)
{
String qsaliasid = querySetAliases[aliases].getId();
String qsaliasname = querySetAliases[aliases].getName();
}
PublishedQuery[] querySetQueries = queryset.getQuerySetQueries();
for (int queries = 0; queries < querySetQueries.length; queries++)
{
String id = querySetQueries[queries].getId();
String qname = querySetQueries[queries].getName();
QuerySignature querySignature = querySetQueries[queries].getSignature();
HPCCColumn[] inParams = querySignature.getInParams();
OutputDataset[] resultSets = querySignature.getResultSets();
HPCCColumn[] outParams = resultSets[0].getOutParams();
}
}
}
}
catch (Exception e) {}
return querysets;
}
public Version getVersion()
{
populateSystemInfo();
return version;
}
private boolean populateSystemInfo()
{
boolean success = false;
if (version == null)
{
WssqlServiceSoapProxy soapProxy = null;
try
{
soapProxy = getSoapProxy();
}
catch (Exception e)
{
log.error("Could not fetch System info, WsSQLServiceProxy not available.");
return false;
}
try
{
GetDBSystemInfoResponse dbSystemInfo = soapProxy.getDBSystemInfo(new GetDBSystemInfoRequest(true));
if (dbSystemInfo != null)
{
version = new Version(dbSystemInfo.getFullVersion());
if (version != null)
success = true;
}
}
catch (RemoteException e)
{
log.error("Error fetching HPCC System info.");
}
}
return success;
}
public String executeSQLWUIDResponse(String sqlText, String targetCluster, String targetQuerySet) throws Exception
{
return executeSQLWUResponse(sqlText, targetCluster, targetQuerySet, DEFAULT_RESULT_LIMIT, null /*resultWindowCount*/, null /*resultWindowStart*/, null /*suppressResults*/, true /*Boolean suppressXmlSchema*/, null /*String userName*/, null /*Integer wait*/).getWuid();
}
public ExecuteSQLResponse executeSQLFullResponse(String sqlText, String targetCluster, String targetQuerySet, Integer resultLimit, Integer resultWindowCount, Integer resultWindowStart, Boolean suppressResults, Boolean suppressXmlSchema, String userName, Integer wait) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
ExecuteSQLRequest executeSQLRequest = new ExecuteSQLRequest();
executeSQLRequest.setResultLimit(resultLimit);
executeSQLRequest.setResultWindowCount(resultWindowCount);
executeSQLRequest.setResultWindowStart(resultWindowStart);
executeSQLRequest.setSqlText(sqlText);
executeSQLRequest.setSuppressResults(suppressResults);
executeSQLRequest.setSuppressXmlSchema(suppressXmlSchema);
executeSQLRequest.setTargetCluster(targetCluster);
executeSQLRequest.setTargetQuerySet(targetQuerySet);
executeSQLRequest.setUserName(userName);
executeSQLRequest.setWait(wait);
ExecuteSQLResponse executeSQLResponse = soapProxy.executeSQL(executeSQLRequest);
if (executeSQLResponse != null)
{
ArrayOfEspException exceptions = executeSQLResponse.getExceptions();
if (exceptions!=null)
handleESPExceptions(exceptions);
if (executeSQLResponse.getWorkunit() != null)
handleECLExceptions(executeSQLResponse.getWorkunit().getExceptions());
return executeSQLResponse;
}
return null;
}
public ECLWorkunit executeSQLWUResponse(String sqlText, String targetCluster, String targetQuerySet, Integer resultLimit, Integer resultWindowCount, Integer resultWindowStart, Boolean suppressResults, Boolean suppressXmlSchema, String userName, Integer wait) throws Exception
{
return executeSQLFullResponse(sqlText, targetCluster, targetQuerySet, DEFAULT_RESULT_LIMIT, null /*resultWindowCount*/, null /*resultWindowStart*/, null /*suppressResults*/, true /*Boolean suppressXmlSchema*/, null /*String userName*/, null /*Integer wait*/).getWorkunit();
}
public List> getResutls(String wuid, Integer resultWindowStart, Integer resultWindowCount) throws Exception
{
return Utils.parseECLResults(getResultResponse(wuid, resultWindowStart, resultWindowCount, true).getResult());
}
public GetResultsResponse getResultResponse(String wuid, Integer resultWindowStart, Integer resultWindowCount, Boolean suppressXmlSchema) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
GetResultsRequest getResultsRequest = new GetResultsRequest();
getResultsRequest.setWuId(wuid);
getResultsRequest.setResultWindowCount(resultWindowCount);
getResultsRequest.setResultWindowStart(resultWindowStart);
getResultsRequest.setSuppressXmlSchema(suppressXmlSchema);
GetResultsResponse results = soapProxy.getResults(getResultsRequest);
if (results != null)
{
ArrayOfEspException exceptions = results.getExceptions();
if (exceptions!=null)
handleESPExceptions(exceptions);
ECLWorkunit workunit = results.getWorkunit();
if (workunit != null)
handleECLExceptions(workunit.getExceptions());
return results;
}
return null;
}
public String getResultSchemaXML(String wuid) throws Exception
{
return Utils.extactResultSchema(""+getResultResponse(wuid, 0, 0, false).getResult()+" ");
}
public List> getResultSchema(String wuid) throws Exception
{
return Utils.parseOutResultSchema(""+getResultResponse(wuid, 0, 0, false).getResult()+" ");
}
public ECLWorkunit prepareSQL(String sqlText, String targetCluster, String targetQuerySet, Integer wait) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
PrepareSQLRequest prepareSQLRequest = new PrepareSQLRequest();
prepareSQLRequest.setSqlText(sqlText);
prepareSQLRequest.setTargetCluster(targetCluster);
prepareSQLRequest.setTargetQuerySet(targetQuerySet);
prepareSQLRequest.setWait(wait);
PrepareSQLResponse prepareSQL = soapProxy.prepareSQL(prepareSQLRequest);
if (prepareSQL != null)
{
ArrayOfEspException exceptions = prepareSQL.getExceptions();
if (exceptions!=null)
handleESPExceptions(exceptions);
ECLWorkunit workunit = prepareSQL.getWorkunit();
if (workunit != null)
handleECLExceptions(workunit.getExceptions());
return workunit;
}
return null;
}
public ECLWorkunit executePreparedSQL(String wuid, String targetCluster, NamedValue[] variables, Integer wait, Integer resultLimit, String userName) throws Exception
{
return executePreparedSQL(wuid, targetCluster, variables, wait, resultLimit, null, null, userName, true, true).getWorkunit();
}
public List> executePreparedSQL(String wuid, String targetCluster, NamedValue[] variables, Integer wait, Integer resultLimit, String userName, String somesing) throws Exception
{
ExecutePreparedSQLResponse executePreparedSQL = executePreparedSQL(wuid, targetCluster, variables, wait, resultLimit, null, null, userName, true, true);
String result = executePreparedSQL.getResult();
return Utils.parseECLResults(result);
}
public ExecutePreparedSQLResponse executePreparedSQL(String wuid, String targetCluster, NamedValue[] variables, Integer wait, Integer resultLimit, Integer resultWindowStart, Integer resultWindowCount, String userName, Boolean suppressXmlSchema, Boolean suppressResults) throws Exception
{
WssqlServiceSoapProxy soapProxy = getSoapProxy();
ExecutePreparedSQLRequest executePreparedSQLRequest = new ExecutePreparedSQLRequest();
executePreparedSQLRequest.setWuId(wuid);
executePreparedSQLRequest.setVariables(variables);
executePreparedSQLRequest.setResultWindowStart(resultWindowStart);
executePreparedSQLRequest.setResultWindowCount(resultWindowCount);
executePreparedSQLRequest.setSuppressXmlSchema(suppressXmlSchema);
executePreparedSQLRequest.setSuppressResults(suppressResults);
executePreparedSQLRequest.setTargetCluster(targetCluster);
executePreparedSQLRequest.setUserName(userName);
executePreparedSQLRequest.setWait(wait);
ExecutePreparedSQLResponse executePreparedSQL = soapProxy.executePreparedSQL(executePreparedSQLRequest);
if (executePreparedSQL != null)
{
ArrayOfEspException exceptions = executePreparedSQL.getExceptions();
if (exceptions!=null)
handleESPExceptions(exceptions);
if (executePreparedSQL.getWorkunit() != null)
handleECLExceptions(executePreparedSQL.getWorkunit().getExceptions());
return executePreparedSQL;
}
return null;
}
private void handleESPExceptions(ArrayOfEspException exp) throws Exception
{
if (exp != null && exp.getException() != null && exp.getException().length > 0)
{
String message = "";
for (int i = 0; i < exp.getException().length; i++)
{
EspException ex = exp.getException()[i];
log.error(ex.getMessage());
message = message + "Audience: " + ex.getAudience() + " Source: " + ex.getSource() + " Message: " + ex.getMessage()+"\n";
}
throw new Exception(message);
}
}
private void handleECLExceptions(ECLException[] eclexceptions) throws Exception
{
if (eclexceptions != null)
{
String message = "";
for (int eclexceptionindex = 0; eclexceptionindex < eclexceptions.length; eclexceptionindex++)
{
ECLException eclException = eclexceptions[eclexceptionindex];
log.error(eclException.getMessage());
message = message + "Severity: " + eclException.getSeverity() + " Source: " + eclException.getSource() + " Message: " + eclException.getMessage()+"\n";
}
throw new Exception(message);
}
}
public static void main (String[] args)
{
/* this main is only meant to be a example*/
//HPCCWsClient connector = new HPCCWsClient("http", "192.168.56.120","8010");
HPCCWsSQLClient wssqlclient = new HPCCWsSQLClient("http", "192.168.56.120","8018", null, null);
if (wssqlclient.isWsSQLReachable())
{
String wuid = null;
try
{
wuid = wssqlclient.executeSQLWUIDResponse("select * from regress::thor_local::personindexid", "hthor", null);
System.out.println("wuid: " + wuid);
}
catch (Exception e)
{
System.out.println("Exception while executing SQL: " + e.getLocalizedMessage());
}
try
{
wuid = wssqlclient.executeSQLWUIDResponse("select * from regress::hthor_payload::book", "hthor", null);
System.out.println("wuid: " + wuid);
}
catch (Exception e)
{
System.out.println("Exception while executing SQL: " + e.getLocalizedMessage());
}
if (wuid != null)
{
try
{
String resultSchema = wssqlclient.getResultSchemaXML(wuid);
if (resultSchema != null)
System.out.println(resultSchema);
List> resultSchema2 = wssqlclient.getResultSchema(wuid);
for (List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy