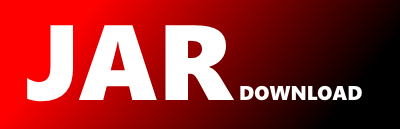
org.hpccsystems.ws.client.BaseHPCCWsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wsclient Show documentation
Show all versions of wsclient Show documentation
This project allows a user to interact with ESP services in a controlled manner. The API calls available under org.hpccsystems.ws.client.platform allow for a user to target ESP's across multiple environments running a range of hpccsystems-platform versions. There is no guarantee that if a user utilizes org.hpccsystems.ws.client.gen generated stub code from wsdl, that the calls will be backwards compatible with older hpccsystems-platform versions.
package org.hpccsystems.ws.client;
import java.io.ByteArrayInputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpression;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.apache.axis2.AxisFault;
import org.apache.axis2.addressing.EndpointReference;
import org.apache.axis2.client.Options;
import org.apache.axis2.client.Stub;
import org.apache.axis2.kernel.http.HTTPConstants;
import org.apache.axis2.transport.http.impl.httpclient4.HttpTransportPropertiesImpl;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.impl.client.BasicCredentialsProvider;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.hpccsystems.ws.client.platform.Version;
import org.hpccsystems.ws.client.utils.Connection;
import org.hpccsystems.ws.client.utils.DataSingleton;
import org.hpccsystems.ws.client.utils.EqualsUtil;
import org.hpccsystems.ws.client.utils.HashCodeUtil;
import org.hpccsystems.ws.client.utils.Utils;
import org.hpccsystems.ws.client.wrappers.ArrayOfECLExceptionWrapper;
import org.hpccsystems.ws.client.wrappers.ArrayOfEspExceptionWrapper;
import org.hpccsystems.ws.client.wrappers.EspSoapFaultWrapper;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import io.opentelemetry.api.GlobalOpenTelemetry;
import io.opentelemetry.api.OpenTelemetry;
import io.opentelemetry.api.trace.SpanBuilder;
import io.opentelemetry.api.trace.SpanKind;
import io.opentelemetry.api.trace.Tracer;
import io.opentelemetry.api.trace.propagation.W3CTraceContextPropagator;
import io.opentelemetry.context.Context;
import io.opentelemetry.instrumentation.annotations.WithSpan;
import io.opentelemetry.semconv.HttpAttributes;
import io.opentelemetry.semconv.ServerAttributes;
/**
* Defines functionality common to all HPCC Systems web service clients.
*
* Typically implemented by specialized HPCC Web service clients.
*/
public abstract class BaseHPCCWsClient extends DataSingleton
{
public static final String PROJECT_NAME = "WsClient";
private static OpenTelemetry globalOTel = null;
/** Constant log
*/
protected static final Logger log = LogManager.getLogger(BaseHPCCWsClient.class);
/** Constant DEAFULTECLWATCHPORT="8010"
*/
public static final String DEAFULTECLWATCHPORT = "8010";
/** Constant DEFAULTECLWATCHTLSPORT="18010"
*/
public static final String DEFAULTECLWATCHTLSPORT = "18010";
/** Constant DEFAULTSERVICEPORT="DEAFULTECLWATCHPORT"
*/
public static String DEFAULTSERVICEPORT = DEAFULTECLWATCHPORT;
protected Connection wsconn = null;
protected boolean verbose = false;
protected String initErrMessage = "";
protected Version targetHPCCBuildVersion = null;
protected Double targetESPInterfaceVer = null;
protected Boolean targetsContainerizedHPCC = null;
public boolean isTargetHPCCContainerized() throws Exception
{
if (targetsContainerizedHPCC == null)
{
if (wsconn == null)
throw new Exception("BaseHPCCWsClient: Cannot get target HPCC containerized mode, client connection has not been initialized.");
targetsContainerizedHPCC = getTargetHPCCIsContainerized(wsconn);
}
return targetsContainerizedHPCC;
}
@WithSpan
private boolean getTargetHPCCIsContainerized(Connection conn) throws Exception
{
if (wsconn == null)
throw new Exception("Cannot get target HPCC containerized mode, client connection has not been initialized.");
String response = wsconn.sendGetRequest("wssmc/getbuildinfo");//throws
if (response == null || response.isEmpty())
throw new Exception("Cannot get target HPCC containerized mode, received empty " + wsconn.getBaseUrl() + " wssmc/getbuildinfo response");
setUpContainerizedParser();
Document document = null;
synchronized(m_XMLParser)
{
document = m_XMLParser.parse(new ByteArrayInputStream(response.getBytes(StandardCharsets.UTF_8)));
}
if (document == null)
throw new Exception("Cannot parse HPCC isContainerizedMode response.");
NodeList namedValuesList = (NodeList) m_containerizedXpathExpression.evaluate(document,XPathConstants.NODESET);
for(int i = 0; i < namedValuesList.getLength(); i++)
{
Node ithNamedValuePair = namedValuesList.item(i);
NodeList nameAndValue = ithNamedValuePair.getChildNodes();
if (nameAndValue.getLength() == 2)
{
String name = null;
String value = null;
if (nameAndValue.item(0).getNodeName().equalsIgnoreCase("Name"))
{
name = nameAndValue.item(0).getFirstChild().getNodeValue();
value = nameAndValue.item(1).getFirstChild().getNodeValue();
}
else
{
name = nameAndValue.item(1).getFirstChild().getNodeValue();
value = nameAndValue.item(0).getFirstChild().getNodeValue();
}
if (name.equalsIgnoreCase("CONTAINERIZED"))
{
if (value.equalsIgnoreCase("ON"))
return true;
else
return false;
}
}
}
return false; //No CONTAINERIZED entry has to be assumed to mean target is not CONTAINERIZED
}
/**
* Gets the target HPCC build version
*
* @return the HPCC version
*/
public Version getTargetHPCCBuildVersion()
{
return targetHPCCBuildVersion;
}
@WithSpan
private String getTargetHPCCBuildVersionString() throws Exception
{
if (wsconn == null)
throw new Exception("Cannot get target HPCC build version, client connection has not been initialized.");
String response = wsconn.sendGetRequest("WsSMC/Activity?rawxml_");//throws IOException if http != ok
if (response == null || response.isEmpty())
throw new Exception("Cannot get target HPCC build version, received empty " + wsconn.getBaseUrl() + " wssmc/activity response");
String header = response.substring(0, 100).trim(); //crude, but can prevent wasteful overhead
if (header.startsWith("
© 2015 - 2025 Weber Informatics LLC | Privacy Policy