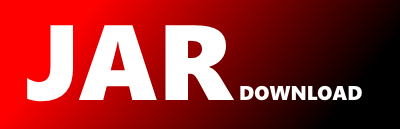
hprose.net.Reactor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hprose-java Show documentation
Show all versions of hprose-java Show documentation
Hprose is a High Performance Remote Object Service Engine.
It is a modern, lightweight, cross-language, cross-platform, object-oriented, high performance, remote dynamic communication middleware. It is not only easy to use, but powerful. You just need a little time to learn, then you can use it to easily construct cross language cross platform distributed application system.
Hprose supports many programming languages, for example:
* AAuto Quicker
* ActionScript
* ASP
* C++
* Dart
* Delphi/Free Pascal
* dotNET(C#, Visual Basic...)
* Golang
* Java
* JavaScript
* Node.js
* Objective-C
* Perl
* PHP
* Python
* Ruby
* ...
Through Hprose, You can conveniently and efficiently intercommunicate between those programming languages.
This project is the implementation of Hprose for Java.
/**********************************************************\
| |
| hprose |
| |
| Official WebSite: http://www.hprose.com/ |
| http://www.hprose.org/ |
| |
\**********************************************************/
/**********************************************************\
* *
* Reactor.java *
* *
* hprose Reactor class for Java. *
* *
* LastModified: Mar 8, 2016 *
* Author: Ma Bingyao *
* *
\**********************************************************/
package hprose.net;
import java.io.IOException;
import java.nio.channels.CancelledKeyException;
import java.nio.channels.ClosedChannelException;
import java.nio.channels.ClosedSelectorException;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.util.Iterator;
import java.util.Queue;
import java.util.Set;
import java.util.concurrent.ConcurrentLinkedQueue;
public final class Reactor extends Thread {
private final Selector selector;
private final Queue connQueue = new ConcurrentLinkedQueue();
private final Queue writeQueue = new ConcurrentLinkedQueue();
public Reactor() throws IOException {
super();
selector = Selector.open();
}
@Override
public void run() {
try {
while (!isInterrupted()) {
try {
process();
dispatch();
}
catch (IOException e) {}
}
}
catch (ClosedSelectorException e) {}
}
public void close() {
try {
Set keys = selector.keys();
for (SelectionKey key: keys.toArray(new SelectionKey[0])) {
Connection conn = (Connection) key.attachment();
conn.close();
}
selector.close();
}
catch (IOException e) {}
}
private void process() {
for (;;) {
final Connection conn = connQueue.poll();
if (conn == null) {
break;
}
try {
conn.connected(this, selector);
}
catch (ClosedChannelException e) {}
}
for (;;) {
final Connection conn = writeQueue.poll();
if (conn == null) {
break;
}
conn.send();
}
}
private void dispatch() throws IOException {
int n = selector.select();
if (n == 0) return;
Iterator it = selector.selectedKeys().iterator();
while (it.hasNext()) {
SelectionKey key = it.next();
Connection conn = (Connection) key.attachment();
it.remove();
try {
int readyOps = key.readyOps();
if ((readyOps & SelectionKey.OP_READ) != 0) {
if (!conn.receive()) continue;
}
if ((readyOps & SelectionKey.OP_WRITE) != 0) {
conn.send();
}
}
catch (CancelledKeyException e) {
conn.close();
}
}
}
public void register(Connection conn) {
connQueue.offer(conn);
selector.wakeup();
}
public void write(Connection conn) {
writeQueue.offer(conn);
selector.wakeup();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy