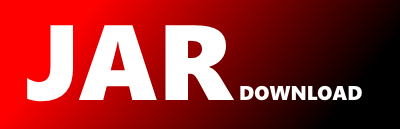
org.http4k.connect.amazon.s3.s3bucketExtensions.kt Maven / Gradle / Ivy
package org.http4k.connect.amazon.s3
import dev.forkhandles.result4k.Result
import java.io.InputStream
import kotlin.Int
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.sequences.Sequence
import org.http4k.connect.RemoteFailure
import org.http4k.connect.amazon.core.model.Tag
import org.http4k.connect.amazon.s3.action.CopyObject
import org.http4k.connect.amazon.s3.action.DeleteBucket
import org.http4k.connect.amazon.s3.action.DeleteObject
import org.http4k.connect.amazon.s3.action.DeleteObjectTagging
import org.http4k.connect.amazon.s3.action.GetObject
import org.http4k.connect.amazon.s3.action.GetObjectTagging
import org.http4k.connect.amazon.s3.action.HeadBucket
import org.http4k.connect.amazon.s3.action.HeadObject
import org.http4k.connect.amazon.s3.action.ListObjectsV2
import org.http4k.connect.amazon.s3.action.ObjectList
import org.http4k.connect.amazon.s3.action.PutObject
import org.http4k.connect.amazon.s3.action.PutObjectTagging
import org.http4k.connect.amazon.s3.action.RestoreObject
import org.http4k.connect.amazon.s3.action.TaggingDirective
import org.http4k.connect.amazon.s3.model.BucketKey
import org.http4k.connect.amazon.s3.model.BucketName
import org.http4k.connect.amazon.s3.model.ObjectDetails
import org.http4k.connect.amazon.s3.model.ObjectSummary
import org.http4k.connect.amazon.s3.model.RestoreTier
import org.http4k.connect.amazon.s3.model.StorageClass
import org.http4k.core.Headers
/**
* @see org.http4k.connect.amazon.s3.action.CopyObject
*/
public fun S3Bucket.copyObject(
sourceBucket: BucketName,
source: BucketKey,
destination: BucketKey,
storageClass: StorageClass? = null,
tags: List? = null,
taggingDirective: TaggingDirective? = null,
headers: Headers = emptyList(),
): Result = invoke(CopyObject(sourceBucket, source, destination, storageClass,
tags, taggingDirective, headers))
/**
* @see org.http4k.connect.amazon.s3.action.DeleteBucket
*/
public fun S3Bucket.deleteBucket(): Result = invoke(DeleteBucket())
/**
* @see org.http4k.connect.amazon.s3.action.DeleteObject
*/
public fun S3Bucket.deleteObject(key: BucketKey): Result =
invoke(DeleteObject(key))
/**
* @see org.http4k.connect.amazon.s3.action.DeleteObjectTagging
*/
public fun S3Bucket.deleteObjectTagging(key: BucketKey): Result =
invoke(DeleteObjectTagging(key))
/**
* @see org.http4k.connect.amazon.s3.action.GetObject
*/
public fun S3Bucket.getObject(key: BucketKey): Result =
invoke(GetObject(key))
/**
* @see org.http4k.connect.amazon.s3.action.GetObjectTagging
*/
public fun S3Bucket.getObjectTagging(key: BucketKey): Result, RemoteFailure> =
invoke(GetObjectTagging(key))
/**
* @see org.http4k.connect.amazon.s3.action.HeadBucket
*/
public fun S3Bucket.headBucket(expectedBucketOwner: String? = null): Result =
invoke(HeadBucket(expectedBucketOwner))
/**
* @see org.http4k.connect.amazon.s3.action.HeadObject
*/
public fun S3Bucket.headObject(key: BucketKey): Result =
invoke(HeadObject(key))
/**
* @see org.http4k.connect.amazon.s3.action.ListObjectsV2
*/
public fun S3Bucket.listObjectsV2(
continuationToken: String? = null,
maxKeys: Int? = null,
prefix: String? = null,
delimiter: String? = null,
encodingType: String? = null,
expectedBucketOwner: String? = null,
requestPayer: String? = null,
): Result = invoke(ListObjectsV2(continuationToken, maxKeys, prefix,
delimiter, encodingType, expectedBucketOwner, requestPayer))
/**
* @see org.http4k.connect.amazon.s3.action.ListObjectsV2
*/
public fun S3Bucket.listObjectsV2Paginated(
continuationToken: String? = null,
maxKeys: Int? = null,
prefix: String? = null,
delimiter: String? = null,
encodingType: String? = null,
expectedBucketOwner: String? = null,
requestPayer: String? = null,
): Sequence, RemoteFailure>> = org.http4k.connect.paginated(::invoke,
ListObjectsV2(continuationToken, maxKeys, prefix, delimiter, encodingType, expectedBucketOwner,
requestPayer))
/**
* @see org.http4k.connect.amazon.s3.action.PutObject
*/
public fun S3Bucket.putObject(
key: BucketKey,
content: InputStream,
headers: Headers = emptyList(),
tags: List = emptyList(),
storageClass: StorageClass? = null,
): Result = invoke(PutObject(key, content, headers, tags, storageClass))
/**
* @see org.http4k.connect.amazon.s3.action.PutObjectTagging
*/
public fun S3Bucket.putObjectTagging(key: BucketKey, tags: List): Result =
invoke(PutObjectTagging(key, tags))
/**
* @see org.http4k.connect.amazon.s3.action.RestoreObject
*/
public fun S3Bucket.restoreObject(
key: BucketKey,
days: Int,
description: String? = null,
tier: RestoreTier? = null,
): Result = invoke(RestoreObject(key, days, description, tier))
© 2015 - 2025 Weber Informatics LLC | Privacy Policy