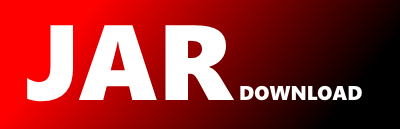
org.http4k.format.adapters.kt Maven / Gradle / Ivy
package org.http4k.format
import com.squareup.moshi.JsonAdapter
import com.squareup.moshi.JsonReader
import com.squareup.moshi.JsonWriter
import com.squareup.moshi.Moshi
import com.squareup.moshi.Types
import dev.forkhandles.values.AbstractValue
import org.http4k.events.Event
import java.lang.reflect.Type
import kotlin.reflect.KClass
/**
* Convenience class to create Moshi Adapter Factory
*/
open class SimpleMoshiAdapterFactory(vararg typesToAdapters: Pair JsonAdapter<*>>) :
JsonAdapter.Factory {
private val mappings = typesToAdapters.toMap()
override fun create(type: Type, annotations: Set, moshi: Moshi) =
mappings[Types.getRawType(type).typeName]?.let { it(moshi) }
}
/**
* Convenience function to create Moshi Adapter.
*/
inline fun , reified K> adapter(noinline fn: (Moshi) -> T) = K::class.java.name to fn
/**
* Convenience function to create Moshi Adapter Factory for a simple Moshi Adapter
*/
inline fun JsonAdapter.asFactory() = SimpleMoshiAdapterFactory(K::class.java.name to { this })
/**
* Convenience function to add a custom adapter.
*/
inline fun , reified K> Moshi.Builder.addTyped(fn: T): Moshi.Builder =
add(K::class.java, fn)
/**
* This adapter factory will capture ALL instances of a particular superclass/interface.
*/
abstract class IsAnInstanceOfAdapter(
private val clazz: KClass,
private val resolveAdapter: Moshi.(KClass) -> JsonAdapter = { adapter(it.java) }
) : JsonAdapter.Factory {
override fun create(type: Type, annotations: Set, moshi: Moshi) =
with(Types.getRawType(type)) {
when {
isA(clazz.java) -> moshi.resolveAdapter(clazz)
else -> null
}
}
private fun Class<*>?.isA(testCase: Class<*>): Boolean =
this?.let { testCase != this && testCase.isAssignableFrom(this) } ?: false
}
/**
* These adapters are the edge case adapters for dealing with Moshi
*/
object ThrowableAdapter : IsAnInstanceOfAdapter(Throwable::class)
object MapAdapter : IsAnInstanceOfAdapter
© 2015 - 2024 Weber Informatics LLC | Privacy Policy