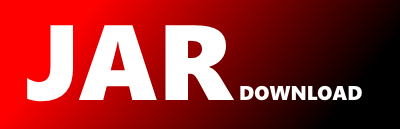
org.http4k.format.read.kt Maven / Gradle / Ivy
package org.http4k.format
import com.squareup.moshi.JsonReader
import com.squareup.moshi.JsonReader.Token.NULL
fun JsonReader.obj(mk: () -> T, fn: T.(String) -> Unit): T {
beginObject()
val item = mk()
while (hasNext()) item.fn(nextName())
endObject()
return item
}
fun JsonReader.obj(build: (Map) -> T, item: (String) -> Any): T {
beginObject()
val map = mutableMapOf()
while (hasNext()) nextName().also { map[it] = item(it) }
return build(map).also { endObject() }
}
fun JsonReader.list(mk: () -> T, item: T.(String) -> Unit) = skipNullOr {
val items = mutableListOf()
beginArray()
while (hasNext()) items += obj(mk, item)
endArray()
items
}
fun JsonReader.list(item: () -> T) = skipNullOr {
val items = mutableListOf()
beginArray()
while (hasNext()) items += item()
endArray()
items
}
fun JsonReader.stringList() = skipNullOr {
beginArray()
val list = mutableListOf()
while (hasNext()) list += nextString()
endArray()
list
}
fun JsonReader.stringMap(): Map? = skipNullOr {
beginObject()
val map = mutableMapOf()
while (hasNext()) map += nextName() to nextString()
endObject()
map
}
fun JsonReader.map(valueFn: () -> T) = skipNullOr {
beginObject()
val map = mutableMapOf()
while (hasNext()) map += nextName() to valueFn()
endObject()
map
}
fun JsonReader.stringOrNull() = if (peek() == NULL) nextNull() else nextString()
private fun JsonReader.skipNullOr(fn: JsonReader.() -> T) = if (peek() == NULL) skipValue().let { null } else fn()
© 2015 - 2024 Weber Informatics LLC | Privacy Policy