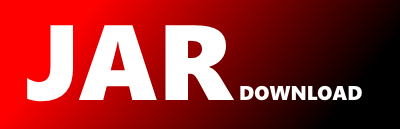
org.http4k.serverless.ApiGatewayRest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http4k-serverless-lambda Show documentation
Show all versions of http4k-serverless-lambda Show documentation
Http4k Serverless support for AWS Lambda
package org.http4k.serverless
import com.amazonaws.services.lambda.runtime.Context
import org.http4k.core.HttpHandler
import org.http4k.core.Method
import org.http4k.core.Request
import org.http4k.core.Response
import org.http4k.core.Uri
import org.http4k.core.toUrlFormEncoded
/**
* Function loader for ApiGatewayRest Lambdas
*
* Use main constructor if you need to read ENV variables to make your HttpHandler and the AWS context
*/
class ApiGatewayRestFnLoader(input: AppLoaderWithContexts) :
ApiGatewayFnLoader(ApiGatewayRestAwsHttpAdapter, input) {
/**
* Use this constructor if you need to read ENV variables to make your HttpHandler
*/
constructor(input: AppLoader) : this(AppLoaderWithContexts { env, _ -> input(env) })
/**
* Use this constructor if you just want to convert a standard HttpHandler
*/
constructor(input: HttpHandler) : this(AppLoader { input })
}
/**
* This is the main entry point for lambda invocations using the REST payload format.
* It uses the local environment to instantiate the HttpHandler which can be used
* for further invocations.
*/
abstract class ApiGatewayRestLambdaFunction(appLoader: AppLoaderWithContexts) :
AwsLambdaEventFunction(ApiGatewayRestFnLoader(appLoader)) {
constructor(input: AppLoader) : this(AppLoaderWithContexts { env, _ -> input(env) })
constructor(input: HttpHandler) : this(AppLoader { input })
}
object ApiGatewayRestAwsHttpAdapter : AwsHttpAdapter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy