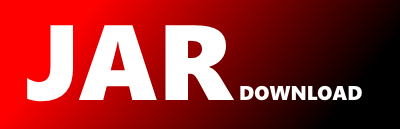
org.hudsonci.maven.model.config.BuildConfigurationDTO Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.06.15 at 07:34:37 PM PDT
//
package org.hudsonci.maven.model.config;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.annotation.Generated;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import com.flipthebird.gwthashcodeequals.EqualsBuilder;
import com.flipthebird.gwthashcodeequals.HashCodeBuilder;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import org.codehaus.jackson.annotate.JsonProperty;
import org.hudsonci.maven.model.PropertiesDTO;
/**
* Java class for buildConfiguration complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="buildConfiguration">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="installationId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="goals" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="privateRepository" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="privateTmpdir" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="pomFile" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="properties" type="{http://hudson-ci.org/xsd/hudson/2.1.0/maven/common}properties" minOccurs="0"/>
* <element name="errors" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="verbosity" type="{http://hudson-ci.org/xsd/hudson/2.1.0/maven/config}verbosity" minOccurs="0"/>
* <element name="offline" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="snapshotUpdateMode" type="{http://hudson-ci.org/xsd/hudson/2.1.0/maven/config}snapshotUpdateMode" minOccurs="0"/>
* <element name="profile" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="recursive" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="checksumMode" type="{http://hudson-ci.org/xsd/hudson/2.1.0/maven/config}checksumMode" minOccurs="0"/>
* <element name="failMode" type="{http://hudson-ci.org/xsd/hudson/2.1.0/maven/config}failMode" minOccurs="0"/>
* <element name="makeMode" type="{http://hudson-ci.org/xsd/hudson/2.1.0/maven/config}makeMode" minOccurs="0"/>
* <element name="project" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="resumeFrom" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="threading" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="mavenOpts" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="settingsId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="globalSettingsId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="toolChainsId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "buildConfiguration", propOrder = {
"installationId",
"goals",
"privateRepository",
"privateTmpdir",
"pomFile",
"properties",
"errors",
"verbosity",
"offline",
"snapshotUpdateMode",
"profiles",
"recursive",
"checksumMode",
"failMode",
"makeMode",
"projects",
"resumeFrom",
"threading",
"mavenOpts",
"settingsId",
"globalSettingsId",
"toolChainsId"
})
@XmlRootElement(name = "buildConfiguration")
@XStreamAlias("buildConfiguration")
@Generated(value = "XJC hudson-jaxb-ri-2.1-2", date = "2012-06-15T19:34:37")
public class BuildConfigurationDTO
implements Serializable
{
private final static long serialVersionUID = 1L;
@JsonProperty("installationId")
protected String installationId;
@JsonProperty("goals")
protected String goals;
@JsonProperty("privateRepository")
protected Boolean privateRepository;
@JsonProperty("privateTmpdir")
protected Boolean privateTmpdir;
@JsonProperty("pomFile")
protected String pomFile;
@JsonProperty("properties")
protected PropertiesDTO properties;
@JsonProperty("errors")
protected Boolean errors;
@JsonProperty("verbosity")
protected VerbosityDTO verbosity;
@JsonProperty("offline")
protected Boolean offline;
@JsonProperty("snapshotUpdateMode")
protected SnapshotUpdateModeDTO snapshotUpdateMode;
@XmlElement(name = "profile")
@JsonProperty("profiles")
protected List profiles;
@JsonProperty("recursive")
protected Boolean recursive;
@JsonProperty("checksumMode")
protected ChecksumModeDTO checksumMode;
@JsonProperty("failMode")
protected FailModeDTO failMode;
@JsonProperty("makeMode")
protected MakeModeDTO makeMode;
@XmlElement(name = "project")
@JsonProperty("projects")
protected List projects;
@JsonProperty("resumeFrom")
protected String resumeFrom;
@JsonProperty("threading")
protected String threading;
@JsonProperty("mavenOpts")
protected String mavenOpts;
@JsonProperty("settingsId")
protected String settingsId;
@JsonProperty("globalSettingsId")
protected String globalSettingsId;
@JsonProperty("toolChainsId")
protected String toolChainsId;
/**
* Gets the value of the installationId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInstallationId() {
return installationId;
}
/**
* Sets the value of the installationId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInstallationId(String value) {
this.installationId = value;
}
/**
* Gets the value of the goals property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGoals() {
return goals;
}
/**
* Sets the value of the goals property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGoals(String value) {
this.goals = value;
}
/**
* Gets the value of the privateRepository property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getPrivateRepository() {
return privateRepository;
}
/**
* Sets the value of the privateRepository property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setPrivateRepository(Boolean value) {
this.privateRepository = value;
}
/**
* Gets the value of the privateTmpdir property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getPrivateTmpdir() {
return privateTmpdir;
}
/**
* Sets the value of the privateTmpdir property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setPrivateTmpdir(Boolean value) {
this.privateTmpdir = value;
}
/**
* Gets the value of the pomFile property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPomFile() {
return pomFile;
}
/**
* Sets the value of the pomFile property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPomFile(String value) {
this.pomFile = value;
}
/**
* Gets the value of the properties property.
*
* @return
* possible object is
* {@link PropertiesDTO }
*
*/
public PropertiesDTO getProperties() {
return properties;
}
/**
* Sets the value of the properties property.
*
* @param value
* allowed object is
* {@link PropertiesDTO }
*
*/
public void setProperties(PropertiesDTO value) {
this.properties = value;
}
/**
* Gets the value of the errors property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getErrors() {
return errors;
}
/**
* Sets the value of the errors property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setErrors(Boolean value) {
this.errors = value;
}
/**
* Gets the value of the verbosity property.
*
* @return
* possible object is
* {@link VerbosityDTO }
*
*/
public VerbosityDTO getVerbosity() {
return verbosity;
}
/**
* Sets the value of the verbosity property.
*
* @param value
* allowed object is
* {@link VerbosityDTO }
*
*/
public void setVerbosity(VerbosityDTO value) {
this.verbosity = value;
}
/**
* Gets the value of the offline property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getOffline() {
return offline;
}
/**
* Sets the value of the offline property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setOffline(Boolean value) {
this.offline = value;
}
/**
* Gets the value of the snapshotUpdateMode property.
*
* @return
* possible object is
* {@link SnapshotUpdateModeDTO }
*
*/
public SnapshotUpdateModeDTO getSnapshotUpdateMode() {
return snapshotUpdateMode;
}
/**
* Sets the value of the snapshotUpdateMode property.
*
* @param value
* allowed object is
* {@link SnapshotUpdateModeDTO }
*
*/
public void setSnapshotUpdateMode(SnapshotUpdateModeDTO value) {
this.snapshotUpdateMode = value;
}
/**
* Gets the value of the profiles property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the profiles property.
*
*
* For example, to add a new item, do as follows:
*
* getProfiles().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getProfiles() {
if (profiles == null) {
profiles = new ArrayList();
}
return this.profiles;
}
/**
* Gets the value of the recursive property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getRecursive() {
return recursive;
}
/**
* Sets the value of the recursive property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRecursive(Boolean value) {
this.recursive = value;
}
/**
* Gets the value of the checksumMode property.
*
* @return
* possible object is
* {@link ChecksumModeDTO }
*
*/
public ChecksumModeDTO getChecksumMode() {
return checksumMode;
}
/**
* Sets the value of the checksumMode property.
*
* @param value
* allowed object is
* {@link ChecksumModeDTO }
*
*/
public void setChecksumMode(ChecksumModeDTO value) {
this.checksumMode = value;
}
/**
* Gets the value of the failMode property.
*
* @return
* possible object is
* {@link FailModeDTO }
*
*/
public FailModeDTO getFailMode() {
return failMode;
}
/**
* Sets the value of the failMode property.
*
* @param value
* allowed object is
* {@link FailModeDTO }
*
*/
public void setFailMode(FailModeDTO value) {
this.failMode = value;
}
/**
* Gets the value of the makeMode property.
*
* @return
* possible object is
* {@link MakeModeDTO }
*
*/
public MakeModeDTO getMakeMode() {
return makeMode;
}
/**
* Sets the value of the makeMode property.
*
* @param value
* allowed object is
* {@link MakeModeDTO }
*
*/
public void setMakeMode(MakeModeDTO value) {
this.makeMode = value;
}
/**
* Gets the value of the projects property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the projects property.
*
*
* For example, to add a new item, do as follows:
*
* getProjects().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getProjects() {
if (projects == null) {
projects = new ArrayList();
}
return this.projects;
}
/**
* Gets the value of the resumeFrom property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getResumeFrom() {
return resumeFrom;
}
/**
* Sets the value of the resumeFrom property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setResumeFrom(String value) {
this.resumeFrom = value;
}
/**
* Gets the value of the threading property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getThreading() {
return threading;
}
/**
* Sets the value of the threading property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setThreading(String value) {
this.threading = value;
}
/**
* Gets the value of the mavenOpts property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMavenOpts() {
return mavenOpts;
}
/**
* Sets the value of the mavenOpts property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMavenOpts(String value) {
this.mavenOpts = value;
}
/**
* Gets the value of the settingsId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSettingsId() {
return settingsId;
}
/**
* Sets the value of the settingsId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSettingsId(String value) {
this.settingsId = value;
}
/**
* Gets the value of the globalSettingsId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGlobalSettingsId() {
return globalSettingsId;
}
/**
* Sets the value of the globalSettingsId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGlobalSettingsId(String value) {
this.globalSettingsId = value;
}
/**
* Gets the value of the toolChainsId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getToolChainsId() {
return toolChainsId;
}
/**
* Sets the value of the toolChainsId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setToolChainsId(String value) {
this.toolChainsId = value;
}
public BuildConfigurationDTO withInstallationId(String value) {
setInstallationId(value);
return this;
}
public BuildConfigurationDTO withGoals(String value) {
setGoals(value);
return this;
}
public BuildConfigurationDTO withPrivateRepository(Boolean value) {
setPrivateRepository(value);
return this;
}
public BuildConfigurationDTO withPrivateTmpdir(Boolean value) {
setPrivateTmpdir(value);
return this;
}
public BuildConfigurationDTO withPomFile(String value) {
setPomFile(value);
return this;
}
public BuildConfigurationDTO withProperties(PropertiesDTO value) {
setProperties(value);
return this;
}
public BuildConfigurationDTO withErrors(Boolean value) {
setErrors(value);
return this;
}
public BuildConfigurationDTO withVerbosity(VerbosityDTO value) {
setVerbosity(value);
return this;
}
public BuildConfigurationDTO withOffline(Boolean value) {
setOffline(value);
return this;
}
public BuildConfigurationDTO withSnapshotUpdateMode(SnapshotUpdateModeDTO value) {
setSnapshotUpdateMode(value);
return this;
}
public BuildConfigurationDTO withProfiles(String... values) {
if (values!= null) {
for (String value: values) {
getProfiles().add(value);
}
}
return this;
}
public BuildConfigurationDTO withProfiles(Collection values) {
if (values!= null) {
getProfiles().addAll(values);
}
return this;
}
public BuildConfigurationDTO withRecursive(Boolean value) {
setRecursive(value);
return this;
}
public BuildConfigurationDTO withChecksumMode(ChecksumModeDTO value) {
setChecksumMode(value);
return this;
}
public BuildConfigurationDTO withFailMode(FailModeDTO value) {
setFailMode(value);
return this;
}
public BuildConfigurationDTO withMakeMode(MakeModeDTO value) {
setMakeMode(value);
return this;
}
public BuildConfigurationDTO withProjects(String... values) {
if (values!= null) {
for (String value: values) {
getProjects().add(value);
}
}
return this;
}
public BuildConfigurationDTO withProjects(Collection values) {
if (values!= null) {
getProjects().addAll(values);
}
return this;
}
public BuildConfigurationDTO withResumeFrom(String value) {
setResumeFrom(value);
return this;
}
public BuildConfigurationDTO withThreading(String value) {
setThreading(value);
return this;
}
public BuildConfigurationDTO withMavenOpts(String value) {
setMavenOpts(value);
return this;
}
public BuildConfigurationDTO withSettingsId(String value) {
setSettingsId(value);
return this;
}
public BuildConfigurationDTO withGlobalSettingsId(String value) {
setGlobalSettingsId(value);
return this;
}
public BuildConfigurationDTO withToolChainsId(String value) {
setToolChainsId(value);
return this;
}
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof BuildConfigurationDTO)) {
return false;
}
final BuildConfigurationDTO that = ((BuildConfigurationDTO) obj);
final EqualsBuilder builder = new EqualsBuilder();
builder.append(this.getInstallationId(), that.getInstallationId());
builder.append(this.getGoals(), that.getGoals());
builder.append(this.getPrivateRepository(), that.getPrivateRepository());
builder.append(this.getPrivateTmpdir(), that.getPrivateTmpdir());
builder.append(this.getPomFile(), that.getPomFile());
builder.append(this.getProperties(), that.getProperties());
builder.append(this.getErrors(), that.getErrors());
builder.append(this.getVerbosity(), that.getVerbosity());
builder.append(this.getOffline(), that.getOffline());
builder.append(this.getSnapshotUpdateMode(), that.getSnapshotUpdateMode());
builder.append(this.getProfiles(), that.getProfiles());
builder.append(this.getRecursive(), that.getRecursive());
builder.append(this.getChecksumMode(), that.getChecksumMode());
builder.append(this.getFailMode(), that.getFailMode());
builder.append(this.getMakeMode(), that.getMakeMode());
builder.append(this.getProjects(), that.getProjects());
builder.append(this.getResumeFrom(), that.getResumeFrom());
builder.append(this.getThreading(), that.getThreading());
builder.append(this.getMavenOpts(), that.getMavenOpts());
builder.append(this.getSettingsId(), that.getSettingsId());
builder.append(this.getGlobalSettingsId(), that.getGlobalSettingsId());
builder.append(this.getToolChainsId(), that.getToolChainsId());
return builder.build();
}
@Override
public int hashCode() {
final HashCodeBuilder builder = new HashCodeBuilder();
builder.append(this.getInstallationId());
builder.append(this.getGoals());
builder.append(this.getPrivateRepository());
builder.append(this.getPrivateTmpdir());
builder.append(this.getPomFile());
builder.append(this.getProperties());
builder.append(this.getErrors());
builder.append(this.getVerbosity());
builder.append(this.getOffline());
builder.append(this.getSnapshotUpdateMode());
builder.append(this.getProfiles());
builder.append(this.getRecursive());
builder.append(this.getChecksumMode());
builder.append(this.getFailMode());
builder.append(this.getMakeMode());
builder.append(this.getProjects());
builder.append(this.getResumeFrom());
builder.append(this.getThreading());
builder.append(this.getMavenOpts());
builder.append(this.getSettingsId());
builder.append(this.getGlobalSettingsId());
builder.append(this.getToolChainsId());
return builder.build();
}
}