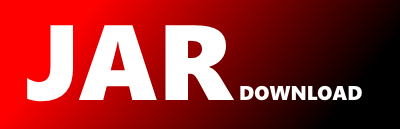
org.hudsonci.rest.model.build.BuildDTO Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.05.19 at 02:21:23 PM PDT
//
package org.hudsonci.rest.model.build;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import com.flipthebird.gwthashcodeequals.EqualsBuilder;
import com.flipthebird.gwthashcodeequals.HashCodeBuilder;
import org.codehaus.jackson.annotate.JsonProperty;
/**
* Java class for build complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="build">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="type" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="url" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="number" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="projectName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="duration" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="timeStamp" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="result" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/build}buildResult" minOccurs="0"/>
* <element name="state" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/build}buildState" minOccurs="0"/>
* <element name="kept" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="causes" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/build}cause" maxOccurs="unbounded" minOccurs="0"/>
* <element name="participants" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="culprits" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="changesAvailable" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="testsAvailable" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "build", propOrder = {
"type",
"url",
"id",
"number",
"description",
"projectName",
"duration",
"timeStamp",
"result",
"state",
"kept",
"causes",
"participants",
"culprits",
"changesAvailable",
"testsAvailable"
})
@XmlRootElement(name = "build")
public class BuildDTO {
@XmlElement(required = true)
@JsonProperty("type")
protected String type;
@XmlElement(required = true)
@JsonProperty("url")
protected String url;
@XmlElement(required = true)
@JsonProperty("id")
protected String id;
@JsonProperty("number")
protected int number;
@JsonProperty("description")
protected String description;
@XmlElement(required = true)
@JsonProperty("projectName")
protected String projectName;
@JsonProperty("duration")
protected Long duration;
@JsonProperty("timeStamp")
protected long timeStamp;
@JsonProperty("result")
protected BuildResultDTO result;
@JsonProperty("state")
protected BuildStateDTO state;
@JsonProperty("kept")
protected boolean kept;
@JsonProperty("causes")
protected List causes;
@JsonProperty("participants")
protected List participants;
@JsonProperty("culprits")
protected List culprits;
@JsonProperty("changesAvailable")
protected boolean changesAvailable;
@JsonProperty("testsAvailable")
protected boolean testsAvailable;
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setType(String value) {
this.type = value;
}
/**
* Gets the value of the url property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUrl() {
return url;
}
/**
* Sets the value of the url property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUrl(String value) {
this.url = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the number property.
*
*/
public int getNumber() {
return number;
}
/**
* Sets the value of the number property.
*
*/
public void setNumber(int value) {
this.number = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the projectName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProjectName() {
return projectName;
}
/**
* Sets the value of the projectName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProjectName(String value) {
this.projectName = value;
}
/**
* Gets the value of the duration property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getDuration() {
return duration;
}
/**
* Sets the value of the duration property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setDuration(Long value) {
this.duration = value;
}
/**
* Gets the value of the timeStamp property.
*
*/
public long getTimeStamp() {
return timeStamp;
}
/**
* Sets the value of the timeStamp property.
*
*/
public void setTimeStamp(long value) {
this.timeStamp = value;
}
/**
* Gets the value of the result property.
*
* @return
* possible object is
* {@link BuildResultDTO }
*
*/
public BuildResultDTO getResult() {
return result;
}
/**
* Sets the value of the result property.
*
* @param value
* allowed object is
* {@link BuildResultDTO }
*
*/
public void setResult(BuildResultDTO value) {
this.result = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link BuildStateDTO }
*
*/
public BuildStateDTO getState() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link BuildStateDTO }
*
*/
public void setState(BuildStateDTO value) {
this.state = value;
}
/**
* Gets the value of the kept property.
*
*/
public boolean isKept() {
return kept;
}
/**
* Sets the value of the kept property.
*
*/
public void setKept(boolean value) {
this.kept = value;
}
/**
* Gets the value of the causes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the causes property.
*
*
* For example, to add a new item, do as follows:
*
* getCauses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CauseDTO }
*
*
*/
public List getCauses() {
if (causes == null) {
causes = new ArrayList();
}
return this.causes;
}
/**
* Gets the value of the participants property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the participants property.
*
*
* For example, to add a new item, do as follows:
*
* getParticipants().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getParticipants() {
if (participants == null) {
participants = new ArrayList();
}
return this.participants;
}
/**
* Gets the value of the culprits property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the culprits property.
*
*
* For example, to add a new item, do as follows:
*
* getCulprits().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getCulprits() {
if (culprits == null) {
culprits = new ArrayList();
}
return this.culprits;
}
/**
* Gets the value of the changesAvailable property.
*
*/
public boolean isChangesAvailable() {
return changesAvailable;
}
/**
* Sets the value of the changesAvailable property.
*
*/
public void setChangesAvailable(boolean value) {
this.changesAvailable = value;
}
/**
* Gets the value of the testsAvailable property.
*
*/
public boolean isTestsAvailable() {
return testsAvailable;
}
/**
* Sets the value of the testsAvailable property.
*
*/
public void setTestsAvailable(boolean value) {
this.testsAvailable = value;
}
public BuildDTO withType(String value) {
setType(value);
return this;
}
public BuildDTO withUrl(String value) {
setUrl(value);
return this;
}
public BuildDTO withId(String value) {
setId(value);
return this;
}
public BuildDTO withNumber(int value) {
setNumber(value);
return this;
}
public BuildDTO withDescription(String value) {
setDescription(value);
return this;
}
public BuildDTO withProjectName(String value) {
setProjectName(value);
return this;
}
public BuildDTO withDuration(Long value) {
setDuration(value);
return this;
}
public BuildDTO withTimeStamp(long value) {
setTimeStamp(value);
return this;
}
public BuildDTO withResult(BuildResultDTO value) {
setResult(value);
return this;
}
public BuildDTO withState(BuildStateDTO value) {
setState(value);
return this;
}
public BuildDTO withKept(boolean value) {
setKept(value);
return this;
}
public BuildDTO withCauses(CauseDTO... values) {
if (values!= null) {
for (CauseDTO value: values) {
getCauses().add(value);
}
}
return this;
}
public BuildDTO withCauses(Collection values) {
if (values!= null) {
getCauses().addAll(values);
}
return this;
}
public BuildDTO withParticipants(String... values) {
if (values!= null) {
for (String value: values) {
getParticipants().add(value);
}
}
return this;
}
public BuildDTO withParticipants(Collection values) {
if (values!= null) {
getParticipants().addAll(values);
}
return this;
}
public BuildDTO withCulprits(String... values) {
if (values!= null) {
for (String value: values) {
getCulprits().add(value);
}
}
return this;
}
public BuildDTO withCulprits(Collection values) {
if (values!= null) {
getCulprits().addAll(values);
}
return this;
}
public BuildDTO withChangesAvailable(boolean value) {
setChangesAvailable(value);
return this;
}
public BuildDTO withTestsAvailable(boolean value) {
setTestsAvailable(value);
return this;
}
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof BuildDTO)) {
return false;
}
final BuildDTO that = ((BuildDTO) obj);
final EqualsBuilder builder = new EqualsBuilder();
builder.append(this.getType(), that.getType());
builder.append(this.getUrl(), that.getUrl());
builder.append(this.getId(), that.getId());
builder.append(this.getNumber(), that.getNumber());
builder.append(this.getDescription(), that.getDescription());
builder.append(this.getProjectName(), that.getProjectName());
builder.append(this.getDuration(), that.getDuration());
builder.append(this.getTimeStamp(), that.getTimeStamp());
builder.append(this.getResult(), that.getResult());
builder.append(this.getState(), that.getState());
builder.append(this.isKept(), that.isKept());
builder.append(this.getCauses(), that.getCauses());
builder.append(this.getParticipants(), that.getParticipants());
builder.append(this.getCulprits(), that.getCulprits());
builder.append(this.isChangesAvailable(), that.isChangesAvailable());
builder.append(this.isTestsAvailable(), that.isTestsAvailable());
return builder.build();
}
@Override
public int hashCode() {
final HashCodeBuilder builder = new HashCodeBuilder();
builder.append(this.getType());
builder.append(this.getUrl());
builder.append(this.getId());
builder.append(this.getNumber());
builder.append(this.getDescription());
builder.append(this.getProjectName());
builder.append(this.getDuration());
builder.append(this.getTimeStamp());
builder.append(this.getResult());
builder.append(this.getState());
builder.append(this.isKept());
builder.append(this.getCauses());
builder.append(this.getParticipants());
builder.append(this.getCulprits());
builder.append(this.isChangesAvailable());
builder.append(this.isTestsAvailable());
return builder.build();
}
}