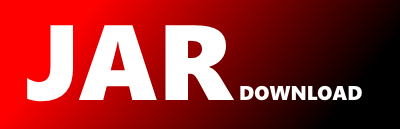
org.hudsonci.rest.model.project.ProjectDTO Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.05.19 at 02:21:23 PM PDT
//
package org.hudsonci.rest.model.project;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import com.flipthebird.gwthashcodeequals.EqualsBuilder;
import com.flipthebird.gwthashcodeequals.HashCodeBuilder;
import org.codehaus.jackson.annotate.JsonProperty;
import org.hudsonci.rest.model.build.BuildDTO;
/**
* Java class for project complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="project">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ref" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/project}projectReference"/>
* <element name="type" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="url" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="title" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="enabled" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="configurable" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="concurrent" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="queued" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="lastBuild" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/build}build" minOccurs="0"/>
* <element name="blocked" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="blockedReason" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="health" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/project}health" minOccurs="0"/>
* <element name="parent" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/project}projectReference"/>
* <element name="descendant" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/project}projectReference" maxOccurs="unbounded"/>
* <element name="upstream" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/project}projectReference" maxOccurs="unbounded"/>
* <element name="downstream" type="{http://hudson-ci.org/xsd/hudson/2.1.0/rest/project}projectReference" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "project", propOrder = {
"ref",
"type",
"id",
"url",
"name",
"title",
"description",
"enabled",
"configurable",
"concurrent",
"queued",
"lastBuild",
"blocked",
"blockedReason",
"health",
"parent",
"descendants",
"upstreams",
"downstreams"
})
@XmlRootElement(name = "project")
public class ProjectDTO {
@XmlElement(required = true)
@JsonProperty("ref")
protected ProjectReferenceDTO ref;
@XmlElement(required = true)
@JsonProperty("type")
protected String type;
@XmlElement(required = true)
@JsonProperty("id")
protected String id;
@XmlElement(required = true)
@JsonProperty("url")
protected String url;
@XmlElement(required = true)
@JsonProperty("name")
protected String name;
@XmlElement(required = true)
@JsonProperty("title")
protected String title;
@JsonProperty("description")
protected String description;
@JsonProperty("enabled")
protected boolean enabled;
@JsonProperty("configurable")
protected boolean configurable;
@JsonProperty("concurrent")
protected boolean concurrent;
@JsonProperty("queued")
protected boolean queued;
@JsonProperty("lastBuild")
protected BuildDTO lastBuild;
@JsonProperty("blocked")
protected Boolean blocked;
@JsonProperty("blockedReason")
protected String blockedReason;
@JsonProperty("health")
protected HealthDTO health;
@XmlElement(required = true)
@JsonProperty("parent")
protected ProjectReferenceDTO parent;
@XmlElement(name = "descendant", required = true)
@JsonProperty("descendants")
protected List descendants;
@XmlElement(name = "upstream", required = true)
@JsonProperty("upstreams")
protected List upstreams;
@XmlElement(name = "downstream", required = true)
@JsonProperty("downstreams")
protected List downstreams;
/**
* Gets the value of the ref property.
*
* @return
* possible object is
* {@link ProjectReferenceDTO }
*
*/
public ProjectReferenceDTO getRef() {
return ref;
}
/**
* Sets the value of the ref property.
*
* @param value
* allowed object is
* {@link ProjectReferenceDTO }
*
*/
public void setRef(ProjectReferenceDTO value) {
this.ref = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setType(String value) {
this.type = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the url property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUrl() {
return url;
}
/**
* Sets the value of the url property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUrl(String value) {
this.url = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the enabled property.
*
*/
public boolean isEnabled() {
return enabled;
}
/**
* Sets the value of the enabled property.
*
*/
public void setEnabled(boolean value) {
this.enabled = value;
}
/**
* Gets the value of the configurable property.
*
*/
public boolean isConfigurable() {
return configurable;
}
/**
* Sets the value of the configurable property.
*
*/
public void setConfigurable(boolean value) {
this.configurable = value;
}
/**
* Gets the value of the concurrent property.
*
*/
public boolean isConcurrent() {
return concurrent;
}
/**
* Sets the value of the concurrent property.
*
*/
public void setConcurrent(boolean value) {
this.concurrent = value;
}
/**
* Gets the value of the queued property.
*
*/
public boolean isQueued() {
return queued;
}
/**
* Sets the value of the queued property.
*
*/
public void setQueued(boolean value) {
this.queued = value;
}
/**
* Gets the value of the lastBuild property.
*
* @return
* possible object is
* {@link BuildDTO }
*
*/
public BuildDTO getLastBuild() {
return lastBuild;
}
/**
* Sets the value of the lastBuild property.
*
* @param value
* allowed object is
* {@link BuildDTO }
*
*/
public void setLastBuild(BuildDTO value) {
this.lastBuild = value;
}
/**
* Gets the value of the blocked property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isBlocked() {
return blocked;
}
/**
* Sets the value of the blocked property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setBlocked(Boolean value) {
this.blocked = value;
}
/**
* Gets the value of the blockedReason property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBlockedReason() {
return blockedReason;
}
/**
* Sets the value of the blockedReason property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBlockedReason(String value) {
this.blockedReason = value;
}
/**
* Gets the value of the health property.
*
* @return
* possible object is
* {@link HealthDTO }
*
*/
public HealthDTO getHealth() {
return health;
}
/**
* Sets the value of the health property.
*
* @param value
* allowed object is
* {@link HealthDTO }
*
*/
public void setHealth(HealthDTO value) {
this.health = value;
}
/**
* Gets the value of the parent property.
*
* @return
* possible object is
* {@link ProjectReferenceDTO }
*
*/
public ProjectReferenceDTO getParent() {
return parent;
}
/**
* Sets the value of the parent property.
*
* @param value
* allowed object is
* {@link ProjectReferenceDTO }
*
*/
public void setParent(ProjectReferenceDTO value) {
this.parent = value;
}
/**
* Gets the value of the descendants property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the descendants property.
*
*
* For example, to add a new item, do as follows:
*
* getDescendants().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProjectReferenceDTO }
*
*
*/
public List getDescendants() {
if (descendants == null) {
descendants = new ArrayList();
}
return this.descendants;
}
/**
* Gets the value of the upstreams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the upstreams property.
*
*
* For example, to add a new item, do as follows:
*
* getUpstreams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProjectReferenceDTO }
*
*
*/
public List getUpstreams() {
if (upstreams == null) {
upstreams = new ArrayList();
}
return this.upstreams;
}
/**
* Gets the value of the downstreams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the downstreams property.
*
*
* For example, to add a new item, do as follows:
*
* getDownstreams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProjectReferenceDTO }
*
*
*/
public List getDownstreams() {
if (downstreams == null) {
downstreams = new ArrayList();
}
return this.downstreams;
}
public ProjectDTO withRef(ProjectReferenceDTO value) {
setRef(value);
return this;
}
public ProjectDTO withType(String value) {
setType(value);
return this;
}
public ProjectDTO withId(String value) {
setId(value);
return this;
}
public ProjectDTO withUrl(String value) {
setUrl(value);
return this;
}
public ProjectDTO withName(String value) {
setName(value);
return this;
}
public ProjectDTO withTitle(String value) {
setTitle(value);
return this;
}
public ProjectDTO withDescription(String value) {
setDescription(value);
return this;
}
public ProjectDTO withEnabled(boolean value) {
setEnabled(value);
return this;
}
public ProjectDTO withConfigurable(boolean value) {
setConfigurable(value);
return this;
}
public ProjectDTO withConcurrent(boolean value) {
setConcurrent(value);
return this;
}
public ProjectDTO withQueued(boolean value) {
setQueued(value);
return this;
}
public ProjectDTO withLastBuild(BuildDTO value) {
setLastBuild(value);
return this;
}
public ProjectDTO withBlocked(Boolean value) {
setBlocked(value);
return this;
}
public ProjectDTO withBlockedReason(String value) {
setBlockedReason(value);
return this;
}
public ProjectDTO withHealth(HealthDTO value) {
setHealth(value);
return this;
}
public ProjectDTO withParent(ProjectReferenceDTO value) {
setParent(value);
return this;
}
public ProjectDTO withDescendants(ProjectReferenceDTO... values) {
if (values!= null) {
for (ProjectReferenceDTO value: values) {
getDescendants().add(value);
}
}
return this;
}
public ProjectDTO withDescendants(Collection values) {
if (values!= null) {
getDescendants().addAll(values);
}
return this;
}
public ProjectDTO withUpstreams(ProjectReferenceDTO... values) {
if (values!= null) {
for (ProjectReferenceDTO value: values) {
getUpstreams().add(value);
}
}
return this;
}
public ProjectDTO withUpstreams(Collection values) {
if (values!= null) {
getUpstreams().addAll(values);
}
return this;
}
public ProjectDTO withDownstreams(ProjectReferenceDTO... values) {
if (values!= null) {
for (ProjectReferenceDTO value: values) {
getDownstreams().add(value);
}
}
return this;
}
public ProjectDTO withDownstreams(Collection values) {
if (values!= null) {
getDownstreams().addAll(values);
}
return this;
}
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof ProjectDTO)) {
return false;
}
final ProjectDTO that = ((ProjectDTO) obj);
final EqualsBuilder builder = new EqualsBuilder();
builder.append(this.getRef(), that.getRef());
builder.append(this.getType(), that.getType());
builder.append(this.getId(), that.getId());
builder.append(this.getUrl(), that.getUrl());
builder.append(this.getName(), that.getName());
builder.append(this.getTitle(), that.getTitle());
builder.append(this.getDescription(), that.getDescription());
builder.append(this.isEnabled(), that.isEnabled());
builder.append(this.isConfigurable(), that.isConfigurable());
builder.append(this.isConcurrent(), that.isConcurrent());
builder.append(this.isQueued(), that.isQueued());
builder.append(this.getLastBuild(), that.getLastBuild());
builder.append(this.isBlocked(), that.isBlocked());
builder.append(this.getBlockedReason(), that.getBlockedReason());
builder.append(this.getHealth(), that.getHealth());
builder.append(this.getParent(), that.getParent());
builder.append(this.getDescendants(), that.getDescendants());
builder.append(this.getUpstreams(), that.getUpstreams());
builder.append(this.getDownstreams(), that.getDownstreams());
return builder.build();
}
@Override
public int hashCode() {
final HashCodeBuilder builder = new HashCodeBuilder();
builder.append(this.getRef());
builder.append(this.getType());
builder.append(this.getId());
builder.append(this.getUrl());
builder.append(this.getName());
builder.append(this.getTitle());
builder.append(this.getDescription());
builder.append(this.isEnabled());
builder.append(this.isConfigurable());
builder.append(this.isConcurrent());
builder.append(this.isQueued());
builder.append(this.getLastBuild());
builder.append(this.isBlocked());
builder.append(this.getBlockedReason());
builder.append(this.getHealth());
builder.append(this.getParent());
builder.append(this.getDescendants());
builder.append(this.getUpstreams());
builder.append(this.getDownstreams());
return builder.build();
}
}