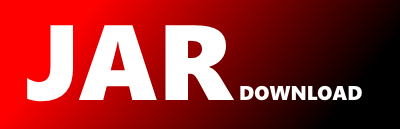
org.hyperledger.fabric.protos.token.Transaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fabric-chaincode-protos Show documentation
Show all versions of fabric-chaincode-protos Show documentation
Hyperldger Fabric Java chaincode protobuf files
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: token/transaction.proto
package org.hyperledger.fabric.protos.token;
public final class Transaction {
private Transaction() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface TokenTransactionOrBuilder extends
// @@protoc_insertion_point(interface_extends:TokenTransaction)
com.google.protobuf.MessageOrBuilder {
/**
* optional .PlainTokenAction plain_action = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction getPlainAction();
/**
* optional .PlainTokenAction plain_action = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder getPlainActionOrBuilder();
public org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.ActionCase getActionCase();
}
/**
*
* TokenTransaction governs the structure of Payload.data, when
* the transaction's envelope header indicates a transaction of type
* "Token"
*
*
* Protobuf type {@code TokenTransaction}
*/
public static final class TokenTransaction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:TokenTransaction)
TokenTransactionOrBuilder {
// Use TokenTransaction.newBuilder() to construct.
private TokenTransaction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TokenTransaction() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private TokenTransaction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder subBuilder = null;
if (actionCase_ == 1) {
subBuilder = ((org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_).toBuilder();
}
action_ =
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_);
action_ = subBuilder.buildPartial();
}
actionCase_ = 1;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_TokenTransaction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_TokenTransaction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.class, org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.Builder.class);
}
private int actionCase_ = 0;
private java.lang.Object action_;
public enum ActionCase
implements com.google.protobuf.Internal.EnumLite {
PLAIN_ACTION(1),
ACTION_NOT_SET(0);
private final int value;
private ActionCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ActionCase valueOf(int value) {
return forNumber(value);
}
public static ActionCase forNumber(int value) {
switch (value) {
case 1: return PLAIN_ACTION;
case 0: return ACTION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ActionCase
getActionCase() {
return ActionCase.forNumber(
actionCase_);
}
public static final int PLAIN_ACTION_FIELD_NUMBER = 1;
/**
* optional .PlainTokenAction plain_action = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction getPlainAction() {
if (actionCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder getPlainActionOrBuilder() {
if (actionCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (actionCase_ == 1) {
output.writeMessage(1, (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (actionCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.TokenTransaction)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.TokenTransaction other = (org.hyperledger.fabric.protos.token.Transaction.TokenTransaction) obj;
boolean result = true;
result = result && getActionCase().equals(
other.getActionCase());
if (!result) return false;
switch (actionCase_) {
case 1:
result = result && getPlainAction()
.equals(other.getPlainAction());
break;
case 0:
default:
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
switch (actionCase_) {
case 1:
hash = (37 * hash) + PLAIN_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getPlainAction().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.TokenTransaction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TokenTransaction governs the structure of Payload.data, when
* the transaction's envelope header indicates a transaction of type
* "Token"
*
*
* Protobuf type {@code TokenTransaction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:TokenTransaction)
org.hyperledger.fabric.protos.token.Transaction.TokenTransactionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_TokenTransaction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_TokenTransaction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.class, org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
actionCase_ = 0;
action_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_TokenTransaction_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.TokenTransaction getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.TokenTransaction build() {
org.hyperledger.fabric.protos.token.Transaction.TokenTransaction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.TokenTransaction buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.TokenTransaction result = new org.hyperledger.fabric.protos.token.Transaction.TokenTransaction(this);
if (actionCase_ == 1) {
if (plainActionBuilder_ == null) {
result.action_ = action_;
} else {
result.action_ = plainActionBuilder_.build();
}
}
result.actionCase_ = actionCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.TokenTransaction) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.TokenTransaction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.TokenTransaction other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.TokenTransaction.getDefaultInstance()) return this;
switch (other.getActionCase()) {
case PLAIN_ACTION: {
mergePlainAction(other.getPlainAction());
break;
}
case ACTION_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.TokenTransaction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.TokenTransaction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int actionCase_ = 0;
private java.lang.Object action_;
public ActionCase
getActionCase() {
return ActionCase.forNumber(
actionCase_);
}
public Builder clearAction() {
actionCase_ = 0;
action_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction, org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder> plainActionBuilder_;
/**
* optional .PlainTokenAction plain_action = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction getPlainAction() {
if (plainActionBuilder_ == null) {
if (actionCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
} else {
if (actionCase_ == 1) {
return plainActionBuilder_.getMessage();
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
}
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public Builder setPlainAction(org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction value) {
if (plainActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
plainActionBuilder_.setMessage(value);
}
actionCase_ = 1;
return this;
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public Builder setPlainAction(
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder builderForValue) {
if (plainActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
plainActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 1;
return this;
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public Builder mergePlainAction(org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction value) {
if (plainActionBuilder_ == null) {
if (actionCase_ == 1 &&
action_ != org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance()) {
action_ = org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.newBuilder((org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_)
.mergeFrom(value).buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 1) {
plainActionBuilder_.mergeFrom(value);
}
plainActionBuilder_.setMessage(value);
}
actionCase_ = 1;
return this;
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public Builder clearPlainAction() {
if (plainActionBuilder_ == null) {
if (actionCase_ == 1) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 1) {
actionCase_ = 0;
action_ = null;
}
plainActionBuilder_.clear();
}
return this;
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder getPlainActionBuilder() {
return getPlainActionFieldBuilder().getBuilder();
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder getPlainActionOrBuilder() {
if ((actionCase_ == 1) && (plainActionBuilder_ != null)) {
return plainActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
}
}
/**
* optional .PlainTokenAction plain_action = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction, org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder>
getPlainActionFieldBuilder() {
if (plainActionBuilder_ == null) {
if (!(actionCase_ == 1)) {
action_ = org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
}
plainActionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction, org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder>(
(org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 1;
onChanged();;
return plainActionBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:TokenTransaction)
}
// @@protoc_insertion_point(class_scope:TokenTransaction)
private static final org.hyperledger.fabric.protos.token.Transaction.TokenTransaction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.TokenTransaction();
}
public static org.hyperledger.fabric.protos.token.Transaction.TokenTransaction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TokenTransaction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TokenTransaction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.TokenTransaction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainTokenActionOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainTokenAction)
com.google.protobuf.MessageOrBuilder {
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainImport getPlainImport();
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder getPlainImportOrBuilder();
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getPlainTransfer();
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder getPlainTransferOrBuilder();
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getPlainRedeem();
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder getPlainRedeemOrBuilder();
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainApprove getPlainApprove();
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder getPlainApproveOrBuilder();
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom getPlainTransferFrom();
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder getPlainTransferFromOrBuilder();
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.DataCase getDataCase();
}
/**
*
* PlainTokenAction governs the structure of a token action that is
* subjected to no privacy restrictions
*
*
* Protobuf type {@code PlainTokenAction}
*/
public static final class PlainTokenAction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainTokenAction)
PlainTokenActionOrBuilder {
// Use PlainTokenAction.newBuilder() to construct.
private PlainTokenAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainTokenAction() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainTokenAction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder subBuilder = null;
if (dataCase_ == 1) {
subBuilder = ((org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_).toBuilder();
}
data_ =
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainImport.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 1;
break;
}
case 18: {
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder subBuilder = null;
if (dataCase_ == 2) {
subBuilder = ((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_).toBuilder();
}
data_ =
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 2;
break;
}
case 26: {
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder subBuilder = null;
if (dataCase_ == 3) {
subBuilder = ((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_).toBuilder();
}
data_ =
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 3;
break;
}
case 34: {
org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder subBuilder = null;
if (dataCase_ == 4) {
subBuilder = ((org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_).toBuilder();
}
data_ =
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainApprove.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 4;
break;
}
case 42: {
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder subBuilder = null;
if (dataCase_ == 5) {
subBuilder = ((org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_).toBuilder();
}
data_ =
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 5;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTokenAction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTokenAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.class, org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder.class);
}
private int dataCase_ = 0;
private java.lang.Object data_;
public enum DataCase
implements com.google.protobuf.Internal.EnumLite {
PLAIN_IMPORT(1),
PLAIN_TRANSFER(2),
PLAIN_REDEEM(3),
PLAIN_APPROVE(4),
PLAIN_TRANSFER_FROM(5),
DATA_NOT_SET(0);
private final int value;
private DataCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DataCase valueOf(int value) {
return forNumber(value);
}
public static DataCase forNumber(int value) {
switch (value) {
case 1: return PLAIN_IMPORT;
case 2: return PLAIN_TRANSFER;
case 3: return PLAIN_REDEEM;
case 4: return PLAIN_APPROVE;
case 5: return PLAIN_TRANSFER_FROM;
case 0: return DATA_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
public static final int PLAIN_IMPORT_FIELD_NUMBER = 1;
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainImport getPlainImport() {
if (dataCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder getPlainImportOrBuilder() {
if (dataCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
}
public static final int PLAIN_TRANSFER_FIELD_NUMBER = 2;
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getPlainTransfer() {
if (dataCase_ == 2) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder getPlainTransferOrBuilder() {
if (dataCase_ == 2) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
public static final int PLAIN_REDEEM_FIELD_NUMBER = 3;
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getPlainRedeem() {
if (dataCase_ == 3) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder getPlainRedeemOrBuilder() {
if (dataCase_ == 3) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
public static final int PLAIN_APPROVE_FIELD_NUMBER = 4;
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove getPlainApprove() {
if (dataCase_ == 4) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder getPlainApproveOrBuilder() {
if (dataCase_ == 4) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
}
public static final int PLAIN_TRANSFER_FROM_FIELD_NUMBER = 5;
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom getPlainTransferFrom() {
if (dataCase_ == 5) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder getPlainTransferFromOrBuilder() {
if (dataCase_ == 5) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (dataCase_ == 1) {
output.writeMessage(1, (org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_);
}
if (dataCase_ == 2) {
output.writeMessage(2, (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_);
}
if (dataCase_ == 3) {
output.writeMessage(3, (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_);
}
if (dataCase_ == 4) {
output.writeMessage(4, (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_);
}
if (dataCase_ == 5) {
output.writeMessage(5, (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (dataCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_);
}
if (dataCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_);
}
if (dataCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_);
}
if (dataCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_);
}
if (dataCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction other = (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) obj;
boolean result = true;
result = result && getDataCase().equals(
other.getDataCase());
if (!result) return false;
switch (dataCase_) {
case 1:
result = result && getPlainImport()
.equals(other.getPlainImport());
break;
case 2:
result = result && getPlainTransfer()
.equals(other.getPlainTransfer());
break;
case 3:
result = result && getPlainRedeem()
.equals(other.getPlainRedeem());
break;
case 4:
result = result && getPlainApprove()
.equals(other.getPlainApprove());
break;
case 5:
result = result && getPlainTransferFrom()
.equals(other.getPlainTransferFrom());
break;
case 0:
default:
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
switch (dataCase_) {
case 1:
hash = (37 * hash) + PLAIN_IMPORT_FIELD_NUMBER;
hash = (53 * hash) + getPlainImport().hashCode();
break;
case 2:
hash = (37 * hash) + PLAIN_TRANSFER_FIELD_NUMBER;
hash = (53 * hash) + getPlainTransfer().hashCode();
break;
case 3:
hash = (37 * hash) + PLAIN_REDEEM_FIELD_NUMBER;
hash = (53 * hash) + getPlainRedeem().hashCode();
break;
case 4:
hash = (37 * hash) + PLAIN_APPROVE_FIELD_NUMBER;
hash = (53 * hash) + getPlainApprove().hashCode();
break;
case 5:
hash = (37 * hash) + PLAIN_TRANSFER_FROM_FIELD_NUMBER;
hash = (53 * hash) + getPlainTransferFrom().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* PlainTokenAction governs the structure of a token action that is
* subjected to no privacy restrictions
*
*
* Protobuf type {@code PlainTokenAction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainTokenAction)
org.hyperledger.fabric.protos.token.Transaction.PlainTokenActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTokenAction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTokenAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.class, org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
dataCase_ = 0;
data_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTokenAction_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction build() {
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction result = new org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction(this);
if (dataCase_ == 1) {
if (plainImportBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = plainImportBuilder_.build();
}
}
if (dataCase_ == 2) {
if (plainTransferBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = plainTransferBuilder_.build();
}
}
if (dataCase_ == 3) {
if (plainRedeemBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = plainRedeemBuilder_.build();
}
}
if (dataCase_ == 4) {
if (plainApproveBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = plainApproveBuilder_.build();
}
}
if (dataCase_ == 5) {
if (plainTransferFromBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = plainTransferFromBuilder_.build();
}
}
result.dataCase_ = dataCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction.getDefaultInstance()) return this;
switch (other.getDataCase()) {
case PLAIN_IMPORT: {
mergePlainImport(other.getPlainImport());
break;
}
case PLAIN_TRANSFER: {
mergePlainTransfer(other.getPlainTransfer());
break;
}
case PLAIN_REDEEM: {
mergePlainRedeem(other.getPlainRedeem());
break;
}
case PLAIN_APPROVE: {
mergePlainApprove(other.getPlainApprove());
break;
}
case PLAIN_TRANSFER_FROM: {
mergePlainTransferFrom(other.getPlainTransferFrom());
break;
}
case DATA_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int dataCase_ = 0;
private java.lang.Object data_;
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
public Builder clearData() {
dataCase_ = 0;
data_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainImport, org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder> plainImportBuilder_;
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainImport getPlainImport() {
if (plainImportBuilder_ == null) {
if (dataCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
} else {
if (dataCase_ == 1) {
return plainImportBuilder_.getMessage();
}
return org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
}
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public Builder setPlainImport(org.hyperledger.fabric.protos.token.Transaction.PlainImport value) {
if (plainImportBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
plainImportBuilder_.setMessage(value);
}
dataCase_ = 1;
return this;
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public Builder setPlainImport(
org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder builderForValue) {
if (plainImportBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
plainImportBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 1;
return this;
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public Builder mergePlainImport(org.hyperledger.fabric.protos.token.Transaction.PlainImport value) {
if (plainImportBuilder_ == null) {
if (dataCase_ == 1 &&
data_ != org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance()) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainImport.newBuilder((org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 1) {
plainImportBuilder_.mergeFrom(value);
}
plainImportBuilder_.setMessage(value);
}
dataCase_ = 1;
return this;
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public Builder clearPlainImport() {
if (plainImportBuilder_ == null) {
if (dataCase_ == 1) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 1) {
dataCase_ = 0;
data_ = null;
}
plainImportBuilder_.clear();
}
return this;
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder getPlainImportBuilder() {
return getPlainImportFieldBuilder().getBuilder();
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder getPlainImportOrBuilder() {
if ((dataCase_ == 1) && (plainImportBuilder_ != null)) {
return plainImportBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 1) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
}
}
/**
*
* A plaintext token import transaction
*
*
* optional .PlainImport plain_import = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainImport, org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder>
getPlainImportFieldBuilder() {
if (plainImportBuilder_ == null) {
if (!(dataCase_ == 1)) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
}
plainImportBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainImport, org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder>(
(org.hyperledger.fabric.protos.token.Transaction.PlainImport) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 1;
onChanged();;
return plainImportBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder> plainTransferBuilder_;
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getPlainTransfer() {
if (plainTransferBuilder_ == null) {
if (dataCase_ == 2) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
} else {
if (dataCase_ == 2) {
return plainTransferBuilder_.getMessage();
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public Builder setPlainTransfer(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer value) {
if (plainTransferBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
plainTransferBuilder_.setMessage(value);
}
dataCase_ = 2;
return this;
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public Builder setPlainTransfer(
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder builderForValue) {
if (plainTransferBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
plainTransferBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 2;
return this;
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public Builder mergePlainTransfer(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer value) {
if (plainTransferBuilder_ == null) {
if (dataCase_ == 2 &&
data_ != org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance()) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.newBuilder((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 2) {
plainTransferBuilder_.mergeFrom(value);
}
plainTransferBuilder_.setMessage(value);
}
dataCase_ = 2;
return this;
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public Builder clearPlainTransfer() {
if (plainTransferBuilder_ == null) {
if (dataCase_ == 2) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 2) {
dataCase_ = 0;
data_ = null;
}
plainTransferBuilder_.clear();
}
return this;
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder getPlainTransferBuilder() {
return getPlainTransferFieldBuilder().getBuilder();
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder getPlainTransferOrBuilder() {
if ((dataCase_ == 2) && (plainTransferBuilder_ != null)) {
return plainTransferBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 2) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
}
/**
*
* A plaintext token transfer transaction
*
*
* optional .PlainTransfer plain_transfer = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder>
getPlainTransferFieldBuilder() {
if (plainTransferBuilder_ == null) {
if (!(dataCase_ == 2)) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
plainTransferBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder>(
(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 2;
onChanged();;
return plainTransferBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder> plainRedeemBuilder_;
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getPlainRedeem() {
if (plainRedeemBuilder_ == null) {
if (dataCase_ == 3) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
} else {
if (dataCase_ == 3) {
return plainRedeemBuilder_.getMessage();
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public Builder setPlainRedeem(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer value) {
if (plainRedeemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
plainRedeemBuilder_.setMessage(value);
}
dataCase_ = 3;
return this;
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public Builder setPlainRedeem(
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder builderForValue) {
if (plainRedeemBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
plainRedeemBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 3;
return this;
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public Builder mergePlainRedeem(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer value) {
if (plainRedeemBuilder_ == null) {
if (dataCase_ == 3 &&
data_ != org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance()) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.newBuilder((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 3) {
plainRedeemBuilder_.mergeFrom(value);
}
plainRedeemBuilder_.setMessage(value);
}
dataCase_ = 3;
return this;
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public Builder clearPlainRedeem() {
if (plainRedeemBuilder_ == null) {
if (dataCase_ == 3) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 3) {
dataCase_ = 0;
data_ = null;
}
plainRedeemBuilder_.clear();
}
return this;
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder getPlainRedeemBuilder() {
return getPlainRedeemFieldBuilder().getBuilder();
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder getPlainRedeemOrBuilder() {
if ((dataCase_ == 3) && (plainRedeemBuilder_ != null)) {
return plainRedeemBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 3) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
}
/**
*
* A plaintext token redeem transaction
*
*
* optional .PlainTransfer plain_redeem = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder>
getPlainRedeemFieldBuilder() {
if (plainRedeemBuilder_ == null) {
if (!(dataCase_ == 3)) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
plainRedeemBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder>(
(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 3;
onChanged();;
return plainRedeemBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainApprove, org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder> plainApproveBuilder_;
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove getPlainApprove() {
if (plainApproveBuilder_ == null) {
if (dataCase_ == 4) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
} else {
if (dataCase_ == 4) {
return plainApproveBuilder_.getMessage();
}
return org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
}
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public Builder setPlainApprove(org.hyperledger.fabric.protos.token.Transaction.PlainApprove value) {
if (plainApproveBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
plainApproveBuilder_.setMessage(value);
}
dataCase_ = 4;
return this;
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public Builder setPlainApprove(
org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder builderForValue) {
if (plainApproveBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
plainApproveBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 4;
return this;
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public Builder mergePlainApprove(org.hyperledger.fabric.protos.token.Transaction.PlainApprove value) {
if (plainApproveBuilder_ == null) {
if (dataCase_ == 4 &&
data_ != org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance()) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainApprove.newBuilder((org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 4) {
plainApproveBuilder_.mergeFrom(value);
}
plainApproveBuilder_.setMessage(value);
}
dataCase_ = 4;
return this;
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public Builder clearPlainApprove() {
if (plainApproveBuilder_ == null) {
if (dataCase_ == 4) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 4) {
dataCase_ = 0;
data_ = null;
}
plainApproveBuilder_.clear();
}
return this;
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder getPlainApproveBuilder() {
return getPlainApproveFieldBuilder().getBuilder();
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder getPlainApproveOrBuilder() {
if ((dataCase_ == 4) && (plainApproveBuilder_ != null)) {
return plainApproveBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 4) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
}
}
/**
*
* A plaintext token approve transaction
*
*
* optional .PlainApprove plain_approve = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainApprove, org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder>
getPlainApproveFieldBuilder() {
if (plainApproveBuilder_ == null) {
if (!(dataCase_ == 4)) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
}
plainApproveBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainApprove, org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder>(
(org.hyperledger.fabric.protos.token.Transaction.PlainApprove) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 4;
onChanged();;
return plainApproveBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder> plainTransferFromBuilder_;
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom getPlainTransferFrom() {
if (plainTransferFromBuilder_ == null) {
if (dataCase_ == 5) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
} else {
if (dataCase_ == 5) {
return plainTransferFromBuilder_.getMessage();
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
}
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public Builder setPlainTransferFrom(org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom value) {
if (plainTransferFromBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
plainTransferFromBuilder_.setMessage(value);
}
dataCase_ = 5;
return this;
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public Builder setPlainTransferFrom(
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder builderForValue) {
if (plainTransferFromBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
plainTransferFromBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 5;
return this;
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public Builder mergePlainTransferFrom(org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom value) {
if (plainTransferFromBuilder_ == null) {
if (dataCase_ == 5 &&
data_ != org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance()) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.newBuilder((org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 5) {
plainTransferFromBuilder_.mergeFrom(value);
}
plainTransferFromBuilder_.setMessage(value);
}
dataCase_ = 5;
return this;
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public Builder clearPlainTransferFrom() {
if (plainTransferFromBuilder_ == null) {
if (dataCase_ == 5) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 5) {
dataCase_ = 0;
data_ = null;
}
plainTransferFromBuilder_.clear();
}
return this;
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder getPlainTransferFromBuilder() {
return getPlainTransferFromFieldBuilder().getBuilder();
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder getPlainTransferFromOrBuilder() {
if ((dataCase_ == 5) && (plainTransferFromBuilder_ != null)) {
return plainTransferFromBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 5) {
return (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_;
}
return org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
}
}
/**
*
* A plaintext token transfer from transaction
*
*
* optional .PlainTransferFrom plain_transfer_From = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder>
getPlainTransferFromFieldBuilder() {
if (plainTransferFromBuilder_ == null) {
if (!(dataCase_ == 5)) {
data_ = org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
}
plainTransferFromBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder>(
(org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 5;
onChanged();;
return plainTransferFromBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainTokenAction)
}
// @@protoc_insertion_point(class_scope:PlainTokenAction)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainTokenAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainTokenAction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTokenAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainImportOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainImport)
com.google.protobuf.MessageOrBuilder {
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
java.util.List
getOutputsList();
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index);
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
int getOutputsCount();
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList();
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index);
}
/**
*
* PlainImport specifies an import of one or more tokens in plaintext format
*
*
* Protobuf type {@code PlainImport}
*/
public static final class PlainImport extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainImport)
PlainImportOrBuilder {
// Use PlainImport.newBuilder() to construct.
private PlainImport(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainImport() {
outputs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainImport(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
outputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
outputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainOutput.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainImport_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainImport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainImport.class, org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder.class);
}
public static final int OUTPUTS_FIELD_NUMBER = 1;
private java.util.List outputs_;
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public java.util.List getOutputsList() {
return outputs_;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList() {
return outputs_;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public int getOutputsCount() {
return outputs_.size();
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index) {
return outputs_.get(index);
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index) {
return outputs_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < outputs_.size(); i++) {
output.writeMessage(1, outputs_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < outputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, outputs_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainImport)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainImport other = (org.hyperledger.fabric.protos.token.Transaction.PlainImport) obj;
boolean result = true;
result = result && getOutputsList()
.equals(other.getOutputsList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getOutputsCount() > 0) {
hash = (37 * hash) + OUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getOutputsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainImport prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* PlainImport specifies an import of one or more tokens in plaintext format
*
*
* Protobuf type {@code PlainImport}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainImport)
org.hyperledger.fabric.protos.token.Transaction.PlainImportOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainImport_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainImport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainImport.class, org.hyperledger.fabric.protos.token.Transaction.PlainImport.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainImport.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOutputsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
outputsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainImport_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainImport getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainImport build() {
org.hyperledger.fabric.protos.token.Transaction.PlainImport result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainImport buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainImport result = new org.hyperledger.fabric.protos.token.Transaction.PlainImport(this);
int from_bitField0_ = bitField0_;
if (outputsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.outputs_ = outputs_;
} else {
result.outputs_ = outputsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainImport) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainImport)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainImport other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainImport.getDefaultInstance()) return this;
if (outputsBuilder_ == null) {
if (!other.outputs_.isEmpty()) {
if (outputs_.isEmpty()) {
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureOutputsIsMutable();
outputs_.addAll(other.outputs_);
}
onChanged();
}
} else {
if (!other.outputs_.isEmpty()) {
if (outputsBuilder_.isEmpty()) {
outputsBuilder_.dispose();
outputsBuilder_ = null;
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000001);
outputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputsFieldBuilder() : null;
} else {
outputsBuilder_.addAllMessages(other.outputs_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainImport parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainImport) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List outputs_ =
java.util.Collections.emptyList();
private void ensureOutputsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
outputs_ = new java.util.ArrayList(outputs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder> outputsBuilder_;
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public java.util.List getOutputsList() {
if (outputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputs_);
} else {
return outputsBuilder_.getMessageList();
}
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public int getOutputsCount() {
if (outputsBuilder_ == null) {
return outputs_.size();
} else {
return outputsBuilder_.getCount();
}
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index);
} else {
return outputsBuilder_.getMessage(index);
}
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder setOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.set(index, value);
onChanged();
} else {
outputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder setOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.set(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder addOutputs(org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(value);
onChanged();
} else {
outputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder addOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(index, value);
onChanged();
} else {
outputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder addOutputs(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder addOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder addAllOutputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.PlainOutput> values) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputs_);
onChanged();
} else {
outputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder clearOutputs() {
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
outputsBuilder_.clear();
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public Builder removeOutputs(int index) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.remove(index);
onChanged();
} else {
outputsBuilder_.remove(index);
}
return this;
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder getOutputsBuilder(
int index) {
return getOutputsFieldBuilder().getBuilder(index);
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index); } else {
return outputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList() {
if (outputsBuilder_ != null) {
return outputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputs_);
}
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder addOutputsBuilder() {
return getOutputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance());
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder addOutputsBuilder(
int index) {
return getOutputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance());
}
/**
*
* An import transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 1;
*/
public java.util.List
getOutputsBuilderList() {
return getOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsFieldBuilder() {
if (outputsBuilder_ == null) {
outputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>(
outputs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
outputs_ = null;
}
return outputsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainImport)
}
// @@protoc_insertion_point(class_scope:PlainImport)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainImport DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainImport();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainImport getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainImport parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainImport(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainImport getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainTransferOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainTransfer)
com.google.protobuf.MessageOrBuilder {
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
java.util.List
getInputsList();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index);
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
int getInputsCount();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index);
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
java.util.List
getOutputsList();
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index);
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
int getOutputsCount();
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList();
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index);
}
/**
*
* PlainTransfer specifies a transfer of one or more plaintext tokens to one or more outputs
*
*
* Protobuf type {@code PlainTransfer}
*/
public static final class PlainTransfer extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainTransfer)
PlainTransferOrBuilder {
// Use PlainTransfer.newBuilder() to construct.
private PlainTransfer(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainTransfer() {
inputs_ = java.util.Collections.emptyList();
outputs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainTransfer(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
inputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.InputId.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
outputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainOutput.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = java.util.Collections.unmodifiableList(inputs_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransfer_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransfer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.class, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder.class);
}
public static final int INPUTS_FIELD_NUMBER = 1;
private java.util.List inputs_;
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List getInputsList() {
return inputs_;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList() {
return inputs_;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public int getInputsCount() {
return inputs_.size();
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index) {
return inputs_.get(index);
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index) {
return inputs_.get(index);
}
public static final int OUTPUTS_FIELD_NUMBER = 2;
private java.util.List outputs_;
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List getOutputsList() {
return outputs_;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList() {
return outputs_;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public int getOutputsCount() {
return outputs_.size();
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index) {
return outputs_.get(index);
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index) {
return outputs_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < inputs_.size(); i++) {
output.writeMessage(1, inputs_.get(i));
}
for (int i = 0; i < outputs_.size(); i++) {
output.writeMessage(2, outputs_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < inputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, inputs_.get(i));
}
for (int i = 0; i < outputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, outputs_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainTransfer)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer other = (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) obj;
boolean result = true;
result = result && getInputsList()
.equals(other.getInputsList());
result = result && getOutputsList()
.equals(other.getOutputsList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getInputsCount() > 0) {
hash = (37 * hash) + INPUTS_FIELD_NUMBER;
hash = (53 * hash) + getInputsList().hashCode();
}
if (getOutputsCount() > 0) {
hash = (37 * hash) + OUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getOutputsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* PlainTransfer specifies a transfer of one or more plaintext tokens to one or more outputs
*
*
* Protobuf type {@code PlainTransfer}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainTransfer)
org.hyperledger.fabric.protos.token.Transaction.PlainTransferOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransfer_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransfer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.class, org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getInputsFieldBuilder();
getOutputsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (inputsBuilder_ == null) {
inputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
inputsBuilder_.clear();
}
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
outputsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransfer_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer build() {
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer result = new org.hyperledger.fabric.protos.token.Transaction.PlainTransfer(this);
int from_bitField0_ = bitField0_;
if (inputsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = java.util.Collections.unmodifiableList(inputs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.inputs_ = inputs_;
} else {
result.inputs_ = inputsBuilder_.build();
}
if (outputsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.outputs_ = outputs_;
} else {
result.outputs_ = outputsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTransfer)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainTransfer other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainTransfer.getDefaultInstance()) return this;
if (inputsBuilder_ == null) {
if (!other.inputs_.isEmpty()) {
if (inputs_.isEmpty()) {
inputs_ = other.inputs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureInputsIsMutable();
inputs_.addAll(other.inputs_);
}
onChanged();
}
} else {
if (!other.inputs_.isEmpty()) {
if (inputsBuilder_.isEmpty()) {
inputsBuilder_.dispose();
inputsBuilder_ = null;
inputs_ = other.inputs_;
bitField0_ = (bitField0_ & ~0x00000001);
inputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getInputsFieldBuilder() : null;
} else {
inputsBuilder_.addAllMessages(other.inputs_);
}
}
}
if (outputsBuilder_ == null) {
if (!other.outputs_.isEmpty()) {
if (outputs_.isEmpty()) {
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureOutputsIsMutable();
outputs_.addAll(other.outputs_);
}
onChanged();
}
} else {
if (!other.outputs_.isEmpty()) {
if (outputsBuilder_.isEmpty()) {
outputsBuilder_.dispose();
outputsBuilder_ = null;
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000002);
outputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputsFieldBuilder() : null;
} else {
outputsBuilder_.addAllMessages(other.outputs_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainTransfer parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainTransfer) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List inputs_ =
java.util.Collections.emptyList();
private void ensureInputsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = new java.util.ArrayList(inputs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder> inputsBuilder_;
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List getInputsList() {
if (inputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(inputs_);
} else {
return inputsBuilder_.getMessageList();
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public int getInputsCount() {
if (inputsBuilder_ == null) {
return inputs_.size();
} else {
return inputsBuilder_.getCount();
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index) {
if (inputsBuilder_ == null) {
return inputs_.get(index);
} else {
return inputsBuilder_.getMessage(index);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder setInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.set(index, value);
onChanged();
} else {
inputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder setInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.set(index, builderForValue.build());
onChanged();
} else {
inputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.add(value);
onChanged();
} else {
inputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.add(index, value);
onChanged();
} else {
inputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.add(builderForValue.build());
onChanged();
} else {
inputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.add(index, builderForValue.build());
onChanged();
} else {
inputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addAllInputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.InputId> values) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, inputs_);
onChanged();
} else {
inputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder clearInputs() {
if (inputsBuilder_ == null) {
inputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
inputsBuilder_.clear();
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder removeInputs(int index) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.remove(index);
onChanged();
} else {
inputsBuilder_.remove(index);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder getInputsBuilder(
int index) {
return getInputsFieldBuilder().getBuilder(index);
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index) {
if (inputsBuilder_ == null) {
return inputs_.get(index); } else {
return inputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList() {
if (inputsBuilder_ != null) {
return inputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(inputs_);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder addInputsBuilder() {
return getInputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance());
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder addInputsBuilder(
int index) {
return getInputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance());
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List
getInputsBuilderList() {
return getInputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsFieldBuilder() {
if (inputsBuilder_ == null) {
inputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>(
inputs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
inputs_ = null;
}
return inputsBuilder_;
}
private java.util.List outputs_ =
java.util.Collections.emptyList();
private void ensureOutputsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = new java.util.ArrayList(outputs_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder> outputsBuilder_;
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List getOutputsList() {
if (outputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputs_);
} else {
return outputsBuilder_.getMessageList();
}
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public int getOutputsCount() {
if (outputsBuilder_ == null) {
return outputs_.size();
} else {
return outputsBuilder_.getCount();
}
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index);
} else {
return outputsBuilder_.getMessage(index);
}
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder setOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.set(index, value);
onChanged();
} else {
outputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder setOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.set(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(value);
onChanged();
} else {
outputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(index, value);
onChanged();
} else {
outputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addAllOutputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.PlainOutput> values) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputs_);
onChanged();
} else {
outputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder clearOutputs() {
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
outputsBuilder_.clear();
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder removeOutputs(int index) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.remove(index);
onChanged();
} else {
outputsBuilder_.remove(index);
}
return this;
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder getOutputsBuilder(
int index) {
return getOutputsFieldBuilder().getBuilder(index);
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index); } else {
return outputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList() {
if (outputsBuilder_ != null) {
return outputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputs_);
}
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder addOutputsBuilder() {
return getOutputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance());
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder addOutputsBuilder(
int index) {
return getOutputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance());
}
/**
*
* A transfer transaction may contain one or more outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List
getOutputsBuilderList() {
return getOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsFieldBuilder() {
if (outputsBuilder_ == null) {
outputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>(
outputs_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
outputs_ = null;
}
return outputsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainTransfer)
}
// @@protoc_insertion_point(class_scope:PlainTransfer)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainTransfer DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainTransfer();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainTransfer parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainTransfer(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransfer getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainApproveOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainApprove)
com.google.protobuf.MessageOrBuilder {
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
java.util.List
getInputsList();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index);
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
int getInputsCount();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index);
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
java.util.List
getDelegatedOutputsList();
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDelegatedOutputs(int index);
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
int getDelegatedOutputsCount();
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>
getDelegatedOutputsOrBuilderList();
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder getDelegatedOutputsOrBuilder(
int index);
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
boolean hasOutput();
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutput();
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputOrBuilder();
}
/**
*
* PlainApprove specifies an approve of one or more tokens in plaintext format
*
*
* Protobuf type {@code PlainApprove}
*/
public static final class PlainApprove extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainApprove)
PlainApproveOrBuilder {
// Use PlainApprove.newBuilder() to construct.
private PlainApprove(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainApprove() {
inputs_ = java.util.Collections.emptyList();
delegatedOutputs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainApprove(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
inputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.InputId.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
delegatedOutputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
delegatedOutputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.parser(), extensionRegistry));
break;
}
case 26: {
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder subBuilder = null;
if (output_ != null) {
subBuilder = output_.toBuilder();
}
output_ = input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainOutput.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(output_);
output_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = java.util.Collections.unmodifiableList(inputs_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
delegatedOutputs_ = java.util.Collections.unmodifiableList(delegatedOutputs_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainApprove_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainApprove_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainApprove.class, org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder.class);
}
private int bitField0_;
public static final int INPUTS_FIELD_NUMBER = 1;
private java.util.List inputs_;
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List getInputsList() {
return inputs_;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList() {
return inputs_;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public int getInputsCount() {
return inputs_.size();
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index) {
return inputs_.get(index);
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index) {
return inputs_.get(index);
}
public static final int DELEGATED_OUTPUTS_FIELD_NUMBER = 2;
private java.util.List delegatedOutputs_;
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public java.util.List getDelegatedOutputsList() {
return delegatedOutputs_;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>
getDelegatedOutputsOrBuilderList() {
return delegatedOutputs_;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public int getDelegatedOutputsCount() {
return delegatedOutputs_.size();
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDelegatedOutputs(int index) {
return delegatedOutputs_.get(index);
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder getDelegatedOutputsOrBuilder(
int index) {
return delegatedOutputs_.get(index);
}
public static final int OUTPUT_FIELD_NUMBER = 3;
private org.hyperledger.fabric.protos.token.Transaction.PlainOutput output_;
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public boolean hasOutput() {
return output_ != null;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutput() {
return output_ == null ? org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance() : output_;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputOrBuilder() {
return getOutput();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < inputs_.size(); i++) {
output.writeMessage(1, inputs_.get(i));
}
for (int i = 0; i < delegatedOutputs_.size(); i++) {
output.writeMessage(2, delegatedOutputs_.get(i));
}
if (output_ != null) {
output.writeMessage(3, getOutput());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < inputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, inputs_.get(i));
}
for (int i = 0; i < delegatedOutputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, delegatedOutputs_.get(i));
}
if (output_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getOutput());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainApprove)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainApprove other = (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) obj;
boolean result = true;
result = result && getInputsList()
.equals(other.getInputsList());
result = result && getDelegatedOutputsList()
.equals(other.getDelegatedOutputsList());
result = result && (hasOutput() == other.hasOutput());
if (hasOutput()) {
result = result && getOutput()
.equals(other.getOutput());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getInputsCount() > 0) {
hash = (37 * hash) + INPUTS_FIELD_NUMBER;
hash = (53 * hash) + getInputsList().hashCode();
}
if (getDelegatedOutputsCount() > 0) {
hash = (37 * hash) + DELEGATED_OUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatedOutputsList().hashCode();
}
if (hasOutput()) {
hash = (37 * hash) + OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + getOutput().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainApprove prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* PlainApprove specifies an approve of one or more tokens in plaintext format
*
*
* Protobuf type {@code PlainApprove}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainApprove)
org.hyperledger.fabric.protos.token.Transaction.PlainApproveOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainApprove_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainApprove_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainApprove.class, org.hyperledger.fabric.protos.token.Transaction.PlainApprove.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainApprove.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getInputsFieldBuilder();
getDelegatedOutputsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (inputsBuilder_ == null) {
inputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
inputsBuilder_.clear();
}
if (delegatedOutputsBuilder_ == null) {
delegatedOutputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
delegatedOutputsBuilder_.clear();
}
if (outputBuilder_ == null) {
output_ = null;
} else {
output_ = null;
outputBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainApprove_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove build() {
org.hyperledger.fabric.protos.token.Transaction.PlainApprove result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainApprove result = new org.hyperledger.fabric.protos.token.Transaction.PlainApprove(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (inputsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = java.util.Collections.unmodifiableList(inputs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.inputs_ = inputs_;
} else {
result.inputs_ = inputsBuilder_.build();
}
if (delegatedOutputsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
delegatedOutputs_ = java.util.Collections.unmodifiableList(delegatedOutputs_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.delegatedOutputs_ = delegatedOutputs_;
} else {
result.delegatedOutputs_ = delegatedOutputsBuilder_.build();
}
if (outputBuilder_ == null) {
result.output_ = output_;
} else {
result.output_ = outputBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainApprove) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainApprove)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainApprove other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainApprove.getDefaultInstance()) return this;
if (inputsBuilder_ == null) {
if (!other.inputs_.isEmpty()) {
if (inputs_.isEmpty()) {
inputs_ = other.inputs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureInputsIsMutable();
inputs_.addAll(other.inputs_);
}
onChanged();
}
} else {
if (!other.inputs_.isEmpty()) {
if (inputsBuilder_.isEmpty()) {
inputsBuilder_.dispose();
inputsBuilder_ = null;
inputs_ = other.inputs_;
bitField0_ = (bitField0_ & ~0x00000001);
inputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getInputsFieldBuilder() : null;
} else {
inputsBuilder_.addAllMessages(other.inputs_);
}
}
}
if (delegatedOutputsBuilder_ == null) {
if (!other.delegatedOutputs_.isEmpty()) {
if (delegatedOutputs_.isEmpty()) {
delegatedOutputs_ = other.delegatedOutputs_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.addAll(other.delegatedOutputs_);
}
onChanged();
}
} else {
if (!other.delegatedOutputs_.isEmpty()) {
if (delegatedOutputsBuilder_.isEmpty()) {
delegatedOutputsBuilder_.dispose();
delegatedOutputsBuilder_ = null;
delegatedOutputs_ = other.delegatedOutputs_;
bitField0_ = (bitField0_ & ~0x00000002);
delegatedOutputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDelegatedOutputsFieldBuilder() : null;
} else {
delegatedOutputsBuilder_.addAllMessages(other.delegatedOutputs_);
}
}
}
if (other.hasOutput()) {
mergeOutput(other.getOutput());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainApprove parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainApprove) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List inputs_ =
java.util.Collections.emptyList();
private void ensureInputsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = new java.util.ArrayList(inputs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder> inputsBuilder_;
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List getInputsList() {
if (inputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(inputs_);
} else {
return inputsBuilder_.getMessageList();
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public int getInputsCount() {
if (inputsBuilder_ == null) {
return inputs_.size();
} else {
return inputsBuilder_.getCount();
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index) {
if (inputsBuilder_ == null) {
return inputs_.get(index);
} else {
return inputsBuilder_.getMessage(index);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder setInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.set(index, value);
onChanged();
} else {
inputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder setInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.set(index, builderForValue.build());
onChanged();
} else {
inputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.add(value);
onChanged();
} else {
inputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.add(index, value);
onChanged();
} else {
inputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.add(builderForValue.build());
onChanged();
} else {
inputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.add(index, builderForValue.build());
onChanged();
} else {
inputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addAllInputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.InputId> values) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, inputs_);
onChanged();
} else {
inputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder clearInputs() {
if (inputsBuilder_ == null) {
inputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
inputsBuilder_.clear();
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder removeInputs(int index) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.remove(index);
onChanged();
} else {
inputsBuilder_.remove(index);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder getInputsBuilder(
int index) {
return getInputsFieldBuilder().getBuilder(index);
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index) {
if (inputsBuilder_ == null) {
return inputs_.get(index); } else {
return inputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList() {
if (inputsBuilder_ != null) {
return inputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(inputs_);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder addInputsBuilder() {
return getInputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance());
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder addInputsBuilder(
int index) {
return getInputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance());
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List
getInputsBuilderList() {
return getInputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsFieldBuilder() {
if (inputsBuilder_ == null) {
inputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>(
inputs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
inputs_ = null;
}
return inputsBuilder_;
}
private java.util.List delegatedOutputs_ =
java.util.Collections.emptyList();
private void ensureDelegatedOutputsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
delegatedOutputs_ = new java.util.ArrayList(delegatedOutputs_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder> delegatedOutputsBuilder_;
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public java.util.List getDelegatedOutputsList() {
if (delegatedOutputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(delegatedOutputs_);
} else {
return delegatedOutputsBuilder_.getMessageList();
}
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public int getDelegatedOutputsCount() {
if (delegatedOutputsBuilder_ == null) {
return delegatedOutputs_.size();
} else {
return delegatedOutputsBuilder_.getCount();
}
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDelegatedOutputs(int index) {
if (delegatedOutputsBuilder_ == null) {
return delegatedOutputs_.get(index);
} else {
return delegatedOutputsBuilder_.getMessage(index);
}
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder setDelegatedOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput value) {
if (delegatedOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.set(index, value);
onChanged();
} else {
delegatedOutputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder setDelegatedOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder builderForValue) {
if (delegatedOutputsBuilder_ == null) {
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.set(index, builderForValue.build());
onChanged();
} else {
delegatedOutputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder addDelegatedOutputs(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput value) {
if (delegatedOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.add(value);
onChanged();
} else {
delegatedOutputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder addDelegatedOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput value) {
if (delegatedOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.add(index, value);
onChanged();
} else {
delegatedOutputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder addDelegatedOutputs(
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder builderForValue) {
if (delegatedOutputsBuilder_ == null) {
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.add(builderForValue.build());
onChanged();
} else {
delegatedOutputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder addDelegatedOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder builderForValue) {
if (delegatedOutputsBuilder_ == null) {
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.add(index, builderForValue.build());
onChanged();
} else {
delegatedOutputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder addAllDelegatedOutputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput> values) {
if (delegatedOutputsBuilder_ == null) {
ensureDelegatedOutputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, delegatedOutputs_);
onChanged();
} else {
delegatedOutputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder clearDelegatedOutputs() {
if (delegatedOutputsBuilder_ == null) {
delegatedOutputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
delegatedOutputsBuilder_.clear();
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public Builder removeDelegatedOutputs(int index) {
if (delegatedOutputsBuilder_ == null) {
ensureDelegatedOutputsIsMutable();
delegatedOutputs_.remove(index);
onChanged();
} else {
delegatedOutputsBuilder_.remove(index);
}
return this;
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder getDelegatedOutputsBuilder(
int index) {
return getDelegatedOutputsFieldBuilder().getBuilder(index);
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder getDelegatedOutputsOrBuilder(
int index) {
if (delegatedOutputsBuilder_ == null) {
return delegatedOutputs_.get(index); } else {
return delegatedOutputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>
getDelegatedOutputsOrBuilderList() {
if (delegatedOutputsBuilder_ != null) {
return delegatedOutputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(delegatedOutputs_);
}
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder addDelegatedOutputsBuilder() {
return getDelegatedOutputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance());
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder addDelegatedOutputsBuilder(
int index) {
return getDelegatedOutputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance());
}
/**
*
* An approve transaction contains one or more plain delegated outputs
*
*
* repeated .PlainDelegatedOutput delegated_outputs = 2;
*/
public java.util.List
getDelegatedOutputsBuilderList() {
return getDelegatedOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>
getDelegatedOutputsFieldBuilder() {
if (delegatedOutputsBuilder_ == null) {
delegatedOutputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>(
delegatedOutputs_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
delegatedOutputs_ = null;
}
return delegatedOutputsBuilder_;
}
private org.hyperledger.fabric.protos.token.Transaction.PlainOutput output_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder> outputBuilder_;
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public boolean hasOutput() {
return outputBuilder_ != null || output_ != null;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutput() {
if (outputBuilder_ == null) {
return output_ == null ? org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance() : output_;
} else {
return outputBuilder_.getMessage();
}
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public Builder setOutput(org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
output_ = value;
onChanged();
} else {
outputBuilder_.setMessage(value);
}
return this;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public Builder setOutput(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputBuilder_ == null) {
output_ = builderForValue.build();
onChanged();
} else {
outputBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public Builder mergeOutput(org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputBuilder_ == null) {
if (output_ != null) {
output_ =
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.newBuilder(output_).mergeFrom(value).buildPartial();
} else {
output_ = value;
}
onChanged();
} else {
outputBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public Builder clearOutput() {
if (outputBuilder_ == null) {
output_ = null;
onChanged();
} else {
output_ = null;
outputBuilder_ = null;
}
return this;
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder getOutputBuilder() {
onChanged();
return getOutputFieldBuilder().getBuilder();
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputOrBuilder() {
if (outputBuilder_ != null) {
return outputBuilder_.getMessageOrBuilder();
} else {
return output_ == null ?
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance() : output_;
}
}
/**
*
* An approve transaction contains one plain output
*
*
* optional .PlainOutput output = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputFieldBuilder() {
if (outputBuilder_ == null) {
outputBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>(
getOutput(),
getParentForChildren(),
isClean());
output_ = null;
}
return outputBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainApprove)
}
// @@protoc_insertion_point(class_scope:PlainApprove)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainApprove DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainApprove();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainApprove getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainApprove parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainApprove(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainApprove getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainTransferFromOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainTransferFrom)
com.google.protobuf.MessageOrBuilder {
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
java.util.List
getInputsList();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index);
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
int getInputsCount();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList();
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index);
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
java.util.List
getOutputsList();
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index);
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
int getOutputsCount();
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList();
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index);
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
boolean hasDelegatedOutput();
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDelegatedOutput();
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder getDelegatedOutputOrBuilder();
}
/**
*
* PlainTransferFrom specifies a transfer of one or more plaintext delegated tokens to one or more outputs
* an to a delegated output
*
*
* Protobuf type {@code PlainTransferFrom}
*/
public static final class PlainTransferFrom extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainTransferFrom)
PlainTransferFromOrBuilder {
// Use PlainTransferFrom.newBuilder() to construct.
private PlainTransferFrom(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainTransferFrom() {
inputs_ = java.util.Collections.emptyList();
outputs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainTransferFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
inputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.InputId.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
outputs_.add(
input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainOutput.parser(), extensionRegistry));
break;
}
case 26: {
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder subBuilder = null;
if (delegatedOutput_ != null) {
subBuilder = delegatedOutput_.toBuilder();
}
delegatedOutput_ = input.readMessage(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(delegatedOutput_);
delegatedOutput_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = java.util.Collections.unmodifiableList(inputs_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransferFrom_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransferFrom_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.class, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder.class);
}
private int bitField0_;
public static final int INPUTS_FIELD_NUMBER = 1;
private java.util.List inputs_;
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List getInputsList() {
return inputs_;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList() {
return inputs_;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public int getInputsCount() {
return inputs_.size();
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index) {
return inputs_.get(index);
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index) {
return inputs_.get(index);
}
public static final int OUTPUTS_FIELD_NUMBER = 2;
private java.util.List outputs_;
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List getOutputsList() {
return outputs_;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList() {
return outputs_;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public int getOutputsCount() {
return outputs_.size();
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index) {
return outputs_.get(index);
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index) {
return outputs_.get(index);
}
public static final int DELEGATED_OUTPUT_FIELD_NUMBER = 3;
private org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput delegatedOutput_;
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public boolean hasDelegatedOutput() {
return delegatedOutput_ != null;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDelegatedOutput() {
return delegatedOutput_ == null ? org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance() : delegatedOutput_;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder getDelegatedOutputOrBuilder() {
return getDelegatedOutput();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < inputs_.size(); i++) {
output.writeMessage(1, inputs_.get(i));
}
for (int i = 0; i < outputs_.size(); i++) {
output.writeMessage(2, outputs_.get(i));
}
if (delegatedOutput_ != null) {
output.writeMessage(3, getDelegatedOutput());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < inputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, inputs_.get(i));
}
for (int i = 0; i < outputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, outputs_.get(i));
}
if (delegatedOutput_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getDelegatedOutput());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom other = (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) obj;
boolean result = true;
result = result && getInputsList()
.equals(other.getInputsList());
result = result && getOutputsList()
.equals(other.getOutputsList());
result = result && (hasDelegatedOutput() == other.hasDelegatedOutput());
if (hasDelegatedOutput()) {
result = result && getDelegatedOutput()
.equals(other.getDelegatedOutput());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getInputsCount() > 0) {
hash = (37 * hash) + INPUTS_FIELD_NUMBER;
hash = (53 * hash) + getInputsList().hashCode();
}
if (getOutputsCount() > 0) {
hash = (37 * hash) + OUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getOutputsList().hashCode();
}
if (hasDelegatedOutput()) {
hash = (37 * hash) + DELEGATED_OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + getDelegatedOutput().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* PlainTransferFrom specifies a transfer of one or more plaintext delegated tokens to one or more outputs
* an to a delegated output
*
*
* Protobuf type {@code PlainTransferFrom}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainTransferFrom)
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFromOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransferFrom_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransferFrom_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.class, org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getInputsFieldBuilder();
getOutputsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (inputsBuilder_ == null) {
inputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
inputsBuilder_.clear();
}
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
outputsBuilder_.clear();
}
if (delegatedOutputBuilder_ == null) {
delegatedOutput_ = null;
} else {
delegatedOutput_ = null;
delegatedOutputBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainTransferFrom_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom build() {
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom result = new org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (inputsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = java.util.Collections.unmodifiableList(inputs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.inputs_ = inputs_;
} else {
result.inputs_ = inputsBuilder_.build();
}
if (outputsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.outputs_ = outputs_;
} else {
result.outputs_ = outputsBuilder_.build();
}
if (delegatedOutputBuilder_ == null) {
result.delegatedOutput_ = delegatedOutput_;
} else {
result.delegatedOutput_ = delegatedOutputBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom.getDefaultInstance()) return this;
if (inputsBuilder_ == null) {
if (!other.inputs_.isEmpty()) {
if (inputs_.isEmpty()) {
inputs_ = other.inputs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureInputsIsMutable();
inputs_.addAll(other.inputs_);
}
onChanged();
}
} else {
if (!other.inputs_.isEmpty()) {
if (inputsBuilder_.isEmpty()) {
inputsBuilder_.dispose();
inputsBuilder_ = null;
inputs_ = other.inputs_;
bitField0_ = (bitField0_ & ~0x00000001);
inputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getInputsFieldBuilder() : null;
} else {
inputsBuilder_.addAllMessages(other.inputs_);
}
}
}
if (outputsBuilder_ == null) {
if (!other.outputs_.isEmpty()) {
if (outputs_.isEmpty()) {
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureOutputsIsMutable();
outputs_.addAll(other.outputs_);
}
onChanged();
}
} else {
if (!other.outputs_.isEmpty()) {
if (outputsBuilder_.isEmpty()) {
outputsBuilder_.dispose();
outputsBuilder_ = null;
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000002);
outputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputsFieldBuilder() : null;
} else {
outputsBuilder_.addAllMessages(other.outputs_);
}
}
}
if (other.hasDelegatedOutput()) {
mergeDelegatedOutput(other.getDelegatedOutput());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List inputs_ =
java.util.Collections.emptyList();
private void ensureInputsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
inputs_ = new java.util.ArrayList(inputs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder> inputsBuilder_;
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List getInputsList() {
if (inputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(inputs_);
} else {
return inputsBuilder_.getMessageList();
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public int getInputsCount() {
if (inputsBuilder_ == null) {
return inputs_.size();
} else {
return inputsBuilder_.getCount();
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId getInputs(int index) {
if (inputsBuilder_ == null) {
return inputs_.get(index);
} else {
return inputsBuilder_.getMessage(index);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder setInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.set(index, value);
onChanged();
} else {
inputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder setInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.set(index, builderForValue.build());
onChanged();
} else {
inputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.add(value);
onChanged();
} else {
inputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId value) {
if (inputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputsIsMutable();
inputs_.add(index, value);
onChanged();
} else {
inputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.add(builderForValue.build());
onChanged();
} else {
inputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addInputs(
int index, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder builderForValue) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.add(index, builderForValue.build());
onChanged();
} else {
inputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder addAllInputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.InputId> values) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, inputs_);
onChanged();
} else {
inputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder clearInputs() {
if (inputsBuilder_ == null) {
inputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
inputsBuilder_.clear();
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public Builder removeInputs(int index) {
if (inputsBuilder_ == null) {
ensureInputsIsMutable();
inputs_.remove(index);
onChanged();
} else {
inputsBuilder_.remove(index);
}
return this;
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder getInputsBuilder(
int index) {
return getInputsFieldBuilder().getBuilder(index);
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder getInputsOrBuilder(
int index) {
if (inputsBuilder_ == null) {
return inputs_.get(index); } else {
return inputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsOrBuilderList() {
if (inputsBuilder_ != null) {
return inputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(inputs_);
}
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder addInputsBuilder() {
return getInputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance());
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public org.hyperledger.fabric.protos.token.Transaction.InputId.Builder addInputsBuilder(
int index) {
return getInputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance());
}
/**
*
* The inputs to the transfer transaction are specified by their ID
*
*
* repeated .InputId inputs = 1;
*/
public java.util.List
getInputsBuilderList() {
return getInputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>
getInputsFieldBuilder() {
if (inputsBuilder_ == null) {
inputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.InputId, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder, org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder>(
inputs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
inputs_ = null;
}
return inputsBuilder_;
}
private java.util.List outputs_ =
java.util.Collections.emptyList();
private void ensureOutputsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
outputs_ = new java.util.ArrayList(outputs_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder> outputsBuilder_;
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List getOutputsList() {
if (outputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputs_);
} else {
return outputsBuilder_.getMessageList();
}
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public int getOutputsCount() {
if (outputsBuilder_ == null) {
return outputs_.size();
} else {
return outputsBuilder_.getCount();
}
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getOutputs(int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index);
} else {
return outputsBuilder_.getMessage(index);
}
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder setOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.set(index, value);
onChanged();
} else {
outputsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder setOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.set(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(value);
onChanged();
} else {
outputsBuilder_.addMessage(value);
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(index, value);
onChanged();
} else {
outputsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addOutputs(
int index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder addAllOutputs(
java.lang.Iterable extends org.hyperledger.fabric.protos.token.Transaction.PlainOutput> values) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputs_);
onChanged();
} else {
outputsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder clearOutputs() {
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
outputsBuilder_.clear();
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public Builder removeOutputs(int index) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.remove(index);
onChanged();
} else {
outputsBuilder_.remove(index);
}
return this;
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder getOutputsBuilder(
int index) {
return getOutputsFieldBuilder().getBuilder(index);
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder getOutputsOrBuilder(
int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index); } else {
return outputsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List extends org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsOrBuilderList() {
if (outputsBuilder_ != null) {
return outputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputs_);
}
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder addOutputsBuilder() {
return getOutputsFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance());
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder addOutputsBuilder(
int index) {
return getOutputsFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance());
}
/**
*
* A transferFrom transaction contains multiple outputs
*
*
* repeated .PlainOutput outputs = 2;
*/
public java.util.List
getOutputsBuilderList() {
return getOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>
getOutputsFieldBuilder() {
if (outputsBuilder_ == null) {
outputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainOutput, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder>(
outputs_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
outputs_ = null;
}
return outputsBuilder_;
}
private org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput delegatedOutput_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder> delegatedOutputBuilder_;
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public boolean hasDelegatedOutput() {
return delegatedOutputBuilder_ != null || delegatedOutput_ != null;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDelegatedOutput() {
if (delegatedOutputBuilder_ == null) {
return delegatedOutput_ == null ? org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance() : delegatedOutput_;
} else {
return delegatedOutputBuilder_.getMessage();
}
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public Builder setDelegatedOutput(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput value) {
if (delegatedOutputBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
delegatedOutput_ = value;
onChanged();
} else {
delegatedOutputBuilder_.setMessage(value);
}
return this;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public Builder setDelegatedOutput(
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder builderForValue) {
if (delegatedOutputBuilder_ == null) {
delegatedOutput_ = builderForValue.build();
onChanged();
} else {
delegatedOutputBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public Builder mergeDelegatedOutput(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput value) {
if (delegatedOutputBuilder_ == null) {
if (delegatedOutput_ != null) {
delegatedOutput_ =
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.newBuilder(delegatedOutput_).mergeFrom(value).buildPartial();
} else {
delegatedOutput_ = value;
}
onChanged();
} else {
delegatedOutputBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public Builder clearDelegatedOutput() {
if (delegatedOutputBuilder_ == null) {
delegatedOutput_ = null;
onChanged();
} else {
delegatedOutput_ = null;
delegatedOutputBuilder_ = null;
}
return this;
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder getDelegatedOutputBuilder() {
onChanged();
return getDelegatedOutputFieldBuilder().getBuilder();
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder getDelegatedOutputOrBuilder() {
if (delegatedOutputBuilder_ != null) {
return delegatedOutputBuilder_.getMessageOrBuilder();
} else {
return delegatedOutput_ == null ?
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance() : delegatedOutput_;
}
}
/**
*
* A transferFrom transaction may contain one delegatable output
*
*
* optional .PlainDelegatedOutput delegated_output = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>
getDelegatedOutputFieldBuilder() {
if (delegatedOutputBuilder_ == null) {
delegatedOutputBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder>(
getDelegatedOutput(),
getParentForChildren(),
isClean());
delegatedOutput_ = null;
}
return delegatedOutputBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainTransferFrom)
}
// @@protoc_insertion_point(class_scope:PlainTransferFrom)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainTransferFrom parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainTransferFrom(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainTransferFrom getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainOutputOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainOutput)
com.google.protobuf.MessageOrBuilder {
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
com.google.protobuf.ByteString getOwner();
/**
*
* The token type
*
*
* optional string type = 2;
*/
java.lang.String getType();
/**
*
* The token type
*
*
* optional string type = 2;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 3;
*/
long getQuantity();
}
/**
*
* A PlainOutput is the result of import and transfer transactions using plaintext tokens
*
*
* Protobuf type {@code PlainOutput}
*/
public static final class PlainOutput extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainOutput)
PlainOutputOrBuilder {
// Use PlainOutput.newBuilder() to construct.
private PlainOutput(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainOutput() {
owner_ = com.google.protobuf.ByteString.EMPTY;
type_ = "";
quantity_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainOutput(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
owner_ = input.readBytes();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
type_ = s;
break;
}
case 24: {
quantity_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainOutput_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.class, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder.class);
}
public static final int OWNER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString owner_;
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public com.google.protobuf.ByteString getOwner() {
return owner_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private volatile java.lang.Object type_;
/**
*
* The token type
*
*
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
* The token type
*
*
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUANTITY_FIELD_NUMBER = 3;
private long quantity_;
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 3;
*/
public long getQuantity() {
return quantity_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!owner_.isEmpty()) {
output.writeBytes(1, owner_);
}
if (!getTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, type_);
}
if (quantity_ != 0L) {
output.writeUInt64(3, quantity_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!owner_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, owner_);
}
if (!getTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, type_);
}
if (quantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, quantity_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainOutput)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainOutput other = (org.hyperledger.fabric.protos.token.Transaction.PlainOutput) obj;
boolean result = true;
result = result && getOwner()
.equals(other.getOwner());
result = result && getType()
.equals(other.getType());
result = result && (getQuantity()
== other.getQuantity());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + OWNER_FIELD_NUMBER;
hash = (53 * hash) + getOwner().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
hash = (37 * hash) + QUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getQuantity());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainOutput prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A PlainOutput is the result of import and transfer transactions using plaintext tokens
*
*
* Protobuf type {@code PlainOutput}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainOutput)
org.hyperledger.fabric.protos.token.Transaction.PlainOutputOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainOutput_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainOutput.class, org.hyperledger.fabric.protos.token.Transaction.PlainOutput.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainOutput.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
owner_ = com.google.protobuf.ByteString.EMPTY;
type_ = "";
quantity_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainOutput_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput build() {
org.hyperledger.fabric.protos.token.Transaction.PlainOutput result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainOutput result = new org.hyperledger.fabric.protos.token.Transaction.PlainOutput(this);
result.owner_ = owner_;
result.type_ = type_;
result.quantity_ = quantity_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainOutput) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainOutput)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainOutput other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainOutput.getDefaultInstance()) return this;
if (other.getOwner() != com.google.protobuf.ByteString.EMPTY) {
setOwner(other.getOwner());
}
if (!other.getType().isEmpty()) {
type_ = other.type_;
onChanged();
}
if (other.getQuantity() != 0L) {
setQuantity(other.getQuantity());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainOutput parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainOutput) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString owner_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public com.google.protobuf.ByteString getOwner() {
return owner_;
}
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public Builder setOwner(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
owner_ = value;
onChanged();
return this;
}
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public Builder clearOwner() {
owner_ = getDefaultInstance().getOwner();
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
*
* The token type
*
*
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The token type
*
*
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The token type
*
*
* optional string type = 2;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
return this;
}
/**
*
* The token type
*
*
* optional string type = 2;
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
*
* The token type
*
*
* optional string type = 2;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
onChanged();
return this;
}
private long quantity_ ;
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 3;
*/
public long getQuantity() {
return quantity_;
}
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 3;
*/
public Builder setQuantity(long value) {
quantity_ = value;
onChanged();
return this;
}
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 3;
*/
public Builder clearQuantity() {
quantity_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainOutput)
}
// @@protoc_insertion_point(class_scope:PlainOutput)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainOutput DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainOutput();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainOutput getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainOutput parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainOutput(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainOutput getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InputIdOrBuilder extends
// @@protoc_insertion_point(interface_extends:InputId)
com.google.protobuf.MessageOrBuilder {
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
java.lang.String getTxId();
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
com.google.protobuf.ByteString
getTxIdBytes();
/**
*
* The index of the output in the transaction
*
*
* optional uint32 index = 2;
*/
int getIndex();
}
/**
*
* An InputId specifies an output using the transaction ID and the index of the output in the transaction
*
*
* Protobuf type {@code InputId}
*/
public static final class InputId extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:InputId)
InputIdOrBuilder {
// Use InputId.newBuilder() to construct.
private InputId(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InputId() {
txId_ = "";
index_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private InputId(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
txId_ = s;
break;
}
case 16: {
index_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_InputId_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_InputId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.InputId.class, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder.class);
}
public static final int TX_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object txId_;
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public java.lang.String getTxId() {
java.lang.Object ref = txId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
txId_ = s;
return s;
}
}
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public com.google.protobuf.ByteString
getTxIdBytes() {
java.lang.Object ref = txId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
txId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INDEX_FIELD_NUMBER = 2;
private int index_;
/**
*
* The index of the output in the transaction
*
*
* optional uint32 index = 2;
*/
public int getIndex() {
return index_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTxIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, txId_);
}
if (index_ != 0) {
output.writeUInt32(2, index_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTxIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, txId_);
}
if (index_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, index_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.InputId)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.InputId other = (org.hyperledger.fabric.protos.token.Transaction.InputId) obj;
boolean result = true;
result = result && getTxId()
.equals(other.getTxId());
result = result && (getIndex()
== other.getIndex());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + TX_ID_FIELD_NUMBER;
hash = (53 * hash) + getTxId().hashCode();
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + getIndex();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.InputId prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An InputId specifies an output using the transaction ID and the index of the output in the transaction
*
*
* Protobuf type {@code InputId}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:InputId)
org.hyperledger.fabric.protos.token.Transaction.InputIdOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_InputId_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_InputId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.InputId.class, org.hyperledger.fabric.protos.token.Transaction.InputId.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.InputId.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
txId_ = "";
index_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_InputId_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.InputId getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.InputId build() {
org.hyperledger.fabric.protos.token.Transaction.InputId result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.InputId buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.InputId result = new org.hyperledger.fabric.protos.token.Transaction.InputId(this);
result.txId_ = txId_;
result.index_ = index_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.InputId) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.InputId)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.InputId other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.InputId.getDefaultInstance()) return this;
if (!other.getTxId().isEmpty()) {
txId_ = other.txId_;
onChanged();
}
if (other.getIndex() != 0) {
setIndex(other.getIndex());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.InputId parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.InputId) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object txId_ = "";
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public java.lang.String getTxId() {
java.lang.Object ref = txId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
txId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public com.google.protobuf.ByteString
getTxIdBytes() {
java.lang.Object ref = txId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
txId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public Builder setTxId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
txId_ = value;
onChanged();
return this;
}
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public Builder clearTxId() {
txId_ = getDefaultInstance().getTxId();
onChanged();
return this;
}
/**
*
* The transaction ID
*
*
* optional string tx_id = 1;
*/
public Builder setTxIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
txId_ = value;
onChanged();
return this;
}
private int index_ ;
/**
*
* The index of the output in the transaction
*
*
* optional uint32 index = 2;
*/
public int getIndex() {
return index_;
}
/**
*
* The index of the output in the transaction
*
*
* optional uint32 index = 2;
*/
public Builder setIndex(int value) {
index_ = value;
onChanged();
return this;
}
/**
*
* The index of the output in the transaction
*
*
* optional uint32 index = 2;
*/
public Builder clearIndex() {
index_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:InputId)
}
// @@protoc_insertion_point(class_scope:InputId)
private static final org.hyperledger.fabric.protos.token.Transaction.InputId DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.InputId();
}
public static org.hyperledger.fabric.protos.token.Transaction.InputId getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public InputId parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InputId(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.InputId getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PlainDelegatedOutputOrBuilder extends
// @@protoc_insertion_point(interface_extends:PlainDelegatedOutput)
com.google.protobuf.MessageOrBuilder {
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
com.google.protobuf.ByteString getOwner();
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
java.util.List getDelegateesList();
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
int getDelegateesCount();
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
com.google.protobuf.ByteString getDelegatees(int index);
/**
*
* The token type
*
*
* optional string type = 3;
*/
java.lang.String getType();
/**
*
* The token type
*
*
* optional string type = 3;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 4;
*/
long getQuantity();
}
/**
*
* A PlainDelegatedOutput is the result of approve transactions using plaintext tokens
*
*
* Protobuf type {@code PlainDelegatedOutput}
*/
public static final class PlainDelegatedOutput extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PlainDelegatedOutput)
PlainDelegatedOutputOrBuilder {
// Use PlainDelegatedOutput.newBuilder() to construct.
private PlainDelegatedOutput(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PlainDelegatedOutput() {
owner_ = com.google.protobuf.ByteString.EMPTY;
delegatees_ = java.util.Collections.emptyList();
type_ = "";
quantity_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PlainDelegatedOutput(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
owner_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
delegatees_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
delegatees_.add(input.readBytes());
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
type_ = s;
break;
}
case 32: {
quantity_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
delegatees_ = java.util.Collections.unmodifiableList(delegatees_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainDelegatedOutput_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainDelegatedOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.class, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder.class);
}
private int bitField0_;
public static final int OWNER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString owner_;
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public com.google.protobuf.ByteString getOwner() {
return owner_;
}
public static final int DELEGATEES_FIELD_NUMBER = 2;
private java.util.List delegatees_;
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public java.util.List
getDelegateesList() {
return delegatees_;
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public int getDelegateesCount() {
return delegatees_.size();
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public com.google.protobuf.ByteString getDelegatees(int index) {
return delegatees_.get(index);
}
public static final int TYPE_FIELD_NUMBER = 3;
private volatile java.lang.Object type_;
/**
*
* The token type
*
*
* optional string type = 3;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
* The token type
*
*
* optional string type = 3;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUANTITY_FIELD_NUMBER = 4;
private long quantity_;
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 4;
*/
public long getQuantity() {
return quantity_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!owner_.isEmpty()) {
output.writeBytes(1, owner_);
}
for (int i = 0; i < delegatees_.size(); i++) {
output.writeBytes(2, delegatees_.get(i));
}
if (!getTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, type_);
}
if (quantity_ != 0L) {
output.writeUInt64(4, quantity_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!owner_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, owner_);
}
{
int dataSize = 0;
for (int i = 0; i < delegatees_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(delegatees_.get(i));
}
size += dataSize;
size += 1 * getDelegateesList().size();
}
if (!getTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, type_);
}
if (quantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, quantity_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput other = (org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput) obj;
boolean result = true;
result = result && getOwner()
.equals(other.getOwner());
result = result && getDelegateesList()
.equals(other.getDelegateesList());
result = result && getType()
.equals(other.getType());
result = result && (getQuantity()
== other.getQuantity());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + OWNER_FIELD_NUMBER;
hash = (53 * hash) + getOwner().hashCode();
if (getDelegateesCount() > 0) {
hash = (37 * hash) + DELEGATEES_FIELD_NUMBER;
hash = (53 * hash) + getDelegateesList().hashCode();
}
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
hash = (37 * hash) + QUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getQuantity());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A PlainDelegatedOutput is the result of approve transactions using plaintext tokens
*
*
* Protobuf type {@code PlainDelegatedOutput}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PlainDelegatedOutput)
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutputOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainDelegatedOutput_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainDelegatedOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.class, org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
owner_ = com.google.protobuf.ByteString.EMPTY;
delegatees_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
type_ = "";
quantity_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.token.Transaction.internal_static_PlainDelegatedOutput_descriptor;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance();
}
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput build() {
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput buildPartial() {
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput result = new org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.owner_ = owner_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
delegatees_ = java.util.Collections.unmodifiableList(delegatees_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.delegatees_ = delegatees_;
result.type_ = type_;
result.quantity_ = quantity_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput) {
return mergeFrom((org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput other) {
if (other == org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput.getDefaultInstance()) return this;
if (other.getOwner() != com.google.protobuf.ByteString.EMPTY) {
setOwner(other.getOwner());
}
if (!other.delegatees_.isEmpty()) {
if (delegatees_.isEmpty()) {
delegatees_ = other.delegatees_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureDelegateesIsMutable();
delegatees_.addAll(other.delegatees_);
}
onChanged();
}
if (!other.getType().isEmpty()) {
type_ = other.type_;
onChanged();
}
if (other.getQuantity() != 0L) {
setQuantity(other.getQuantity());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString owner_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public com.google.protobuf.ByteString getOwner() {
return owner_;
}
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public Builder setOwner(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
owner_ = value;
onChanged();
return this;
}
/**
*
* The owner is the serialization of a SerializedIdentity struct
*
*
* optional bytes owner = 1;
*/
public Builder clearOwner() {
owner_ = getDefaultInstance().getOwner();
onChanged();
return this;
}
private java.util.List delegatees_ = java.util.Collections.emptyList();
private void ensureDelegateesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
delegatees_ = new java.util.ArrayList(delegatees_);
bitField0_ |= 0x00000002;
}
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public java.util.List
getDelegateesList() {
return java.util.Collections.unmodifiableList(delegatees_);
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public int getDelegateesCount() {
return delegatees_.size();
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public com.google.protobuf.ByteString getDelegatees(int index) {
return delegatees_.get(index);
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public Builder setDelegatees(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDelegateesIsMutable();
delegatees_.set(index, value);
onChanged();
return this;
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public Builder addDelegatees(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDelegateesIsMutable();
delegatees_.add(value);
onChanged();
return this;
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public Builder addAllDelegatees(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureDelegateesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, delegatees_);
onChanged();
return this;
}
/**
*
* The delegatees is an arrary of the serialized identities that can spend the output on behalf
* the owner
*
*
* repeated bytes delegatees = 2;
*/
public Builder clearDelegatees() {
delegatees_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
*
* The token type
*
*
* optional string type = 3;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The token type
*
*
* optional string type = 3;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The token type
*
*
* optional string type = 3;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
return this;
}
/**
*
* The token type
*
*
* optional string type = 3;
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
*
* The token type
*
*
* optional string type = 3;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
onChanged();
return this;
}
private long quantity_ ;
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 4;
*/
public long getQuantity() {
return quantity_;
}
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 4;
*/
public Builder setQuantity(long value) {
quantity_ = value;
onChanged();
return this;
}
/**
*
* The quantity of tokens
*
*
* optional uint64 quantity = 4;
*/
public Builder clearQuantity() {
quantity_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:PlainDelegatedOutput)
}
// @@protoc_insertion_point(class_scope:PlainDelegatedOutput)
private static final org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput();
}
public static org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PlainDelegatedOutput parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlainDelegatedOutput(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.hyperledger.fabric.protos.token.Transaction.PlainDelegatedOutput getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TokenTransaction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_TokenTransaction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainTokenAction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainTokenAction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainImport_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainImport_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainTransfer_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainTransfer_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainApprove_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainApprove_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainTransferFrom_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainTransferFrom_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainOutput_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainOutput_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_InputId_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_InputId_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PlainDelegatedOutput_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PlainDelegatedOutput_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\027token/transaction.proto\"G\n\020TokenTransa" +
"ction\022)\n\014plain_action\030\001 \001(\0132\021.PlainToken" +
"ActionH\000B\010\n\006action\"\355\001\n\020PlainTokenAction\022" +
"$\n\014plain_import\030\001 \001(\0132\014.PlainImportH\000\022(\n" +
"\016plain_transfer\030\002 \001(\0132\016.PlainTransferH\000\022" +
"&\n\014plain_redeem\030\003 \001(\0132\016.PlainTransferH\000\022" +
"&\n\rplain_approve\030\004 \001(\0132\r.PlainApproveH\000\022" +
"1\n\023plain_transfer_From\030\005 \001(\0132\022.PlainTran" +
"sferFromH\000B\006\n\004data\",\n\013PlainImport\022\035\n\007out" +
"puts\030\001 \003(\0132\014.PlainOutput\"H\n\rPlainTransfe",
"r\022\030\n\006inputs\030\001 \003(\0132\010.InputId\022\035\n\007outputs\030\002" +
" \003(\0132\014.PlainOutput\"x\n\014PlainApprove\022\030\n\006in" +
"puts\030\001 \003(\0132\010.InputId\0220\n\021delegated_output" +
"s\030\002 \003(\0132\025.PlainDelegatedOutput\022\034\n\006output" +
"\030\003 \001(\0132\014.PlainOutput\"}\n\021PlainTransferFro" +
"m\022\030\n\006inputs\030\001 \003(\0132\010.InputId\022\035\n\007outputs\030\002" +
" \003(\0132\014.PlainOutput\022/\n\020delegated_output\030\003" +
" \001(\0132\025.PlainDelegatedOutput\"<\n\013PlainOutp" +
"ut\022\r\n\005owner\030\001 \001(\014\022\014\n\004type\030\002 \001(\t\022\020\n\010quant" +
"ity\030\003 \001(\004\"\'\n\007InputId\022\r\n\005tx_id\030\001 \001(\t\022\r\n\005i",
"ndex\030\002 \001(\r\"Y\n\024PlainDelegatedOutput\022\r\n\005ow" +
"ner\030\001 \001(\014\022\022\n\ndelegatees\030\002 \003(\014\022\014\n\004type\030\003 " +
"\001(\t\022\020\n\010quantity\030\004 \001(\004BQ\n#org.hyperledger" +
".fabric.protos.tokenZ*github.com/hyperle" +
"dger/fabric/protos/tokenb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_TokenTransaction_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_TokenTransaction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_TokenTransaction_descriptor,
new java.lang.String[] { "PlainAction", "Action", });
internal_static_PlainTokenAction_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_PlainTokenAction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainTokenAction_descriptor,
new java.lang.String[] { "PlainImport", "PlainTransfer", "PlainRedeem", "PlainApprove", "PlainTransferFrom", "Data", });
internal_static_PlainImport_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_PlainImport_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainImport_descriptor,
new java.lang.String[] { "Outputs", });
internal_static_PlainTransfer_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_PlainTransfer_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainTransfer_descriptor,
new java.lang.String[] { "Inputs", "Outputs", });
internal_static_PlainApprove_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_PlainApprove_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainApprove_descriptor,
new java.lang.String[] { "Inputs", "DelegatedOutputs", "Output", });
internal_static_PlainTransferFrom_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_PlainTransferFrom_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainTransferFrom_descriptor,
new java.lang.String[] { "Inputs", "Outputs", "DelegatedOutput", });
internal_static_PlainOutput_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_PlainOutput_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainOutput_descriptor,
new java.lang.String[] { "Owner", "Type", "Quantity", });
internal_static_InputId_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_InputId_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_InputId_descriptor,
new java.lang.String[] { "TxId", "Index", });
internal_static_PlainDelegatedOutput_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_PlainDelegatedOutput_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PlainDelegatedOutput_descriptor,
new java.lang.String[] { "Owner", "Delegatees", "Type", "Quantity", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy