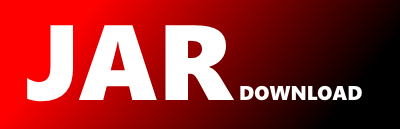
org.hyperledger.fabric.shim.helper.Channel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fabric-chaincode-shim Show documentation
Show all versions of fabric-chaincode-shim Show documentation
Hyperledger Fabric Java Chaincode Shim
/*
Copyright IBM Corp., DTCC All Rights Reserved.
SPDX-License-Identifier: Apache-2.0
*/
package org.hyperledger.fabric.shim.helper;
import java.io.Closeable;
import java.util.HashSet;
import java.util.concurrent.LinkedBlockingQueue;
@SuppressWarnings("serial")
public class Channel extends LinkedBlockingQueue implements Closeable {
private boolean closed = false;
private HashSet waiting = new HashSet<>();
// TODO add other methods to secure closing behavior
@Override
public E take() throws InterruptedException {
synchronized (waiting) {
if (closed) throw new InterruptedException("Channel closed");
waiting.add(Thread.currentThread());
}
E e = super.take();
synchronized (waiting) {
waiting.remove(Thread.currentThread());
}
return e;
}
@Override
public boolean add(E e) {
if (closed) {
throw new IllegalStateException("Channel is closed");
}
return super.add(e);
}
@Override
public void close() {
synchronized (waiting) {
closed = true;
for (Thread t : waiting) {
t.interrupt();
}
waiting.clear();
clear();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy