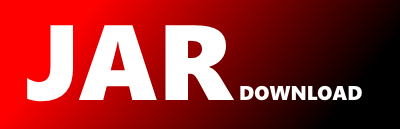
org.hyperledger.fabric.protos.msp.MspConfigPackage Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: msp/msp_config.proto
package org.hyperledger.fabric.protos.msp;
public final class MspConfigPackage {
private MspConfigPackage() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface MSPConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.MSPConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
* Type holds the type of the MSP; the default one would
* be of type FABRIC implementing an X.509 based provider
*
*
* int32 type = 1;
*/
int getType();
/**
*
* Config is MSP dependent configuration info
*
*
* bytes config = 2;
*/
com.google.protobuf.ByteString getConfig();
}
/**
*
* MSPConfig collects all the configuration information for
* an MSP. The Config field should be unmarshalled in a way
* that depends on the Type
*
*
* Protobuf type {@code msp.MSPConfig}
*/
public static final class MSPConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.MSPConfig)
MSPConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use MSPConfig.newBuilder() to construct.
private MSPConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MSPConfig() {
config_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MSPConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
type_ = input.readInt32();
break;
}
case 18: {
config_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_MSPConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_MSPConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.Builder.class);
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
*
* Type holds the type of the MSP; the default one would
* be of type FABRIC implementing an X.509 based provider
*
*
* int32 type = 1;
*/
public int getType() {
return type_;
}
public static final int CONFIG_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString config_;
/**
*
* Config is MSP dependent configuration info
*
*
* bytes config = 2;
*/
public com.google.protobuf.ByteString getConfig() {
return config_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != 0) {
output.writeInt32(1, type_);
}
if (!config_.isEmpty()) {
output.writeBytes(2, config_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, type_);
}
if (!config_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, config_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig) obj;
if (getType()
!= other.getType()) return false;
if (!getConfig()
.equals(other.getConfig())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType();
hash = (37 * hash) + CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getConfig().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MSPConfig collects all the configuration information for
* an MSP. The Config field should be unmarshalled in a way
* that depends on the Type
*
*
* Protobuf type {@code msp.MSPConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.MSPConfig)
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_MSPConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_MSPConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
config_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_MSPConfig_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig(this);
result.type_ = type_;
result.config_ = config_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig.getDefaultInstance()) return this;
if (other.getType() != 0) {
setType(other.getType());
}
if (other.getConfig() != com.google.protobuf.ByteString.EMPTY) {
setConfig(other.getConfig());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int type_ ;
/**
*
* Type holds the type of the MSP; the default one would
* be of type FABRIC implementing an X.509 based provider
*
*
* int32 type = 1;
*/
public int getType() {
return type_;
}
/**
*
* Type holds the type of the MSP; the default one would
* be of type FABRIC implementing an X.509 based provider
*
*
* int32 type = 1;
*/
public Builder setType(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Type holds the type of the MSP; the default one would
* be of type FABRIC implementing an X.509 based provider
*
*
* int32 type = 1;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString config_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Config is MSP dependent configuration info
*
*
* bytes config = 2;
*/
public com.google.protobuf.ByteString getConfig() {
return config_;
}
/**
*
* Config is MSP dependent configuration info
*
*
* bytes config = 2;
*/
public Builder setConfig(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
config_ = value;
onChanged();
return this;
}
/**
*
* Config is MSP dependent configuration info
*
*
* bytes config = 2;
*/
public Builder clearConfig() {
config_ = getDefaultInstance().getConfig();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.MSPConfig)
}
// @@protoc_insertion_point(class_scope:msp.MSPConfig)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MSPConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MSPConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.MSPConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FabricMSPConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.FabricMSPConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
java.util.List getRootCertsList();
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
int getRootCertsCount();
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
com.google.protobuf.ByteString getRootCerts(int index);
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
java.util.List getIntermediateCertsList();
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
int getIntermediateCertsCount();
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
com.google.protobuf.ByteString getIntermediateCerts(int index);
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
java.util.List getAdminsList();
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
int getAdminsCount();
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
com.google.protobuf.ByteString getAdmins(int index);
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
java.util.List getRevocationListList();
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
int getRevocationListCount();
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
com.google.protobuf.ByteString getRevocationList(int index);
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
boolean hasSigningIdentity();
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo getSigningIdentity();
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder getSigningIdentityOrBuilder();
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
java.util.List
getOrganizationalUnitIdentifiersList();
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getOrganizationalUnitIdentifiers(int index);
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
int getOrganizationalUnitIdentifiersCount();
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
java.util.List extends org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getOrganizationalUnitIdentifiersOrBuilderList();
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getOrganizationalUnitIdentifiersOrBuilder(
int index);
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
boolean hasCryptoConfig();
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig getCryptoConfig();
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder getCryptoConfigOrBuilder();
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
java.util.List getTlsRootCertsList();
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
int getTlsRootCertsCount();
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
com.google.protobuf.ByteString getTlsRootCerts(int index);
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
java.util.List getTlsIntermediateCertsList();
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
int getTlsIntermediateCertsCount();
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
com.google.protobuf.ByteString getTlsIntermediateCerts(int index);
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
boolean hasFabricNodeOus();
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs getFabricNodeOus();
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder getFabricNodeOusOrBuilder();
}
/**
*
* FabricMSPConfig collects all the configuration information for
* a Fabric MSP.
* Here we assume a default certificate validation policy, where
* any certificate signed by any of the listed rootCA certs would
* be considered as valid under this MSP.
* This MSP may or may not come with a signing identity. If it does,
* it can also issue signing identities. If it does not, it can only
* be used to validate and verify certificates.
*
*
* Protobuf type {@code msp.FabricMSPConfig}
*/
public static final class FabricMSPConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.FabricMSPConfig)
FabricMSPConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use FabricMSPConfig.newBuilder() to construct.
private FabricMSPConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FabricMSPConfig() {
name_ = "";
rootCerts_ = java.util.Collections.emptyList();
intermediateCerts_ = java.util.Collections.emptyList();
admins_ = java.util.Collections.emptyList();
revocationList_ = java.util.Collections.emptyList();
organizationalUnitIdentifiers_ = java.util.Collections.emptyList();
tlsRootCerts_ = java.util.Collections.emptyList();
tlsIntermediateCerts_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FabricMSPConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
rootCerts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
rootCerts_.add(input.readBytes());
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
intermediateCerts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
intermediateCerts_.add(input.readBytes());
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
admins_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
admins_.add(input.readBytes());
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
revocationList_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
revocationList_.add(input.readBytes());
break;
}
case 50: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder subBuilder = null;
if (signingIdentity_ != null) {
subBuilder = signingIdentity_.toBuilder();
}
signingIdentity_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(signingIdentity_);
signingIdentity_ = subBuilder.buildPartial();
}
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
organizationalUnitIdentifiers_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
organizationalUnitIdentifiers_.add(
input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.parser(), extensionRegistry));
break;
}
case 66: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder subBuilder = null;
if (cryptoConfig_ != null) {
subBuilder = cryptoConfig_.toBuilder();
}
cryptoConfig_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(cryptoConfig_);
cryptoConfig_ = subBuilder.buildPartial();
}
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000100) != 0)) {
tlsRootCerts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
tlsRootCerts_.add(input.readBytes());
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000200) != 0)) {
tlsIntermediateCerts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
tlsIntermediateCerts_.add(input.readBytes());
break;
}
case 90: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder subBuilder = null;
if (fabricNodeOus_ != null) {
subBuilder = fabricNodeOus_.toBuilder();
}
fabricNodeOus_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(fabricNodeOus_);
fabricNodeOus_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
rootCerts_ = java.util.Collections.unmodifiableList(rootCerts_); // C
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
intermediateCerts_ = java.util.Collections.unmodifiableList(intermediateCerts_); // C
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
admins_ = java.util.Collections.unmodifiableList(admins_); // C
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
revocationList_ = java.util.Collections.unmodifiableList(revocationList_); // C
}
if (((mutable_bitField0_ & 0x00000040) != 0)) {
organizationalUnitIdentifiers_ = java.util.Collections.unmodifiableList(organizationalUnitIdentifiers_);
}
if (((mutable_bitField0_ & 0x00000100) != 0)) {
tlsRootCerts_ = java.util.Collections.unmodifiableList(tlsRootCerts_); // C
}
if (((mutable_bitField0_ & 0x00000200) != 0)) {
tlsIntermediateCerts_ = java.util.Collections.unmodifiableList(tlsIntermediateCerts_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricMSPConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricMSPConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROOT_CERTS_FIELD_NUMBER = 2;
private java.util.List rootCerts_;
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public java.util.List
getRootCertsList() {
return rootCerts_;
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public int getRootCertsCount() {
return rootCerts_.size();
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public com.google.protobuf.ByteString getRootCerts(int index) {
return rootCerts_.get(index);
}
public static final int INTERMEDIATE_CERTS_FIELD_NUMBER = 3;
private java.util.List intermediateCerts_;
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public java.util.List
getIntermediateCertsList() {
return intermediateCerts_;
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public int getIntermediateCertsCount() {
return intermediateCerts_.size();
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public com.google.protobuf.ByteString getIntermediateCerts(int index) {
return intermediateCerts_.get(index);
}
public static final int ADMINS_FIELD_NUMBER = 4;
private java.util.List admins_;
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public java.util.List
getAdminsList() {
return admins_;
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public int getAdminsCount() {
return admins_.size();
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public com.google.protobuf.ByteString getAdmins(int index) {
return admins_.get(index);
}
public static final int REVOCATION_LIST_FIELD_NUMBER = 5;
private java.util.List revocationList_;
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public java.util.List
getRevocationListList() {
return revocationList_;
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public int getRevocationListCount() {
return revocationList_.size();
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public com.google.protobuf.ByteString getRevocationList(int index) {
return revocationList_.get(index);
}
public static final int SIGNING_IDENTITY_FIELD_NUMBER = 6;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo signingIdentity_;
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public boolean hasSigningIdentity() {
return signingIdentity_ != null;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo getSigningIdentity() {
return signingIdentity_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.getDefaultInstance() : signingIdentity_;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder getSigningIdentityOrBuilder() {
return getSigningIdentity();
}
public static final int ORGANIZATIONAL_UNIT_IDENTIFIERS_FIELD_NUMBER = 7;
private java.util.List organizationalUnitIdentifiers_;
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public java.util.List getOrganizationalUnitIdentifiersList() {
return organizationalUnitIdentifiers_;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public java.util.List extends org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getOrganizationalUnitIdentifiersOrBuilderList() {
return organizationalUnitIdentifiers_;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public int getOrganizationalUnitIdentifiersCount() {
return organizationalUnitIdentifiers_.size();
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getOrganizationalUnitIdentifiers(int index) {
return organizationalUnitIdentifiers_.get(index);
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getOrganizationalUnitIdentifiersOrBuilder(
int index) {
return organizationalUnitIdentifiers_.get(index);
}
public static final int CRYPTO_CONFIG_FIELD_NUMBER = 8;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig cryptoConfig_;
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public boolean hasCryptoConfig() {
return cryptoConfig_ != null;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig getCryptoConfig() {
return cryptoConfig_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.getDefaultInstance() : cryptoConfig_;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder getCryptoConfigOrBuilder() {
return getCryptoConfig();
}
public static final int TLS_ROOT_CERTS_FIELD_NUMBER = 9;
private java.util.List tlsRootCerts_;
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public java.util.List
getTlsRootCertsList() {
return tlsRootCerts_;
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public int getTlsRootCertsCount() {
return tlsRootCerts_.size();
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public com.google.protobuf.ByteString getTlsRootCerts(int index) {
return tlsRootCerts_.get(index);
}
public static final int TLS_INTERMEDIATE_CERTS_FIELD_NUMBER = 10;
private java.util.List tlsIntermediateCerts_;
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public java.util.List
getTlsIntermediateCertsList() {
return tlsIntermediateCerts_;
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public int getTlsIntermediateCertsCount() {
return tlsIntermediateCerts_.size();
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public com.google.protobuf.ByteString getTlsIntermediateCerts(int index) {
return tlsIntermediateCerts_.get(index);
}
public static final int FABRIC_NODE_OUS_FIELD_NUMBER = 11;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs fabricNodeOus_;
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public boolean hasFabricNodeOus() {
return fabricNodeOus_ != null;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs getFabricNodeOus() {
return fabricNodeOus_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.getDefaultInstance() : fabricNodeOus_;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder getFabricNodeOusOrBuilder() {
return getFabricNodeOus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
for (int i = 0; i < rootCerts_.size(); i++) {
output.writeBytes(2, rootCerts_.get(i));
}
for (int i = 0; i < intermediateCerts_.size(); i++) {
output.writeBytes(3, intermediateCerts_.get(i));
}
for (int i = 0; i < admins_.size(); i++) {
output.writeBytes(4, admins_.get(i));
}
for (int i = 0; i < revocationList_.size(); i++) {
output.writeBytes(5, revocationList_.get(i));
}
if (signingIdentity_ != null) {
output.writeMessage(6, getSigningIdentity());
}
for (int i = 0; i < organizationalUnitIdentifiers_.size(); i++) {
output.writeMessage(7, organizationalUnitIdentifiers_.get(i));
}
if (cryptoConfig_ != null) {
output.writeMessage(8, getCryptoConfig());
}
for (int i = 0; i < tlsRootCerts_.size(); i++) {
output.writeBytes(9, tlsRootCerts_.get(i));
}
for (int i = 0; i < tlsIntermediateCerts_.size(); i++) {
output.writeBytes(10, tlsIntermediateCerts_.get(i));
}
if (fabricNodeOus_ != null) {
output.writeMessage(11, getFabricNodeOus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
{
int dataSize = 0;
for (int i = 0; i < rootCerts_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(rootCerts_.get(i));
}
size += dataSize;
size += 1 * getRootCertsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < intermediateCerts_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(intermediateCerts_.get(i));
}
size += dataSize;
size += 1 * getIntermediateCertsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < admins_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(admins_.get(i));
}
size += dataSize;
size += 1 * getAdminsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < revocationList_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(revocationList_.get(i));
}
size += dataSize;
size += 1 * getRevocationListList().size();
}
if (signingIdentity_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getSigningIdentity());
}
for (int i = 0; i < organizationalUnitIdentifiers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, organizationalUnitIdentifiers_.get(i));
}
if (cryptoConfig_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getCryptoConfig());
}
{
int dataSize = 0;
for (int i = 0; i < tlsRootCerts_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(tlsRootCerts_.get(i));
}
size += dataSize;
size += 1 * getTlsRootCertsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < tlsIntermediateCerts_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(tlsIntermediateCerts_.get(i));
}
size += dataSize;
size += 1 * getTlsIntermediateCertsList().size();
}
if (fabricNodeOus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getFabricNodeOus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getRootCertsList()
.equals(other.getRootCertsList())) return false;
if (!getIntermediateCertsList()
.equals(other.getIntermediateCertsList())) return false;
if (!getAdminsList()
.equals(other.getAdminsList())) return false;
if (!getRevocationListList()
.equals(other.getRevocationListList())) return false;
if (hasSigningIdentity() != other.hasSigningIdentity()) return false;
if (hasSigningIdentity()) {
if (!getSigningIdentity()
.equals(other.getSigningIdentity())) return false;
}
if (!getOrganizationalUnitIdentifiersList()
.equals(other.getOrganizationalUnitIdentifiersList())) return false;
if (hasCryptoConfig() != other.hasCryptoConfig()) return false;
if (hasCryptoConfig()) {
if (!getCryptoConfig()
.equals(other.getCryptoConfig())) return false;
}
if (!getTlsRootCertsList()
.equals(other.getTlsRootCertsList())) return false;
if (!getTlsIntermediateCertsList()
.equals(other.getTlsIntermediateCertsList())) return false;
if (hasFabricNodeOus() != other.hasFabricNodeOus()) return false;
if (hasFabricNodeOus()) {
if (!getFabricNodeOus()
.equals(other.getFabricNodeOus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (getRootCertsCount() > 0) {
hash = (37 * hash) + ROOT_CERTS_FIELD_NUMBER;
hash = (53 * hash) + getRootCertsList().hashCode();
}
if (getIntermediateCertsCount() > 0) {
hash = (37 * hash) + INTERMEDIATE_CERTS_FIELD_NUMBER;
hash = (53 * hash) + getIntermediateCertsList().hashCode();
}
if (getAdminsCount() > 0) {
hash = (37 * hash) + ADMINS_FIELD_NUMBER;
hash = (53 * hash) + getAdminsList().hashCode();
}
if (getRevocationListCount() > 0) {
hash = (37 * hash) + REVOCATION_LIST_FIELD_NUMBER;
hash = (53 * hash) + getRevocationListList().hashCode();
}
if (hasSigningIdentity()) {
hash = (37 * hash) + SIGNING_IDENTITY_FIELD_NUMBER;
hash = (53 * hash) + getSigningIdentity().hashCode();
}
if (getOrganizationalUnitIdentifiersCount() > 0) {
hash = (37 * hash) + ORGANIZATIONAL_UNIT_IDENTIFIERS_FIELD_NUMBER;
hash = (53 * hash) + getOrganizationalUnitIdentifiersList().hashCode();
}
if (hasCryptoConfig()) {
hash = (37 * hash) + CRYPTO_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getCryptoConfig().hashCode();
}
if (getTlsRootCertsCount() > 0) {
hash = (37 * hash) + TLS_ROOT_CERTS_FIELD_NUMBER;
hash = (53 * hash) + getTlsRootCertsList().hashCode();
}
if (getTlsIntermediateCertsCount() > 0) {
hash = (37 * hash) + TLS_INTERMEDIATE_CERTS_FIELD_NUMBER;
hash = (53 * hash) + getTlsIntermediateCertsList().hashCode();
}
if (hasFabricNodeOus()) {
hash = (37 * hash) + FABRIC_NODE_OUS_FIELD_NUMBER;
hash = (53 * hash) + getFabricNodeOus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* FabricMSPConfig collects all the configuration information for
* a Fabric MSP.
* Here we assume a default certificate validation policy, where
* any certificate signed by any of the listed rootCA certs would
* be considered as valid under this MSP.
* This MSP may or may not come with a signing identity. If it does,
* it can also issue signing identities. If it does not, it can only
* be used to validate and verify certificates.
*
*
* Protobuf type {@code msp.FabricMSPConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.FabricMSPConfig)
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricMSPConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricMSPConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOrganizationalUnitIdentifiersFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
rootCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
intermediateCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
admins_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
revocationList_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
if (signingIdentityBuilder_ == null) {
signingIdentity_ = null;
} else {
signingIdentity_ = null;
signingIdentityBuilder_ = null;
}
if (organizationalUnitIdentifiersBuilder_ == null) {
organizationalUnitIdentifiers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
organizationalUnitIdentifiersBuilder_.clear();
}
if (cryptoConfigBuilder_ == null) {
cryptoConfig_ = null;
} else {
cryptoConfig_ = null;
cryptoConfigBuilder_ = null;
}
tlsRootCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
tlsIntermediateCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
if (fabricNodeOusBuilder_ == null) {
fabricNodeOus_ = null;
} else {
fabricNodeOus_ = null;
fabricNodeOusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricMSPConfig_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.name_ = name_;
if (((bitField0_ & 0x00000002) != 0)) {
rootCerts_ = java.util.Collections.unmodifiableList(rootCerts_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.rootCerts_ = rootCerts_;
if (((bitField0_ & 0x00000004) != 0)) {
intermediateCerts_ = java.util.Collections.unmodifiableList(intermediateCerts_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.intermediateCerts_ = intermediateCerts_;
if (((bitField0_ & 0x00000008) != 0)) {
admins_ = java.util.Collections.unmodifiableList(admins_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.admins_ = admins_;
if (((bitField0_ & 0x00000010) != 0)) {
revocationList_ = java.util.Collections.unmodifiableList(revocationList_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.revocationList_ = revocationList_;
if (signingIdentityBuilder_ == null) {
result.signingIdentity_ = signingIdentity_;
} else {
result.signingIdentity_ = signingIdentityBuilder_.build();
}
if (organizationalUnitIdentifiersBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
organizationalUnitIdentifiers_ = java.util.Collections.unmodifiableList(organizationalUnitIdentifiers_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.organizationalUnitIdentifiers_ = organizationalUnitIdentifiers_;
} else {
result.organizationalUnitIdentifiers_ = organizationalUnitIdentifiersBuilder_.build();
}
if (cryptoConfigBuilder_ == null) {
result.cryptoConfig_ = cryptoConfig_;
} else {
result.cryptoConfig_ = cryptoConfigBuilder_.build();
}
if (((bitField0_ & 0x00000100) != 0)) {
tlsRootCerts_ = java.util.Collections.unmodifiableList(tlsRootCerts_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.tlsRootCerts_ = tlsRootCerts_;
if (((bitField0_ & 0x00000200) != 0)) {
tlsIntermediateCerts_ = java.util.Collections.unmodifiableList(tlsIntermediateCerts_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.tlsIntermediateCerts_ = tlsIntermediateCerts_;
if (fabricNodeOusBuilder_ == null) {
result.fabricNodeOus_ = fabricNodeOus_;
} else {
result.fabricNodeOus_ = fabricNodeOusBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.rootCerts_.isEmpty()) {
if (rootCerts_.isEmpty()) {
rootCerts_ = other.rootCerts_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRootCertsIsMutable();
rootCerts_.addAll(other.rootCerts_);
}
onChanged();
}
if (!other.intermediateCerts_.isEmpty()) {
if (intermediateCerts_.isEmpty()) {
intermediateCerts_ = other.intermediateCerts_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureIntermediateCertsIsMutable();
intermediateCerts_.addAll(other.intermediateCerts_);
}
onChanged();
}
if (!other.admins_.isEmpty()) {
if (admins_.isEmpty()) {
admins_ = other.admins_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureAdminsIsMutable();
admins_.addAll(other.admins_);
}
onChanged();
}
if (!other.revocationList_.isEmpty()) {
if (revocationList_.isEmpty()) {
revocationList_ = other.revocationList_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureRevocationListIsMutable();
revocationList_.addAll(other.revocationList_);
}
onChanged();
}
if (other.hasSigningIdentity()) {
mergeSigningIdentity(other.getSigningIdentity());
}
if (organizationalUnitIdentifiersBuilder_ == null) {
if (!other.organizationalUnitIdentifiers_.isEmpty()) {
if (organizationalUnitIdentifiers_.isEmpty()) {
organizationalUnitIdentifiers_ = other.organizationalUnitIdentifiers_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.addAll(other.organizationalUnitIdentifiers_);
}
onChanged();
}
} else {
if (!other.organizationalUnitIdentifiers_.isEmpty()) {
if (organizationalUnitIdentifiersBuilder_.isEmpty()) {
organizationalUnitIdentifiersBuilder_.dispose();
organizationalUnitIdentifiersBuilder_ = null;
organizationalUnitIdentifiers_ = other.organizationalUnitIdentifiers_;
bitField0_ = (bitField0_ & ~0x00000040);
organizationalUnitIdentifiersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOrganizationalUnitIdentifiersFieldBuilder() : null;
} else {
organizationalUnitIdentifiersBuilder_.addAllMessages(other.organizationalUnitIdentifiers_);
}
}
}
if (other.hasCryptoConfig()) {
mergeCryptoConfig(other.getCryptoConfig());
}
if (!other.tlsRootCerts_.isEmpty()) {
if (tlsRootCerts_.isEmpty()) {
tlsRootCerts_ = other.tlsRootCerts_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureTlsRootCertsIsMutable();
tlsRootCerts_.addAll(other.tlsRootCerts_);
}
onChanged();
}
if (!other.tlsIntermediateCerts_.isEmpty()) {
if (tlsIntermediateCerts_.isEmpty()) {
tlsIntermediateCerts_ = other.tlsIntermediateCerts_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureTlsIntermediateCertsIsMutable();
tlsIntermediateCerts_.addAll(other.tlsIntermediateCerts_);
}
onChanged();
}
if (other.hasFabricNodeOus()) {
mergeFabricNodeOus(other.getFabricNodeOus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name holds the identifier of the MSP; MSP identifier
* is chosen by the application that governs this MSP.
* For example, and assuming the default implementation of MSP,
* that is X.509-based and considers a single Issuer,
* this can refer to the Subject OU field or the Issuer OU field.
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.util.List rootCerts_ = java.util.Collections.emptyList();
private void ensureRootCertsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
rootCerts_ = new java.util.ArrayList(rootCerts_);
bitField0_ |= 0x00000002;
}
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public java.util.List
getRootCertsList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(rootCerts_) : rootCerts_;
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public int getRootCertsCount() {
return rootCerts_.size();
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public com.google.protobuf.ByteString getRootCerts(int index) {
return rootCerts_.get(index);
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public Builder setRootCerts(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRootCertsIsMutable();
rootCerts_.set(index, value);
onChanged();
return this;
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public Builder addRootCerts(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRootCertsIsMutable();
rootCerts_.add(value);
onChanged();
return this;
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public Builder addAllRootCerts(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureRootCertsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rootCerts_);
onChanged();
return this;
}
/**
*
* List of root certificates trusted by this MSP
* they are used upon certificate validation (see
* comment for IntermediateCerts below)
*
*
* repeated bytes root_certs = 2;
*/
public Builder clearRootCerts() {
rootCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private java.util.List intermediateCerts_ = java.util.Collections.emptyList();
private void ensureIntermediateCertsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
intermediateCerts_ = new java.util.ArrayList(intermediateCerts_);
bitField0_ |= 0x00000004;
}
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public java.util.List
getIntermediateCertsList() {
return ((bitField0_ & 0x00000004) != 0) ?
java.util.Collections.unmodifiableList(intermediateCerts_) : intermediateCerts_;
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public int getIntermediateCertsCount() {
return intermediateCerts_.size();
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public com.google.protobuf.ByteString getIntermediateCerts(int index) {
return intermediateCerts_.get(index);
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public Builder setIntermediateCerts(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureIntermediateCertsIsMutable();
intermediateCerts_.set(index, value);
onChanged();
return this;
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public Builder addIntermediateCerts(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureIntermediateCertsIsMutable();
intermediateCerts_.add(value);
onChanged();
return this;
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public Builder addAllIntermediateCerts(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureIntermediateCertsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, intermediateCerts_);
onChanged();
return this;
}
/**
*
* List of intermediate certificates trusted by this MSP;
* they are used upon certificate validation as follows:
* validation attempts to build a path from the certificate
* to be validated (which is at one end of the path) and
* one of the certs in the RootCerts field (which is at
* the other end of the path). If the path is longer than
* 2, certificates in the middle are searched within the
* IntermediateCerts pool
*
*
* repeated bytes intermediate_certs = 3;
*/
public Builder clearIntermediateCerts() {
intermediateCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private java.util.List admins_ = java.util.Collections.emptyList();
private void ensureAdminsIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
admins_ = new java.util.ArrayList(admins_);
bitField0_ |= 0x00000008;
}
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public java.util.List
getAdminsList() {
return ((bitField0_ & 0x00000008) != 0) ?
java.util.Collections.unmodifiableList(admins_) : admins_;
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public int getAdminsCount() {
return admins_.size();
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public com.google.protobuf.ByteString getAdmins(int index) {
return admins_.get(index);
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public Builder setAdmins(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAdminsIsMutable();
admins_.set(index, value);
onChanged();
return this;
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public Builder addAdmins(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAdminsIsMutable();
admins_.add(value);
onChanged();
return this;
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public Builder addAllAdmins(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureAdminsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, admins_);
onChanged();
return this;
}
/**
*
* Identity denoting the administrator of this MSP
*
*
* repeated bytes admins = 4;
*/
public Builder clearAdmins() {
admins_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
private java.util.List revocationList_ = java.util.Collections.emptyList();
private void ensureRevocationListIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
revocationList_ = new java.util.ArrayList(revocationList_);
bitField0_ |= 0x00000010;
}
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public java.util.List
getRevocationListList() {
return ((bitField0_ & 0x00000010) != 0) ?
java.util.Collections.unmodifiableList(revocationList_) : revocationList_;
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public int getRevocationListCount() {
return revocationList_.size();
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public com.google.protobuf.ByteString getRevocationList(int index) {
return revocationList_.get(index);
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public Builder setRevocationList(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRevocationListIsMutable();
revocationList_.set(index, value);
onChanged();
return this;
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public Builder addRevocationList(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRevocationListIsMutable();
revocationList_.add(value);
onChanged();
return this;
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public Builder addAllRevocationList(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureRevocationListIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, revocationList_);
onChanged();
return this;
}
/**
*
* Identity revocation list
*
*
* repeated bytes revocation_list = 5;
*/
public Builder clearRevocationList() {
revocationList_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo signingIdentity_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder> signingIdentityBuilder_;
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public boolean hasSigningIdentity() {
return signingIdentityBuilder_ != null || signingIdentity_ != null;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo getSigningIdentity() {
if (signingIdentityBuilder_ == null) {
return signingIdentity_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.getDefaultInstance() : signingIdentity_;
} else {
return signingIdentityBuilder_.getMessage();
}
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public Builder setSigningIdentity(org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo value) {
if (signingIdentityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
signingIdentity_ = value;
onChanged();
} else {
signingIdentityBuilder_.setMessage(value);
}
return this;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public Builder setSigningIdentity(
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder builderForValue) {
if (signingIdentityBuilder_ == null) {
signingIdentity_ = builderForValue.build();
onChanged();
} else {
signingIdentityBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public Builder mergeSigningIdentity(org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo value) {
if (signingIdentityBuilder_ == null) {
if (signingIdentity_ != null) {
signingIdentity_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.newBuilder(signingIdentity_).mergeFrom(value).buildPartial();
} else {
signingIdentity_ = value;
}
onChanged();
} else {
signingIdentityBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public Builder clearSigningIdentity() {
if (signingIdentityBuilder_ == null) {
signingIdentity_ = null;
onChanged();
} else {
signingIdentity_ = null;
signingIdentityBuilder_ = null;
}
return this;
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder getSigningIdentityBuilder() {
onChanged();
return getSigningIdentityFieldBuilder().getBuilder();
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder getSigningIdentityOrBuilder() {
if (signingIdentityBuilder_ != null) {
return signingIdentityBuilder_.getMessageOrBuilder();
} else {
return signingIdentity_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.getDefaultInstance() : signingIdentity_;
}
}
/**
*
* SigningIdentity holds information on the signing identity
* this peer is to use, and which is to be imported by the
* MSP defined before
*
*
* .msp.SigningIdentityInfo signing_identity = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder>
getSigningIdentityFieldBuilder() {
if (signingIdentityBuilder_ == null) {
signingIdentityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder>(
getSigningIdentity(),
getParentForChildren(),
isClean());
signingIdentity_ = null;
}
return signingIdentityBuilder_;
}
private java.util.List organizationalUnitIdentifiers_ =
java.util.Collections.emptyList();
private void ensureOrganizationalUnitIdentifiersIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
organizationalUnitIdentifiers_ = new java.util.ArrayList(organizationalUnitIdentifiers_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder> organizationalUnitIdentifiersBuilder_;
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public java.util.List getOrganizationalUnitIdentifiersList() {
if (organizationalUnitIdentifiersBuilder_ == null) {
return java.util.Collections.unmodifiableList(organizationalUnitIdentifiers_);
} else {
return organizationalUnitIdentifiersBuilder_.getMessageList();
}
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public int getOrganizationalUnitIdentifiersCount() {
if (organizationalUnitIdentifiersBuilder_ == null) {
return organizationalUnitIdentifiers_.size();
} else {
return organizationalUnitIdentifiersBuilder_.getCount();
}
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getOrganizationalUnitIdentifiers(int index) {
if (organizationalUnitIdentifiersBuilder_ == null) {
return organizationalUnitIdentifiers_.get(index);
} else {
return organizationalUnitIdentifiersBuilder_.getMessage(index);
}
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder setOrganizationalUnitIdentifiers(
int index, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (organizationalUnitIdentifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.set(index, value);
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder setOrganizationalUnitIdentifiers(
int index, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (organizationalUnitIdentifiersBuilder_ == null) {
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.set(index, builderForValue.build());
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder addOrganizationalUnitIdentifiers(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (organizationalUnitIdentifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.add(value);
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.addMessage(value);
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder addOrganizationalUnitIdentifiers(
int index, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (organizationalUnitIdentifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.add(index, value);
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder addOrganizationalUnitIdentifiers(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (organizationalUnitIdentifiersBuilder_ == null) {
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.add(builderForValue.build());
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder addOrganizationalUnitIdentifiers(
int index, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (organizationalUnitIdentifiersBuilder_ == null) {
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.add(index, builderForValue.build());
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder addAllOrganizationalUnitIdentifiers(
java.lang.Iterable extends org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier> values) {
if (organizationalUnitIdentifiersBuilder_ == null) {
ensureOrganizationalUnitIdentifiersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, organizationalUnitIdentifiers_);
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder clearOrganizationalUnitIdentifiers() {
if (organizationalUnitIdentifiersBuilder_ == null) {
organizationalUnitIdentifiers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.clear();
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public Builder removeOrganizationalUnitIdentifiers(int index) {
if (organizationalUnitIdentifiersBuilder_ == null) {
ensureOrganizationalUnitIdentifiersIsMutable();
organizationalUnitIdentifiers_.remove(index);
onChanged();
} else {
organizationalUnitIdentifiersBuilder_.remove(index);
}
return this;
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder getOrganizationalUnitIdentifiersBuilder(
int index) {
return getOrganizationalUnitIdentifiersFieldBuilder().getBuilder(index);
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getOrganizationalUnitIdentifiersOrBuilder(
int index) {
if (organizationalUnitIdentifiersBuilder_ == null) {
return organizationalUnitIdentifiers_.get(index); } else {
return organizationalUnitIdentifiersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public java.util.List extends org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getOrganizationalUnitIdentifiersOrBuilderList() {
if (organizationalUnitIdentifiersBuilder_ != null) {
return organizationalUnitIdentifiersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(organizationalUnitIdentifiers_);
}
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder addOrganizationalUnitIdentifiersBuilder() {
return getOrganizationalUnitIdentifiersFieldBuilder().addBuilder(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance());
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder addOrganizationalUnitIdentifiersBuilder(
int index) {
return getOrganizationalUnitIdentifiersFieldBuilder().addBuilder(
index, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance());
}
/**
*
* OrganizationalUnitIdentifiers holds one or more
* fabric organizational unit identifiers that belong to
* this MSP configuration
*
*
* repeated .msp.FabricOUIdentifier organizational_unit_identifiers = 7;
*/
public java.util.List
getOrganizationalUnitIdentifiersBuilderList() {
return getOrganizationalUnitIdentifiersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getOrganizationalUnitIdentifiersFieldBuilder() {
if (organizationalUnitIdentifiersBuilder_ == null) {
organizationalUnitIdentifiersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>(
organizationalUnitIdentifiers_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
organizationalUnitIdentifiers_ = null;
}
return organizationalUnitIdentifiersBuilder_;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig cryptoConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder> cryptoConfigBuilder_;
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public boolean hasCryptoConfig() {
return cryptoConfigBuilder_ != null || cryptoConfig_ != null;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig getCryptoConfig() {
if (cryptoConfigBuilder_ == null) {
return cryptoConfig_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.getDefaultInstance() : cryptoConfig_;
} else {
return cryptoConfigBuilder_.getMessage();
}
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public Builder setCryptoConfig(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig value) {
if (cryptoConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cryptoConfig_ = value;
onChanged();
} else {
cryptoConfigBuilder_.setMessage(value);
}
return this;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public Builder setCryptoConfig(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder builderForValue) {
if (cryptoConfigBuilder_ == null) {
cryptoConfig_ = builderForValue.build();
onChanged();
} else {
cryptoConfigBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public Builder mergeCryptoConfig(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig value) {
if (cryptoConfigBuilder_ == null) {
if (cryptoConfig_ != null) {
cryptoConfig_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.newBuilder(cryptoConfig_).mergeFrom(value).buildPartial();
} else {
cryptoConfig_ = value;
}
onChanged();
} else {
cryptoConfigBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public Builder clearCryptoConfig() {
if (cryptoConfigBuilder_ == null) {
cryptoConfig_ = null;
onChanged();
} else {
cryptoConfig_ = null;
cryptoConfigBuilder_ = null;
}
return this;
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder getCryptoConfigBuilder() {
onChanged();
return getCryptoConfigFieldBuilder().getBuilder();
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder getCryptoConfigOrBuilder() {
if (cryptoConfigBuilder_ != null) {
return cryptoConfigBuilder_.getMessageOrBuilder();
} else {
return cryptoConfig_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.getDefaultInstance() : cryptoConfig_;
}
}
/**
*
* FabricCryptoConfig contains the configuration parameters
* for the cryptographic algorithms used by this MSP
*
*
* .msp.FabricCryptoConfig crypto_config = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder>
getCryptoConfigFieldBuilder() {
if (cryptoConfigBuilder_ == null) {
cryptoConfigBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder>(
getCryptoConfig(),
getParentForChildren(),
isClean());
cryptoConfig_ = null;
}
return cryptoConfigBuilder_;
}
private java.util.List tlsRootCerts_ = java.util.Collections.emptyList();
private void ensureTlsRootCertsIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
tlsRootCerts_ = new java.util.ArrayList(tlsRootCerts_);
bitField0_ |= 0x00000100;
}
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public java.util.List
getTlsRootCertsList() {
return ((bitField0_ & 0x00000100) != 0) ?
java.util.Collections.unmodifiableList(tlsRootCerts_) : tlsRootCerts_;
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public int getTlsRootCertsCount() {
return tlsRootCerts_.size();
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public com.google.protobuf.ByteString getTlsRootCerts(int index) {
return tlsRootCerts_.get(index);
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public Builder setTlsRootCerts(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTlsRootCertsIsMutable();
tlsRootCerts_.set(index, value);
onChanged();
return this;
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public Builder addTlsRootCerts(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTlsRootCertsIsMutable();
tlsRootCerts_.add(value);
onChanged();
return this;
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public Builder addAllTlsRootCerts(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureTlsRootCertsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tlsRootCerts_);
onChanged();
return this;
}
/**
*
* List of TLS root certificates trusted by this MSP.
* They are returned by GetTLSRootCerts.
*
*
* repeated bytes tls_root_certs = 9;
*/
public Builder clearTlsRootCerts() {
tlsRootCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
private java.util.List tlsIntermediateCerts_ = java.util.Collections.emptyList();
private void ensureTlsIntermediateCertsIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
tlsIntermediateCerts_ = new java.util.ArrayList(tlsIntermediateCerts_);
bitField0_ |= 0x00000200;
}
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public java.util.List
getTlsIntermediateCertsList() {
return ((bitField0_ & 0x00000200) != 0) ?
java.util.Collections.unmodifiableList(tlsIntermediateCerts_) : tlsIntermediateCerts_;
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public int getTlsIntermediateCertsCount() {
return tlsIntermediateCerts_.size();
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public com.google.protobuf.ByteString getTlsIntermediateCerts(int index) {
return tlsIntermediateCerts_.get(index);
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public Builder setTlsIntermediateCerts(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTlsIntermediateCertsIsMutable();
tlsIntermediateCerts_.set(index, value);
onChanged();
return this;
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public Builder addTlsIntermediateCerts(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTlsIntermediateCertsIsMutable();
tlsIntermediateCerts_.add(value);
onChanged();
return this;
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public Builder addAllTlsIntermediateCerts(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureTlsIntermediateCertsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tlsIntermediateCerts_);
onChanged();
return this;
}
/**
*
* List of TLS intermediate certificates trusted by this MSP;
* They are returned by GetTLSIntermediateCerts.
*
*
* repeated bytes tls_intermediate_certs = 10;
*/
public Builder clearTlsIntermediateCerts() {
tlsIntermediateCerts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs fabricNodeOus_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder> fabricNodeOusBuilder_;
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public boolean hasFabricNodeOus() {
return fabricNodeOusBuilder_ != null || fabricNodeOus_ != null;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs getFabricNodeOus() {
if (fabricNodeOusBuilder_ == null) {
return fabricNodeOus_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.getDefaultInstance() : fabricNodeOus_;
} else {
return fabricNodeOusBuilder_.getMessage();
}
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public Builder setFabricNodeOus(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs value) {
if (fabricNodeOusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
fabricNodeOus_ = value;
onChanged();
} else {
fabricNodeOusBuilder_.setMessage(value);
}
return this;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public Builder setFabricNodeOus(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder builderForValue) {
if (fabricNodeOusBuilder_ == null) {
fabricNodeOus_ = builderForValue.build();
onChanged();
} else {
fabricNodeOusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public Builder mergeFabricNodeOus(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs value) {
if (fabricNodeOusBuilder_ == null) {
if (fabricNodeOus_ != null) {
fabricNodeOus_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.newBuilder(fabricNodeOus_).mergeFrom(value).buildPartial();
} else {
fabricNodeOus_ = value;
}
onChanged();
} else {
fabricNodeOusBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public Builder clearFabricNodeOus() {
if (fabricNodeOusBuilder_ == null) {
fabricNodeOus_ = null;
onChanged();
} else {
fabricNodeOus_ = null;
fabricNodeOusBuilder_ = null;
}
return this;
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder getFabricNodeOusBuilder() {
onChanged();
return getFabricNodeOusFieldBuilder().getBuilder();
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder getFabricNodeOusOrBuilder() {
if (fabricNodeOusBuilder_ != null) {
return fabricNodeOusBuilder_.getMessageOrBuilder();
} else {
return fabricNodeOus_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.getDefaultInstance() : fabricNodeOus_;
}
}
/**
*
* fabric_node_ous contains the configuration to distinguish clients from peers from orderers
* based on the OUs.
*
*
* .msp.FabricNodeOUs fabric_node_ous = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder>
getFabricNodeOusFieldBuilder() {
if (fabricNodeOusBuilder_ == null) {
fabricNodeOusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder>(
getFabricNodeOus(),
getParentForChildren(),
isClean());
fabricNodeOus_ = null;
}
return fabricNodeOusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.FabricMSPConfig)
}
// @@protoc_insertion_point(class_scope:msp.FabricMSPConfig)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FabricMSPConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FabricMSPConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricMSPConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FabricCryptoConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.FabricCryptoConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
java.lang.String getSignatureHashFamily();
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
com.google.protobuf.ByteString
getSignatureHashFamilyBytes();
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
java.lang.String getIdentityIdentifierHashFunction();
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
com.google.protobuf.ByteString
getIdentityIdentifierHashFunctionBytes();
}
/**
*
* FabricCryptoConfig contains configuration parameters
* for the cryptographic algorithms used by the MSP
* this configuration refers to
*
*
* Protobuf type {@code msp.FabricCryptoConfig}
*/
public static final class FabricCryptoConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.FabricCryptoConfig)
FabricCryptoConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use FabricCryptoConfig.newBuilder() to construct.
private FabricCryptoConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FabricCryptoConfig() {
signatureHashFamily_ = "";
identityIdentifierHashFunction_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FabricCryptoConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
signatureHashFamily_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
identityIdentifierHashFunction_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricCryptoConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricCryptoConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder.class);
}
public static final int SIGNATURE_HASH_FAMILY_FIELD_NUMBER = 1;
private volatile java.lang.Object signatureHashFamily_;
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public java.lang.String getSignatureHashFamily() {
java.lang.Object ref = signatureHashFamily_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signatureHashFamily_ = s;
return s;
}
}
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public com.google.protobuf.ByteString
getSignatureHashFamilyBytes() {
java.lang.Object ref = signatureHashFamily_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signatureHashFamily_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IDENTITY_IDENTIFIER_HASH_FUNCTION_FIELD_NUMBER = 2;
private volatile java.lang.Object identityIdentifierHashFunction_;
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public java.lang.String getIdentityIdentifierHashFunction() {
java.lang.Object ref = identityIdentifierHashFunction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identityIdentifierHashFunction_ = s;
return s;
}
}
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public com.google.protobuf.ByteString
getIdentityIdentifierHashFunctionBytes() {
java.lang.Object ref = identityIdentifierHashFunction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identityIdentifierHashFunction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getSignatureHashFamilyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, signatureHashFamily_);
}
if (!getIdentityIdentifierHashFunctionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, identityIdentifierHashFunction_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getSignatureHashFamilyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, signatureHashFamily_);
}
if (!getIdentityIdentifierHashFunctionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, identityIdentifierHashFunction_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig) obj;
if (!getSignatureHashFamily()
.equals(other.getSignatureHashFamily())) return false;
if (!getIdentityIdentifierHashFunction()
.equals(other.getIdentityIdentifierHashFunction())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SIGNATURE_HASH_FAMILY_FIELD_NUMBER;
hash = (53 * hash) + getSignatureHashFamily().hashCode();
hash = (37 * hash) + IDENTITY_IDENTIFIER_HASH_FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getIdentityIdentifierHashFunction().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* FabricCryptoConfig contains configuration parameters
* for the cryptographic algorithms used by the MSP
* this configuration refers to
*
*
* Protobuf type {@code msp.FabricCryptoConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.FabricCryptoConfig)
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricCryptoConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricCryptoConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
signatureHashFamily_ = "";
identityIdentifierHashFunction_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricCryptoConfig_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig(this);
result.signatureHashFamily_ = signatureHashFamily_;
result.identityIdentifierHashFunction_ = identityIdentifierHashFunction_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig.getDefaultInstance()) return this;
if (!other.getSignatureHashFamily().isEmpty()) {
signatureHashFamily_ = other.signatureHashFamily_;
onChanged();
}
if (!other.getIdentityIdentifierHashFunction().isEmpty()) {
identityIdentifierHashFunction_ = other.identityIdentifierHashFunction_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object signatureHashFamily_ = "";
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public java.lang.String getSignatureHashFamily() {
java.lang.Object ref = signatureHashFamily_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signatureHashFamily_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public com.google.protobuf.ByteString
getSignatureHashFamilyBytes() {
java.lang.Object ref = signatureHashFamily_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signatureHashFamily_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public Builder setSignatureHashFamily(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
signatureHashFamily_ = value;
onChanged();
return this;
}
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public Builder clearSignatureHashFamily() {
signatureHashFamily_ = getDefaultInstance().getSignatureHashFamily();
onChanged();
return this;
}
/**
*
* SignatureHashFamily is a string representing the hash family to be used
* during sign and verify operations.
* Allowed values are "SHA2" and "SHA3".
*
*
* string signature_hash_family = 1;
*/
public Builder setSignatureHashFamilyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
signatureHashFamily_ = value;
onChanged();
return this;
}
private java.lang.Object identityIdentifierHashFunction_ = "";
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public java.lang.String getIdentityIdentifierHashFunction() {
java.lang.Object ref = identityIdentifierHashFunction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identityIdentifierHashFunction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public com.google.protobuf.ByteString
getIdentityIdentifierHashFunctionBytes() {
java.lang.Object ref = identityIdentifierHashFunction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identityIdentifierHashFunction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public Builder setIdentityIdentifierHashFunction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
identityIdentifierHashFunction_ = value;
onChanged();
return this;
}
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public Builder clearIdentityIdentifierHashFunction() {
identityIdentifierHashFunction_ = getDefaultInstance().getIdentityIdentifierHashFunction();
onChanged();
return this;
}
/**
*
* IdentityIdentifierHashFunction is a string representing the hash function
* to be used during the computation of the identity identifier of an MSP identity.
* Allowed values are "SHA256", "SHA384" and "SHA3_256", "SHA3_384".
*
*
* string identity_identifier_hash_function = 2;
*/
public Builder setIdentityIdentifierHashFunctionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
identityIdentifierHashFunction_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.FabricCryptoConfig)
}
// @@protoc_insertion_point(class_scope:msp.FabricCryptoConfig)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FabricCryptoConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FabricCryptoConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricCryptoConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IdemixMSPConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.IdemixMSPConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* ipk represents the (serialized) issuer public key
*
*
* bytes ipk = 2;
*/
com.google.protobuf.ByteString getIpk();
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
boolean hasSigner();
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig getSigner();
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder getSignerOrBuilder();
/**
*
* revocation_pk is the public key used for revocation of credentials
*
*
* bytes revocation_pk = 4;
*/
com.google.protobuf.ByteString getRevocationPk();
/**
*
* epoch represents the current epoch (time interval) used for revocation
*
*
* int64 epoch = 5;
*/
long getEpoch();
}
/**
*
* IdemixMSPConfig collects all the configuration information for
* an Idemix MSP.
*
*
* Protobuf type {@code msp.IdemixMSPConfig}
*/
public static final class IdemixMSPConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.IdemixMSPConfig)
IdemixMSPConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use IdemixMSPConfig.newBuilder() to construct.
private IdemixMSPConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IdemixMSPConfig() {
name_ = "";
ipk_ = com.google.protobuf.ByteString.EMPTY;
revocationPk_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IdemixMSPConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
ipk_ = input.readBytes();
break;
}
case 26: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder subBuilder = null;
if (signer_ != null) {
subBuilder = signer_.toBuilder();
}
signer_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(signer_);
signer_ = subBuilder.buildPartial();
}
break;
}
case 34: {
revocationPk_ = input.readBytes();
break;
}
case 40: {
epoch_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IPK_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString ipk_;
/**
*
* ipk represents the (serialized) issuer public key
*
*
* bytes ipk = 2;
*/
public com.google.protobuf.ByteString getIpk() {
return ipk_;
}
public static final int SIGNER_FIELD_NUMBER = 3;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig signer_;
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public boolean hasSigner() {
return signer_ != null;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig getSigner() {
return signer_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.getDefaultInstance() : signer_;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder getSignerOrBuilder() {
return getSigner();
}
public static final int REVOCATION_PK_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString revocationPk_;
/**
*
* revocation_pk is the public key used for revocation of credentials
*
*
* bytes revocation_pk = 4;
*/
public com.google.protobuf.ByteString getRevocationPk() {
return revocationPk_;
}
public static final int EPOCH_FIELD_NUMBER = 5;
private long epoch_;
/**
*
* epoch represents the current epoch (time interval) used for revocation
*
*
* int64 epoch = 5;
*/
public long getEpoch() {
return epoch_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!ipk_.isEmpty()) {
output.writeBytes(2, ipk_);
}
if (signer_ != null) {
output.writeMessage(3, getSigner());
}
if (!revocationPk_.isEmpty()) {
output.writeBytes(4, revocationPk_);
}
if (epoch_ != 0L) {
output.writeInt64(5, epoch_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!ipk_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, ipk_);
}
if (signer_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSigner());
}
if (!revocationPk_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, revocationPk_);
}
if (epoch_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, epoch_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getIpk()
.equals(other.getIpk())) return false;
if (hasSigner() != other.hasSigner()) return false;
if (hasSigner()) {
if (!getSigner()
.equals(other.getSigner())) return false;
}
if (!getRevocationPk()
.equals(other.getRevocationPk())) return false;
if (getEpoch()
!= other.getEpoch()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + IPK_FIELD_NUMBER;
hash = (53 * hash) + getIpk().hashCode();
if (hasSigner()) {
hash = (37 * hash) + SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getSigner().hashCode();
}
hash = (37 * hash) + REVOCATION_PK_FIELD_NUMBER;
hash = (53 * hash) + getRevocationPk().hashCode();
hash = (37 * hash) + EPOCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEpoch());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* IdemixMSPConfig collects all the configuration information for
* an Idemix MSP.
*
*
* Protobuf type {@code msp.IdemixMSPConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.IdemixMSPConfig)
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
ipk_ = com.google.protobuf.ByteString.EMPTY;
if (signerBuilder_ == null) {
signer_ = null;
} else {
signer_ = null;
signerBuilder_ = null;
}
revocationPk_ = com.google.protobuf.ByteString.EMPTY;
epoch_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPConfig_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig(this);
result.name_ = name_;
result.ipk_ = ipk_;
if (signerBuilder_ == null) {
result.signer_ = signer_;
} else {
result.signer_ = signerBuilder_.build();
}
result.revocationPk_ = revocationPk_;
result.epoch_ = epoch_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.getIpk() != com.google.protobuf.ByteString.EMPTY) {
setIpk(other.getIpk());
}
if (other.hasSigner()) {
mergeSigner(other.getSigner());
}
if (other.getRevocationPk() != com.google.protobuf.ByteString.EMPTY) {
setRevocationPk(other.getRevocationPk());
}
if (other.getEpoch() != 0L) {
setEpoch(other.getEpoch());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name holds the identifier of the MSP
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString ipk_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* ipk represents the (serialized) issuer public key
*
*
* bytes ipk = 2;
*/
public com.google.protobuf.ByteString getIpk() {
return ipk_;
}
/**
*
* ipk represents the (serialized) issuer public key
*
*
* bytes ipk = 2;
*/
public Builder setIpk(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ipk_ = value;
onChanged();
return this;
}
/**
*
* ipk represents the (serialized) issuer public key
*
*
* bytes ipk = 2;
*/
public Builder clearIpk() {
ipk_ = getDefaultInstance().getIpk();
onChanged();
return this;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig signer_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder> signerBuilder_;
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public boolean hasSigner() {
return signerBuilder_ != null || signer_ != null;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig getSigner() {
if (signerBuilder_ == null) {
return signer_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.getDefaultInstance() : signer_;
} else {
return signerBuilder_.getMessage();
}
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public Builder setSigner(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig value) {
if (signerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
signer_ = value;
onChanged();
} else {
signerBuilder_.setMessage(value);
}
return this;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public Builder setSigner(
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder builderForValue) {
if (signerBuilder_ == null) {
signer_ = builderForValue.build();
onChanged();
} else {
signerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public Builder mergeSigner(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig value) {
if (signerBuilder_ == null) {
if (signer_ != null) {
signer_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.newBuilder(signer_).mergeFrom(value).buildPartial();
} else {
signer_ = value;
}
onChanged();
} else {
signerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public Builder clearSigner() {
if (signerBuilder_ == null) {
signer_ = null;
onChanged();
} else {
signer_ = null;
signerBuilder_ = null;
}
return this;
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder getSignerBuilder() {
onChanged();
return getSignerFieldBuilder().getBuilder();
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder getSignerOrBuilder() {
if (signerBuilder_ != null) {
return signerBuilder_.getMessageOrBuilder();
} else {
return signer_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.getDefaultInstance() : signer_;
}
}
/**
*
* signer may contain crypto material to configure a default signer
*
*
* .msp.IdemixMSPSignerConfig signer = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder>
getSignerFieldBuilder() {
if (signerBuilder_ == null) {
signerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder>(
getSigner(),
getParentForChildren(),
isClean());
signer_ = null;
}
return signerBuilder_;
}
private com.google.protobuf.ByteString revocationPk_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* revocation_pk is the public key used for revocation of credentials
*
*
* bytes revocation_pk = 4;
*/
public com.google.protobuf.ByteString getRevocationPk() {
return revocationPk_;
}
/**
*
* revocation_pk is the public key used for revocation of credentials
*
*
* bytes revocation_pk = 4;
*/
public Builder setRevocationPk(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
revocationPk_ = value;
onChanged();
return this;
}
/**
*
* revocation_pk is the public key used for revocation of credentials
*
*
* bytes revocation_pk = 4;
*/
public Builder clearRevocationPk() {
revocationPk_ = getDefaultInstance().getRevocationPk();
onChanged();
return this;
}
private long epoch_ ;
/**
*
* epoch represents the current epoch (time interval) used for revocation
*
*
* int64 epoch = 5;
*/
public long getEpoch() {
return epoch_;
}
/**
*
* epoch represents the current epoch (time interval) used for revocation
*
*
* int64 epoch = 5;
*/
public Builder setEpoch(long value) {
epoch_ = value;
onChanged();
return this;
}
/**
*
* epoch represents the current epoch (time interval) used for revocation
*
*
* int64 epoch = 5;
*/
public Builder clearEpoch() {
epoch_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.IdemixMSPConfig)
}
// @@protoc_insertion_point(class_scope:msp.IdemixMSPConfig)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IdemixMSPConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IdemixMSPConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IdemixMSPSignerConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.IdemixMSPSignerConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
* cred represents the serialized idemix credential of the default signer
*
*
* bytes cred = 1;
*/
com.google.protobuf.ByteString getCred();
/**
*
* sk is the secret key of the default signer, corresponding to credential Cred
*
*
* bytes sk = 2;
*/
com.google.protobuf.ByteString getSk();
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
java.lang.String getOrganizationalUnitIdentifier();
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
com.google.protobuf.ByteString
getOrganizationalUnitIdentifierBytes();
/**
*
* role defines whether the default signer is admin, peer, member or client
*
*
* int32 role = 4;
*/
int getRole();
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
java.lang.String getEnrollmentId();
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
com.google.protobuf.ByteString
getEnrollmentIdBytes();
/**
*
* credential_revocation_information contains a serialized CredentialRevocationInformation
*
*
* bytes credential_revocation_information = 6;
*/
com.google.protobuf.ByteString getCredentialRevocationInformation();
}
/**
*
* IdemixMSPSIgnerConfig contains the crypto material to set up an idemix signing identity
*
*
* Protobuf type {@code msp.IdemixMSPSignerConfig}
*/
public static final class IdemixMSPSignerConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.IdemixMSPSignerConfig)
IdemixMSPSignerConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use IdemixMSPSignerConfig.newBuilder() to construct.
private IdemixMSPSignerConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IdemixMSPSignerConfig() {
cred_ = com.google.protobuf.ByteString.EMPTY;
sk_ = com.google.protobuf.ByteString.EMPTY;
organizationalUnitIdentifier_ = "";
enrollmentId_ = "";
credentialRevocationInformation_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IdemixMSPSignerConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cred_ = input.readBytes();
break;
}
case 18: {
sk_ = input.readBytes();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
organizationalUnitIdentifier_ = s;
break;
}
case 32: {
role_ = input.readInt32();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
enrollmentId_ = s;
break;
}
case 50: {
credentialRevocationInformation_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPSignerConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPSignerConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder.class);
}
public static final int CRED_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString cred_;
/**
*
* cred represents the serialized idemix credential of the default signer
*
*
* bytes cred = 1;
*/
public com.google.protobuf.ByteString getCred() {
return cred_;
}
public static final int SK_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString sk_;
/**
*
* sk is the secret key of the default signer, corresponding to credential Cred
*
*
* bytes sk = 2;
*/
public com.google.protobuf.ByteString getSk() {
return sk_;
}
public static final int ORGANIZATIONAL_UNIT_IDENTIFIER_FIELD_NUMBER = 3;
private volatile java.lang.Object organizationalUnitIdentifier_;
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public java.lang.String getOrganizationalUnitIdentifier() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
organizationalUnitIdentifier_ = s;
return s;
}
}
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public com.google.protobuf.ByteString
getOrganizationalUnitIdentifierBytes() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
organizationalUnitIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROLE_FIELD_NUMBER = 4;
private int role_;
/**
*
* role defines whether the default signer is admin, peer, member or client
*
*
* int32 role = 4;
*/
public int getRole() {
return role_;
}
public static final int ENROLLMENT_ID_FIELD_NUMBER = 5;
private volatile java.lang.Object enrollmentId_;
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public java.lang.String getEnrollmentId() {
java.lang.Object ref = enrollmentId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
enrollmentId_ = s;
return s;
}
}
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public com.google.protobuf.ByteString
getEnrollmentIdBytes() {
java.lang.Object ref = enrollmentId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
enrollmentId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREDENTIAL_REVOCATION_INFORMATION_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString credentialRevocationInformation_;
/**
*
* credential_revocation_information contains a serialized CredentialRevocationInformation
*
*
* bytes credential_revocation_information = 6;
*/
public com.google.protobuf.ByteString getCredentialRevocationInformation() {
return credentialRevocationInformation_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!cred_.isEmpty()) {
output.writeBytes(1, cred_);
}
if (!sk_.isEmpty()) {
output.writeBytes(2, sk_);
}
if (!getOrganizationalUnitIdentifierBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, organizationalUnitIdentifier_);
}
if (role_ != 0) {
output.writeInt32(4, role_);
}
if (!getEnrollmentIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, enrollmentId_);
}
if (!credentialRevocationInformation_.isEmpty()) {
output.writeBytes(6, credentialRevocationInformation_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!cred_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, cred_);
}
if (!sk_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, sk_);
}
if (!getOrganizationalUnitIdentifierBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, organizationalUnitIdentifier_);
}
if (role_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, role_);
}
if (!getEnrollmentIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, enrollmentId_);
}
if (!credentialRevocationInformation_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, credentialRevocationInformation_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig) obj;
if (!getCred()
.equals(other.getCred())) return false;
if (!getSk()
.equals(other.getSk())) return false;
if (!getOrganizationalUnitIdentifier()
.equals(other.getOrganizationalUnitIdentifier())) return false;
if (getRole()
!= other.getRole()) return false;
if (!getEnrollmentId()
.equals(other.getEnrollmentId())) return false;
if (!getCredentialRevocationInformation()
.equals(other.getCredentialRevocationInformation())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CRED_FIELD_NUMBER;
hash = (53 * hash) + getCred().hashCode();
hash = (37 * hash) + SK_FIELD_NUMBER;
hash = (53 * hash) + getSk().hashCode();
hash = (37 * hash) + ORGANIZATIONAL_UNIT_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getOrganizationalUnitIdentifier().hashCode();
hash = (37 * hash) + ROLE_FIELD_NUMBER;
hash = (53 * hash) + getRole();
hash = (37 * hash) + ENROLLMENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getEnrollmentId().hashCode();
hash = (37 * hash) + CREDENTIAL_REVOCATION_INFORMATION_FIELD_NUMBER;
hash = (53 * hash) + getCredentialRevocationInformation().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* IdemixMSPSIgnerConfig contains the crypto material to set up an idemix signing identity
*
*
* Protobuf type {@code msp.IdemixMSPSignerConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.IdemixMSPSignerConfig)
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPSignerConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPSignerConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
cred_ = com.google.protobuf.ByteString.EMPTY;
sk_ = com.google.protobuf.ByteString.EMPTY;
organizationalUnitIdentifier_ = "";
role_ = 0;
enrollmentId_ = "";
credentialRevocationInformation_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_IdemixMSPSignerConfig_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig(this);
result.cred_ = cred_;
result.sk_ = sk_;
result.organizationalUnitIdentifier_ = organizationalUnitIdentifier_;
result.role_ = role_;
result.enrollmentId_ = enrollmentId_;
result.credentialRevocationInformation_ = credentialRevocationInformation_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig.getDefaultInstance()) return this;
if (other.getCred() != com.google.protobuf.ByteString.EMPTY) {
setCred(other.getCred());
}
if (other.getSk() != com.google.protobuf.ByteString.EMPTY) {
setSk(other.getSk());
}
if (!other.getOrganizationalUnitIdentifier().isEmpty()) {
organizationalUnitIdentifier_ = other.organizationalUnitIdentifier_;
onChanged();
}
if (other.getRole() != 0) {
setRole(other.getRole());
}
if (!other.getEnrollmentId().isEmpty()) {
enrollmentId_ = other.enrollmentId_;
onChanged();
}
if (other.getCredentialRevocationInformation() != com.google.protobuf.ByteString.EMPTY) {
setCredentialRevocationInformation(other.getCredentialRevocationInformation());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString cred_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* cred represents the serialized idemix credential of the default signer
*
*
* bytes cred = 1;
*/
public com.google.protobuf.ByteString getCred() {
return cred_;
}
/**
*
* cred represents the serialized idemix credential of the default signer
*
*
* bytes cred = 1;
*/
public Builder setCred(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
cred_ = value;
onChanged();
return this;
}
/**
*
* cred represents the serialized idemix credential of the default signer
*
*
* bytes cred = 1;
*/
public Builder clearCred() {
cred_ = getDefaultInstance().getCred();
onChanged();
return this;
}
private com.google.protobuf.ByteString sk_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* sk is the secret key of the default signer, corresponding to credential Cred
*
*
* bytes sk = 2;
*/
public com.google.protobuf.ByteString getSk() {
return sk_;
}
/**
*
* sk is the secret key of the default signer, corresponding to credential Cred
*
*
* bytes sk = 2;
*/
public Builder setSk(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
sk_ = value;
onChanged();
return this;
}
/**
*
* sk is the secret key of the default signer, corresponding to credential Cred
*
*
* bytes sk = 2;
*/
public Builder clearSk() {
sk_ = getDefaultInstance().getSk();
onChanged();
return this;
}
private java.lang.Object organizationalUnitIdentifier_ = "";
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public java.lang.String getOrganizationalUnitIdentifier() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
organizationalUnitIdentifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public com.google.protobuf.ByteString
getOrganizationalUnitIdentifierBytes() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
organizationalUnitIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public Builder setOrganizationalUnitIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
organizationalUnitIdentifier_ = value;
onChanged();
return this;
}
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public Builder clearOrganizationalUnitIdentifier() {
organizationalUnitIdentifier_ = getDefaultInstance().getOrganizationalUnitIdentifier();
onChanged();
return this;
}
/**
*
* organizational_unit_identifier defines the organizational unit the default signer is in
*
*
* string organizational_unit_identifier = 3;
*/
public Builder setOrganizationalUnitIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
organizationalUnitIdentifier_ = value;
onChanged();
return this;
}
private int role_ ;
/**
*
* role defines whether the default signer is admin, peer, member or client
*
*
* int32 role = 4;
*/
public int getRole() {
return role_;
}
/**
*
* role defines whether the default signer is admin, peer, member or client
*
*
* int32 role = 4;
*/
public Builder setRole(int value) {
role_ = value;
onChanged();
return this;
}
/**
*
* role defines whether the default signer is admin, peer, member or client
*
*
* int32 role = 4;
*/
public Builder clearRole() {
role_ = 0;
onChanged();
return this;
}
private java.lang.Object enrollmentId_ = "";
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public java.lang.String getEnrollmentId() {
java.lang.Object ref = enrollmentId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
enrollmentId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public com.google.protobuf.ByteString
getEnrollmentIdBytes() {
java.lang.Object ref = enrollmentId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
enrollmentId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public Builder setEnrollmentId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
enrollmentId_ = value;
onChanged();
return this;
}
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public Builder clearEnrollmentId() {
enrollmentId_ = getDefaultInstance().getEnrollmentId();
onChanged();
return this;
}
/**
*
* enrollment_id contains the enrollment id of this signer
*
*
* string enrollment_id = 5;
*/
public Builder setEnrollmentIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
enrollmentId_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString credentialRevocationInformation_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* credential_revocation_information contains a serialized CredentialRevocationInformation
*
*
* bytes credential_revocation_information = 6;
*/
public com.google.protobuf.ByteString getCredentialRevocationInformation() {
return credentialRevocationInformation_;
}
/**
*
* credential_revocation_information contains a serialized CredentialRevocationInformation
*
*
* bytes credential_revocation_information = 6;
*/
public Builder setCredentialRevocationInformation(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
credentialRevocationInformation_ = value;
onChanged();
return this;
}
/**
*
* credential_revocation_information contains a serialized CredentialRevocationInformation
*
*
* bytes credential_revocation_information = 6;
*/
public Builder clearCredentialRevocationInformation() {
credentialRevocationInformation_ = getDefaultInstance().getCredentialRevocationInformation();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.IdemixMSPSignerConfig)
}
// @@protoc_insertion_point(class_scope:msp.IdemixMSPSignerConfig)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IdemixMSPSignerConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IdemixMSPSignerConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.IdemixMSPSignerConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SigningIdentityInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.SigningIdentityInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* PublicSigner carries the public information of the signing
* identity. For an X.509 provider this would be represented by
* an X.509 certificate
*
*
* bytes public_signer = 1;
*/
com.google.protobuf.ByteString getPublicSigner();
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
boolean hasPrivateSigner();
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo getPrivateSigner();
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder getPrivateSignerOrBuilder();
}
/**
*
* SigningIdentityInfo represents the configuration information
* related to the signing identity the peer is to use for generating
* endorsements
*
*
* Protobuf type {@code msp.SigningIdentityInfo}
*/
public static final class SigningIdentityInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.SigningIdentityInfo)
SigningIdentityInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use SigningIdentityInfo.newBuilder() to construct.
private SigningIdentityInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SigningIdentityInfo() {
publicSigner_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SigningIdentityInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
publicSigner_ = input.readBytes();
break;
}
case 18: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder subBuilder = null;
if (privateSigner_ != null) {
subBuilder = privateSigner_.toBuilder();
}
privateSigner_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(privateSigner_);
privateSigner_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_SigningIdentityInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_SigningIdentityInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder.class);
}
public static final int PUBLIC_SIGNER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString publicSigner_;
/**
*
* PublicSigner carries the public information of the signing
* identity. For an X.509 provider this would be represented by
* an X.509 certificate
*
*
* bytes public_signer = 1;
*/
public com.google.protobuf.ByteString getPublicSigner() {
return publicSigner_;
}
public static final int PRIVATE_SIGNER_FIELD_NUMBER = 2;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo privateSigner_;
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public boolean hasPrivateSigner() {
return privateSigner_ != null;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo getPrivateSigner() {
return privateSigner_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.getDefaultInstance() : privateSigner_;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder getPrivateSignerOrBuilder() {
return getPrivateSigner();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!publicSigner_.isEmpty()) {
output.writeBytes(1, publicSigner_);
}
if (privateSigner_ != null) {
output.writeMessage(2, getPrivateSigner());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!publicSigner_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, publicSigner_);
}
if (privateSigner_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPrivateSigner());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo) obj;
if (!getPublicSigner()
.equals(other.getPublicSigner())) return false;
if (hasPrivateSigner() != other.hasPrivateSigner()) return false;
if (hasPrivateSigner()) {
if (!getPrivateSigner()
.equals(other.getPrivateSigner())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PUBLIC_SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getPublicSigner().hashCode();
if (hasPrivateSigner()) {
hash = (37 * hash) + PRIVATE_SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getPrivateSigner().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* SigningIdentityInfo represents the configuration information
* related to the signing identity the peer is to use for generating
* endorsements
*
*
* Protobuf type {@code msp.SigningIdentityInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.SigningIdentityInfo)
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_SigningIdentityInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_SigningIdentityInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
publicSigner_ = com.google.protobuf.ByteString.EMPTY;
if (privateSignerBuilder_ == null) {
privateSigner_ = null;
} else {
privateSigner_ = null;
privateSignerBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_SigningIdentityInfo_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo(this);
result.publicSigner_ = publicSigner_;
if (privateSignerBuilder_ == null) {
result.privateSigner_ = privateSigner_;
} else {
result.privateSigner_ = privateSignerBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo.getDefaultInstance()) return this;
if (other.getPublicSigner() != com.google.protobuf.ByteString.EMPTY) {
setPublicSigner(other.getPublicSigner());
}
if (other.hasPrivateSigner()) {
mergePrivateSigner(other.getPrivateSigner());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString publicSigner_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* PublicSigner carries the public information of the signing
* identity. For an X.509 provider this would be represented by
* an X.509 certificate
*
*
* bytes public_signer = 1;
*/
public com.google.protobuf.ByteString getPublicSigner() {
return publicSigner_;
}
/**
*
* PublicSigner carries the public information of the signing
* identity. For an X.509 provider this would be represented by
* an X.509 certificate
*
*
* bytes public_signer = 1;
*/
public Builder setPublicSigner(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
publicSigner_ = value;
onChanged();
return this;
}
/**
*
* PublicSigner carries the public information of the signing
* identity. For an X.509 provider this would be represented by
* an X.509 certificate
*
*
* bytes public_signer = 1;
*/
public Builder clearPublicSigner() {
publicSigner_ = getDefaultInstance().getPublicSigner();
onChanged();
return this;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo privateSigner_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder> privateSignerBuilder_;
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public boolean hasPrivateSigner() {
return privateSignerBuilder_ != null || privateSigner_ != null;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo getPrivateSigner() {
if (privateSignerBuilder_ == null) {
return privateSigner_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.getDefaultInstance() : privateSigner_;
} else {
return privateSignerBuilder_.getMessage();
}
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public Builder setPrivateSigner(org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo value) {
if (privateSignerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
privateSigner_ = value;
onChanged();
} else {
privateSignerBuilder_.setMessage(value);
}
return this;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public Builder setPrivateSigner(
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder builderForValue) {
if (privateSignerBuilder_ == null) {
privateSigner_ = builderForValue.build();
onChanged();
} else {
privateSignerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public Builder mergePrivateSigner(org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo value) {
if (privateSignerBuilder_ == null) {
if (privateSigner_ != null) {
privateSigner_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.newBuilder(privateSigner_).mergeFrom(value).buildPartial();
} else {
privateSigner_ = value;
}
onChanged();
} else {
privateSignerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public Builder clearPrivateSigner() {
if (privateSignerBuilder_ == null) {
privateSigner_ = null;
onChanged();
} else {
privateSigner_ = null;
privateSignerBuilder_ = null;
}
return this;
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder getPrivateSignerBuilder() {
onChanged();
return getPrivateSignerFieldBuilder().getBuilder();
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder getPrivateSignerOrBuilder() {
if (privateSignerBuilder_ != null) {
return privateSignerBuilder_.getMessageOrBuilder();
} else {
return privateSigner_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.getDefaultInstance() : privateSigner_;
}
}
/**
*
* PrivateSigner denotes a reference to the private key of the
* peer's signing identity
*
*
* .msp.KeyInfo private_signer = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder>
getPrivateSignerFieldBuilder() {
if (privateSignerBuilder_ == null) {
privateSignerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder>(
getPrivateSigner(),
getParentForChildren(),
isClean());
privateSigner_ = null;
}
return privateSignerBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.SigningIdentityInfo)
}
// @@protoc_insertion_point(class_scope:msp.SigningIdentityInfo)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SigningIdentityInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SigningIdentityInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.SigningIdentityInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KeyInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.KeyInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
java.lang.String getKeyIdentifier();
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
com.google.protobuf.ByteString
getKeyIdentifierBytes();
/**
*
* KeyMaterial (optional) for the key to be imported; this is
* properly encoded key bytes, prefixed by the type of the key
*
*
* bytes key_material = 2;
*/
com.google.protobuf.ByteString getKeyMaterial();
}
/**
*
* KeyInfo represents a (secret) key that is either already stored
* in the bccsp/keystore or key material to be imported to the
* bccsp key-store. In later versions it may contain also a
* keystore identifier
*
*
* Protobuf type {@code msp.KeyInfo}
*/
public static final class KeyInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.KeyInfo)
KeyInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeyInfo.newBuilder() to construct.
private KeyInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeyInfo() {
keyIdentifier_ = "";
keyMaterial_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeyInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
keyIdentifier_ = s;
break;
}
case 18: {
keyMaterial_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_KeyInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_KeyInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder.class);
}
public static final int KEY_IDENTIFIER_FIELD_NUMBER = 1;
private volatile java.lang.Object keyIdentifier_;
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public java.lang.String getKeyIdentifier() {
java.lang.Object ref = keyIdentifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
keyIdentifier_ = s;
return s;
}
}
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public com.google.protobuf.ByteString
getKeyIdentifierBytes() {
java.lang.Object ref = keyIdentifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
keyIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_MATERIAL_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString keyMaterial_;
/**
*
* KeyMaterial (optional) for the key to be imported; this is
* properly encoded key bytes, prefixed by the type of the key
*
*
* bytes key_material = 2;
*/
public com.google.protobuf.ByteString getKeyMaterial() {
return keyMaterial_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getKeyIdentifierBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, keyIdentifier_);
}
if (!keyMaterial_.isEmpty()) {
output.writeBytes(2, keyMaterial_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getKeyIdentifierBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, keyIdentifier_);
}
if (!keyMaterial_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, keyMaterial_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo) obj;
if (!getKeyIdentifier()
.equals(other.getKeyIdentifier())) return false;
if (!getKeyMaterial()
.equals(other.getKeyMaterial())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getKeyIdentifier().hashCode();
hash = (37 * hash) + KEY_MATERIAL_FIELD_NUMBER;
hash = (53 * hash) + getKeyMaterial().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* KeyInfo represents a (secret) key that is either already stored
* in the bccsp/keystore or key material to be imported to the
* bccsp key-store. In later versions it may contain also a
* keystore identifier
*
*
* Protobuf type {@code msp.KeyInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.KeyInfo)
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_KeyInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_KeyInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
keyIdentifier_ = "";
keyMaterial_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_KeyInfo_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo(this);
result.keyIdentifier_ = keyIdentifier_;
result.keyMaterial_ = keyMaterial_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo.getDefaultInstance()) return this;
if (!other.getKeyIdentifier().isEmpty()) {
keyIdentifier_ = other.keyIdentifier_;
onChanged();
}
if (other.getKeyMaterial() != com.google.protobuf.ByteString.EMPTY) {
setKeyMaterial(other.getKeyMaterial());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object keyIdentifier_ = "";
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public java.lang.String getKeyIdentifier() {
java.lang.Object ref = keyIdentifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
keyIdentifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public com.google.protobuf.ByteString
getKeyIdentifierBytes() {
java.lang.Object ref = keyIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
keyIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public Builder setKeyIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
keyIdentifier_ = value;
onChanged();
return this;
}
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public Builder clearKeyIdentifier() {
keyIdentifier_ = getDefaultInstance().getKeyIdentifier();
onChanged();
return this;
}
/**
*
* Identifier of the key inside the default keystore; this for
* the case of Software BCCSP as well as the HSM BCCSP would be
* the SKI of the key
*
*
* string key_identifier = 1;
*/
public Builder setKeyIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
keyIdentifier_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString keyMaterial_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* KeyMaterial (optional) for the key to be imported; this is
* properly encoded key bytes, prefixed by the type of the key
*
*
* bytes key_material = 2;
*/
public com.google.protobuf.ByteString getKeyMaterial() {
return keyMaterial_;
}
/**
*
* KeyMaterial (optional) for the key to be imported; this is
* properly encoded key bytes, prefixed by the type of the key
*
*
* bytes key_material = 2;
*/
public Builder setKeyMaterial(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
keyMaterial_ = value;
onChanged();
return this;
}
/**
*
* KeyMaterial (optional) for the key to be imported; this is
* properly encoded key bytes, prefixed by the type of the key
*
*
* bytes key_material = 2;
*/
public Builder clearKeyMaterial() {
keyMaterial_ = getDefaultInstance().getKeyMaterial();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.KeyInfo)
}
// @@protoc_insertion_point(class_scope:msp.KeyInfo)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeyInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeyInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.KeyInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FabricOUIdentifierOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.FabricOUIdentifier)
com.google.protobuf.MessageOrBuilder {
/**
*
* Certificate represents the second certificate in a certification chain.
* (Notice that the first certificate in a certification chain is supposed
* to be the certificate of an identity).
* It must correspond to the certificate of root or intermediate CA
* recognized by the MSP this message belongs to.
* Starting from this certificate, a certification chain is computed
* and bound to the OrganizationUnitIdentifier specified
*
*
* bytes certificate = 1;
*/
com.google.protobuf.ByteString getCertificate();
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
java.lang.String getOrganizationalUnitIdentifier();
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
com.google.protobuf.ByteString
getOrganizationalUnitIdentifierBytes();
}
/**
*
* FabricOUIdentifier represents an organizational unit and
* its related chain of trust identifier.
*
*
* Protobuf type {@code msp.FabricOUIdentifier}
*/
public static final class FabricOUIdentifier extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.FabricOUIdentifier)
FabricOUIdentifierOrBuilder {
private static final long serialVersionUID = 0L;
// Use FabricOUIdentifier.newBuilder() to construct.
private FabricOUIdentifier(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FabricOUIdentifier() {
certificate_ = com.google.protobuf.ByteString.EMPTY;
organizationalUnitIdentifier_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FabricOUIdentifier(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
certificate_ = input.readBytes();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
organizationalUnitIdentifier_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricOUIdentifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricOUIdentifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder.class);
}
public static final int CERTIFICATE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString certificate_;
/**
*
* Certificate represents the second certificate in a certification chain.
* (Notice that the first certificate in a certification chain is supposed
* to be the certificate of an identity).
* It must correspond to the certificate of root or intermediate CA
* recognized by the MSP this message belongs to.
* Starting from this certificate, a certification chain is computed
* and bound to the OrganizationUnitIdentifier specified
*
*
* bytes certificate = 1;
*/
public com.google.protobuf.ByteString getCertificate() {
return certificate_;
}
public static final int ORGANIZATIONAL_UNIT_IDENTIFIER_FIELD_NUMBER = 2;
private volatile java.lang.Object organizationalUnitIdentifier_;
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public java.lang.String getOrganizationalUnitIdentifier() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
organizationalUnitIdentifier_ = s;
return s;
}
}
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public com.google.protobuf.ByteString
getOrganizationalUnitIdentifierBytes() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
organizationalUnitIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!certificate_.isEmpty()) {
output.writeBytes(1, certificate_);
}
if (!getOrganizationalUnitIdentifierBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, organizationalUnitIdentifier_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!certificate_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, certificate_);
}
if (!getOrganizationalUnitIdentifierBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, organizationalUnitIdentifier_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier) obj;
if (!getCertificate()
.equals(other.getCertificate())) return false;
if (!getOrganizationalUnitIdentifier()
.equals(other.getOrganizationalUnitIdentifier())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CERTIFICATE_FIELD_NUMBER;
hash = (53 * hash) + getCertificate().hashCode();
hash = (37 * hash) + ORGANIZATIONAL_UNIT_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getOrganizationalUnitIdentifier().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* FabricOUIdentifier represents an organizational unit and
* its related chain of trust identifier.
*
*
* Protobuf type {@code msp.FabricOUIdentifier}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.FabricOUIdentifier)
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricOUIdentifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricOUIdentifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
certificate_ = com.google.protobuf.ByteString.EMPTY;
organizationalUnitIdentifier_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricOUIdentifier_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier(this);
result.certificate_ = certificate_;
result.organizationalUnitIdentifier_ = organizationalUnitIdentifier_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance()) return this;
if (other.getCertificate() != com.google.protobuf.ByteString.EMPTY) {
setCertificate(other.getCertificate());
}
if (!other.getOrganizationalUnitIdentifier().isEmpty()) {
organizationalUnitIdentifier_ = other.organizationalUnitIdentifier_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString certificate_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Certificate represents the second certificate in a certification chain.
* (Notice that the first certificate in a certification chain is supposed
* to be the certificate of an identity).
* It must correspond to the certificate of root or intermediate CA
* recognized by the MSP this message belongs to.
* Starting from this certificate, a certification chain is computed
* and bound to the OrganizationUnitIdentifier specified
*
*
* bytes certificate = 1;
*/
public com.google.protobuf.ByteString getCertificate() {
return certificate_;
}
/**
*
* Certificate represents the second certificate in a certification chain.
* (Notice that the first certificate in a certification chain is supposed
* to be the certificate of an identity).
* It must correspond to the certificate of root or intermediate CA
* recognized by the MSP this message belongs to.
* Starting from this certificate, a certification chain is computed
* and bound to the OrganizationUnitIdentifier specified
*
*
* bytes certificate = 1;
*/
public Builder setCertificate(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
certificate_ = value;
onChanged();
return this;
}
/**
*
* Certificate represents the second certificate in a certification chain.
* (Notice that the first certificate in a certification chain is supposed
* to be the certificate of an identity).
* It must correspond to the certificate of root or intermediate CA
* recognized by the MSP this message belongs to.
* Starting from this certificate, a certification chain is computed
* and bound to the OrganizationUnitIdentifier specified
*
*
* bytes certificate = 1;
*/
public Builder clearCertificate() {
certificate_ = getDefaultInstance().getCertificate();
onChanged();
return this;
}
private java.lang.Object organizationalUnitIdentifier_ = "";
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public java.lang.String getOrganizationalUnitIdentifier() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
organizationalUnitIdentifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public com.google.protobuf.ByteString
getOrganizationalUnitIdentifierBytes() {
java.lang.Object ref = organizationalUnitIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
organizationalUnitIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public Builder setOrganizationalUnitIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
organizationalUnitIdentifier_ = value;
onChanged();
return this;
}
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public Builder clearOrganizationalUnitIdentifier() {
organizationalUnitIdentifier_ = getDefaultInstance().getOrganizationalUnitIdentifier();
onChanged();
return this;
}
/**
*
* OrganizationUnitIdentifier defines the organizational unit under the
* MSP identified with MSPIdentifier
*
*
* string organizational_unit_identifier = 2;
*/
public Builder setOrganizationalUnitIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
organizationalUnitIdentifier_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.FabricOUIdentifier)
}
// @@protoc_insertion_point(class_scope:msp.FabricOUIdentifier)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FabricOUIdentifier parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FabricOUIdentifier(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FabricNodeOUsOrBuilder extends
// @@protoc_insertion_point(interface_extends:msp.FabricNodeOUs)
com.google.protobuf.MessageOrBuilder {
/**
*
* If true then an msp identity that does not contain any of the specified OU will be considered invalid.
*
*
* bool enable = 1;
*/
boolean getEnable();
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
boolean hasClientOuIdentifier();
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getClientOuIdentifier();
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getClientOuIdentifierOrBuilder();
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
boolean hasPeerOuIdentifier();
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getPeerOuIdentifier();
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getPeerOuIdentifierOrBuilder();
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
boolean hasAdminOuIdentifier();
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getAdminOuIdentifier();
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getAdminOuIdentifierOrBuilder();
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
boolean hasOrdererOuIdentifier();
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getOrdererOuIdentifier();
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getOrdererOuIdentifierOrBuilder();
}
/**
*
* FabricNodeOUs contains configuration to tell apart clients from peers from orderers
* based on OUs. If NodeOUs recognition is enabled then an msp identity
* that does not contain any of the specified OU will be considered invalid.
*
*
* Protobuf type {@code msp.FabricNodeOUs}
*/
public static final class FabricNodeOUs extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:msp.FabricNodeOUs)
FabricNodeOUsOrBuilder {
private static final long serialVersionUID = 0L;
// Use FabricNodeOUs.newBuilder() to construct.
private FabricNodeOUs(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FabricNodeOUs() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FabricNodeOUs(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
enable_ = input.readBool();
break;
}
case 18: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder subBuilder = null;
if (clientOuIdentifier_ != null) {
subBuilder = clientOuIdentifier_.toBuilder();
}
clientOuIdentifier_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientOuIdentifier_);
clientOuIdentifier_ = subBuilder.buildPartial();
}
break;
}
case 26: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder subBuilder = null;
if (peerOuIdentifier_ != null) {
subBuilder = peerOuIdentifier_.toBuilder();
}
peerOuIdentifier_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(peerOuIdentifier_);
peerOuIdentifier_ = subBuilder.buildPartial();
}
break;
}
case 34: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder subBuilder = null;
if (adminOuIdentifier_ != null) {
subBuilder = adminOuIdentifier_.toBuilder();
}
adminOuIdentifier_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(adminOuIdentifier_);
adminOuIdentifier_ = subBuilder.buildPartial();
}
break;
}
case 42: {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder subBuilder = null;
if (ordererOuIdentifier_ != null) {
subBuilder = ordererOuIdentifier_.toBuilder();
}
ordererOuIdentifier_ = input.readMessage(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ordererOuIdentifier_);
ordererOuIdentifier_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricNodeOUs_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricNodeOUs_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder.class);
}
public static final int ENABLE_FIELD_NUMBER = 1;
private boolean enable_;
/**
*
* If true then an msp identity that does not contain any of the specified OU will be considered invalid.
*
*
* bool enable = 1;
*/
public boolean getEnable() {
return enable_;
}
public static final int CLIENT_OU_IDENTIFIER_FIELD_NUMBER = 2;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier clientOuIdentifier_;
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public boolean hasClientOuIdentifier() {
return clientOuIdentifier_ != null;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getClientOuIdentifier() {
return clientOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : clientOuIdentifier_;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getClientOuIdentifierOrBuilder() {
return getClientOuIdentifier();
}
public static final int PEER_OU_IDENTIFIER_FIELD_NUMBER = 3;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier peerOuIdentifier_;
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public boolean hasPeerOuIdentifier() {
return peerOuIdentifier_ != null;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getPeerOuIdentifier() {
return peerOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : peerOuIdentifier_;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getPeerOuIdentifierOrBuilder() {
return getPeerOuIdentifier();
}
public static final int ADMIN_OU_IDENTIFIER_FIELD_NUMBER = 4;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier adminOuIdentifier_;
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public boolean hasAdminOuIdentifier() {
return adminOuIdentifier_ != null;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getAdminOuIdentifier() {
return adminOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : adminOuIdentifier_;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getAdminOuIdentifierOrBuilder() {
return getAdminOuIdentifier();
}
public static final int ORDERER_OU_IDENTIFIER_FIELD_NUMBER = 5;
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier ordererOuIdentifier_;
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public boolean hasOrdererOuIdentifier() {
return ordererOuIdentifier_ != null;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getOrdererOuIdentifier() {
return ordererOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : ordererOuIdentifier_;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getOrdererOuIdentifierOrBuilder() {
return getOrdererOuIdentifier();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (enable_ != false) {
output.writeBool(1, enable_);
}
if (clientOuIdentifier_ != null) {
output.writeMessage(2, getClientOuIdentifier());
}
if (peerOuIdentifier_ != null) {
output.writeMessage(3, getPeerOuIdentifier());
}
if (adminOuIdentifier_ != null) {
output.writeMessage(4, getAdminOuIdentifier());
}
if (ordererOuIdentifier_ != null) {
output.writeMessage(5, getOrdererOuIdentifier());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (enable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, enable_);
}
if (clientOuIdentifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getClientOuIdentifier());
}
if (peerOuIdentifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPeerOuIdentifier());
}
if (adminOuIdentifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getAdminOuIdentifier());
}
if (ordererOuIdentifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getOrdererOuIdentifier());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs)) {
return super.equals(obj);
}
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs other = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs) obj;
if (getEnable()
!= other.getEnable()) return false;
if (hasClientOuIdentifier() != other.hasClientOuIdentifier()) return false;
if (hasClientOuIdentifier()) {
if (!getClientOuIdentifier()
.equals(other.getClientOuIdentifier())) return false;
}
if (hasPeerOuIdentifier() != other.hasPeerOuIdentifier()) return false;
if (hasPeerOuIdentifier()) {
if (!getPeerOuIdentifier()
.equals(other.getPeerOuIdentifier())) return false;
}
if (hasAdminOuIdentifier() != other.hasAdminOuIdentifier()) return false;
if (hasAdminOuIdentifier()) {
if (!getAdminOuIdentifier()
.equals(other.getAdminOuIdentifier())) return false;
}
if (hasOrdererOuIdentifier() != other.hasOrdererOuIdentifier()) return false;
if (hasOrdererOuIdentifier()) {
if (!getOrdererOuIdentifier()
.equals(other.getOrdererOuIdentifier())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ENABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEnable());
if (hasClientOuIdentifier()) {
hash = (37 * hash) + CLIENT_OU_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getClientOuIdentifier().hashCode();
}
if (hasPeerOuIdentifier()) {
hash = (37 * hash) + PEER_OU_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getPeerOuIdentifier().hashCode();
}
if (hasAdminOuIdentifier()) {
hash = (37 * hash) + ADMIN_OU_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getAdminOuIdentifier().hashCode();
}
if (hasOrdererOuIdentifier()) {
hash = (37 * hash) + ORDERER_OU_IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getOrdererOuIdentifier().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* FabricNodeOUs contains configuration to tell apart clients from peers from orderers
* based on OUs. If NodeOUs recognition is enabled then an msp identity
* that does not contain any of the specified OU will be considered invalid.
*
*
* Protobuf type {@code msp.FabricNodeOUs}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:msp.FabricNodeOUs)
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricNodeOUs_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricNodeOUs_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.class, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.Builder.class);
}
// Construct using org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
enable_ = false;
if (clientOuIdentifierBuilder_ == null) {
clientOuIdentifier_ = null;
} else {
clientOuIdentifier_ = null;
clientOuIdentifierBuilder_ = null;
}
if (peerOuIdentifierBuilder_ == null) {
peerOuIdentifier_ = null;
} else {
peerOuIdentifier_ = null;
peerOuIdentifierBuilder_ = null;
}
if (adminOuIdentifierBuilder_ == null) {
adminOuIdentifier_ = null;
} else {
adminOuIdentifier_ = null;
adminOuIdentifierBuilder_ = null;
}
if (ordererOuIdentifierBuilder_ == null) {
ordererOuIdentifier_ = null;
} else {
ordererOuIdentifier_ = null;
ordererOuIdentifierBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.internal_static_msp_FabricNodeOUs_descriptor;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs getDefaultInstanceForType() {
return org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.getDefaultInstance();
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs build() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs buildPartial() {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs result = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs(this);
result.enable_ = enable_;
if (clientOuIdentifierBuilder_ == null) {
result.clientOuIdentifier_ = clientOuIdentifier_;
} else {
result.clientOuIdentifier_ = clientOuIdentifierBuilder_.build();
}
if (peerOuIdentifierBuilder_ == null) {
result.peerOuIdentifier_ = peerOuIdentifier_;
} else {
result.peerOuIdentifier_ = peerOuIdentifierBuilder_.build();
}
if (adminOuIdentifierBuilder_ == null) {
result.adminOuIdentifier_ = adminOuIdentifier_;
} else {
result.adminOuIdentifier_ = adminOuIdentifierBuilder_.build();
}
if (ordererOuIdentifierBuilder_ == null) {
result.ordererOuIdentifier_ = ordererOuIdentifier_;
} else {
result.ordererOuIdentifier_ = ordererOuIdentifierBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs) {
return mergeFrom((org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs other) {
if (other == org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs.getDefaultInstance()) return this;
if (other.getEnable() != false) {
setEnable(other.getEnable());
}
if (other.hasClientOuIdentifier()) {
mergeClientOuIdentifier(other.getClientOuIdentifier());
}
if (other.hasPeerOuIdentifier()) {
mergePeerOuIdentifier(other.getPeerOuIdentifier());
}
if (other.hasAdminOuIdentifier()) {
mergeAdminOuIdentifier(other.getAdminOuIdentifier());
}
if (other.hasOrdererOuIdentifier()) {
mergeOrdererOuIdentifier(other.getOrdererOuIdentifier());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean enable_ ;
/**
*
* If true then an msp identity that does not contain any of the specified OU will be considered invalid.
*
*
* bool enable = 1;
*/
public boolean getEnable() {
return enable_;
}
/**
*
* If true then an msp identity that does not contain any of the specified OU will be considered invalid.
*
*
* bool enable = 1;
*/
public Builder setEnable(boolean value) {
enable_ = value;
onChanged();
return this;
}
/**
*
* If true then an msp identity that does not contain any of the specified OU will be considered invalid.
*
*
* bool enable = 1;
*/
public Builder clearEnable() {
enable_ = false;
onChanged();
return this;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier clientOuIdentifier_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder> clientOuIdentifierBuilder_;
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public boolean hasClientOuIdentifier() {
return clientOuIdentifierBuilder_ != null || clientOuIdentifier_ != null;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getClientOuIdentifier() {
if (clientOuIdentifierBuilder_ == null) {
return clientOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : clientOuIdentifier_;
} else {
return clientOuIdentifierBuilder_.getMessage();
}
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public Builder setClientOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (clientOuIdentifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientOuIdentifier_ = value;
onChanged();
} else {
clientOuIdentifierBuilder_.setMessage(value);
}
return this;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public Builder setClientOuIdentifier(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (clientOuIdentifierBuilder_ == null) {
clientOuIdentifier_ = builderForValue.build();
onChanged();
} else {
clientOuIdentifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public Builder mergeClientOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (clientOuIdentifierBuilder_ == null) {
if (clientOuIdentifier_ != null) {
clientOuIdentifier_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.newBuilder(clientOuIdentifier_).mergeFrom(value).buildPartial();
} else {
clientOuIdentifier_ = value;
}
onChanged();
} else {
clientOuIdentifierBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public Builder clearClientOuIdentifier() {
if (clientOuIdentifierBuilder_ == null) {
clientOuIdentifier_ = null;
onChanged();
} else {
clientOuIdentifier_ = null;
clientOuIdentifierBuilder_ = null;
}
return this;
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder getClientOuIdentifierBuilder() {
onChanged();
return getClientOuIdentifierFieldBuilder().getBuilder();
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getClientOuIdentifierOrBuilder() {
if (clientOuIdentifierBuilder_ != null) {
return clientOuIdentifierBuilder_.getMessageOrBuilder();
} else {
return clientOuIdentifier_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : clientOuIdentifier_;
}
}
/**
*
* OU Identifier of the clients
*
*
* .msp.FabricOUIdentifier client_ou_identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getClientOuIdentifierFieldBuilder() {
if (clientOuIdentifierBuilder_ == null) {
clientOuIdentifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>(
getClientOuIdentifier(),
getParentForChildren(),
isClean());
clientOuIdentifier_ = null;
}
return clientOuIdentifierBuilder_;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier peerOuIdentifier_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder> peerOuIdentifierBuilder_;
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public boolean hasPeerOuIdentifier() {
return peerOuIdentifierBuilder_ != null || peerOuIdentifier_ != null;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getPeerOuIdentifier() {
if (peerOuIdentifierBuilder_ == null) {
return peerOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : peerOuIdentifier_;
} else {
return peerOuIdentifierBuilder_.getMessage();
}
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public Builder setPeerOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (peerOuIdentifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
peerOuIdentifier_ = value;
onChanged();
} else {
peerOuIdentifierBuilder_.setMessage(value);
}
return this;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public Builder setPeerOuIdentifier(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (peerOuIdentifierBuilder_ == null) {
peerOuIdentifier_ = builderForValue.build();
onChanged();
} else {
peerOuIdentifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public Builder mergePeerOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (peerOuIdentifierBuilder_ == null) {
if (peerOuIdentifier_ != null) {
peerOuIdentifier_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.newBuilder(peerOuIdentifier_).mergeFrom(value).buildPartial();
} else {
peerOuIdentifier_ = value;
}
onChanged();
} else {
peerOuIdentifierBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public Builder clearPeerOuIdentifier() {
if (peerOuIdentifierBuilder_ == null) {
peerOuIdentifier_ = null;
onChanged();
} else {
peerOuIdentifier_ = null;
peerOuIdentifierBuilder_ = null;
}
return this;
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder getPeerOuIdentifierBuilder() {
onChanged();
return getPeerOuIdentifierFieldBuilder().getBuilder();
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getPeerOuIdentifierOrBuilder() {
if (peerOuIdentifierBuilder_ != null) {
return peerOuIdentifierBuilder_.getMessageOrBuilder();
} else {
return peerOuIdentifier_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : peerOuIdentifier_;
}
}
/**
*
* OU Identifier of the peers
*
*
* .msp.FabricOUIdentifier peer_ou_identifier = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getPeerOuIdentifierFieldBuilder() {
if (peerOuIdentifierBuilder_ == null) {
peerOuIdentifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>(
getPeerOuIdentifier(),
getParentForChildren(),
isClean());
peerOuIdentifier_ = null;
}
return peerOuIdentifierBuilder_;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier adminOuIdentifier_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder> adminOuIdentifierBuilder_;
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public boolean hasAdminOuIdentifier() {
return adminOuIdentifierBuilder_ != null || adminOuIdentifier_ != null;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getAdminOuIdentifier() {
if (adminOuIdentifierBuilder_ == null) {
return adminOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : adminOuIdentifier_;
} else {
return adminOuIdentifierBuilder_.getMessage();
}
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public Builder setAdminOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (adminOuIdentifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
adminOuIdentifier_ = value;
onChanged();
} else {
adminOuIdentifierBuilder_.setMessage(value);
}
return this;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public Builder setAdminOuIdentifier(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (adminOuIdentifierBuilder_ == null) {
adminOuIdentifier_ = builderForValue.build();
onChanged();
} else {
adminOuIdentifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public Builder mergeAdminOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (adminOuIdentifierBuilder_ == null) {
if (adminOuIdentifier_ != null) {
adminOuIdentifier_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.newBuilder(adminOuIdentifier_).mergeFrom(value).buildPartial();
} else {
adminOuIdentifier_ = value;
}
onChanged();
} else {
adminOuIdentifierBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public Builder clearAdminOuIdentifier() {
if (adminOuIdentifierBuilder_ == null) {
adminOuIdentifier_ = null;
onChanged();
} else {
adminOuIdentifier_ = null;
adminOuIdentifierBuilder_ = null;
}
return this;
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder getAdminOuIdentifierBuilder() {
onChanged();
return getAdminOuIdentifierFieldBuilder().getBuilder();
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getAdminOuIdentifierOrBuilder() {
if (adminOuIdentifierBuilder_ != null) {
return adminOuIdentifierBuilder_.getMessageOrBuilder();
} else {
return adminOuIdentifier_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : adminOuIdentifier_;
}
}
/**
*
* OU Identifier of the admins
*
*
* .msp.FabricOUIdentifier admin_ou_identifier = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getAdminOuIdentifierFieldBuilder() {
if (adminOuIdentifierBuilder_ == null) {
adminOuIdentifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>(
getAdminOuIdentifier(),
getParentForChildren(),
isClean());
adminOuIdentifier_ = null;
}
return adminOuIdentifierBuilder_;
}
private org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier ordererOuIdentifier_;
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder> ordererOuIdentifierBuilder_;
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public boolean hasOrdererOuIdentifier() {
return ordererOuIdentifierBuilder_ != null || ordererOuIdentifier_ != null;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier getOrdererOuIdentifier() {
if (ordererOuIdentifierBuilder_ == null) {
return ordererOuIdentifier_ == null ? org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : ordererOuIdentifier_;
} else {
return ordererOuIdentifierBuilder_.getMessage();
}
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public Builder setOrdererOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (ordererOuIdentifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ordererOuIdentifier_ = value;
onChanged();
} else {
ordererOuIdentifierBuilder_.setMessage(value);
}
return this;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public Builder setOrdererOuIdentifier(
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder builderForValue) {
if (ordererOuIdentifierBuilder_ == null) {
ordererOuIdentifier_ = builderForValue.build();
onChanged();
} else {
ordererOuIdentifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public Builder mergeOrdererOuIdentifier(org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier value) {
if (ordererOuIdentifierBuilder_ == null) {
if (ordererOuIdentifier_ != null) {
ordererOuIdentifier_ =
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.newBuilder(ordererOuIdentifier_).mergeFrom(value).buildPartial();
} else {
ordererOuIdentifier_ = value;
}
onChanged();
} else {
ordererOuIdentifierBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public Builder clearOrdererOuIdentifier() {
if (ordererOuIdentifierBuilder_ == null) {
ordererOuIdentifier_ = null;
onChanged();
} else {
ordererOuIdentifier_ = null;
ordererOuIdentifierBuilder_ = null;
}
return this;
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder getOrdererOuIdentifierBuilder() {
onChanged();
return getOrdererOuIdentifierFieldBuilder().getBuilder();
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder getOrdererOuIdentifierOrBuilder() {
if (ordererOuIdentifierBuilder_ != null) {
return ordererOuIdentifierBuilder_.getMessageOrBuilder();
} else {
return ordererOuIdentifier_ == null ?
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.getDefaultInstance() : ordererOuIdentifier_;
}
}
/**
*
* OU Identifier of the orderers
*
*
* .msp.FabricOUIdentifier orderer_ou_identifier = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>
getOrdererOuIdentifierFieldBuilder() {
if (ordererOuIdentifierBuilder_ == null) {
ordererOuIdentifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifier.Builder, org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricOUIdentifierOrBuilder>(
getOrdererOuIdentifier(),
getParentForChildren(),
isClean());
ordererOuIdentifier_ = null;
}
return ordererOuIdentifierBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:msp.FabricNodeOUs)
}
// @@protoc_insertion_point(class_scope:msp.FabricNodeOUs)
private static final org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs();
}
public static org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FabricNodeOUs parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FabricNodeOUs(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.hyperledger.fabric.protos.msp.MspConfigPackage.FabricNodeOUs getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_MSPConfig_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_MSPConfig_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_FabricMSPConfig_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_FabricMSPConfig_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_FabricCryptoConfig_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_FabricCryptoConfig_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_IdemixMSPConfig_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_IdemixMSPConfig_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_IdemixMSPSignerConfig_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_IdemixMSPSignerConfig_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_SigningIdentityInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_SigningIdentityInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_KeyInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_KeyInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_FabricOUIdentifier_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_FabricOUIdentifier_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_msp_FabricNodeOUs_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_msp_FabricNodeOUs_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024msp/msp_config.proto\022\003msp\")\n\tMSPConfig" +
"\022\014\n\004type\030\001 \001(\005\022\016\n\006config\030\002 \001(\014\"\203\003\n\017Fabri" +
"cMSPConfig\022\014\n\004name\030\001 \001(\t\022\022\n\nroot_certs\030\002" +
" \003(\014\022\032\n\022intermediate_certs\030\003 \003(\014\022\016\n\006admi" +
"ns\030\004 \003(\014\022\027\n\017revocation_list\030\005 \003(\014\0222\n\020sig" +
"ning_identity\030\006 \001(\0132\030.msp.SigningIdentit" +
"yInfo\022@\n\037organizational_unit_identifiers" +
"\030\007 \003(\0132\027.msp.FabricOUIdentifier\022.\n\rcrypt" +
"o_config\030\010 \001(\0132\027.msp.FabricCryptoConfig\022" +
"\026\n\016tls_root_certs\030\t \003(\014\022\036\n\026tls_intermedi" +
"ate_certs\030\n \003(\014\022+\n\017fabric_node_ous\030\013 \001(\013" +
"2\022.msp.FabricNodeOUs\"^\n\022FabricCryptoConf" +
"ig\022\035\n\025signature_hash_family\030\001 \001(\t\022)\n!ide" +
"ntity_identifier_hash_function\030\002 \001(\t\"~\n\017" +
"IdemixMSPConfig\022\014\n\004name\030\001 \001(\t\022\013\n\003ipk\030\002 \001" +
"(\014\022*\n\006signer\030\003 \001(\0132\032.msp.IdemixMSPSigner" +
"Config\022\025\n\rrevocation_pk\030\004 \001(\014\022\r\n\005epoch\030\005" +
" \001(\003\"\251\001\n\025IdemixMSPSignerConfig\022\014\n\004cred\030\001" +
" \001(\014\022\n\n\002sk\030\002 \001(\014\022&\n\036organizational_unit_" +
"identifier\030\003 \001(\t\022\014\n\004role\030\004 \001(\005\022\025\n\renroll" +
"ment_id\030\005 \001(\t\022)\n!credential_revocation_i" +
"nformation\030\006 \001(\014\"R\n\023SigningIdentityInfo\022" +
"\025\n\rpublic_signer\030\001 \001(\014\022$\n\016private_signer" +
"\030\002 \001(\0132\014.msp.KeyInfo\"7\n\007KeyInfo\022\026\n\016key_i" +
"dentifier\030\001 \001(\t\022\024\n\014key_material\030\002 \001(\014\"Q\n" +
"\022FabricOUIdentifier\022\023\n\013certificate\030\001 \001(\014" +
"\022&\n\036organizational_unit_identifier\030\002 \001(\t" +
"\"\371\001\n\rFabricNodeOUs\022\016\n\006enable\030\001 \001(\010\0225\n\024cl" +
"ient_ou_identifier\030\002 \001(\0132\027.msp.FabricOUI" +
"dentifier\0223\n\022peer_ou_identifier\030\003 \001(\0132\027." +
"msp.FabricOUIdentifier\0224\n\023admin_ou_ident" +
"ifier\030\004 \001(\0132\027.msp.FabricOUIdentifier\0226\n\025" +
"orderer_ou_identifier\030\005 \001(\0132\027.msp.Fabric" +
"OUIdentifierBb\n!org.hyperledger.fabric.p" +
"rotos.mspB\020MspConfigPackageZ+github.com/" +
"hyperledger/fabric-protos-go/mspb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_msp_MSPConfig_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_msp_MSPConfig_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_MSPConfig_descriptor,
new java.lang.String[] { "Type", "Config", });
internal_static_msp_FabricMSPConfig_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_msp_FabricMSPConfig_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_FabricMSPConfig_descriptor,
new java.lang.String[] { "Name", "RootCerts", "IntermediateCerts", "Admins", "RevocationList", "SigningIdentity", "OrganizationalUnitIdentifiers", "CryptoConfig", "TlsRootCerts", "TlsIntermediateCerts", "FabricNodeOus", });
internal_static_msp_FabricCryptoConfig_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_msp_FabricCryptoConfig_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_FabricCryptoConfig_descriptor,
new java.lang.String[] { "SignatureHashFamily", "IdentityIdentifierHashFunction", });
internal_static_msp_IdemixMSPConfig_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_msp_IdemixMSPConfig_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_IdemixMSPConfig_descriptor,
new java.lang.String[] { "Name", "Ipk", "Signer", "RevocationPk", "Epoch", });
internal_static_msp_IdemixMSPSignerConfig_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_msp_IdemixMSPSignerConfig_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_IdemixMSPSignerConfig_descriptor,
new java.lang.String[] { "Cred", "Sk", "OrganizationalUnitIdentifier", "Role", "EnrollmentId", "CredentialRevocationInformation", });
internal_static_msp_SigningIdentityInfo_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_msp_SigningIdentityInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_SigningIdentityInfo_descriptor,
new java.lang.String[] { "PublicSigner", "PrivateSigner", });
internal_static_msp_KeyInfo_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_msp_KeyInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_KeyInfo_descriptor,
new java.lang.String[] { "KeyIdentifier", "KeyMaterial", });
internal_static_msp_FabricOUIdentifier_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_msp_FabricOUIdentifier_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_FabricOUIdentifier_descriptor,
new java.lang.String[] { "Certificate", "OrganizationalUnitIdentifier", });
internal_static_msp_FabricNodeOUs_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_msp_FabricNodeOUs_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_msp_FabricNodeOUs_descriptor,
new java.lang.String[] { "Enable", "ClientOuIdentifier", "PeerOuIdentifier", "AdminOuIdentifier", "OrdererOuIdentifier", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy