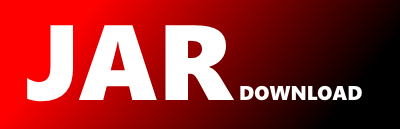
org.hyperledger.fabric.protos.gateway.GatewayGrpc Maven / Gradle / Ivy
package org.hyperledger.fabric.protos.gateway;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* The Gateway API for evaluating and submitting transactions via the gateway.
* Transaction evaluation (query) requires the invocation of the Evaluate service
* Transaction submission (ledger updates) is a two step process invoking Endorse
* followed by Submit. A third step, invoking CommitStatus, is required if the
* clients wish to wait for a Transaction to be committed.
* The proposal and transaction must be signed by the client before each step.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.0)",
comments = "Source: gateway/gateway.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class GatewayGrpc {
private GatewayGrpc() {}
public static final java.lang.String SERVICE_NAME = "gateway.Gateway";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getEndorseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Endorse",
requestType = org.hyperledger.fabric.protos.gateway.EndorseRequest.class,
responseType = org.hyperledger.fabric.protos.gateway.EndorseResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getEndorseMethod() {
io.grpc.MethodDescriptor getEndorseMethod;
if ((getEndorseMethod = GatewayGrpc.getEndorseMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getEndorseMethod = GatewayGrpc.getEndorseMethod) == null) {
GatewayGrpc.getEndorseMethod = getEndorseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Endorse"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.EndorseRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.EndorseResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("Endorse"))
.build();
}
}
}
return getEndorseMethod;
}
private static volatile io.grpc.MethodDescriptor getSubmitMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Submit",
requestType = org.hyperledger.fabric.protos.gateway.SubmitRequest.class,
responseType = org.hyperledger.fabric.protos.gateway.SubmitResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSubmitMethod() {
io.grpc.MethodDescriptor getSubmitMethod;
if ((getSubmitMethod = GatewayGrpc.getSubmitMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getSubmitMethod = GatewayGrpc.getSubmitMethod) == null) {
GatewayGrpc.getSubmitMethod = getSubmitMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Submit"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.SubmitRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.SubmitResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("Submit"))
.build();
}
}
}
return getSubmitMethod;
}
private static volatile io.grpc.MethodDescriptor getCommitStatusMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CommitStatus",
requestType = org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest.class,
responseType = org.hyperledger.fabric.protos.gateway.CommitStatusResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCommitStatusMethod() {
io.grpc.MethodDescriptor getCommitStatusMethod;
if ((getCommitStatusMethod = GatewayGrpc.getCommitStatusMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getCommitStatusMethod = GatewayGrpc.getCommitStatusMethod) == null) {
GatewayGrpc.getCommitStatusMethod = getCommitStatusMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CommitStatus"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.CommitStatusResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("CommitStatus"))
.build();
}
}
}
return getCommitStatusMethod;
}
private static volatile io.grpc.MethodDescriptor getEvaluateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Evaluate",
requestType = org.hyperledger.fabric.protos.gateway.EvaluateRequest.class,
responseType = org.hyperledger.fabric.protos.gateway.EvaluateResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getEvaluateMethod() {
io.grpc.MethodDescriptor getEvaluateMethod;
if ((getEvaluateMethod = GatewayGrpc.getEvaluateMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getEvaluateMethod = GatewayGrpc.getEvaluateMethod) == null) {
GatewayGrpc.getEvaluateMethod = getEvaluateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Evaluate"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.EvaluateRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.EvaluateResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("Evaluate"))
.build();
}
}
}
return getEvaluateMethod;
}
private static volatile io.grpc.MethodDescriptor getChaincodeEventsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ChaincodeEvents",
requestType = org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest.class,
responseType = org.hyperledger.fabric.protos.gateway.ChaincodeEventsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getChaincodeEventsMethod() {
io.grpc.MethodDescriptor getChaincodeEventsMethod;
if ((getChaincodeEventsMethod = GatewayGrpc.getChaincodeEventsMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getChaincodeEventsMethod = GatewayGrpc.getChaincodeEventsMethod) == null) {
GatewayGrpc.getChaincodeEventsMethod = getChaincodeEventsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ChaincodeEvents"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.gateway.ChaincodeEventsResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("ChaincodeEvents"))
.build();
}
}
}
return getChaincodeEventsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static GatewayStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GatewayStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayStub(channel, callOptions);
}
};
return GatewayStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static GatewayBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GatewayBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayBlockingStub(channel, callOptions);
}
};
return GatewayBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static GatewayFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GatewayFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayFutureStub(channel, callOptions);
}
};
return GatewayFutureStub.newStub(factory, channel);
}
/**
*
* The Gateway API for evaluating and submitting transactions via the gateway.
* Transaction evaluation (query) requires the invocation of the Evaluate service
* Transaction submission (ledger updates) is a two step process invoking Endorse
* followed by Submit. A third step, invoking CommitStatus, is required if the
* clients wish to wait for a Transaction to be committed.
* The proposal and transaction must be signed by the client before each step.
*
*/
public interface AsyncService {
/**
*
* The Endorse service passes a proposed transaction to the gateway in order to
* obtain sufficient endorsement.
* The gateway will determine the endorsement plan for the requested chaincode and
* forward to the appropriate peers for endorsement. It will return to the client a
* prepared transaction in the form of an Envelope message as defined
* in common/common.proto. The client must sign the contents of this envelope
* before invoking the Submit service.
*
*/
default void endorse(org.hyperledger.fabric.protos.gateway.EndorseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getEndorseMethod(), responseObserver);
}
/**
*
* The Submit service will process the prepared transaction returned from Endorse service
* once it has been signed by the client. It will wait for the transaction to be submitted to the
* ordering service but the client must invoke the CommitStatus service to wait for the transaction
* to be committed.
*
*/
default void submit(org.hyperledger.fabric.protos.gateway.SubmitRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSubmitMethod(), responseObserver);
}
/**
*
* The CommitStatus service will indicate whether a prepared transaction previously submitted to
* the Submit service has been committed. It will wait for the commit to occur if it hasn’t already
* committed.
*
*/
default void commitStatus(org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCommitStatusMethod(), responseObserver);
}
/**
*
* The Evaluate service passes a proposed transaction to the gateway in order to invoke the
* transaction function and return the result to the client. No ledger updates are made.
* The gateway will select an appropriate peer to query based on block height and load.
*
*/
default void evaluate(org.hyperledger.fabric.protos.gateway.EvaluateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getEvaluateMethod(), responseObserver);
}
/**
*
* The ChaincodeEvents service supplies a stream of responses, each containing all the events emitted by the
* requested chaincode for a specific block. The streamed responses are ordered by ascending block number. Responses
* are only returned for blocks that contain the requested events, while blocks not containing any of the requested
* events are skipped.
*
*/
default void chaincodeEvents(org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getChaincodeEventsMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service Gateway.
*
* The Gateway API for evaluating and submitting transactions via the gateway.
* Transaction evaluation (query) requires the invocation of the Evaluate service
* Transaction submission (ledger updates) is a two step process invoking Endorse
* followed by Submit. A third step, invoking CommitStatus, is required if the
* clients wish to wait for a Transaction to be committed.
* The proposal and transaction must be signed by the client before each step.
*
*/
public static abstract class GatewayImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return GatewayGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service Gateway.
*
* The Gateway API for evaluating and submitting transactions via the gateway.
* Transaction evaluation (query) requires the invocation of the Evaluate service
* Transaction submission (ledger updates) is a two step process invoking Endorse
* followed by Submit. A third step, invoking CommitStatus, is required if the
* clients wish to wait for a Transaction to be committed.
* The proposal and transaction must be signed by the client before each step.
*
*/
public static final class GatewayStub
extends io.grpc.stub.AbstractAsyncStub {
private GatewayStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GatewayStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayStub(channel, callOptions);
}
/**
*
* The Endorse service passes a proposed transaction to the gateway in order to
* obtain sufficient endorsement.
* The gateway will determine the endorsement plan for the requested chaincode and
* forward to the appropriate peers for endorsement. It will return to the client a
* prepared transaction in the form of an Envelope message as defined
* in common/common.proto. The client must sign the contents of this envelope
* before invoking the Submit service.
*
*/
public void endorse(org.hyperledger.fabric.protos.gateway.EndorseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getEndorseMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* The Submit service will process the prepared transaction returned from Endorse service
* once it has been signed by the client. It will wait for the transaction to be submitted to the
* ordering service but the client must invoke the CommitStatus service to wait for the transaction
* to be committed.
*
*/
public void submit(org.hyperledger.fabric.protos.gateway.SubmitRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSubmitMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* The CommitStatus service will indicate whether a prepared transaction previously submitted to
* the Submit service has been committed. It will wait for the commit to occur if it hasn’t already
* committed.
*
*/
public void commitStatus(org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCommitStatusMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* The Evaluate service passes a proposed transaction to the gateway in order to invoke the
* transaction function and return the result to the client. No ledger updates are made.
* The gateway will select an appropriate peer to query based on block height and load.
*
*/
public void evaluate(org.hyperledger.fabric.protos.gateway.EvaluateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getEvaluateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* The ChaincodeEvents service supplies a stream of responses, each containing all the events emitted by the
* requested chaincode for a specific block. The streamed responses are ordered by ascending block number. Responses
* are only returned for blocks that contain the requested events, while blocks not containing any of the requested
* events are skipped.
*
*/
public void chaincodeEvents(org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getChaincodeEventsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service Gateway.
*
* The Gateway API for evaluating and submitting transactions via the gateway.
* Transaction evaluation (query) requires the invocation of the Evaluate service
* Transaction submission (ledger updates) is a two step process invoking Endorse
* followed by Submit. A third step, invoking CommitStatus, is required if the
* clients wish to wait for a Transaction to be committed.
* The proposal and transaction must be signed by the client before each step.
*
*/
public static final class GatewayBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private GatewayBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GatewayBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayBlockingStub(channel, callOptions);
}
/**
*
* The Endorse service passes a proposed transaction to the gateway in order to
* obtain sufficient endorsement.
* The gateway will determine the endorsement plan for the requested chaincode and
* forward to the appropriate peers for endorsement. It will return to the client a
* prepared transaction in the form of an Envelope message as defined
* in common/common.proto. The client must sign the contents of this envelope
* before invoking the Submit service.
*
*/
public org.hyperledger.fabric.protos.gateway.EndorseResponse endorse(org.hyperledger.fabric.protos.gateway.EndorseRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getEndorseMethod(), getCallOptions(), request);
}
/**
*
* The Submit service will process the prepared transaction returned from Endorse service
* once it has been signed by the client. It will wait for the transaction to be submitted to the
* ordering service but the client must invoke the CommitStatus service to wait for the transaction
* to be committed.
*
*/
public org.hyperledger.fabric.protos.gateway.SubmitResponse submit(org.hyperledger.fabric.protos.gateway.SubmitRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSubmitMethod(), getCallOptions(), request);
}
/**
*
* The CommitStatus service will indicate whether a prepared transaction previously submitted to
* the Submit service has been committed. It will wait for the commit to occur if it hasn’t already
* committed.
*
*/
public org.hyperledger.fabric.protos.gateway.CommitStatusResponse commitStatus(org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCommitStatusMethod(), getCallOptions(), request);
}
/**
*
* The Evaluate service passes a proposed transaction to the gateway in order to invoke the
* transaction function and return the result to the client. No ledger updates are made.
* The gateway will select an appropriate peer to query based on block height and load.
*
*/
public org.hyperledger.fabric.protos.gateway.EvaluateResponse evaluate(org.hyperledger.fabric.protos.gateway.EvaluateRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getEvaluateMethod(), getCallOptions(), request);
}
/**
*
* The ChaincodeEvents service supplies a stream of responses, each containing all the events emitted by the
* requested chaincode for a specific block. The streamed responses are ordered by ascending block number. Responses
* are only returned for blocks that contain the requested events, while blocks not containing any of the requested
* events are skipped.
*
*/
public java.util.Iterator chaincodeEvents(
org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getChaincodeEventsMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service Gateway.
*
* The Gateway API for evaluating and submitting transactions via the gateway.
* Transaction evaluation (query) requires the invocation of the Evaluate service
* Transaction submission (ledger updates) is a two step process invoking Endorse
* followed by Submit. A third step, invoking CommitStatus, is required if the
* clients wish to wait for a Transaction to be committed.
* The proposal and transaction must be signed by the client before each step.
*
*/
public static final class GatewayFutureStub
extends io.grpc.stub.AbstractFutureStub {
private GatewayFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GatewayFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayFutureStub(channel, callOptions);
}
/**
*
* The Endorse service passes a proposed transaction to the gateway in order to
* obtain sufficient endorsement.
* The gateway will determine the endorsement plan for the requested chaincode and
* forward to the appropriate peers for endorsement. It will return to the client a
* prepared transaction in the form of an Envelope message as defined
* in common/common.proto. The client must sign the contents of this envelope
* before invoking the Submit service.
*
*/
public com.google.common.util.concurrent.ListenableFuture endorse(
org.hyperledger.fabric.protos.gateway.EndorseRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getEndorseMethod(), getCallOptions()), request);
}
/**
*
* The Submit service will process the prepared transaction returned from Endorse service
* once it has been signed by the client. It will wait for the transaction to be submitted to the
* ordering service but the client must invoke the CommitStatus service to wait for the transaction
* to be committed.
*
*/
public com.google.common.util.concurrent.ListenableFuture submit(
org.hyperledger.fabric.protos.gateway.SubmitRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSubmitMethod(), getCallOptions()), request);
}
/**
*
* The CommitStatus service will indicate whether a prepared transaction previously submitted to
* the Submit service has been committed. It will wait for the commit to occur if it hasn’t already
* committed.
*
*/
public com.google.common.util.concurrent.ListenableFuture commitStatus(
org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCommitStatusMethod(), getCallOptions()), request);
}
/**
*
* The Evaluate service passes a proposed transaction to the gateway in order to invoke the
* transaction function and return the result to the client. No ledger updates are made.
* The gateway will select an appropriate peer to query based on block height and load.
*
*/
public com.google.common.util.concurrent.ListenableFuture evaluate(
org.hyperledger.fabric.protos.gateway.EvaluateRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getEvaluateMethod(), getCallOptions()), request);
}
}
private static final int METHODID_ENDORSE = 0;
private static final int METHODID_SUBMIT = 1;
private static final int METHODID_COMMIT_STATUS = 2;
private static final int METHODID_EVALUATE = 3;
private static final int METHODID_CHAINCODE_EVENTS = 4;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_ENDORSE:
serviceImpl.endorse((org.hyperledger.fabric.protos.gateway.EndorseRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SUBMIT:
serviceImpl.submit((org.hyperledger.fabric.protos.gateway.SubmitRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_COMMIT_STATUS:
serviceImpl.commitStatus((org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EVALUATE:
serviceImpl.evaluate((org.hyperledger.fabric.protos.gateway.EvaluateRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CHAINCODE_EVENTS:
serviceImpl.chaincodeEvents((org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getEndorseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.gateway.EndorseRequest,
org.hyperledger.fabric.protos.gateway.EndorseResponse>(
service, METHODID_ENDORSE)))
.addMethod(
getSubmitMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.gateway.SubmitRequest,
org.hyperledger.fabric.protos.gateway.SubmitResponse>(
service, METHODID_SUBMIT)))
.addMethod(
getCommitStatusMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.gateway.SignedCommitStatusRequest,
org.hyperledger.fabric.protos.gateway.CommitStatusResponse>(
service, METHODID_COMMIT_STATUS)))
.addMethod(
getEvaluateMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.gateway.EvaluateRequest,
org.hyperledger.fabric.protos.gateway.EvaluateResponse>(
service, METHODID_EVALUATE)))
.addMethod(
getChaincodeEventsMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
org.hyperledger.fabric.protos.gateway.SignedChaincodeEventsRequest,
org.hyperledger.fabric.protos.gateway.ChaincodeEventsResponse>(
service, METHODID_CHAINCODE_EVENTS)))
.build();
}
private static abstract class GatewayBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
GatewayBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return org.hyperledger.fabric.protos.gateway.GatewayProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Gateway");
}
}
private static final class GatewayFileDescriptorSupplier
extends GatewayBaseDescriptorSupplier {
GatewayFileDescriptorSupplier() {}
}
private static final class GatewayMethodDescriptorSupplier
extends GatewayBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
GatewayMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (GatewayGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new GatewayFileDescriptorSupplier())
.addMethod(getEndorseMethod())
.addMethod(getSubmitMethod())
.addMethod(getCommitStatusMethod())
.addMethod(getEvaluateMethod())
.addMethod(getChaincodeEventsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy