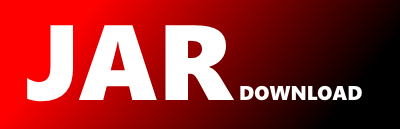
org.hyperledger.fabric.protos.peer.SnapshotGrpc Maven / Gradle / Ivy
package org.hyperledger.fabric.protos.peer;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.0)",
comments = "Source: peer/snapshot.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class SnapshotGrpc {
private SnapshotGrpc() {}
public static final java.lang.String SERVICE_NAME = "protos.Snapshot";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getGenerateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Generate",
requestType = org.hyperledger.fabric.protos.peer.SignedSnapshotRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGenerateMethod() {
io.grpc.MethodDescriptor getGenerateMethod;
if ((getGenerateMethod = SnapshotGrpc.getGenerateMethod) == null) {
synchronized (SnapshotGrpc.class) {
if ((getGenerateMethod = SnapshotGrpc.getGenerateMethod) == null) {
SnapshotGrpc.getGenerateMethod = getGenerateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Generate"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new SnapshotMethodDescriptorSupplier("Generate"))
.build();
}
}
}
return getGenerateMethod;
}
private static volatile io.grpc.MethodDescriptor getCancelMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Cancel",
requestType = org.hyperledger.fabric.protos.peer.SignedSnapshotRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCancelMethod() {
io.grpc.MethodDescriptor getCancelMethod;
if ((getCancelMethod = SnapshotGrpc.getCancelMethod) == null) {
synchronized (SnapshotGrpc.class) {
if ((getCancelMethod = SnapshotGrpc.getCancelMethod) == null) {
SnapshotGrpc.getCancelMethod = getCancelMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Cancel"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new SnapshotMethodDescriptorSupplier("Cancel"))
.build();
}
}
}
return getCancelMethod;
}
private static volatile io.grpc.MethodDescriptor getQueryPendingsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "QueryPendings",
requestType = org.hyperledger.fabric.protos.peer.SignedSnapshotRequest.class,
responseType = org.hyperledger.fabric.protos.peer.QueryPendingSnapshotsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getQueryPendingsMethod() {
io.grpc.MethodDescriptor getQueryPendingsMethod;
if ((getQueryPendingsMethod = SnapshotGrpc.getQueryPendingsMethod) == null) {
synchronized (SnapshotGrpc.class) {
if ((getQueryPendingsMethod = SnapshotGrpc.getQueryPendingsMethod) == null) {
SnapshotGrpc.getQueryPendingsMethod = getQueryPendingsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "QueryPendings"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.hyperledger.fabric.protos.peer.QueryPendingSnapshotsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SnapshotMethodDescriptorSupplier("QueryPendings"))
.build();
}
}
}
return getQueryPendingsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static SnapshotStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SnapshotStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SnapshotStub(channel, callOptions);
}
};
return SnapshotStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SnapshotBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SnapshotBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SnapshotBlockingStub(channel, callOptions);
}
};
return SnapshotBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static SnapshotFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SnapshotFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SnapshotFutureStub(channel, callOptions);
}
};
return SnapshotFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*
* Generate a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
default void generate(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGenerateMethod(), responseObserver);
}
/**
*
* Cancel a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
default void cancel(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCancelMethod(), responseObserver);
}
/**
*
* Query pending snapshots query. SignedSnapshotRequest contains marshalled bytes for SnaphostQuery
*
*/
default void queryPendings(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getQueryPendingsMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service Snapshot.
*/
public static abstract class SnapshotImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return SnapshotGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service Snapshot.
*/
public static final class SnapshotStub
extends io.grpc.stub.AbstractAsyncStub {
private SnapshotStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SnapshotStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SnapshotStub(channel, callOptions);
}
/**
*
* Generate a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
public void generate(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGenerateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Cancel a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
public void cancel(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCancelMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Query pending snapshots query. SignedSnapshotRequest contains marshalled bytes for SnaphostQuery
*
*/
public void queryPendings(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getQueryPendingsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service Snapshot.
*/
public static final class SnapshotBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private SnapshotBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SnapshotBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SnapshotBlockingStub(channel, callOptions);
}
/**
*
* Generate a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
public com.google.protobuf.Empty generate(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGenerateMethod(), getCallOptions(), request);
}
/**
*
* Cancel a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
public com.google.protobuf.Empty cancel(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCancelMethod(), getCallOptions(), request);
}
/**
*
* Query pending snapshots query. SignedSnapshotRequest contains marshalled bytes for SnaphostQuery
*
*/
public org.hyperledger.fabric.protos.peer.QueryPendingSnapshotsResponse queryPendings(org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getQueryPendingsMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service Snapshot.
*/
public static final class SnapshotFutureStub
extends io.grpc.stub.AbstractFutureStub {
private SnapshotFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SnapshotFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SnapshotFutureStub(channel, callOptions);
}
/**
*
* Generate a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
public com.google.common.util.concurrent.ListenableFuture generate(
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGenerateMethod(), getCallOptions()), request);
}
/**
*
* Cancel a snapshot reqeust. SignedSnapshotRequest contains marshalled bytes for SnaphostRequest
*
*/
public com.google.common.util.concurrent.ListenableFuture cancel(
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCancelMethod(), getCallOptions()), request);
}
/**
*
* Query pending snapshots query. SignedSnapshotRequest contains marshalled bytes for SnaphostQuery
*
*/
public com.google.common.util.concurrent.ListenableFuture queryPendings(
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getQueryPendingsMethod(), getCallOptions()), request);
}
}
private static final int METHODID_GENERATE = 0;
private static final int METHODID_CANCEL = 1;
private static final int METHODID_QUERY_PENDINGS = 2;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_GENERATE:
serviceImpl.generate((org.hyperledger.fabric.protos.peer.SignedSnapshotRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CANCEL:
serviceImpl.cancel((org.hyperledger.fabric.protos.peer.SignedSnapshotRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_QUERY_PENDINGS:
serviceImpl.queryPendings((org.hyperledger.fabric.protos.peer.SignedSnapshotRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getGenerateMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest,
com.google.protobuf.Empty>(
service, METHODID_GENERATE)))
.addMethod(
getCancelMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest,
com.google.protobuf.Empty>(
service, METHODID_CANCEL)))
.addMethod(
getQueryPendingsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.hyperledger.fabric.protos.peer.SignedSnapshotRequest,
org.hyperledger.fabric.protos.peer.QueryPendingSnapshotsResponse>(
service, METHODID_QUERY_PENDINGS)))
.build();
}
private static abstract class SnapshotBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
SnapshotBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return org.hyperledger.fabric.protos.peer.SnapshotProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Snapshot");
}
}
private static final class SnapshotFileDescriptorSupplier
extends SnapshotBaseDescriptorSupplier {
SnapshotFileDescriptorSupplier() {}
}
private static final class SnapshotMethodDescriptorSupplier
extends SnapshotBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
SnapshotMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (SnapshotGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new SnapshotFileDescriptorSupplier())
.addMethod(getGenerateMethod())
.addMethod(getCancelMethod())
.addMethod(getQueryPendingsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy